A C++ reference variable is an alias for another variable, allowing you to manipulate the original variable without using pointers.
Here’s a simple code snippet that demonstrates the use of a reference variable:
#include <iostream>
using namespace std;
int main() {
int original = 10; // Original variable
int &ref = original; // Reference variable
cout << "Original: " << original << endl; // Outputs 10
ref = 20; // Modifies the original variable through the reference
cout << "Original after change: " << original << endl; // Outputs 20
return 0;
}
What is a Reference Variable in C++?
A C++ reference variable is essentially an alias for another variable. When you declare a reference variable, you are instructing the compiler to treat this new variable as a reference to an existing variable in memory. The key point is that a reference does not occupy storage of its own; instead, it acts like a new name for an already declared variable.
Understanding the difference between a reference and a pointer is crucial. While a pointer holds the address of another variable and can be reassigned, a reference variable always refers to a single variable, and once it is set, it cannot be changed to refer to another variable. This immutability is one of the key advantages of using reference variables in C++.
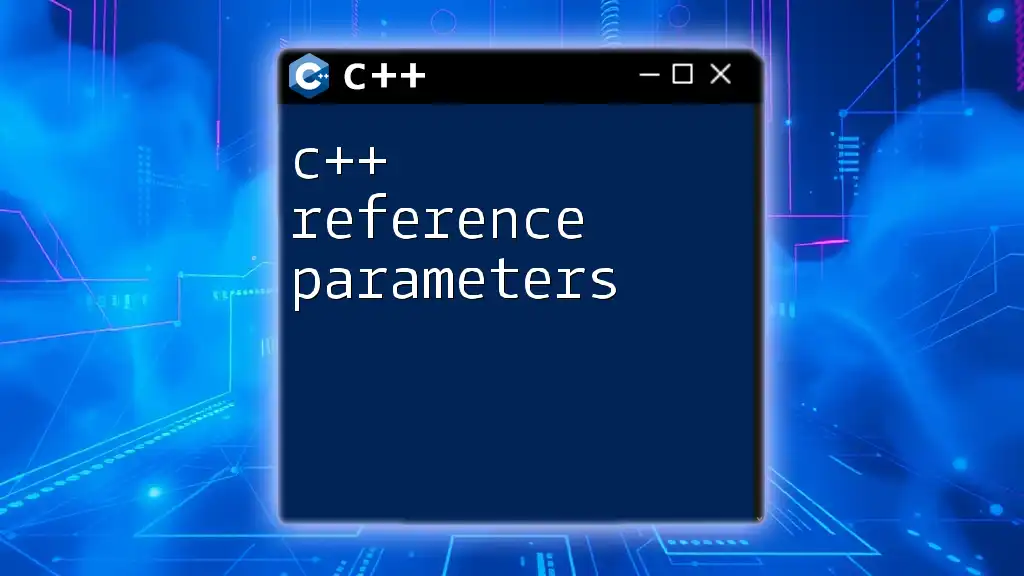
How to Declare a Reference Variable in C++
The syntax for declaring a reference variable follows the format:
type &referenceName = originalVariable;
Here’s a basic example:
int original = 10;
int &ref = original; // ref is a reference to original
In this code, `ref` becomes an alias for `original`. Any changes made to `ref` will directly affect `original`, illustrating one of the most powerful features of reference variables.
Key Characteristics of Reference Variables
- Memory Efficiency: Since they act as aliases, reference variables do not require additional memory allocation.
- Automatic Dereferencing: You do not need to use the dereference operator `*` as you do with pointers.
- Immutability: Once created, a reference variable cannot be changed to refer to another variable.
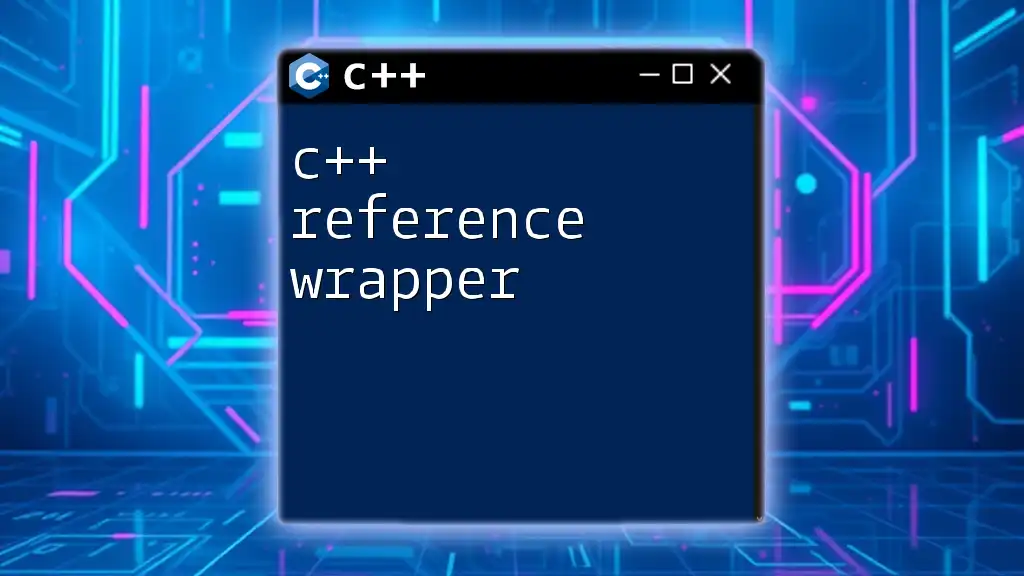
Understanding C++ References
C++ references serve as an important feature of the language. Like pointers, they allow developers to work with the same data in multiple places. However, references offer a cleaner, more intuitive syntax.
The difference between references and pointers lies in their behavior. A pointer can point to any variable of the same type and can be reassigned to point to different variables. In contrast, a reference, after being initialized, cannot change its association.
Example: If you wanted to swap the values of two integers using pointers, you would need to manage pointer dereferencing manually. With references, the syntax is far less cumbersome.
void swap(int &x, int &y) {
int temp = x;
x = y;
y = temp;
}
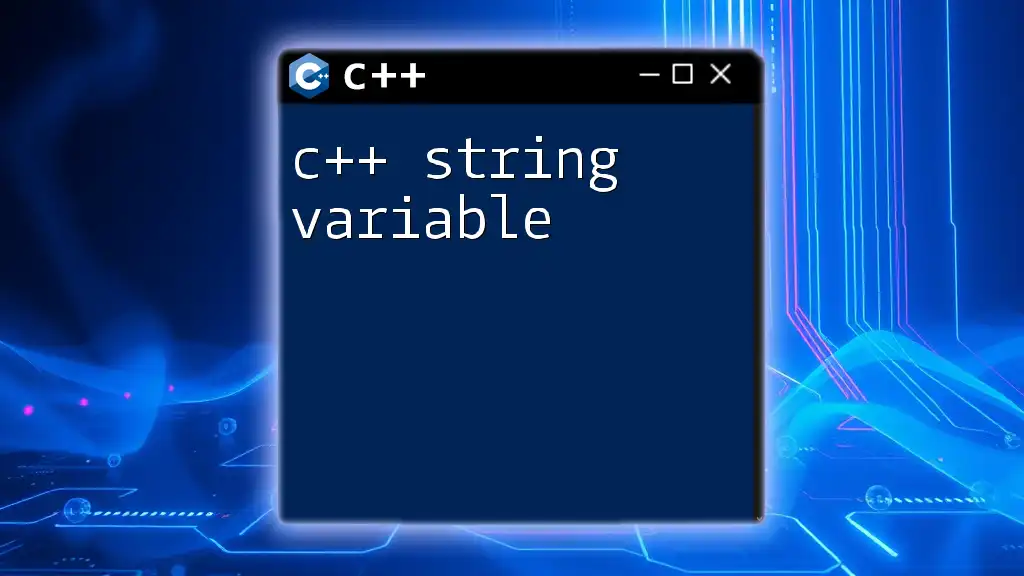
The Role of Reference Variables in Function Parameters
Using reference variables in function parameters allows for efficient data manipulation. When you pass a variable by reference, you provide the function with direct access to the original variable, which can improve performance by avoiding unnecessary copies.
Here’s an example comparing passing by value versus passing by reference:
void byValue(int num) {
num = 20; // This modifies only the copy
}
void byReference(int &num) {
num = 20; // This modifies the original variable
}
int main() {
int x = 10;
byValue(x); // x remains 10
byReference(x); // x changes to 20
}
By using reference variables, the function `byReference` directly modifies `x`, while `byValue` only modifies its local copy.
Advantages of Using Reference Variables in Function Parameters
- Avoiding Copies: Especially for large objects, passing by reference can significantly speed up performance.
- Ease of Use: Reference variables eliminate the need for dereferencing, leading to cleaner code.
- Direct Interaction with Original Variables: This allows functions to modify caller variables transparently.
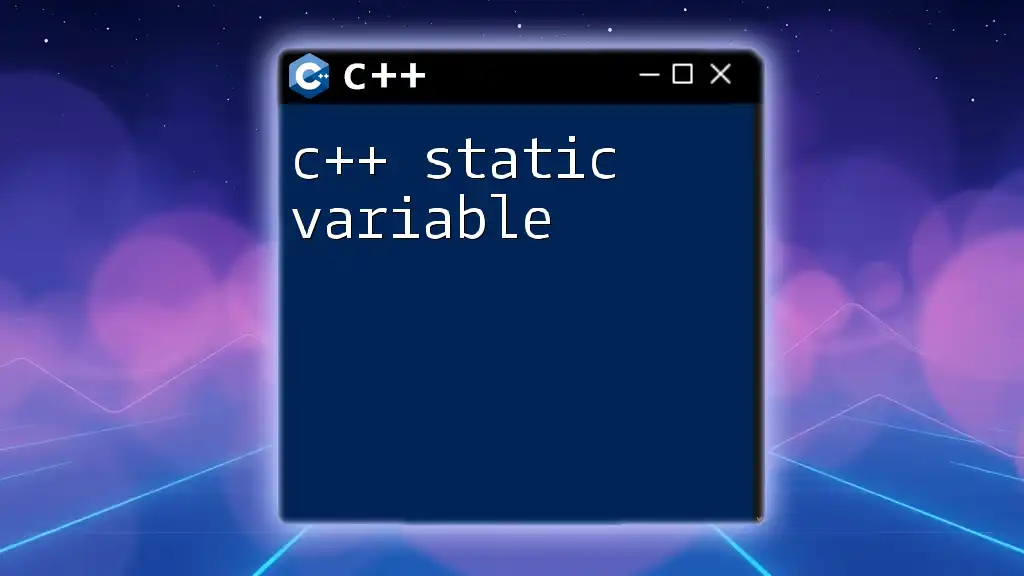
Modifying Variables with Reference Variables
One of the most noticeable features of reference variables is their ability to change values in the original variable. For instance, if you want to manipulate a variable in a function and directly reflect those changes in the calling context, reference variables are your best bet.
Here’s an illustrative code example:
void increment(int &ref) {
ref++; // Increment the original variable
}
int main() {
int num = 5;
increment(num); // num now is 6
}
In this case, calling `increment(num)` modifies the original `num` directly since `ref` is a reference pointing to it.
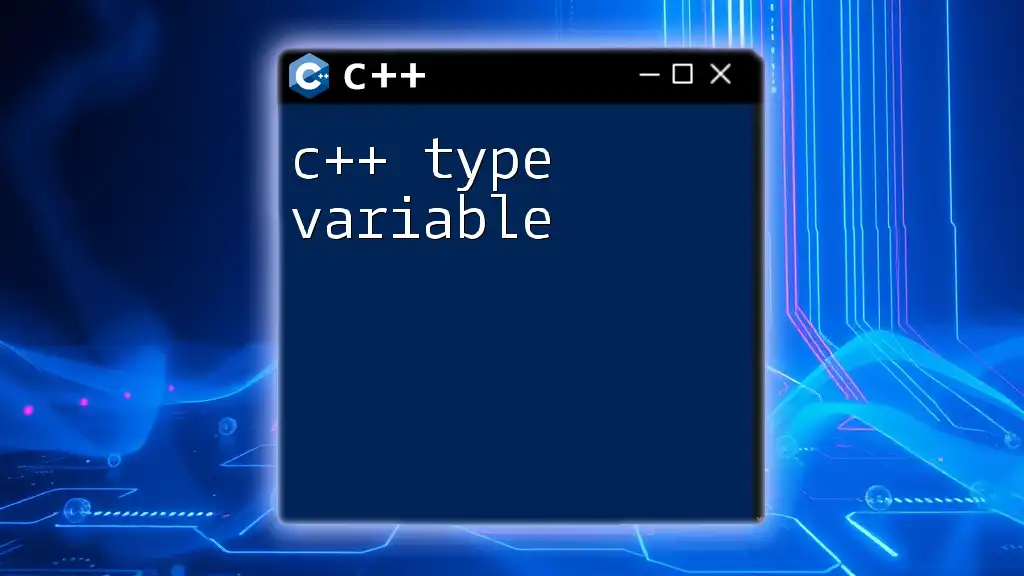
Using Constant Reference Variables
Constant reference variables are used when you want to prevent a function from altering the original data. Declaring a reference as `const` indicates that it cannot be modified, thus providing safety against unintentional changes.
The syntax is as follows:
const type &referenceName = originalVariable;
Here’s a practical example for clarity:
void printValue(const int &num) {
// num cannot be modified here
std::cout << num << std::endl;
}
Benefits of Constant References:
- Read-Only Access: The original variable remains unchanged.
- Efficiency: Similar to regular references, const references prevent unnecessary copying while ensuring data integrity.
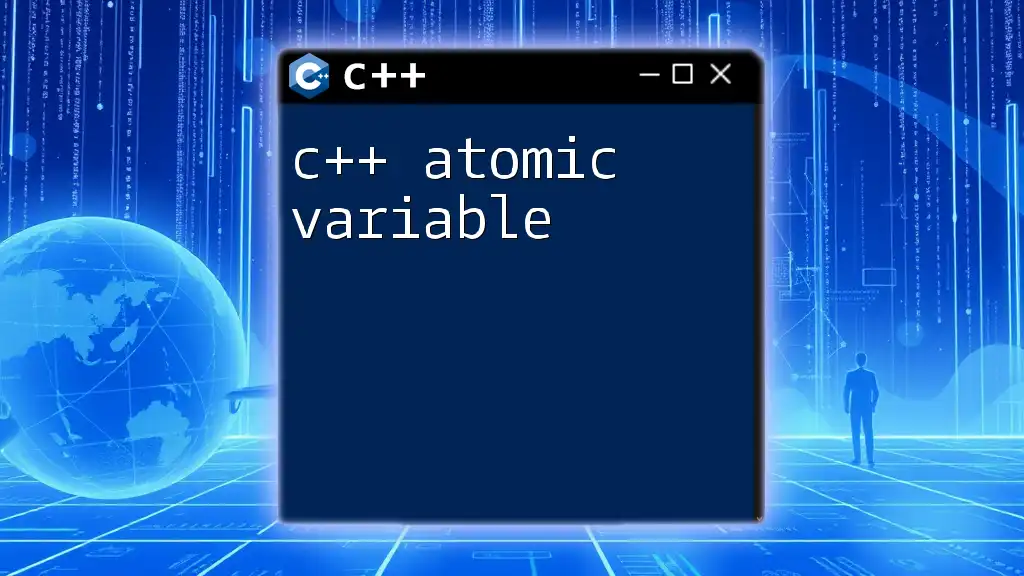
Reference Variables and C++ Standard Library
C++ standard library components often leverage reference variables for efficient memory usage and ease of manipulation. For example, when working with STL (Standard Template Library) containers, many operations utilize references for performance optimizations.
Consider a scenario where you retrieve values from a vector:
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec = {1, 2, 3};
for (int &value : vec) {
value *= 2; // Modify values directly
}
for (const int &value : vec) {
std::cout << value << " "; // Outputs 2 4 6
}
}
In this example, the first loop modifies each element of the `vector` directly via reference, while the second loop uses constant references to simply read the values without modifying them.
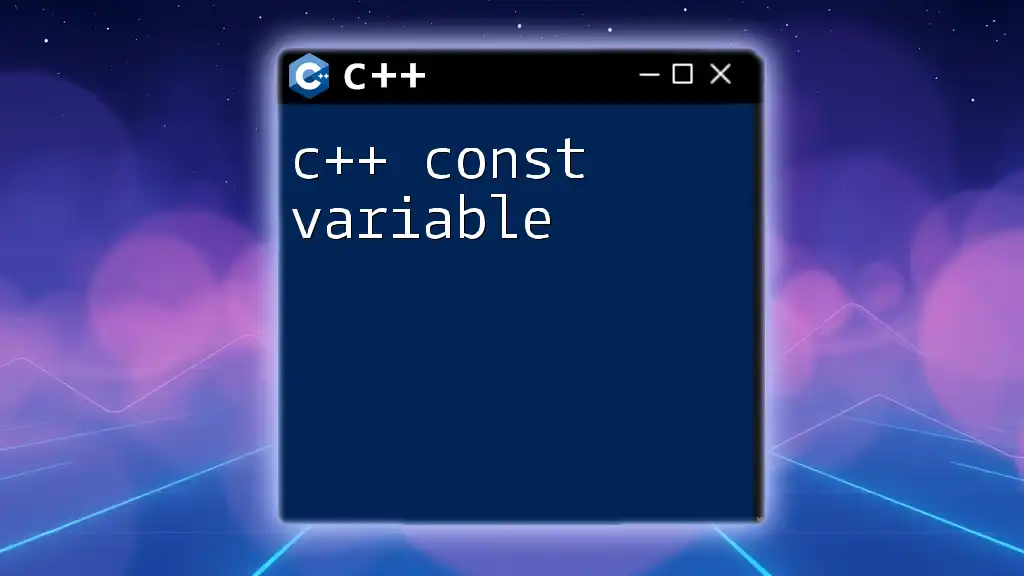
Common Pitfalls and Best Practices
While reference variables offer numerous advantages, they are not without their challenges. Common mistakes include:
- Uninitialized References: Always ensure reference variables are initialized upon declaration; failing to do so can lead to undefined behavior.
- Using in Class Members: When designing classes, avoid holding references as member variables unless absolutely necessary, as lifecycle management becomes complex.
Best Practices:
- Use reference variables where ownership does not need to be transferred.
- Prefer constant reference parameters for functions that do not modify input data.
- Use clear naming conventions to distinguish between regular and reference variables, improving code readability.
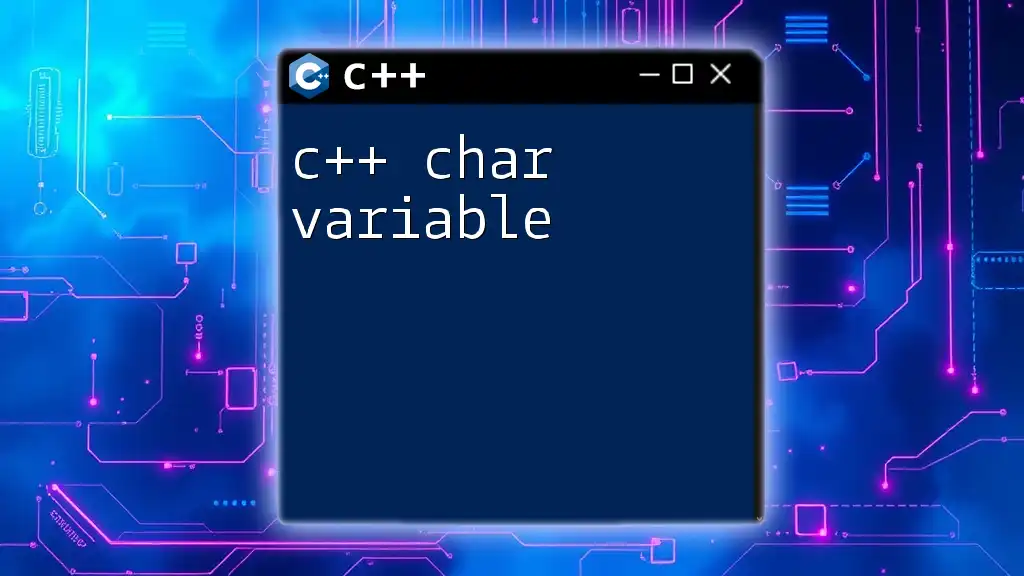
Conclusion
C++ reference variables play a pivotal role in programming, allowing developers to write cleaner, more efficient code. By providing a simple syntax for accessing and modifying variables, they transcend some challenges associated with pointers while enhancing performance through efficient data management. Understanding and effectively utilizing these references is essential for mastering C++ programming.
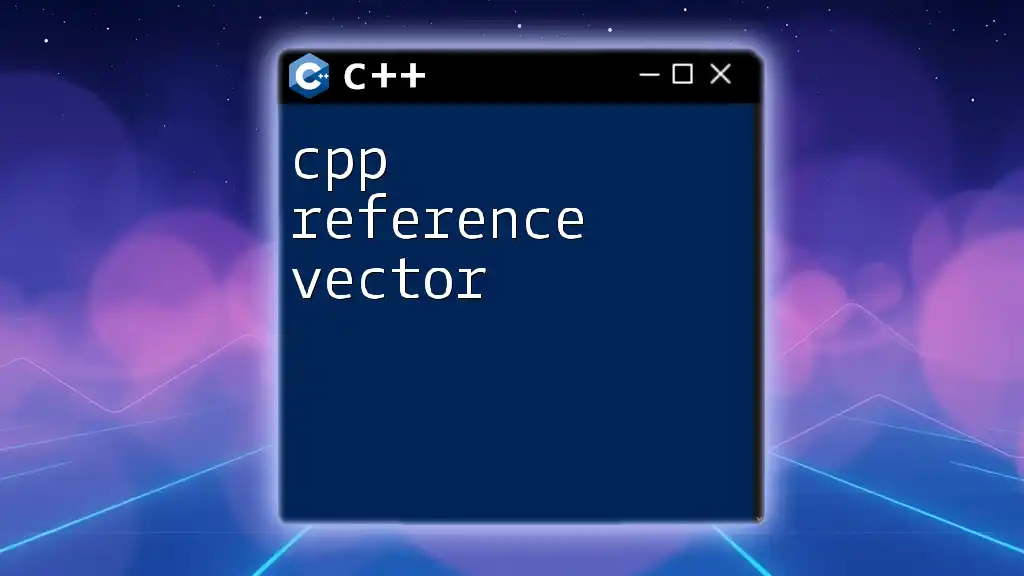
Additional Resources
Exploring further, you may find value in:
- Recommended books such as "The C++ Programming Language" by Bjarne Stroustrup.
- Online courses that focus on advanced C++ techniques.
- Online communities and forums where you can share ideas and learn from others in the C++ field, providing a robust environment for continual learning.
Feel free to check links to official C++ documentation for more detailed explanations and learning resources!