In C++, a string variable is used to represent a sequence of characters and is commonly defined using the `std::string` class, which allows for easy string manipulation and operations.
Here’s an example code snippet:
#include <iostream>
#include <string>
int main() {
std::string greeting = "Hello, World!";
std::cout << greeting << std::endl;
return 0;
}
Understanding Strings in C++
What is a String?
In C++, a string is a sequence of characters used to represent text. Strings are crucial in programming as they allow developers to manipulate text, which is essential in applications such as user interfaces, data processing, and file handling. Unlike character arrays, which are raw data, strings provide a higher-level abstraction making string manipulation easier and more intuitive.
String Library
C++ offers a powerful Standard Library that includes the string class, allowing for convenient string manipulation. To utilize this feature, you must include the string library at the beginning of your program like so:
#include <string>
By doing this, you can take full advantage of the functionalities provided by the string class.
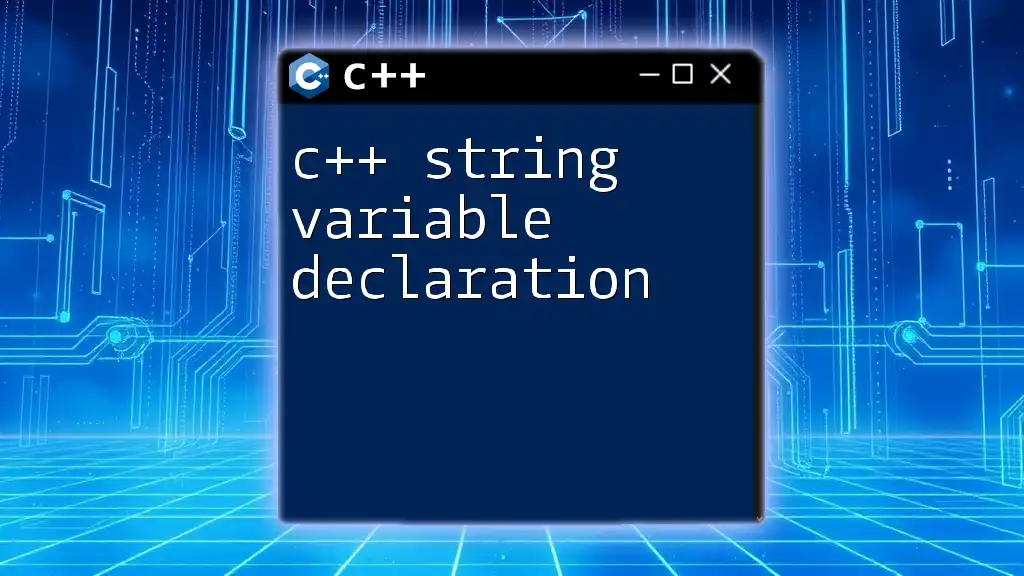
Declaring and Initializing String Variables
Declaring Strings
Declaring a string variable in C++ is straightforward. You simply specify the type as `std::string`, followed by the variable name:
std::string myString;
Initializing Strings
You can initialize a string in several ways. The most common method is during declaration:
std::string myString = "Hello, World!";
You can also initialize a string after its declaration with an assignment:
myString = "Another String!";
Using String Literals
String literals in C++ represent fixed strings. They are defined by enclosing the text in double quotes, making them easy to use in your programs. For example:
std::string greeting = "Hello, Universe!";
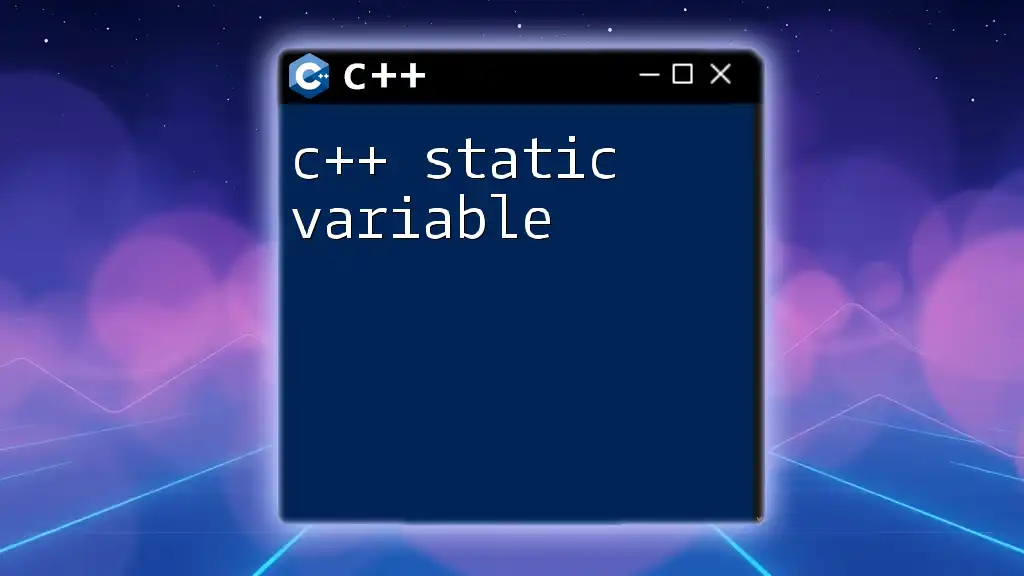
Common String Operations
String Length
Determining the length of a string is crucial in many applications. You can obtain the length using either the `.length()` method or the `.size()` method. They perform the same function, returning the number of characters in the string:
size_t length = myString.length();
Concatenation
Combining two or more strings, known as concatenation, is simple in C++. You can use the `+` operator or the `.append()` method to achieve this:
std::string fullGreeting = myString + " Welcome!";
This results in the string "Hello, World! Welcome!".
Accessing Characters
C++ allows you to access individual characters in a string using the `[]` operator or the `.at()` method. Both methods can be used as follows:
char firstChar = myString[0]; // Accesses the first character 'H'
char secondChar = myString.at(1); // Accesses the second character 'e'
Substrings
Creating a substring from an existing string is simple using the `.substr()` method. This method takes two parameters: the starting index and the length of the substring.
std::string sub = myString.substr(0, 5); // Returns "Hello"
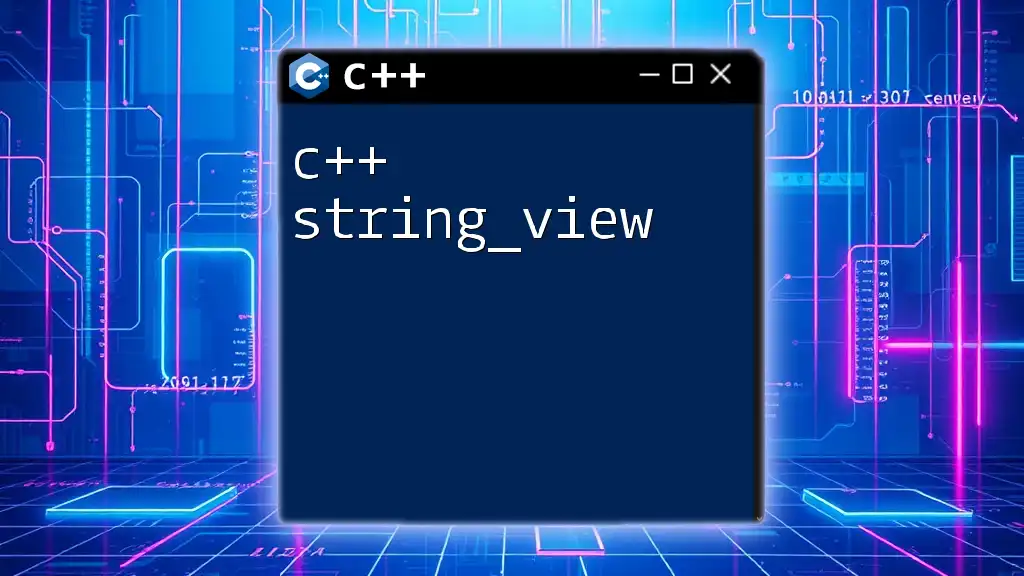
Modifying Strings
Changing Characters
You can modify specific characters in a string easily. For example, to change the first character of `myString` from 'H' to 'h', you could write:
myString[0] = 'h'; // myString is now "hello, World!"
Inserting and Erasing
C++ provides methods like `.insert()` and `.erase()` for more flexible string manipulation. For instance, you can insert a substring at any position:
myString.insert(5, " beautiful"); // Adds " beautiful" after "hello"
Similarly, you can erase a portion of the string:
myString.erase(0, 6); // Removes "hello"
Replacing Substrings
You can replace parts of a string using the `.replace()` method. This allows you to specify the start index, how many characters to replace, and the new string:
myString.replace(7, 9, "C++"); // Replaces "beautiful" with "C++"
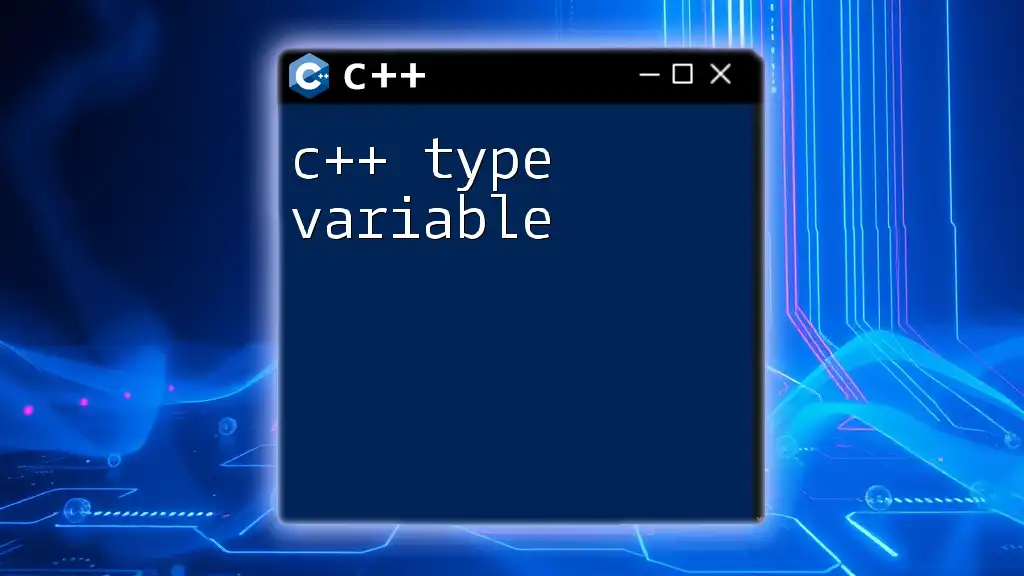
String Comparisons
Comparing Strings
C++ allows for string comparisons using relational operators or the `.compare()` method. The comparison returns `0` if strings are equal, a negative number if the first string is less, and a positive number if the first string is greater:
if (myString.compare("C++") == 0) {
std::cout << "Strings are equal!";
}
Case-Insensitive Comparison
To perform a case-insensitive comparison, you can leverage the `std::equal()` function in conjunction with `std::tolower()`. This allows for more flexible string checking.
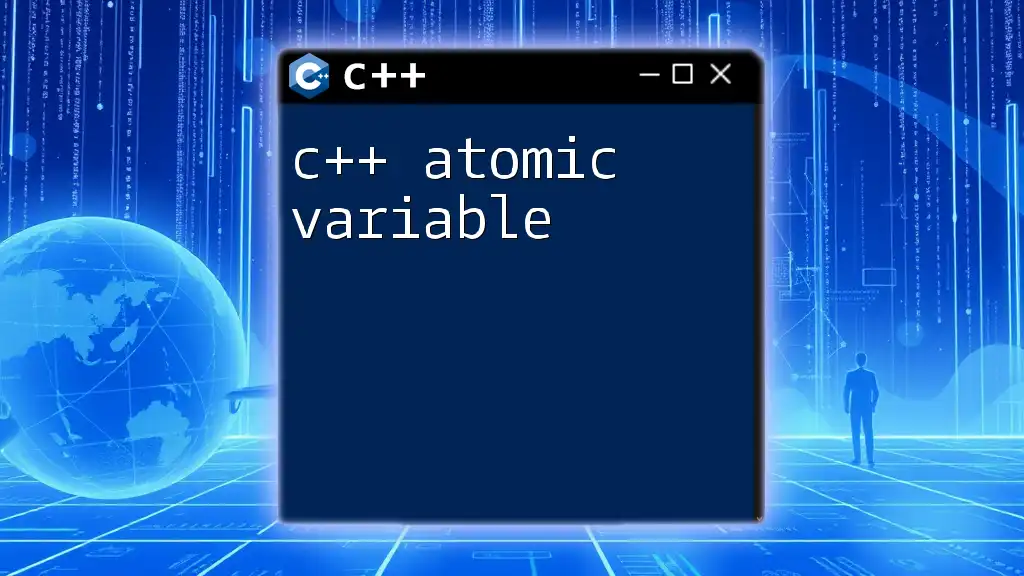
Advanced String Features
String Streams
For more advanced usage, the `std::stringstream` class allows for reading from and writing to strings. This is particularly useful for data formatting and conversions:
std::stringstream ss;
ss << "Number: " << 42;
String and Iterators
You can also utilize iterators to traverse through the characters of a string. This method is useful when you need to process each character in a more controlled manner:
for (std::string::iterator it = myString.begin(); it != myString.end(); ++it) {
std::cout << *it; // Prints each character
}
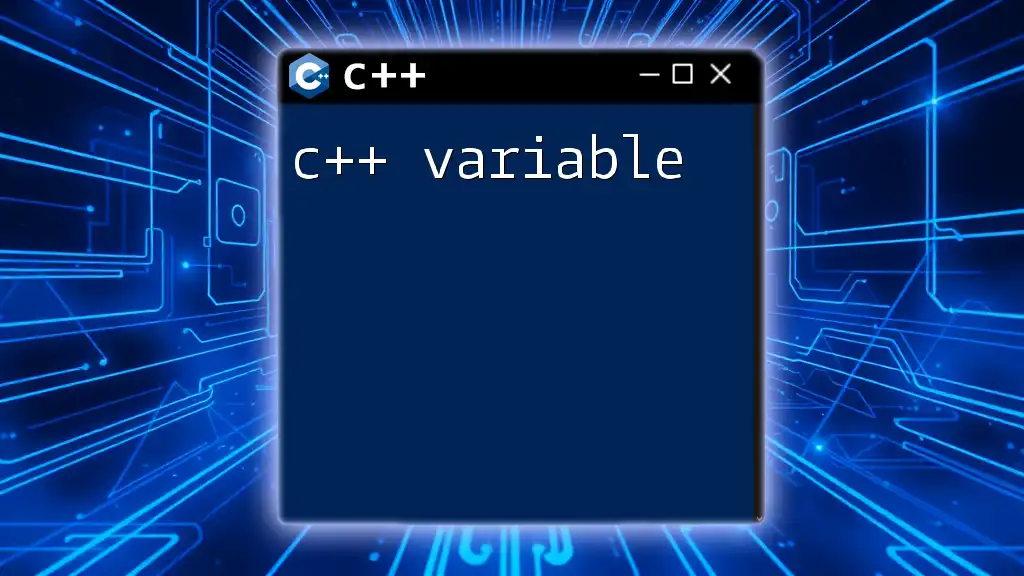
Common Pitfalls and Best Practices
Memory Management
One of the significant advantages of `std::string` is that it performs automatic memory management. However, it’s still essential to be cautious about memory usage, particularly when handling large strings or in performance-critical applications.
Performance Considerations
When dealing with string operations, always consider the performance implications. Avoid unnecessary copies, especially in loops, and opt for references where possible. Utilizing the `.reserve()` method can also help pre-allocate memory if you know the size of the string in advance, improving efficiency.
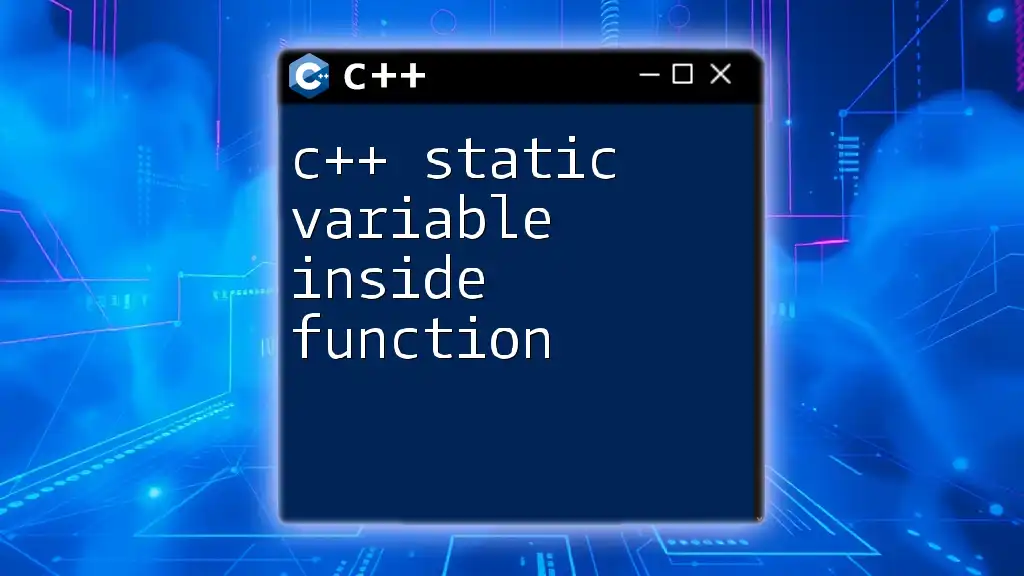
Conclusion
In summary, understanding the `c++ string variable` is fundamental for effective programming in C++. From declaring and initializing strings to advanced manipulation, the string class in C++ provides a comprehensive toolkit for handling text. Through practice and exploration of these features, you can greatly enhance your programming skills and application development.