A static variable inside a function maintains its value between function calls and is initialized only once, allowing for the preservation of state information.
#include <iostream>
void countCalls() {
static int callCount = 0; // Initialized only once
callCount++;
std::cout << "Function called " << callCount << " times." << std::endl;
}
int main() {
countCalls(); // Output: Function called 1 times.
countCalls(); // Output: Function called 2 times.
countCalls(); // Output: Function called 3 times.
return 0;
}
What is a Static Variable?
In C++, a static variable is one that retains its value across function calls and exists for the entire duration of the program. Unlike regular local variables whose lifetime is confined to the function scope, static variables are initialized only once, when the function is first executed. This means that even after the function exits, the static variable holds onto its last value, ready to be used the next time the function is called.
Differences Between Static and Non-static Variables
- Lifetime: A static variable persists for the duration of the program, while a non-static local variable is created upon function entry and destroyed upon exit.
- Scope: A static variable defined within a function is only accessible within that function. Conversely, non-static variables are also limited to the function scope but do not retain values after the function exits.

Understanding Static Variable Inside Functions
What Does It Mean to Have Static Variables in Functions?
When you declare a static variable inside a function, it means that the variable will maintain its state across multiple invocations of that function. This can be incredibly useful for certain types of tasks, such as counting the number of times a function has been called or caching results of expensive computations.
Lifecycle of a Static Variable
The lifecycle of a static variable is an essential aspect to understand. Once declared and initialized, a static variable is created in the data segment of the program memory and retains its value without being reinitialized on each function call.
Consider the following code snippet:
#include <iostream>
void demoFunction() {
static int numCalls = 0; // Initialized once
numCalls++;
std::cout << "Function called " << numCalls << " times." << std::endl;
}
When `demoFunction()` is called multiple times, `numCalls` will continue to hold its incremented value, demonstrating its ability to persist beyond the function's scope.
Memory Allocation for Static Variables
Static variables are stored in the data segment of the program's memory, rather than on the stack which is used for regular local variables. This distinction is crucial as it impacts the efficiency and the behavior of the program.
- Static Variables: Retained in memory for the lifespan of the program.
- Local Variables: Created and destroyed during each function call.

Syntax of Static Variables in C++
Basic Syntax
The syntax for declaring a static variable inside a function is straightforward. You simply precede the variable declaration with the `static` keyword:
static <data_type> <variable_name>;
Practical Example
One common application of static variables is to keep track of function calls:
#include <iostream>
void countCalls() {
static int callCount = 0; // Static variable initialized only once
callCount++;
std::cout << "Function has been called " << callCount << " times." << std::endl;
}
int main() {
countCalls(); // Output: Function has been called 1 times.
countCalls(); // Output: Function has been called 2 times.
countCalls(); // Output: Function has been called 3 times.
return 0;
}
In this example, the variable `callCount` retains its value across multiple calls to `countCalls()`, illustrating the behavior of a C++ static variable inside a function.

Advantages of Using Static Variables in Functions
Memory Efficiency
Using static variables can often be a more memory-efficient solution than global variables. Since static variables are limited to the function's scope, they reduce the risk of unintended modifications from outside the function and help manage memory more effectively.
Control Over Variable Visibility
Static variables provide precise control over variable visibility, allowing for encapsulation of data within a function scope while still retaining state.
Use Cases for Static Function Variables
Static variables can be beneficial in several scenarios:
- Cumulatively Counting Events: As demonstrated, use static variables to keep a running total, like counts of function calls.
- Caching or Storing Intermediate Results: For expensive calculations, static variables can store previous results for quick access.

Common Misconceptions About Static Variables
Static vs Global Variables
While both types of variables can last for the entirety of the program, static variables are confined to the function where they are declared, while global variables are accessible from any part of the program. This localizes state management, reducing potential bugs related to variable access.
Static Variables in Multi-threaded Environments
Static variables are not inherently thread-safe. In a multi-threaded application where multiple threads may call a function with a static variable, it can lead to race conditions. Consequently, proper synchronization measures, such as mutexes, should be implemented if the static variable is accessed simultaneously by multiple threads.
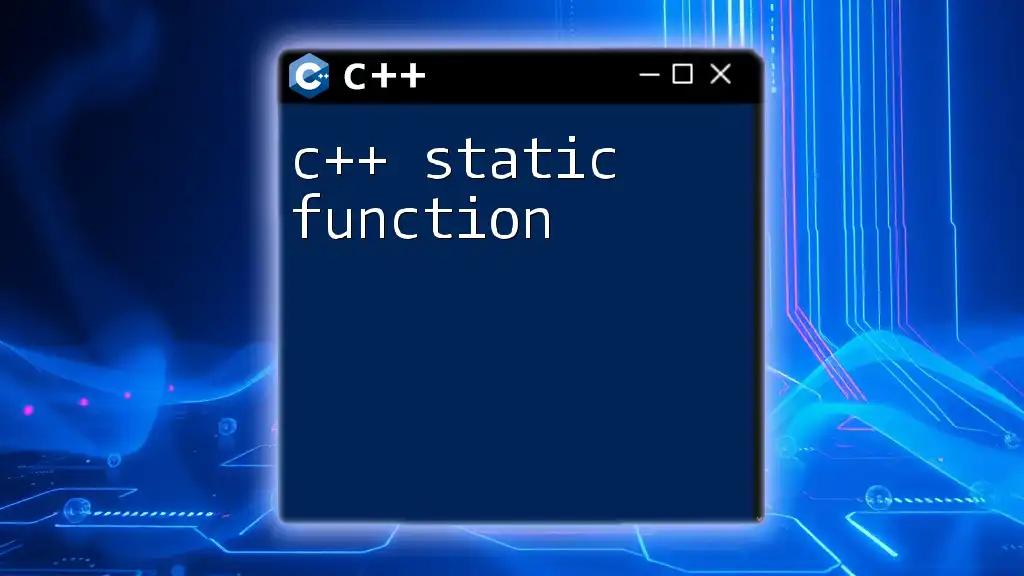
Conclusion
Understanding how C++ static variables inside functions work is crucial for effective programming. These variables provide valuable benefits, such as persistent state across function calls, memory efficiency, and scope control. Proper usage can lead to cleaner and more maintainable code. As with any powerful tool, however, awareness of their limitations and careful implementation are key to leveraging their full potential.

Additional Resources
For further reading, you can explore recommended C++ books or online courses focusing on advanced topics. Access the official C++ documentation for deeper insights into static variables and best practices.
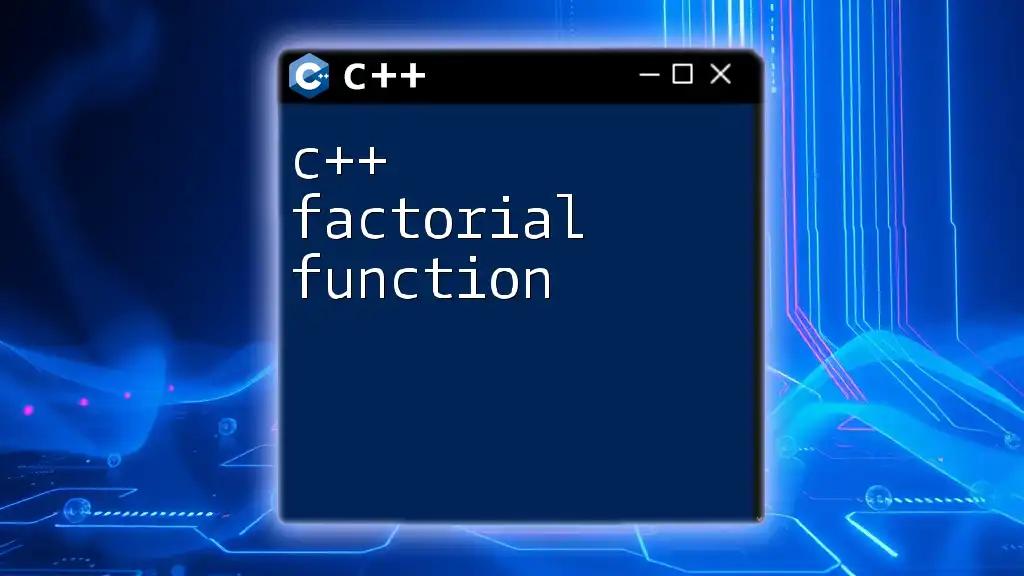
Frequently Asked Questions (FAQs)
Can a static variable be initialized with a function?
Static variables can be initialized using function return values, but care must be taken to ensure that the function returns a constant value that does not change between calls.
What happens if you declare a static variable in a class?
Static class variables are shared across all instances of the class, unlike instance variables. They hold the same value regardless of how many objects are created from the class.
Are static variables thread-safe?
Static variables are not inherently thread-safe, as multiple threads can access and modify the same variable simultaneously. Always use synchronization techniques when dealing with static variables in multi-threaded environments.