A C++ static vector is a fixed-size array that allows you to store elements in contiguous memory and access them via index, providing the benefits of both performance and simplicity.
Here's a code snippet demonstrating the use of a static vector:
#include <iostream>
int main() {
const int size = 5;
int staticVector[size] = {1, 2, 3, 4, 5};
for (int i = 0; i < size; i++) {
std::cout << staticVector[i] << " ";
}
return 0;
}
What is a Static Vector?
A static vector is a container that holds a fixed-size collection of elements of the same type. Unlike dynamic vectors, which can grow and shrink in size during runtime, static vectors are defined with a specific size at compile time. This characteristic makes static vectors particularly useful in scenarios where the maximum number of elements is known ahead of time, providing both memory efficiency and predictable access times.
The Concept of Static Vectors in C++
Static vectors offer a blend of the simplicity of arrays with the functionality of vectors. They are implemented on the stack, which often results in faster access times compared to heap-allocated dynamic vectors. Understanding when to use static vectors over their dynamic counterparts can significantly enhance performance in resource-constrained environments.
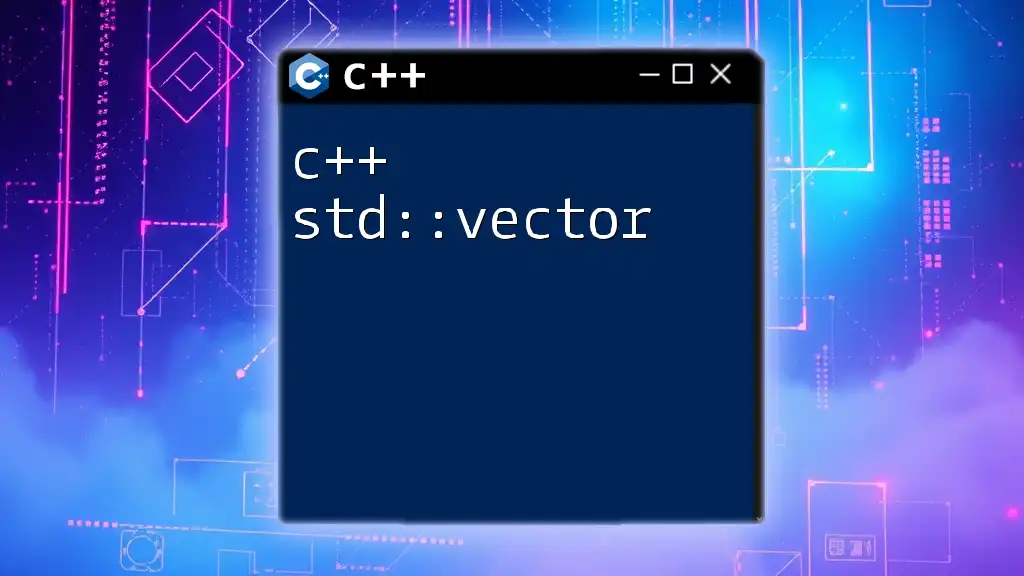
Memory Management
Memory management plays a crucial role in the performance characteristics of static vectors. When a static vector is allocated, it resides on the stack, leading to a faster allocation and deallocation process. The stack's nature allows for constant-time access to elements, making it particularly suitable for applications that require frequent read and write operations on the data.
However, being fixed in size, static vectors can lead to memory wastage if they are not fully utilized. For instance, if a static vector of size 10 is declared but only 3 elements are used, the remaining 7 slots occupy memory without serving a purpose. Therefore, it’s essential to assess the requirements of your application when deciding to use a static vector.
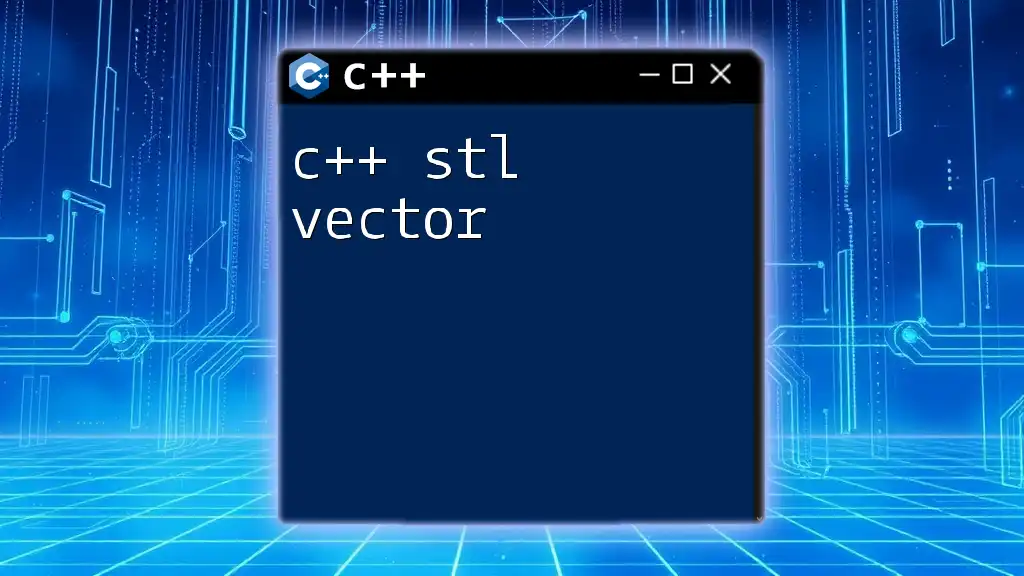
Implementing a Static Vector in C++
Creating a static vector in C++ is straightforward. It can be implemented using standard arrays. Here’s how you can define a static vector:
int myStaticVector[5] = {1, 2, 3, 4, 5};
Basic Operations
Accessing Elements: Accessing the elements of a static vector is similar to accessing elements in an array. Here’s how you can do it:
int firstElement = myStaticVector[0]; // Access the first element
Modifying Elements: Changing values in a static vector can be easily done through indexing:
myStaticVector[2] = 10; // Changes the third element to 10
Iterating through a Static Vector
You can iterate through the elements of a static vector using a range-based for loop, which provides a clean and concise way to access each element:
for (int i : myStaticVector) {
std::cout << i << " "; // Outputs each element in the vector
}
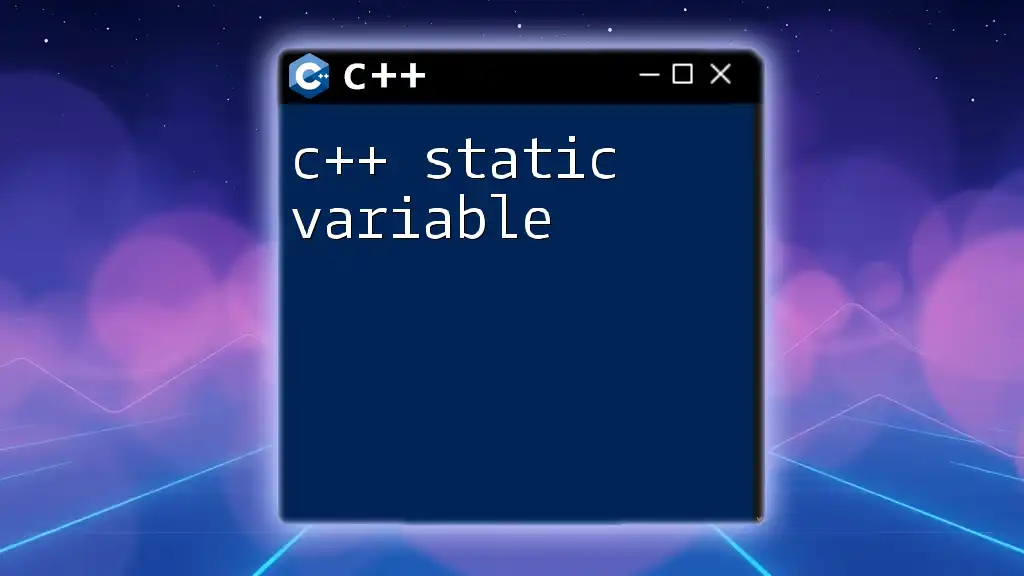
Advanced Features of Static Vectors
To take full advantage of static vectors, you can create a custom static vector class. This encapsulation allows you to introduce additional functionality, such as adding and removing elements while keeping the fixed size intact.
Here’s a basic outline of a static vector implementation:
template <typename T, std::size_t N>
class StaticVector {
public:
T data[N]; // Array to hold the elements
// Constructor
StaticVector() {
// Initialization logic can be implemented here
}
// Member functions for functionalities such as get, set, size, etc.
};
Advantages of Using a Custom Static Vector
By implementing your own static vector, you gain benefits like improved type safety and enhanced functionality. You can create custom methods for operations like `push_back` and `pop_back`, ensuring that your static vector maintains the fixed size while mimicking some of the behavior of dynamic vectors.
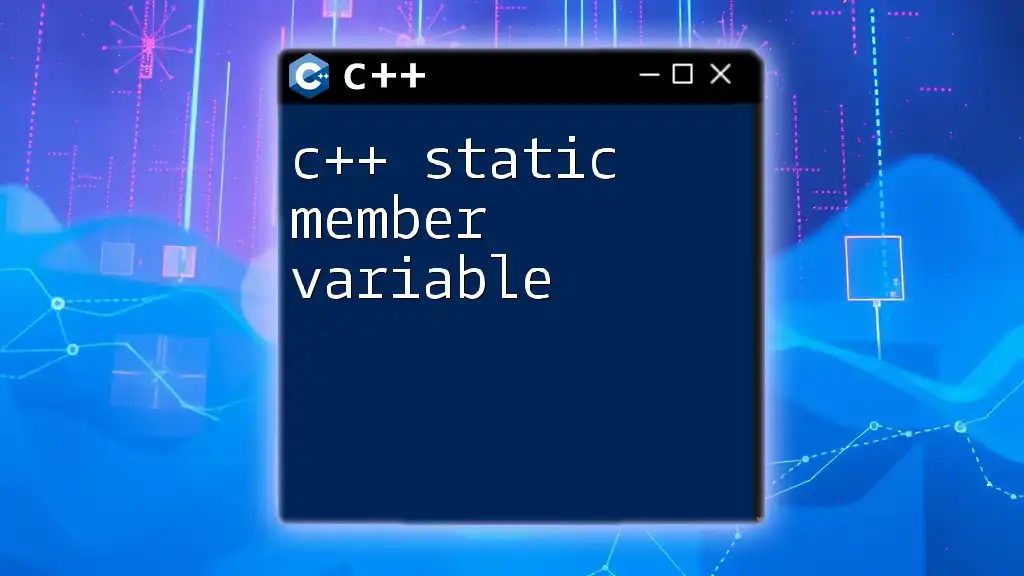
Pros and Cons of Static Vectors
Advantages
One major advantage of static vectors is the significant performance improvement due to quick access and iteration. Since their size is predetermined, the memory layout is contiguous, leading to better cache performance.
Disadvantages
On the flip side, static vectors have their limitations. The fixed size constraint can be restrictive, especially in applications where the size of data can fluctuate. Additionally, there’s the potential for memory waste if the declared size is not fully utilized.
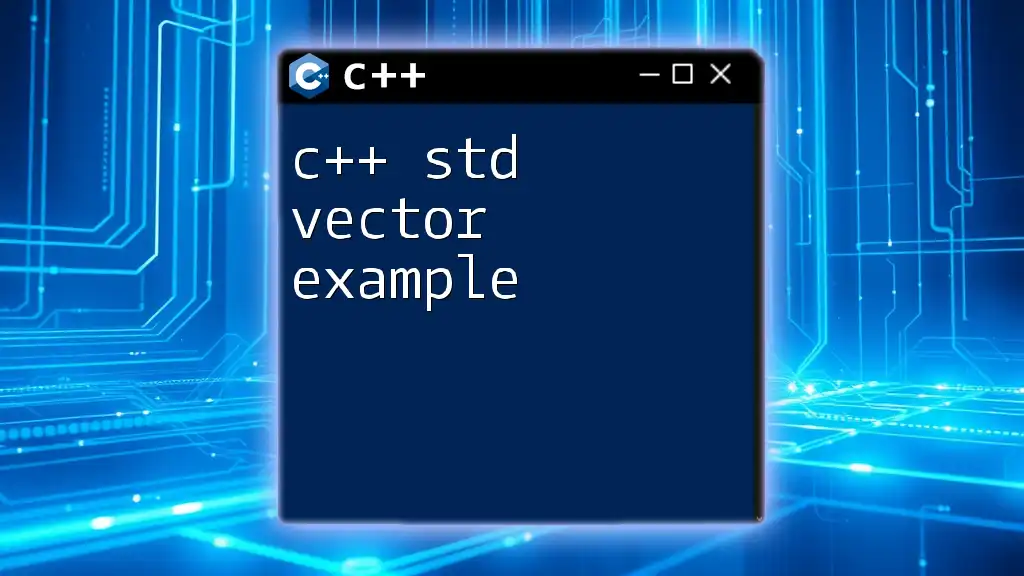
Real-World Use Cases for Static Vectors
Static vectors are particularly useful in scenarios where memory and performance are critical, such as in real-time game development or in embedded systems.
For instance, in game development, static vectors can be used to hold the positions of game entities where the maximum number of entities is known. This eliminates the overhead of dynamic allocation and provides consistent performance during critical loops.
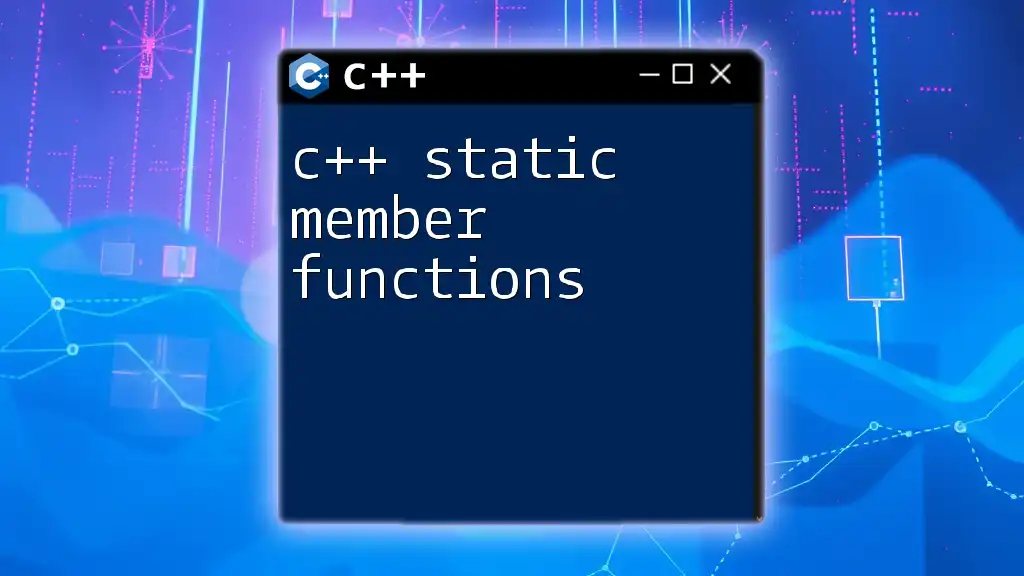
Best Practices for Working with Static Vectors
To avoid common pitfalls such as out-of-bounds access, it’s imperative to ensure that your indices always fall within the valid range. Remember that many modern development environments will not provide runtime checks for array bounds, so proper diligence is necessary.
Performance Considerations
When performance is paramount, especially in environments with strict resource constraints, a well-defined static vector can provide optimized access times compared to dynamic counterparts. Leveraging static vectors can significantly reduce overhead in critical paths of your application, such as rendering loops in a graphics engine.
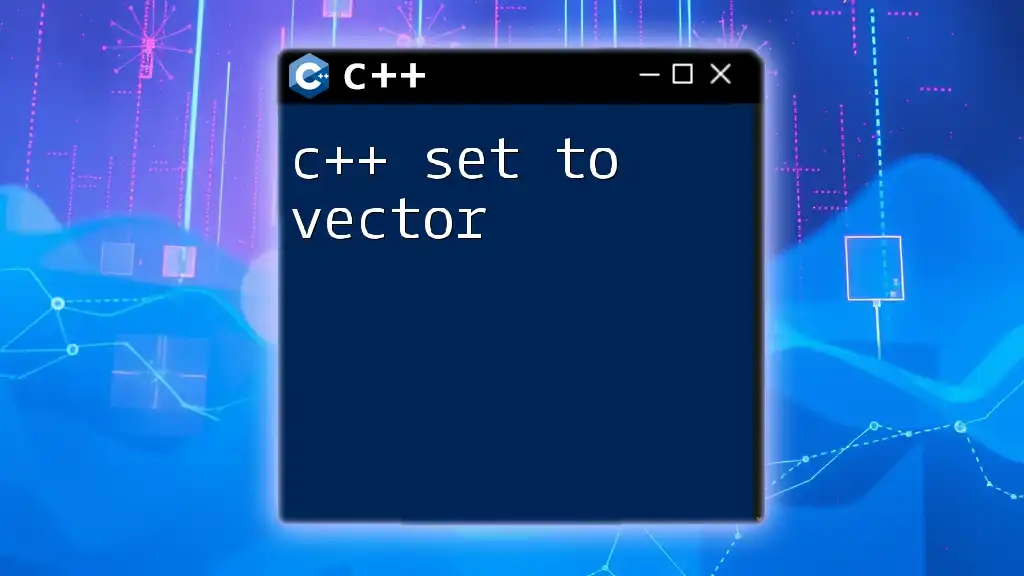
Conclusion
In summary, C++ static vectors offer a powerful alternative to dynamic memory management for cases where the data size is known ahead of time. With their speed and simplicity, they are a compelling choice for efficient applications. Understanding their trade-offs allows developers to make informed decisions on when and how to implement static vectors in their projects.
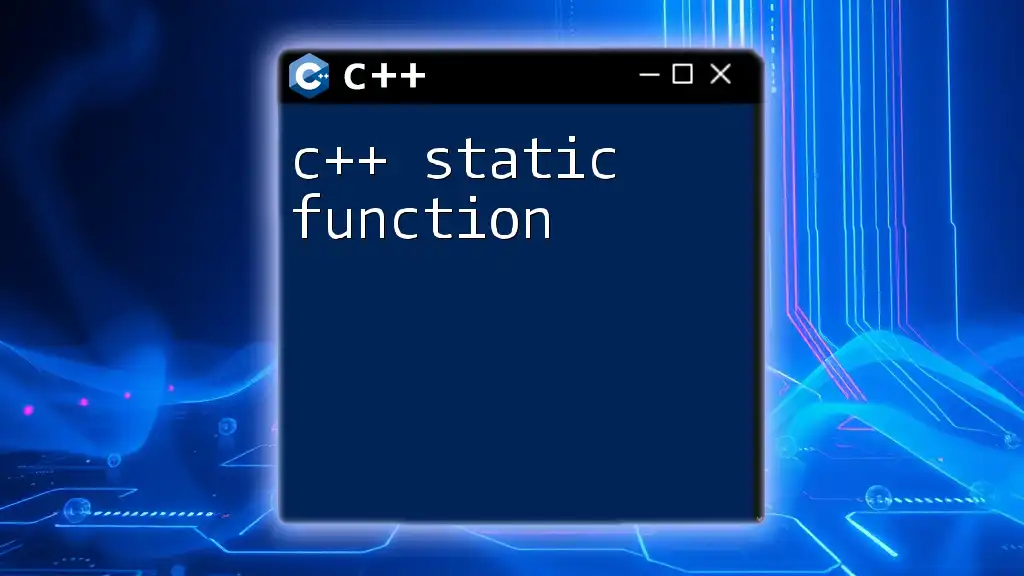
Additional Resources
To deepen your understanding, consider exploring official C++ documentation or take part in online courses focused on C++ data structures. Engaging with additional literature will help you gain a more rounded perspective on C++ static vectors and their myriad capabilities.