In C++, you can convert a `std::set` to a `std::vector` by utilizing the range constructor of `std::vector` that takes two iterators from the `set`.
#include <set>
#include <vector>
std::set<int> mySet = {1, 2, 3, 4, 5};
std::vector<int> myVector(mySet.begin(), mySet.end());
Understanding C++ Set and Vector
What is a C++ Set?
A C++ set is a collection of unique elements, often used for its property of managing distinct values automatically. Sets are implemented as binary search trees, specifically through Red-Black Trees, which ensure that elements are sorted. The primary characteristics of sets are:
- Uniqueness: Each element is unique; duplicates are automatically ignored.
- Ordering: Elements are stored in a specific order based on their value.
Here's a simple example of a set in C++:
#include <iostream>
#include <set>
int main() {
std::set<int> mySet = {5, 2, 8, 3, 5}; // Note the duplicate `5`
for (const auto& num : mySet) {
std::cout << num << " "; // Outputs: 2 3 5 8
}
return 0;
}
In this example, the element `5` appears twice during initialization, but the set only retains it once.
What is a C++ Vector?
A C++ vector is a dynamic array that offers flexibility with its size—allowing you to add or remove elements as needed. This structure provides the following features:
- Dynamic Sizing: Unlike arrays, vectors can grow or shrink, adapting to the number of elements they hold.
- Random Access: Elements can be accessed in constant time using an index.
Here’s a brief code snippet demonstrating a basic vector in C++:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
for (const auto& num : myVector) {
std::cout << num << " "; // Outputs: 1 2 3 4 5
}
return 0;
}
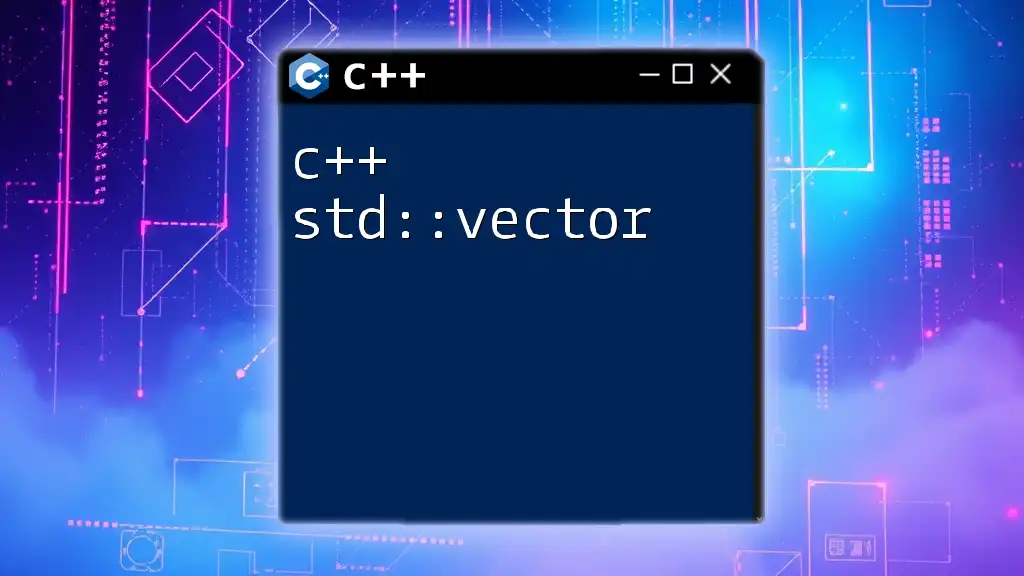
Why Convert Set to Vector?
Benefits of Conversion
Converting a set to a vector can be advantageous due to certain operational requirements. Some clear benefits include:
- Access Patterns: If your application frequently accesses elements by index, a vector is the better choice, as it allows for O(1) time access.
- Performance Considerations: Iterating over a vector can often be faster compared to a set due to better cache performance and contiguous memory allocation.
Use Cases for Conversion
Several scenarios merit converting a set to a vector:
- Removing Duplicates: When needing to ensure a collection of unique values before further processing.
- Sorting Data: While `set` maintains sorted data, converting to a vector allows you to utilize a variety of sorting algorithms or modify the order of elements efficiently.
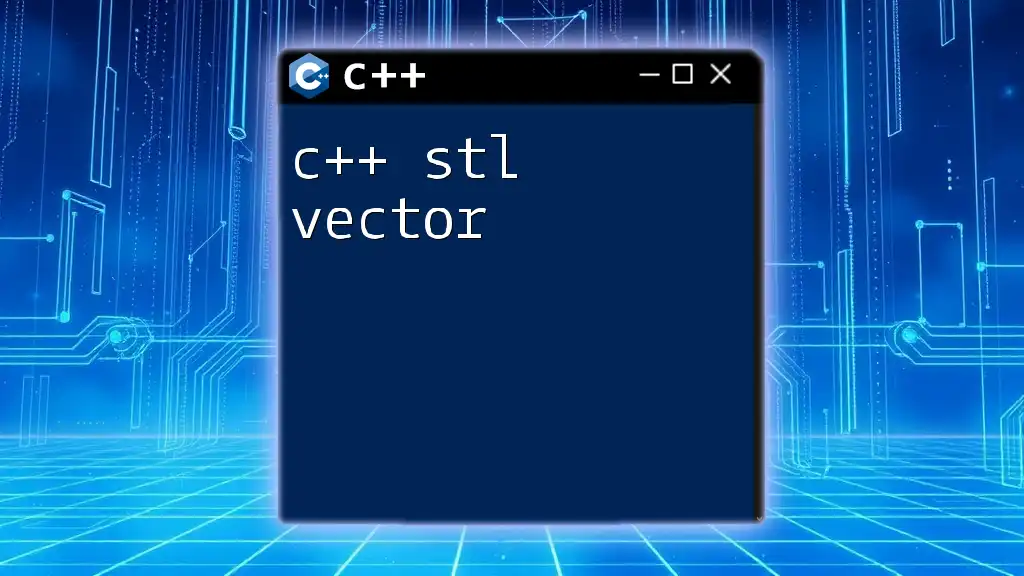
Methods to Convert Set to Vector
Using std::copy
Overview
One of the most straightforward methods for converting a set to a vector is using `std::copy` from the `<algorithm>` library. This method leverages iterators to copy elements efficiently.
Code Example
Here's how you can use `std::copy` to convert a set to a vector:
#include <iostream>
#include <set>
#include <vector>
#include <algorithm>
int main() {
std::set<int> mySet = {1, 2, 3, 4, 5};
std::vector<int> myVector;
std::copy(mySet.begin(), mySet.end(), std::back_inserter(myVector));
for (const auto& num : myVector) {
std::cout << num << " "; // Outputs: 1 2 3 4 5
}
return 0;
}
In this example, we first declare a vector and then use `std::copy` with `std::back_inserter` to fill it with elements from the set. The use of `back_inserter` ensures that the vector expands as needed.
Using Range Constructor
Overview
Another elegant method to convert a set to a vector is to employ the vector's range constructor, which allows for direct initialization using a set's iterators.
Code Example
Consider the following example:
#include <iostream>
#include <set>
#include <vector>
int main() {
std::set<int> mySet = {10, 20, 30, 40, 50};
std::vector<int> myVector(mySet.begin(), mySet.end());
for (const auto& num : myVector) {
std::cout << num << " "; // Outputs: 10 20 30 40 50
}
return 0;
}
In this case, we instantiate the vector directly with the set's iterators. This method is both concise and efficient, enhancing code readability.
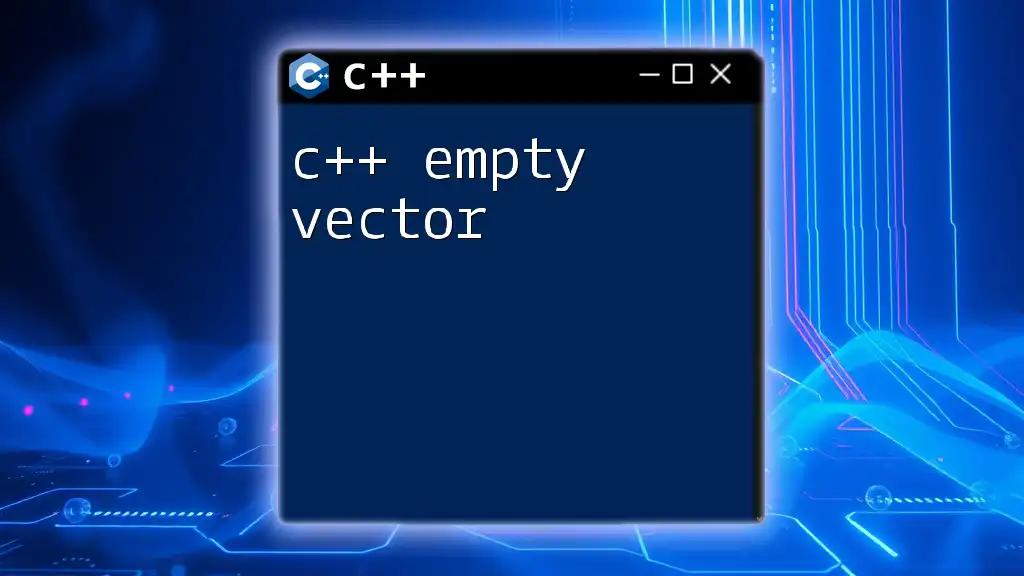
Tips for Working with Sets and Vectors
Best Practices
- Choosing Between Set and Vector: Understand your access patterns. If frequent lookups or sorted properties are needed, consider using a `set`. For indexed access and modifications, favor `vector`.
- Handling Duplicates Efficiently: When your primary goal is to ensure uniqueness, use sets first. Once confirmed unique, convert to a vector for ordered processing.
Common Pitfalls
While converting, be wary of a few common mistakes:
- Assuming that the order of elements will be preserved during conversion. Recall that while sets maintain order by value, vectors are indexed and can be rearranged.
- Overlooking performance implications during extensive conversions in critical sections of code. Ensure to assess time complexity, especially with large datasets.
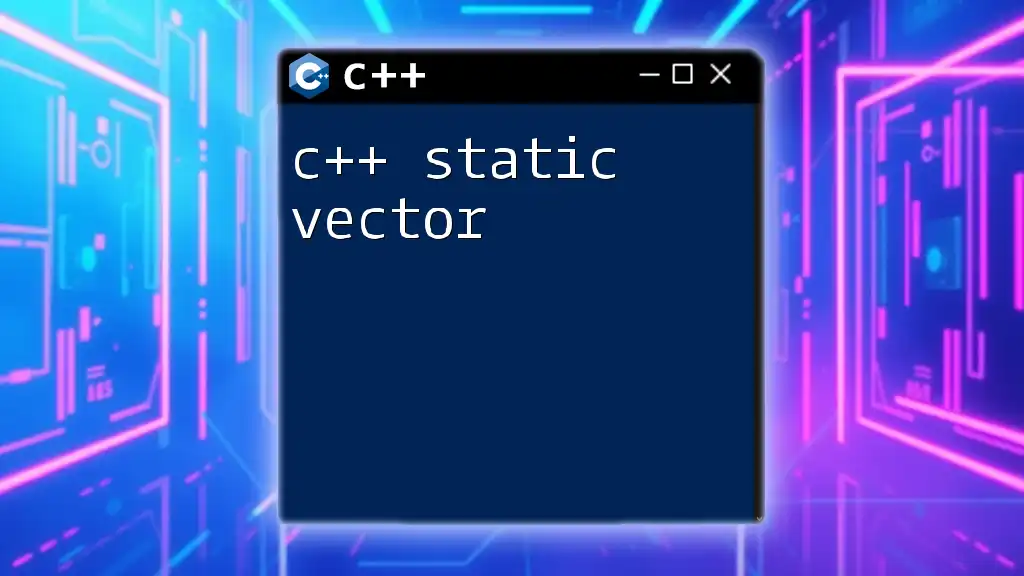
Performance Considerations
Time Complexity of Conversion
When converting from a set to a vector, the time complexity is O(n), where n is the number of elements in the set. This is due to copying each element from one structure to another. Both methods (`std::copy` and range constructor) uphold this complexity model.
Profiling Performance
Employing tools to profile the performance of your conversion logic is vital for ensuring your code is efficient. Consider using built-in profilers or utilities like [gprof](https://gcc.gnu.org/onlinedocs/gprof/) to gauge time-spent in these operations.
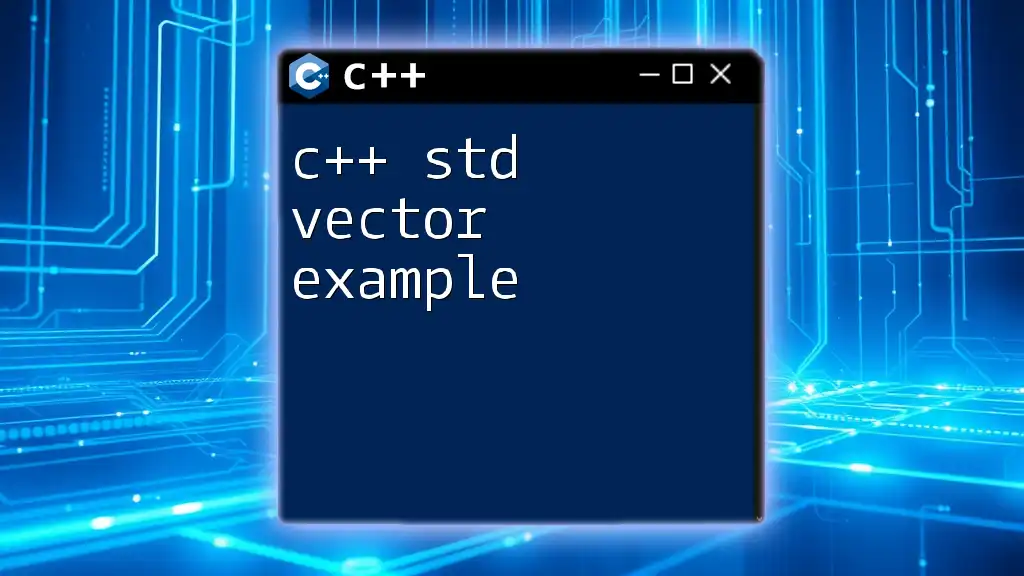
Conclusion
Converting a C++ set to a vector is a valuable technique that can enhance flexibility and performance within your applications. By understanding both structures and knowing when and how to perform this conversion, you can build more efficient data handling processes. Remember to experiment with the provided suggestions and techniques, tailoring them to fit your specific coding needs.
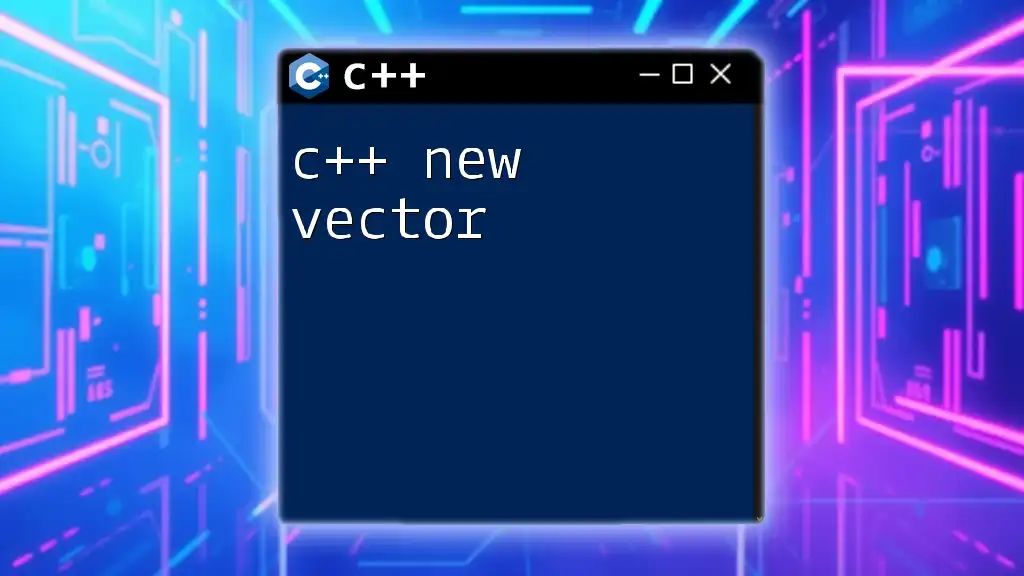
Additional Resources
Recommended Reading
To further develop your understanding of C++ data structures, consider exploring renowned books like:
- "Effective C++" by Scott Meyers
- "The C++ Programming Language" by Bjarne Stroustrup
Community Contributions
Engage with other programmers by joining discussions on forums like Stack Overflow or Reddit’s r/cpp. Sharing insights and questions can facilitate deeper understanding and application of C++ concepts.