C++ set operations allow you to perform various mathematical set functions, such as union, intersection, and difference, on collections of elements using the Standard Template Library (STL) `set` container.
Here's a code snippet demonstrating some basic set operations in C++:
#include <iostream>
#include <set>
#include <algorithm>
int main() {
std::set<int> setA = {1, 2, 3, 4};
std::set<int> setB = {3, 4, 5, 6};
// Union
std::set<int> unionSet;
std::set_union(setA.begin(), setA.end(), setB.begin(), setB.end(), std::inserter(unionSet, unionSet.begin()));
// Intersection
std::set<int> intersectionSet;
std::set_intersection(setA.begin(), setA.end(), setB.begin(), setB.end(), std::inserter(intersectionSet, intersectionSet.begin()));
// Difference
std::set<int> differenceSet;
std::set_difference(setA.begin(), setA.end(), setB.begin(), setB.end(), std::inserter(differenceSet, differenceSet.begin()));
// Display results
std::cout << "Union: ";
for (int num : unionSet) std::cout << num << " ";
std::cout << "\nIntersection: ";
for (int num : intersectionSet) std::cout << num << " ";
std::cout << "\nDifference (A - B): ";
for (int num : differenceSet) std::cout << num << " ";
return 0;
}
What are Sets in C++?
In C++, a set is a container that stores a collection of unique elements. Importantly, sets automatically sort their elements. This means that when you insert elements, you don't need to worry about managing duplicates or sorting them manually. Each element in a set is distinct, which makes it a powerful data structure for various applications.
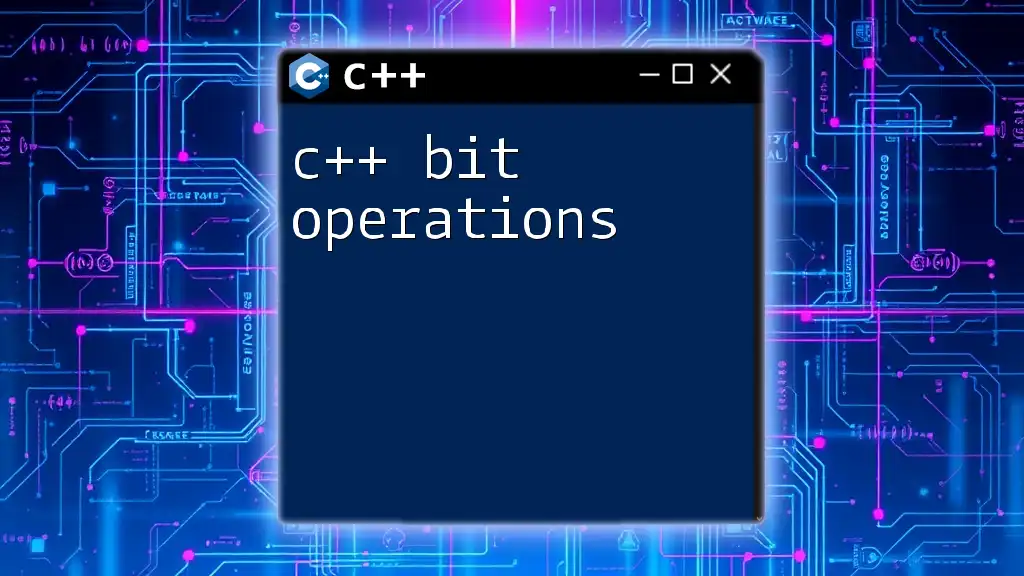
Why Use Sets in C++?
Using sets in your C++ programs can significantly enhance performance and simplify operations. The key advantages include:
- Uniqueness: Sets inherently prevent duplicate entries, ensuring that each element is represented only once.
- Sorted Order: The elements are sorted according to a defined order (typically in ascending order), which makes it easy to work with sorted data.
- Efficiency: Operations such as insertion, deletion, and searching can be performed in logarithmic time, providing efficiency for larger datasets.
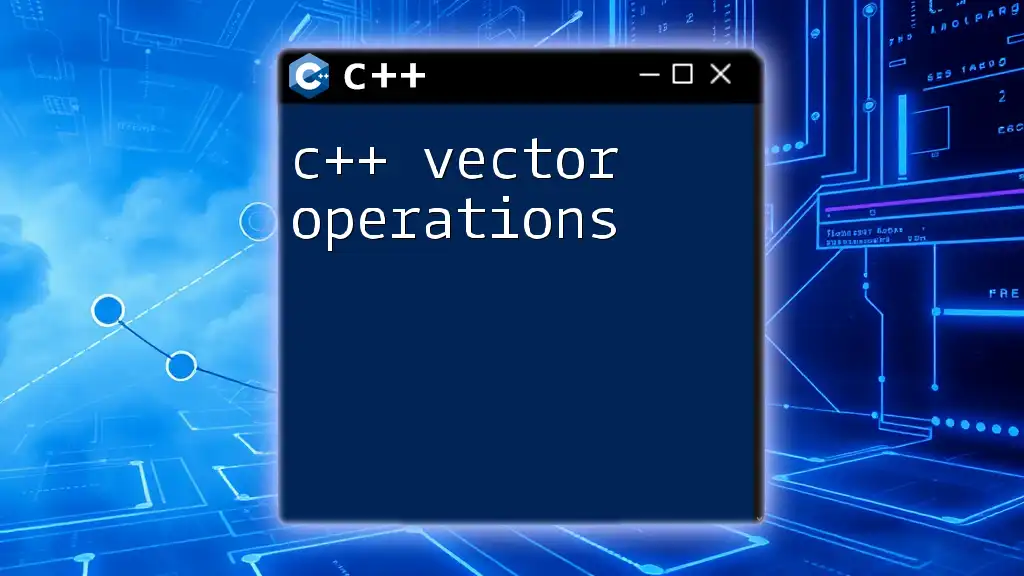
Overview of C++ Set in STL
The Standard Template Library (STL) provides a set implementation that is part of its ambitious framework to facilitate data structure management. C++ sets are defined in the `<set>` header file and utilize balanced binary trees (typically Red-Black trees) to maintain order and uniqueness.
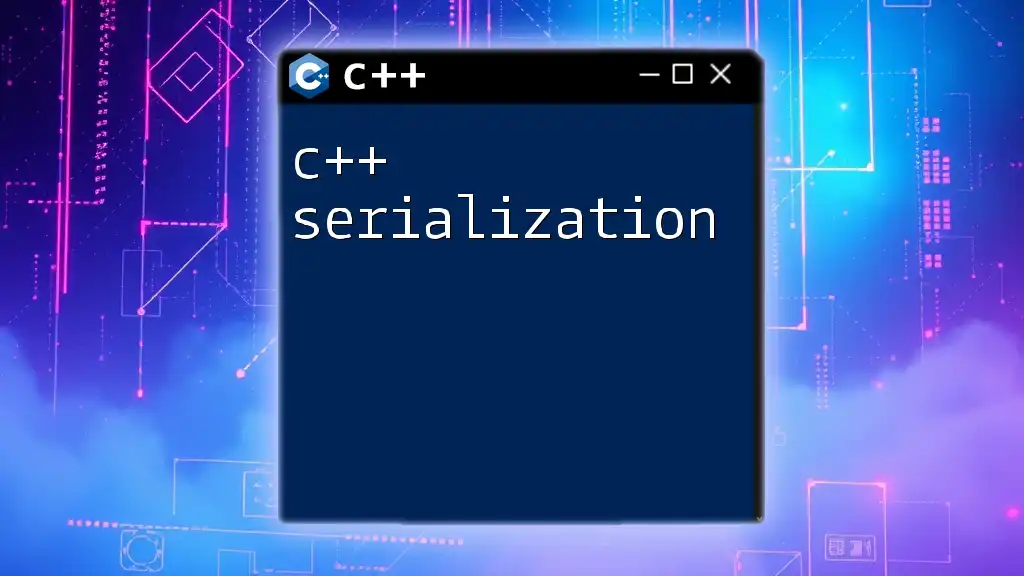
Basic Characteristics of Sets
Unique Elements
When you add an element to a set, it will not be added again if it already exists. This feature is crucial when dealing with large datasets that require distinct entries.
Sorted Order
Sets maintain their elements in a sorted order. For example, inserting the values `5`, `1`, and `3` into a set will result in an ordered collection: `{1, 3, 5}`.
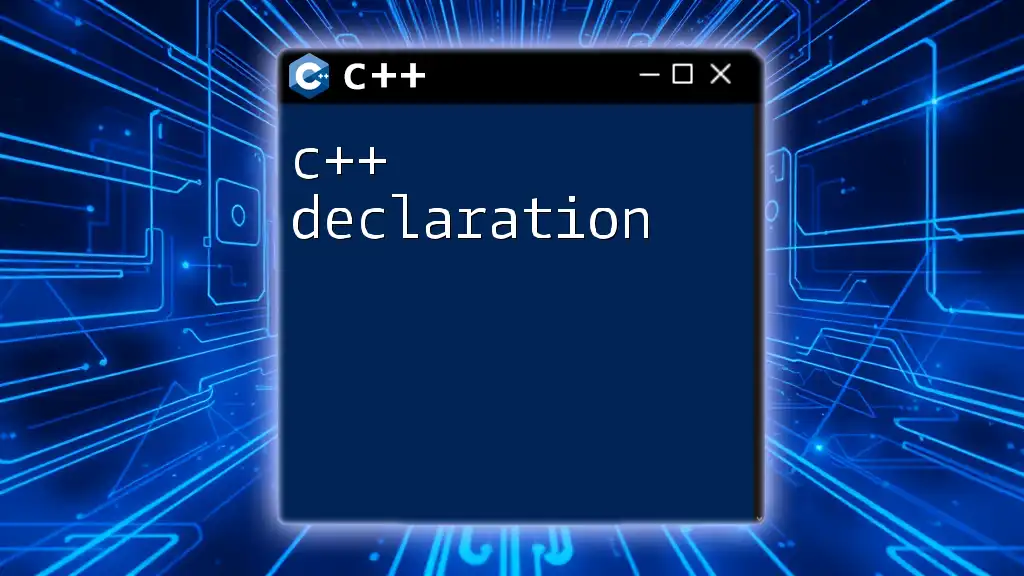
Basic Set Operations
Insertion of Elements
To insert elements into a C++ set, you can use the `insert` method. Here’s a simple code snippet demonstrating how to do this:
#include <iostream>
#include <set>
int main() {
std::set<int> mySet;
mySet.insert(10);
mySet.insert(20);
mySet.insert(10); // Will not insert again
for (const auto& element : mySet) {
std::cout << element << " ";
}
// Output: 10 20
return 0;
}
In this snippet, inserting `10` a second time will not have any effect, illustrating the unique element property of the set.
Deletion of Elements
The `erase` function allows removing specific elements from a set. Here’s how you can delete an element:
#include <iostream>
#include <set>
int main() {
std::set<int> mySet = {10, 20, 30};
mySet.erase(20); // Removes the element
for (const auto& element : mySet) {
std::cout << element << " ";
}
// Output: 10 30
return 0;
}
Searching for Elements
To check if an element exists within a set, you can utilize the `find` method, which returns an iterator pointing to the element if found:
auto it = mySet.find(10);
if (it != mySet.end()) {
std::cout << "Element found: " << *it << "\n";
} else {
std::cout << "Element not found\n";
}
Count Method for Existence Check
Alternatively, you can use the `count` method to check for existence. If the element exists, it will return `1`; otherwise, it returns `0`.
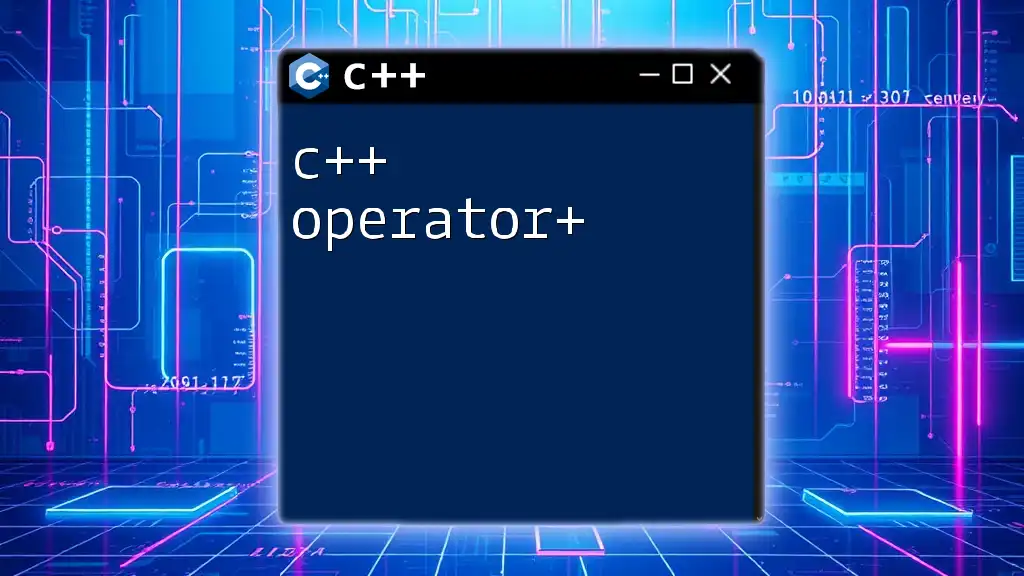
Advanced Set Operations
C++ Set Intersections
A set intersection is an operation that produces a new set containing elements that are common to two sets. Here’s how to perform this operation:
#include <iostream>
#include <set>
#include <algorithm>
int main() {
std::set<int> setA = {1, 2, 3};
std::set<int> setB = {2, 3, 4};
std::set<int> intersection;
std::set_intersection(setA.begin(), setA.end(), setB.begin(), setB.end(),
std::inserter(intersection, intersection.begin()));
for (const auto& elem : intersection) {
std::cout << elem << " ";
}
// Output: 2 3
return 0;
}
C++ Set Unions
Set union combines the elements of both sets, ensuring that only unique elements remain. Here’s an example of performing a union operation:
#include <iostream>
#include <set>
#include <algorithm>
int main() {
std::set<int> setA = {1, 2, 3};
std::set<int> setB = {2, 3, 4};
std::set<int> unionSet;
std::set_union(setA.begin(), setA.end(), setB.begin(), setB.end(),
std::inserter(unionSet, unionSet.begin()));
for (const auto& elem : unionSet) {
std::cout << elem << " ";
}
// Output: 1 2 3 4
return 0;
}
C++ Set Differences
A set difference operation returns elements found in the first set but not in the second. This can be illustrated through the following code:
#include <iostream>
#include <set>
#include <algorithm>
int main() {
std::set<int> setA = {1, 2, 3};
std::set<int> setB = {2, 3, 4};
std::set<int> differenceSet;
std::set_difference(setA.begin(), setA.end(), setB.begin(), setB.end(),
std::inserter(differenceSet, differenceSet.begin()));
for (const auto& elem : differenceSet) {
std::cout << elem << " ";
}
// Output: 1
return 0;
}
![Unlocking the C++ [] Operator: A Step-by-Step Guide](/images/posts/c/cpp-operator.webp)
Practical Applications of Set Operations
The usefulness of C++ sets extends to numerous applications. For instance, when dealing with large databases of user IDs, sets help manage and ensure the uniqueness of these identifiers, which is pivotal in a multi-user system. Similarly, when implementing functionalities like playlist management in music applications, sets guarantee that songs are not repeated.
Performance Considerations
One of the standout features of C++ sets is their efficiency. Insertions, deletions, and searches can be performed in logarithmic time complexity, O(log n), because of their underlying tree structure. This efficiency makes sets an excellent choice for applications that require frequent modifications and queries.
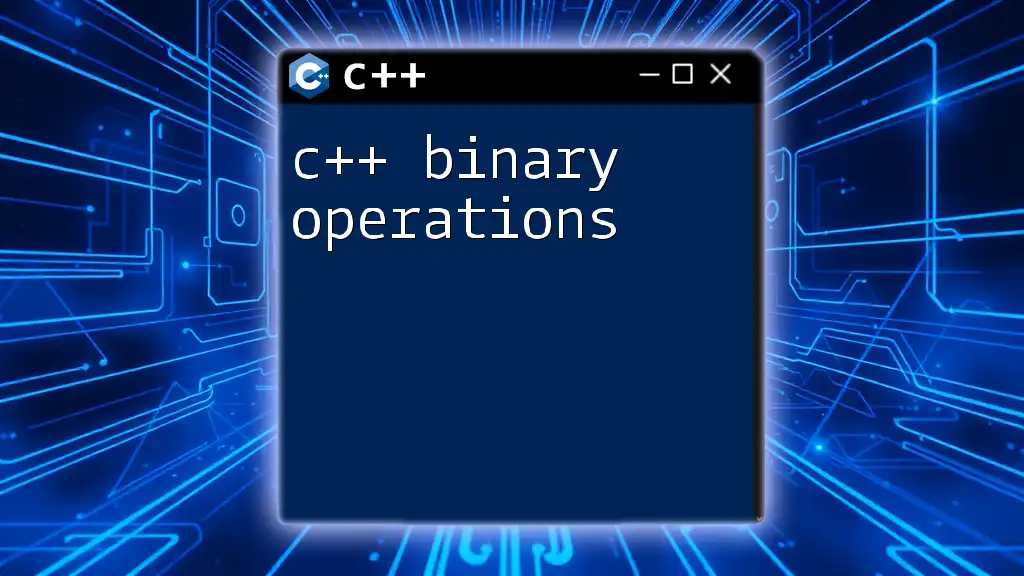
Recap of Key Concepts
In this article, we explored C++ set operations, highlighting their efficiency and functionality. From understanding basic characteristics and insertion methods to more complex operations like intersections and differences, we covered a broad spectrum of topics.
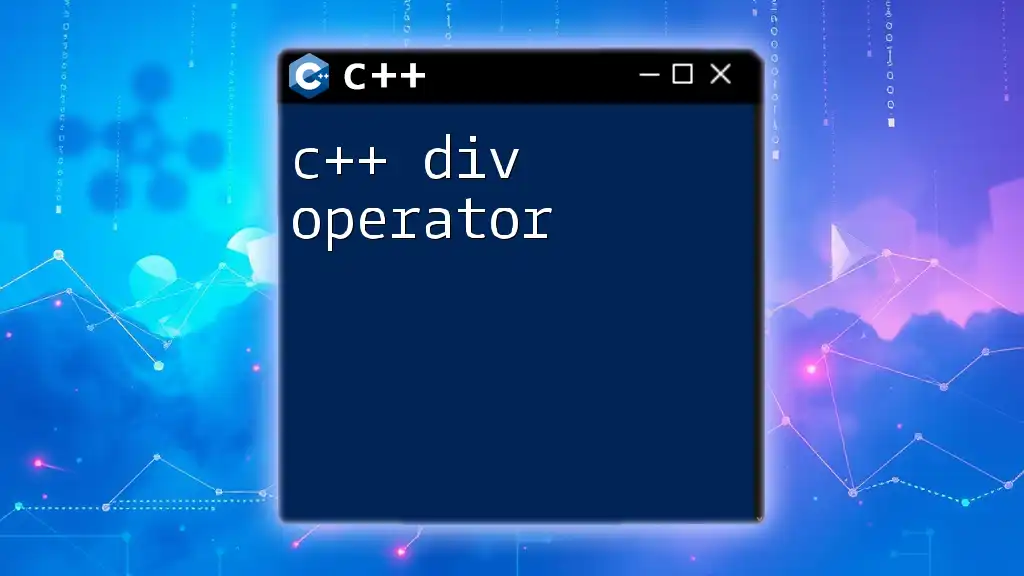
Next Steps in Learning C++ Sets
As you delve deeper into the C++ Standard Template Library, consider experimenting with other containers like `unordered_set` for unsorted collections or exploring the performance implications of using different set types. With practice, you will be able to leverage sets effectively in your C++ applications.
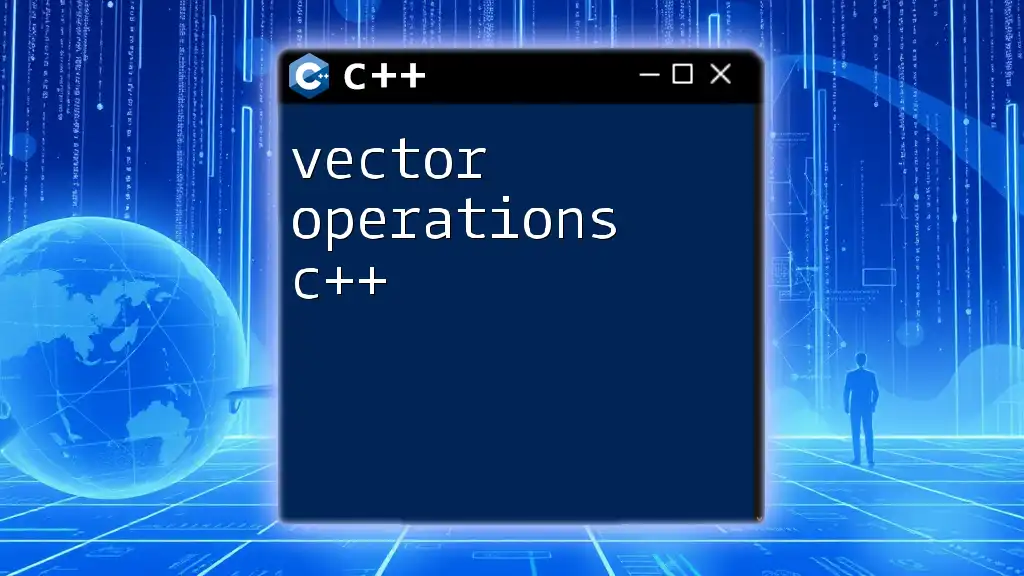
Additional Resources
For a robust and detailed understanding, consider reviewing the official C++ documentation and exploring literature dedicated to data structures and algorithms. These resources will provide further insights into C++ sets and related STL components.