`std::optional` in C++ is a template class that encapsulates an optional value, allowing you to represent the presence or absence of a value without using pointers or special sentinel values.
#include <iostream>
#include <optional>
int main() {
std::optional<int> opt; // Initially empty
opt = 42; // Now contains a value
if (opt) {
std::cout << "Value: " << *opt << std::endl; // Dereference to get the value
} else {
std::cout << "No value" << std::endl;
}
return 0;
}
Understanding `std::optional`
What is `std::optional`?
`std::optional` is a utility introduced in C++17 that holds either a value of a specified type or no value at all. This makes it suitable for representing optional or missing data in a type-safe manner. For example, when a function might not return a result, using `std::optional` can clarify that the return value is optional, distinguishing it from a guaranteed return type.
The Basics of `optional` in C++
`std::optional` enhances code readability by explicitly stating that a value may or may not be present, thus reducing the risks of errors associated with uninitialized variables or null pointers. Unlike raw pointers, which can lead to undefined behavior if dereferenced when not initialized, `std::optional` requires its users to perform checks, promoting safer code practices. Using `std::optional` can also be more expressive than using plain `std::nullopt` as a sentinel value.
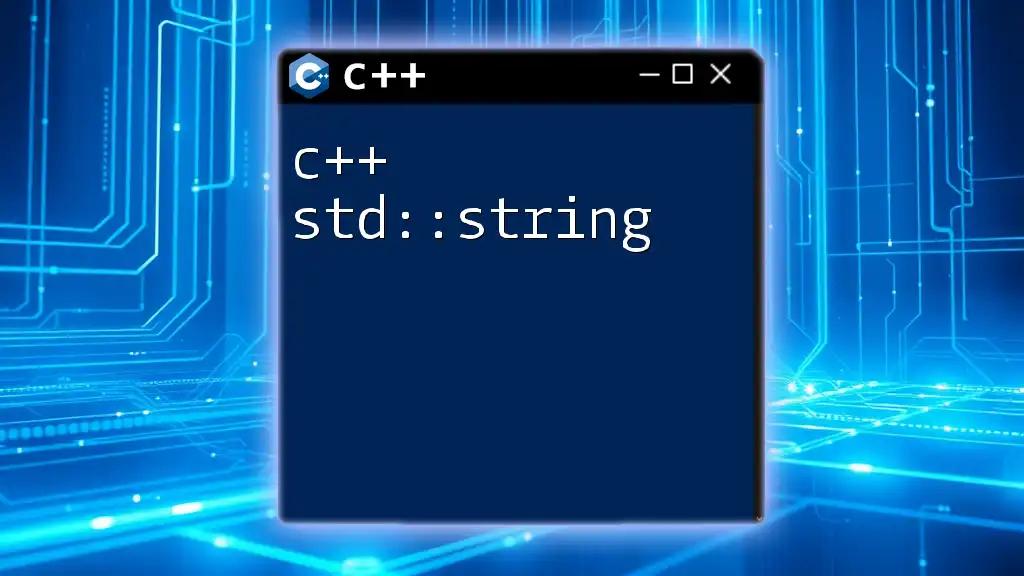
Getting Started with `std::optional`
Including the Necessary Header
To utilize `std::optional`, ensure that you include the associated header file in your C++ program:
#include <optional>
Creating an `std::optional`
Declaring an `std::optional` is straightforward. It can be done by specifying the type it will hold:
std::optional<int> maybeValue;
This declaration creates an optional integer that initially does not contain a value.
Initializing an `std::optional`
You can initialize an `std::optional` with or without a value. For instance:
std::optional<int> valueWithDefault(10); // Initialized with value
std::optional<int> emptyValue; // No value (empty)
In this example, `valueWithDefault` holds the integer 10, while `emptyValue` does not contain any value.

Working with `std::optional`
Checking if a Value is Present
To determine if an `std::optional` contains a value, you can use the `has_value()` method or simply leverage its boolean conversion:
if (maybeValue) {
// The optional contains a value
}
This code snippet checks whether `maybeValue` is non-empty.
Accessing the Value
There are several ways to access the contained value in an `std::optional`. You can use `value()`, `operator*`, or `operator->`.
int& ref = *valueWithDefault; // Using dereference operator
int val = valueWithDefault.value(); // Using value() method
It is important to note that calling `value()` on an empty `std::optional` will throw a `std::bad_optional_access` exception. Therefore, it's advisable to check if the optional is empty before accessing the value.
Modifying an `std::optional`
You can assign new values to an `std::optional` or reset it entirely:
valueWithDefault = 20; // Assign a new value
maybeValue.reset(); // Clear the value
Using `reset()` effectively empties the optional, returning its state to not containing any value.

Practical Use Cases of `std::optional`
Returning Optional Values from Functions
Using `std::optional` in function return types provides clarity in cases where a function may not be able to return a meaningful value. For instance:
std::optional<int> findValue(int key) {
if (dataExists(key)) {
return fetchValue(key);
}
return std::nullopt; // Explicitly no value
}
In this example, the function `findValue` returns an `std::optional<int>`, indicating that the result may or may not be present depending on whether the key exists.
Avoiding Null Pointers
One of the significant advantages of using `std::optional` is its ability to prevent null pointer exceptions. Traditional pointers can often lead to undefined behavior if they are dereferenced when empty. With `std::optional`, it explicitly manages its state through the presence or absence of values, thus promoting safer code:
std::optional<std::string> maybeStr = getString();
if (maybeStr) {
// Safe to use *maybeStr
}
In this case, `maybeStr` must be checked before use, ensuring that you do not mistakenly dereference an invalid pointer.
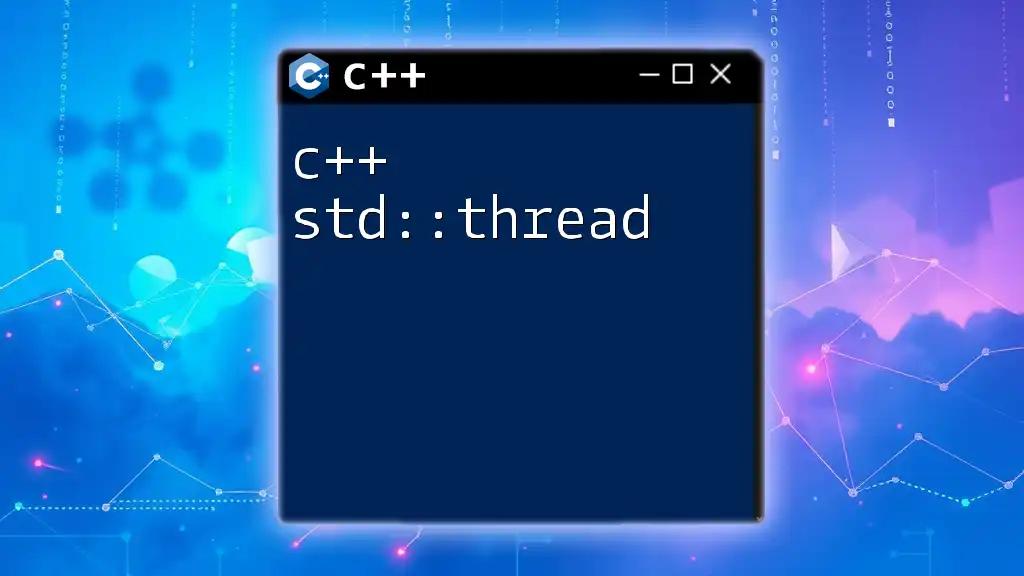
Advanced Features of `std::optional`
Moving and Copying `std::optional`
`std::optional` supports both copy and move semantics. You can easily copy an optional:
std::optional<int> copyValue = valueWithDefault;
For move operations:
std::optional<int> movedValue = std::move(copyValue); // copyValue is now empty
Understanding these mechanisms is crucial for effective memory management and performance optimization in C++.
Interactions with Other Standard Library Features
`std::optional` can also be effectively used in conjunction with other Standard Library features like `std::vector` and `std::map`. For instance:
std::map<int, std::optional<std::string>> optionalMap;
Here, `optionalMap` can store a mapping from integers to potentially absent strings, offering great flexibility when dealing with data that might not always be present.

Common Pitfalls and Best Practices
Understanding When Not to Use `std::optional`
While `std::optional` can be a powerful tool, it is also crucial to recognize when it is not the best fit. Avoid using it in scenarios where a value is guaranteed to be present or when representing multiple optional values (consider using `std::variant` or structures instead).
Performance Considerations
Generally, the performance overhead of `std::optional` is minimal, especially compared to the safety it provides. However, it is always wise to consider profiling in performance-critical applications. When used appropriately, `std::optional` can lead to more maintainable and understandable code.

Conclusion
C++ `std::optional` provides developers with a reliable way to handle optional values, improving code clarity and safety. By using `std::optional`, you can minimize common pitfalls associated with pointers and nullable types, encouraging better practices in C++. As you explore modern C++ features, experimenting with `std::optional` will equip you with tools to write more robust applications.

Additional Resources
For further learning, consider checking out additional books and resources focusing on modern C++ principles, usage of `std::optional`, and enhanced programming techniques.
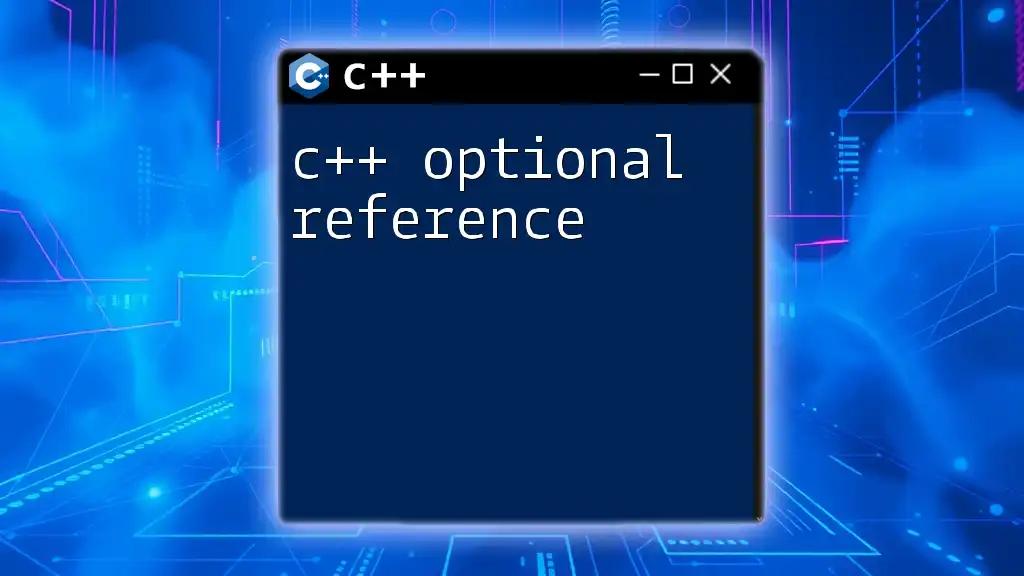
FAQs about `std::optional`
What is the advantage of using `std::optional` over raw pointers?
Using `std::optional` eliminates the risks of dereferencing uninitialized or null pointers, enforcing compile-time checks for value presence and improving overall code safety and readability.
Can `std::optional` be used as a class member variable?
Yes, `std::optional` can be effectively used as a member variable within classes, providing a way to represent optional properties.
How does `std::optional` work with templates?
`std::optional` works seamlessly with templates, allowing you to create optional containers for any type specified in your template parameters. This powerful feature enables more flexible data structures and algorithms.