The `std::transform` function in C++ applies a specified operation to a range of elements and stores the results in a destination range.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::vector<int> result(vec.size());
std::transform(vec.begin(), vec.end(), result.begin(), [](int x) { return x * 2; });
for (int r : result) {
std::cout << r << " ";
}
return 0;
}
What is std::transform?
`std::transform` is a standard algorithm in the C++ Standard Library that allows you to apply a transformation function to a range of elements. This functionality is especially useful when you want to modify, manipulate, or generate new data from existing collections without writing explicit loops.
By utilizing `std::transform`, you can achieve cleaner, more readable code and can often improve performance due to optimized implementations provided by the library.
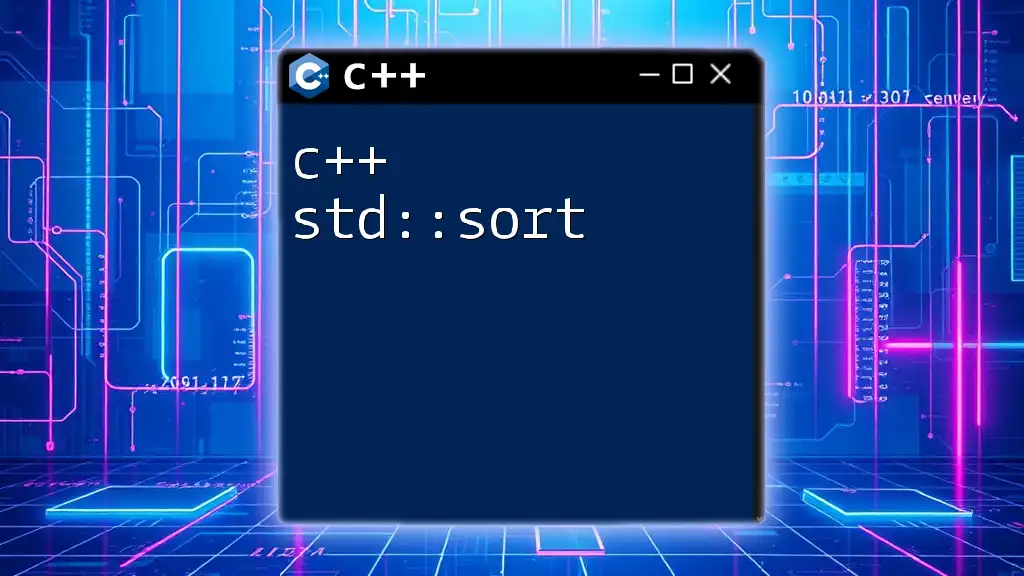
Importance of std::transform in C++
Using `std::transform` offers several benefits:
- Conciseness: It reduces the boilerplate code typically associated with loops, allowing you to express transformations in a compact manner.
- Clarity: The intent of the operation becomes clearer when you use functional-style programming provided by `std::transform`.
- Optimization: Standard Library algorithms are usually optimized for performance, which might help enhance the efficiency of your code compared to manually written loops.
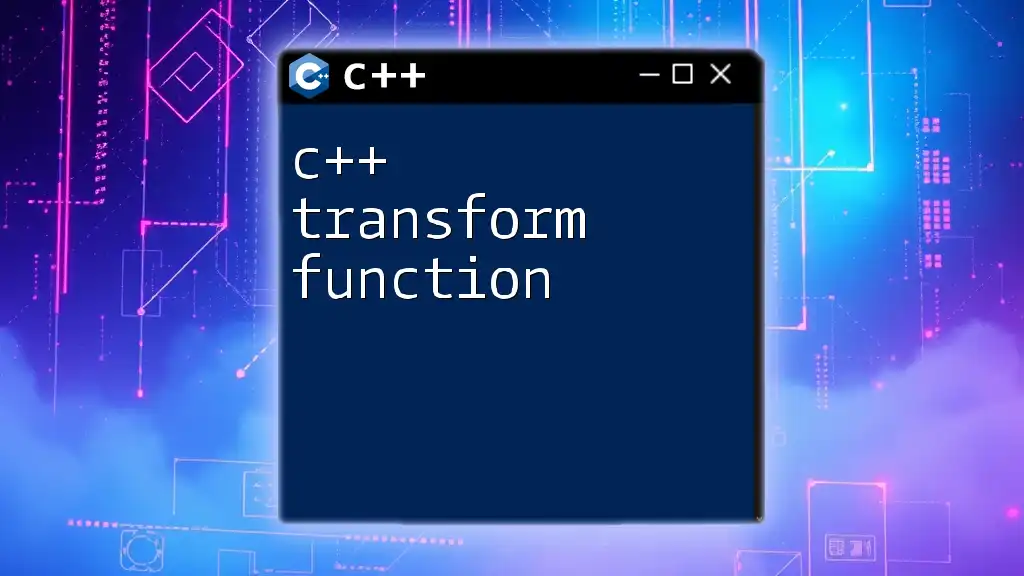
How std::transform Works
Syntax of std::transform
The syntax for `std::transform` is as follows:
std::transform(InputIt first1, InputIt last1, OutputIt d_first, UnaryOperation op);
std::transform(InputIt first1, InputIt last1, InputIt first2, OutputIt d_first, BinaryOperation op);
Parameters Explained
- InputIt first1, last1: These specify the range of inputs on which the transformation will be applied. The operation will iterate over the range defined by `first1` up to (but not including) `last1`.
- InputIt first2: This is applicable when using a binary operation. It represents the starting position of a second input range.
- OutputIt d_first: This is the iterator pointing to the beginning of the output range where the results of the transformation will be stored.
- UnaryOperation op: The transformation function that takes one argument and returns the transformed value.
- BinaryOperation op: This is used when two input ranges are involved and takes two arguments, performing a transformation based on both.
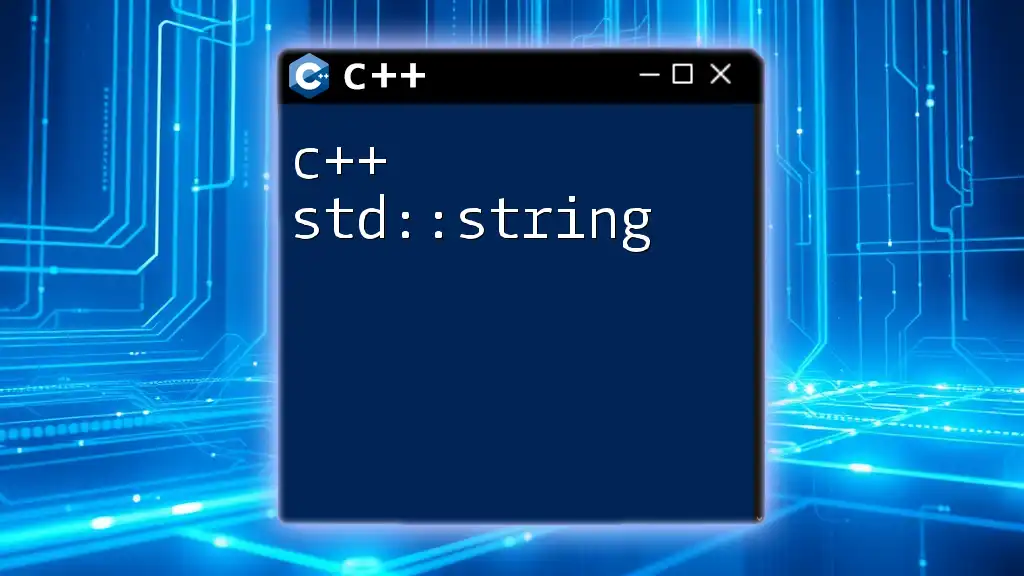
Use Cases for std::transform
Transforming Data in Containers
One of the most common use cases of `std::transform` is transforming elements held within STL containers, such as arrays, `std::vector`, or `std::list`.
Example: Square Elements in a Vector
For instance, if you want to create a vector that stores the squares of elements from an existing vector, you can do so easily:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4};
std::vector<int> result(vec.size());
std::transform(vec.begin(), vec.end(), result.begin(), [](int x) { return x * x; });
for (int n : result) std::cout << n << ' '; // Output: 1 4 9 16
}
In this example, `std::transform` applies a lambda function to each element in `vec`, computing and storing the squares directly into the `result` vector. This demonstrates the power of functional programming in simplifying certain operations.
Combining Two Containers
`std::transform` is not limited to unary operations; it can also perform binary operations by combining elements from two containers.
Example: Adding Two Vectors
Suppose you have two vectors and you want to create a new vector that contains the element-wise sum of these two vectors:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec1 = {1, 2, 3, 4};
std::vector<int> vec2 = {10, 20, 30, 40};
std::vector<int> result(vec1.size());
std::transform(vec1.begin(), vec1.end(), vec2.begin(), result.begin(), std::plus<int>());
for (int n : result) std::cout << n << ' '; // Output: 11 22 33 44
}
Here, `std::transform` uses `std::plus<int>` from the standard library to add the corresponding elements of `vec1` and `vec2`, storing the sum in `result`. This highlights how `std::transform` can efficiently manage operations across multiple data sources.
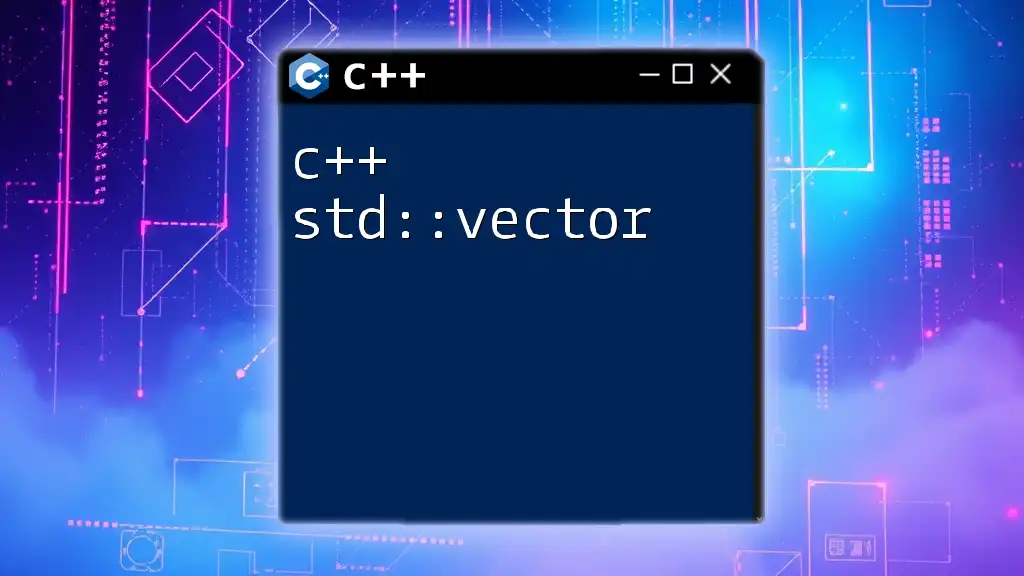
Advanced Usage of std::transform
Passing Custom Functions
One of the strengths of `std::transform` is its ability to accept custom functions or functors, adding flexibility to your transformations.
Example: Custom Functor
You can create a struct that acts as a functor to multiply elements by a specific factor, as shown below:
#include <iostream>
#include <vector>
#include <algorithm>
struct MultiplyBy {
int factor;
MultiplyBy(int f) : factor(f) {}
int operator()(int x) const { return x * factor; }
};
int main() {
std::vector<int> vec = {1, 2, 3, 4};
std::vector<int> result(vec.size());
std::transform(vec.begin(), vec.end(), result.begin(), MultiplyBy(5));
for (int n : result) std::cout << n << ' '; // Output: 5 10 15 20
}
In this example, the custom functor `MultiplyBy` enables you to multiply each element in `vec` by 5, demonstrating the power and usability of user-defined transformation logic while working with `std::transform`.
Handling Edge Cases
While `std::transform` is powerful, it's also important to understand some potential pitfalls. For instance, if the sizes of the input ranges differ, the behavior is undefined when using binary operations. Always ensure that the input iterators are valid and that you're not accessing out-of-bounds.
Performance Considerations
When comparing `std::transform` with manual loops, one major advantage is the potential for the Standard Library implementations to leverage optimizations. Moreover, by using `std::transform`, you can often express your intent in a clearer way, making it easier for others (and yourself) to read and maintain the code later on.
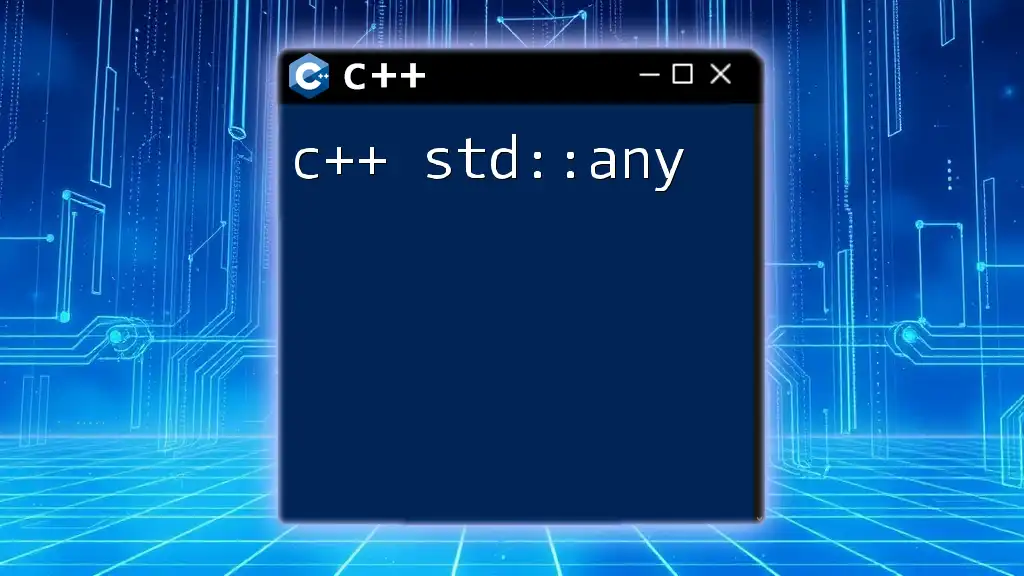
Conclusion
In summary, `std::transform` is a powerful tool in C++ that allows you to efficiently apply transformations to data collections. By using a clear syntax and benefiting from optimized implementations, you can write cleaner, more maintainable code. This algorithm encourages you to adopt a functional programming style, making your programs not only shorter but often more expressive.
To become proficient with `std::transform`, practice using various transformation functions and combining elements in interesting ways. Explore the versatility of this function and how it can simplify coding tasks in C++.
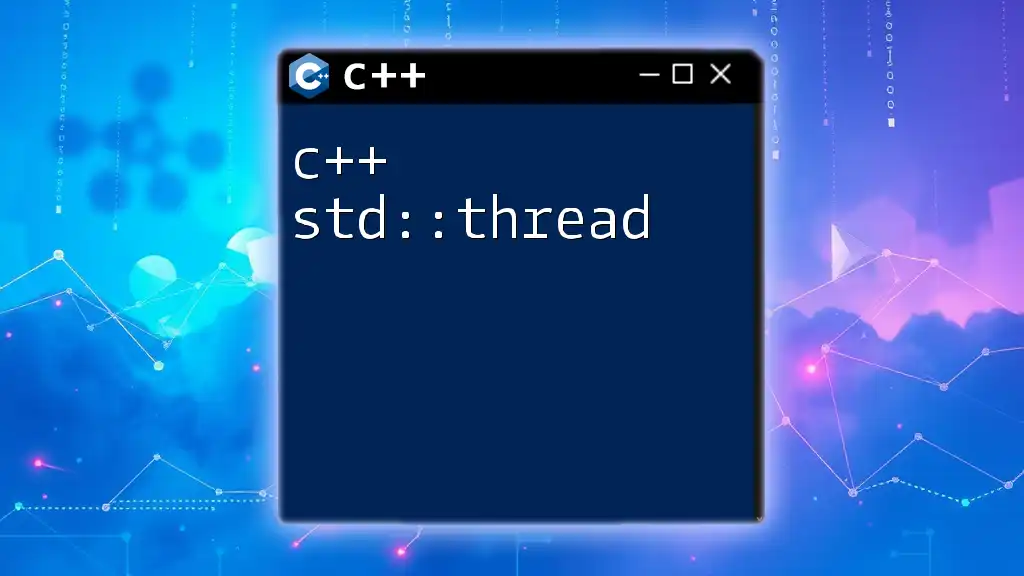
Additional Resources
For more information about `std::transform` and other algorithms, you may visit:
- [cppreference.com](https://en.cppreference.com/w/cpp/algorithm/transform)
- Relevant C++ programming textbooks and courses that cover the Standard Library in depth.
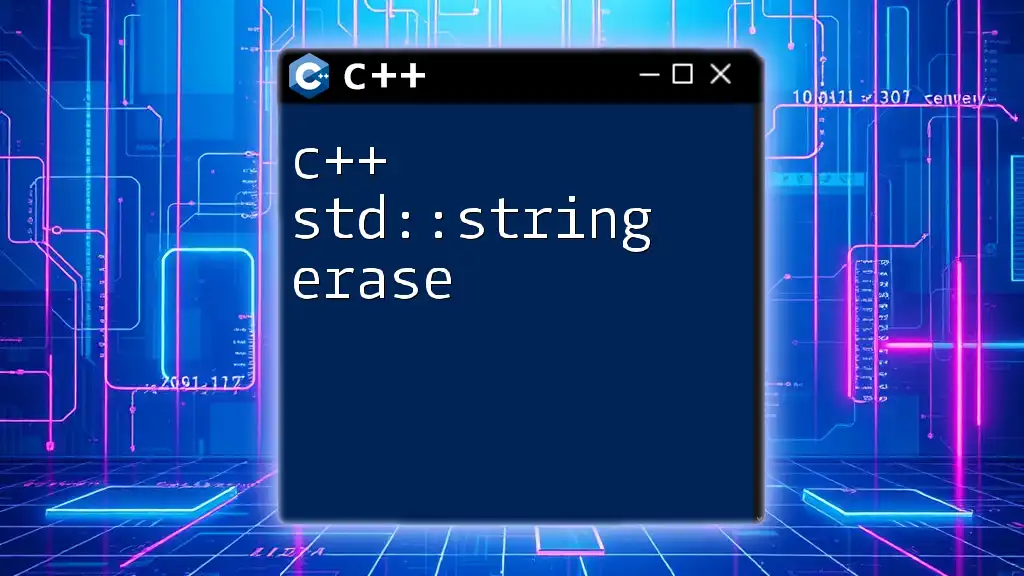
Call to Action
If you found this article helpful, subscribe to our channel for more concise and insightful C++ programming tips and tutorials!