`std::bind` is a function template in C++ that allows you to create a new callable object by binding some arguments to a function or callable, which can be useful for creating functions with fewer parameters or for adapting functions to different contexts.
#include <iostream>
#include <functional>
void printSum(int a, int b) {
std::cout << "Sum: " << a + b << std::endl;
}
int main() {
auto bindPrintSum = std::bind(printSum, 5, std::placeholders::_1);
bindPrintSum(3); // Outputs: Sum: 8
return 0;
}
What is std::bind?
`std::bind` is a powerful utility in C++ that allows developers to bind arguments to functions, generating a new function with some of the original function's arguments pre-set. This binding capability is particularly advantageous when working with function pointers or callbacks, offering flexibility in how functions are called. By using `std::bind`, you can easily adapt existing functions to new contexts without rewriting or duplicating code.
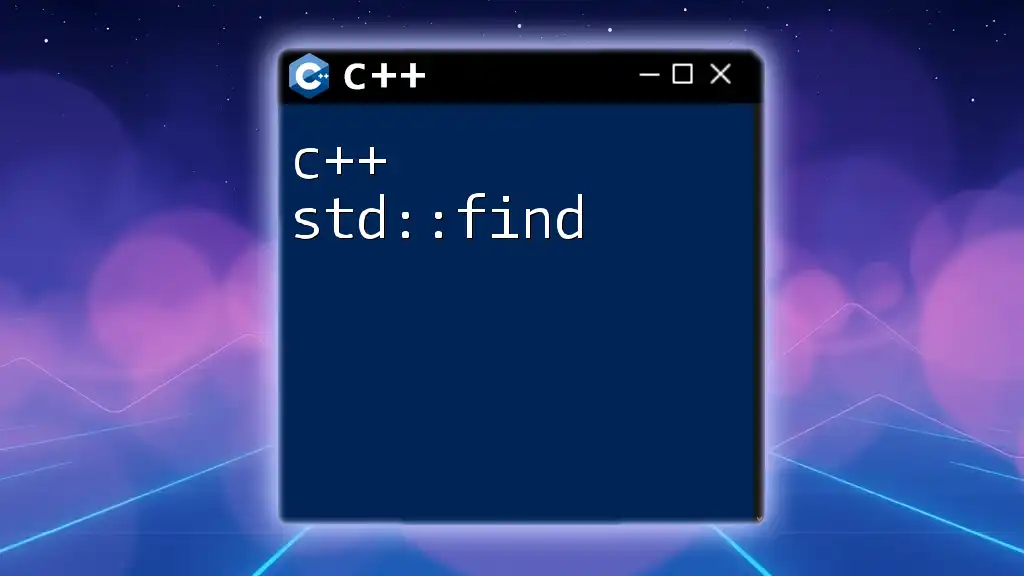
Why Use std::bind?
Using `std::bind` in your C++ code can greatly enhance readability and maintainability. Here are some key benefits:
- Flexibility: You can create new function objects that represent a specific “version” of an existing function with some or all arguments bound.
- Reusability: Functions can be defined once and reused in multiple contexts with different arguments, reducing redundancy.
- Simplicity: In complex scenarios, binding arguments can simplify function calls, making the code clearer and easier to follow.
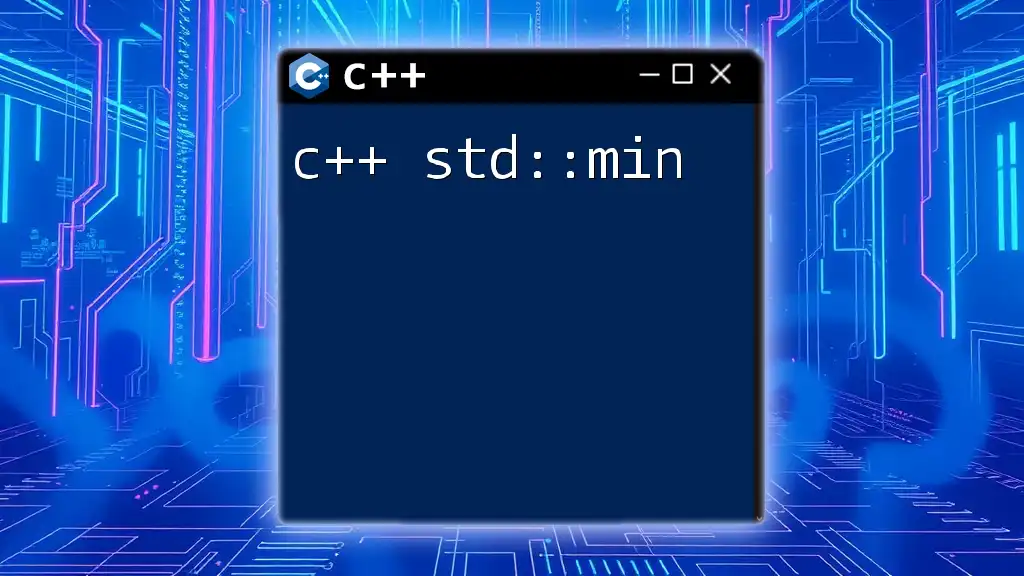
Core Concepts of std::bind
Basic Syntax of std::bind
The syntax for `std::bind` is straightforward:
std::bind(Func func, Args... args);
In this syntax:
- `Func` refers to the function you want to bind.
- `Args...` corresponds to the arguments you want to bind.
Here's a simple example to illustrate its usage:
#include <iostream>
#include <functional>
void greet(const std::string& name) {
std::cout << "Hello, " << name << "!" << std::endl;
}
int main() {
auto greetJohn = std::bind(greet, "John");
greetJohn(); // Output: Hello, John!
return 0;
}
Components of std::bind
-
Function: You can bind free functions, member functions, lambda expressions, and even functors with `std::bind`. This versatility is one of its core strengths.
-
Arguments: The arguments passed to `std::bind` can be either fixed values or placeholders — which substitute for arguments given at the point of the function call. You also can use default values for the arguments depending on the context.
Understanding Placeholders
Placeholders in `std::bind` are represented by `std::placeholders`. These allow you to specify what argument position during the new function’s invocation will be replaced with an actual value. For example:
#include <iostream>
#include <functional>
void multiply(int a, int b) {
std::cout << "Product: " << a * b << std::endl;
}
int main() {
auto doubleValue = std::bind(multiply, std::placeholders::_1, 2);
doubleValue(5); // Output: Product: 10
return 0;
}
In this example, the first argument is bound as a placeholder, allowing flexibility when calling the function.
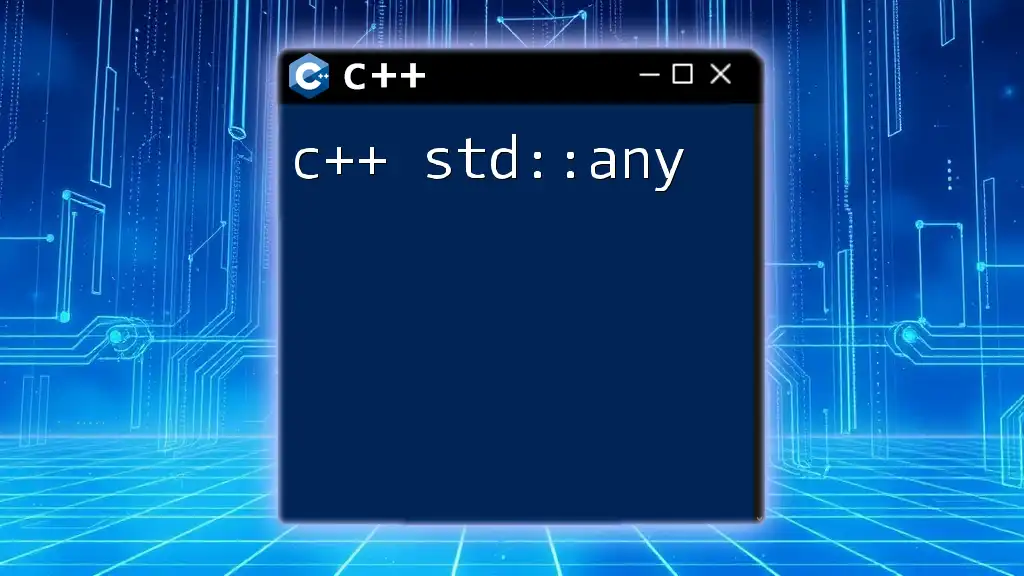
Practical Examples of Using std::bind
Binding Free Functions
You can easily bind free functions using `std::bind`. For instance:
#include <iostream>
#include <functional>
void printSum(int a, int b) {
std::cout << "Sum: " << a + b << std::endl;
}
int main() {
auto addFive = std::bind(printSum, std::placeholders::_1, 5);
addFive(10); // Output: Sum: 15
return 0;
}
In this case, we pre-set the second argument to `5`.
Binding Member Functions
Binding member functions requires an additional parameter for the object instance. Here is an example:
#include <iostream>
#include <functional>
class Multiplier {
public:
void multiply(int a, int b) {
std::cout << "Result: " << a * b << std::endl;
}
};
int main() {
Multiplier m;
auto boundMultiply = std::bind(&Multiplier::multiply, &m, std::placeholders::_1, 4);
boundMultiply(3); // Output: Result: 12
return 0;
}
In this example, a member function `multiply` is bound, allowing us to specify both the instance and one of the parameters.
Binding with Arguments
You can bind multiple arguments as needed, which helps in creating functions tailored to specific use cases. Here’s an example:
#include <iostream>
#include <functional>
void displayInfo(const std::string& name, int age) {
std::cout << name << " is " << age << " years old." << std::endl;
}
int main() {
auto johnInfo = std::bind(displayInfo, "John", std::placeholders::_1);
johnInfo(28); // Output: John is 28 years old.
return 0;
}
Here, the name is fixed while the age can vary.
Combining std::bind with STL Algorithms
A significant benefit of `std::bind` is its compatibility with Standard Template Library (STL) algorithms. For instance, you can use it with `std::for_each`:
#include <iostream>
#include <vector>
#include <algorithm>
#include <functional>
void printSquare(int n) {
std::cout << n * n << std::endl;
}
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::for_each(numbers.begin(), numbers.end(), std::bind(printSquare, std::placeholders::_1));
return 0;
}
This code snippet will print the square of each number in the list, showcasing how `std::bind` can streamline operations on collections.
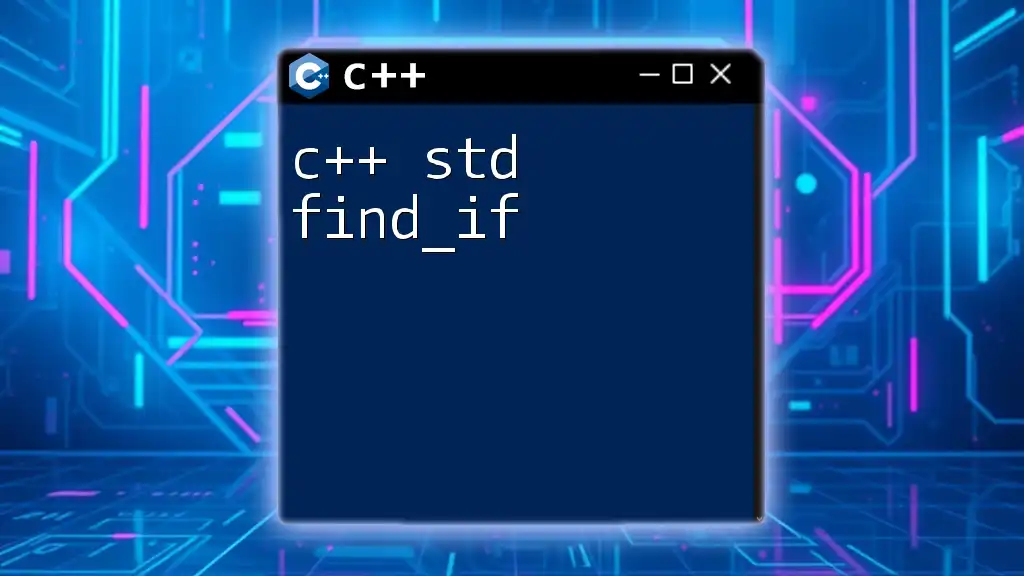
Advanced Usage of std::bind
Error Handling with std::bind
When using `std::bind`, be mindful of potential issues such as binding too many or too few arguments or binding to invalid function types. To handle errors gracefully, ensure that the types and number of bound arguments match the expectations of the function being called.
Performance Considerations
While `std::bind` offers many advantages, consider its performance implications. In certain scenarios, lambdas can provide more optimized performance compared to `std::bind`. It is worth profiling both methods to determine which is more efficient for your use case. Generally, `std::bind` generates more overhead due to additional layers of abstraction it introduces.
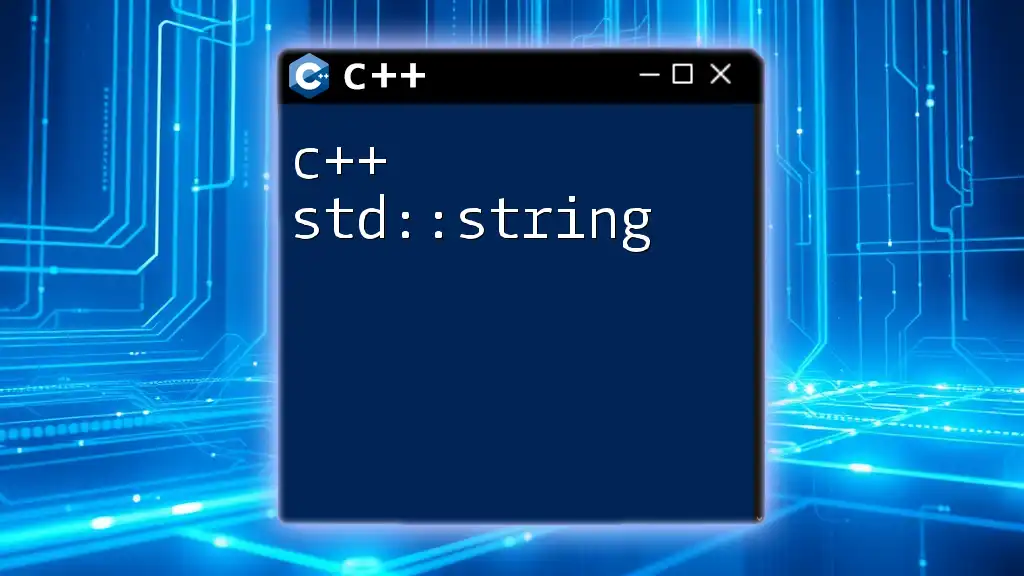
Frequently Asked Questions (FAQ)
Common Confusions about std::bind
Many developers wonder about the differences between `std::bind` and lambda functions. While both can achieve similar goals, `std::bind` is primarily focused on binding arguments, whereas lambdas provide an anonymous function with more flexible capture options.
When to Use std::bind vs. Lambda Functions
- Use std::bind if you need to bind function arguments and have a clean position-based calling format of your function.
- Opt for lambda functions for simplicity and where you might want to encapsulate more complex operations or when you want to capture multiple variables.
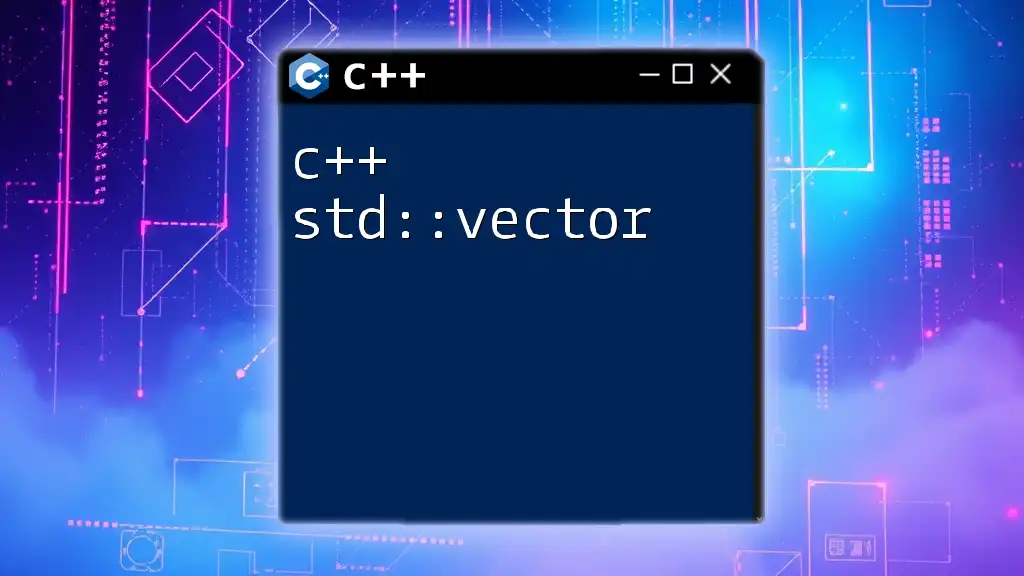
Conclusion
In summary, `c++ std::bind` opens up a world of possibilities for adapting and reusing functions in your code. Whether you are binding free functions or member functions, the ability to partially apply arguments makes your code cleaner and easier to maintain. With practice, you can leverage `std::bind` to improve both the readability and functionality of your C++ applications.
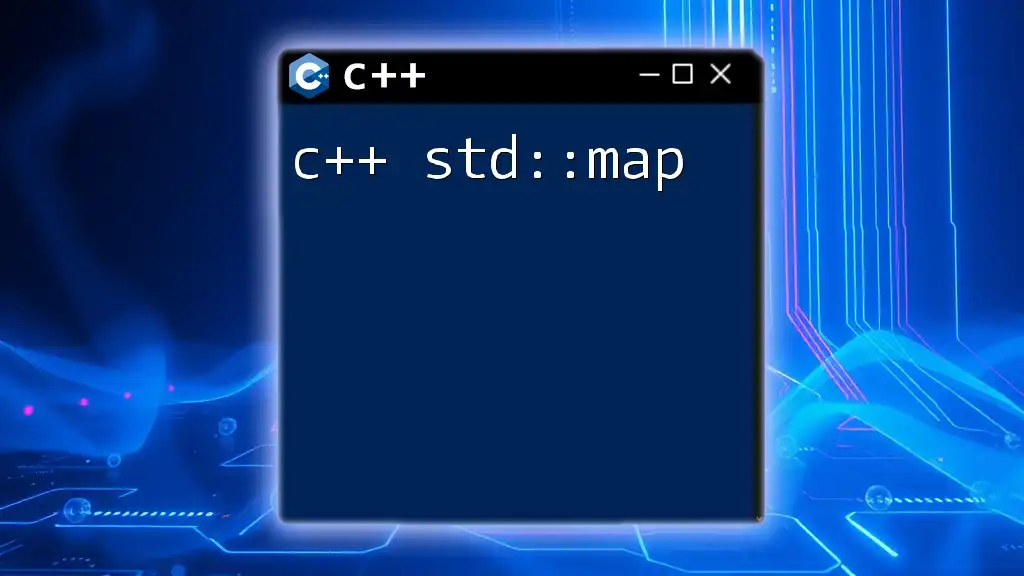
Further Reading and Resources
To deepen your understanding of `std::bind` and C++ in general, consider reviewing the official C++ documentation. There are also various books and online tutorials that provide in-depth explorations of functional programming in C++.