The `std::queue` in C++ is a container adapter that provides a First-In-First-Out (FIFO) data structure, allowing efficient addition and removal of elements from the front and back.
Here's a code snippet demonstrating its basic usage:
#include <iostream>
#include <queue>
int main() {
std::queue<int> q;
// Adding elements to the queue
q.push(10);
q.push(20);
q.push(30);
// Displaying and removing elements from the queue
while (!q.empty()) {
std::cout << q.front() << " "; // Access the front element
q.pop(); // Remove the front element
}
return 0;
}
Understanding Queues in C++
What is a Queue?
A queue is a fundamental data structure that operates on the First In, First Out (FIFO) principle. This means that the first element added to the queue is the first one to be removed. Imagine a line of people waiting for tickets; the person who arrives first will be served first. This analogy effectively captures the essence of how queues function in computing.
Queues are essential in various computing scenarios, particularly in situations where a system must maintain a set of tasks or processes that need to be executed in a specific order.
When to Use a Queue in C++
Queues are incredibly useful in numerous applications. Here are some common scenarios:
- Task Scheduling: When processes or tasks need to be executed in the order they arrive.
- Print Queue Management: Jobs sent to a printer are processed in the order they are received.
- Breadth-First Search in Graph Algorithms: Queues can help maintain the next vertices to explore.
Understanding when to employ a queue can significantly enhance your programming efficiency and problem-solving skills.
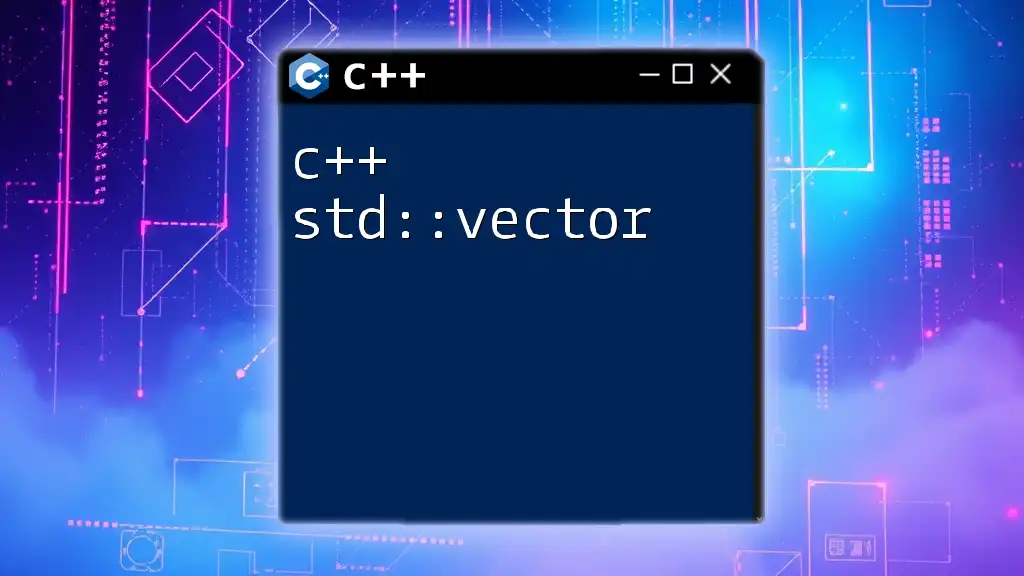
C++ std::queue Overview
Introduction to C++ std::queue
The C++ Standard Library (STL) is a powerful collection of template classes facilitating efficient programming with data structures. Among these is the `std::queue`, which provides access to the functionalities and features of queue data structures with the convenience of C++ syntax.
The `std::queue` class wraps various operations, allowing programmers to manage a collection of elements that follow the FIFO principle seamlessly.
Key Features of std::queue in C++
The `std::queue` class comes with several key features that make it a preferred choice among developers:
- Container Agnostic: `std::queue` can be implemented using different underlying containers such as `std::deque` or `std::list`. This flexibility allows optimizations based on specific use cases.
- Automatic Memory Management: Developers can focus on the logic of their applications while the C++ standard library handles memory allocation and deallocation under the hood.
- Exception Safety: Operations like `pop()` and `front()` are designed to be safely executed, minimizing the chance of crashes due to invalid operations.
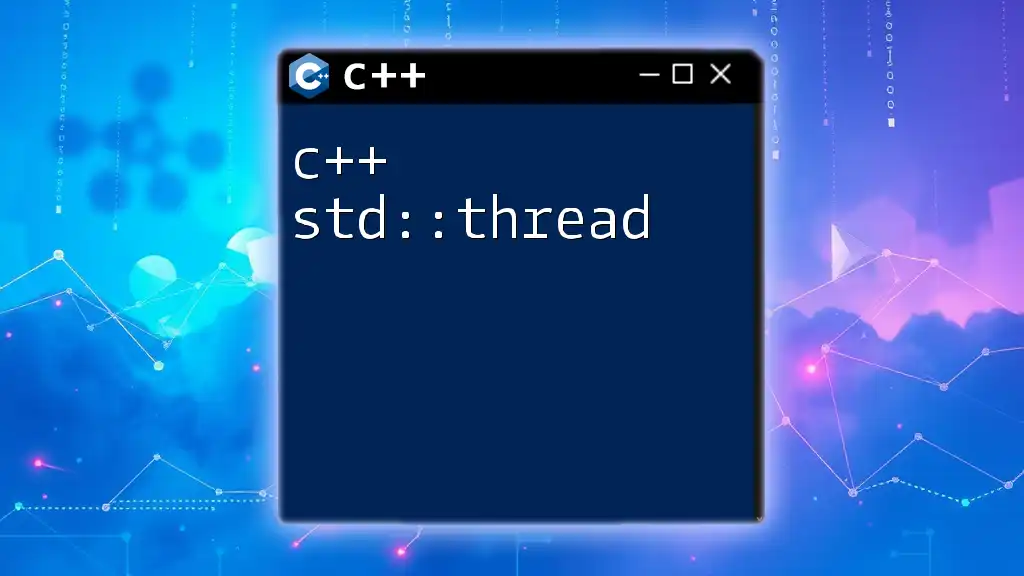
Basic Operations of std::queue
Creating a std::queue
To utilize `std::queue`, you first need to declare one. The syntax is intuitive and straightforward. Here’s a simple example:
#include <iostream>
#include <queue>
int main() {
std::queue<int> myQueue;
return 0;
}
This code snippet initializes an empty queue capable of holding integers.
Adding Elements: enqueue
To add elements to a queue, you use the `push()` method. This action places the new element at the back of the queue, preserving the FIFO order. Here’s how to do it:
myQueue.push(10);
myQueue.push(20);
In this code, `10` is added first, followed by `20`. When elements are dequeued, they will be removed in this exact order.
Removing Elements: dequeue
The removal of elements from a queue is achieved with the `pop()` method. This method removes the front element of the queue, which is the oldest added element:
myQueue.pop(); // Removes the front element.
Note that if you call `pop()` on an empty queue, it results in undefined behavior; hence, it is wise to check if the queue is non-empty before performing this action.
Accessing Front and Back Elements
You can access the front and back elements of a `std::queue` using `front()` and `back()` methods, respectively. This can be quite handy when you want to see what's next in line without modifying the queue. Here’s an example:
std::cout << myQueue.front(); // Access front element
std::cout << myQueue.back(); // Access back element
These methods allow you to retrieve the values while maintaining the integrity of the queue structure.
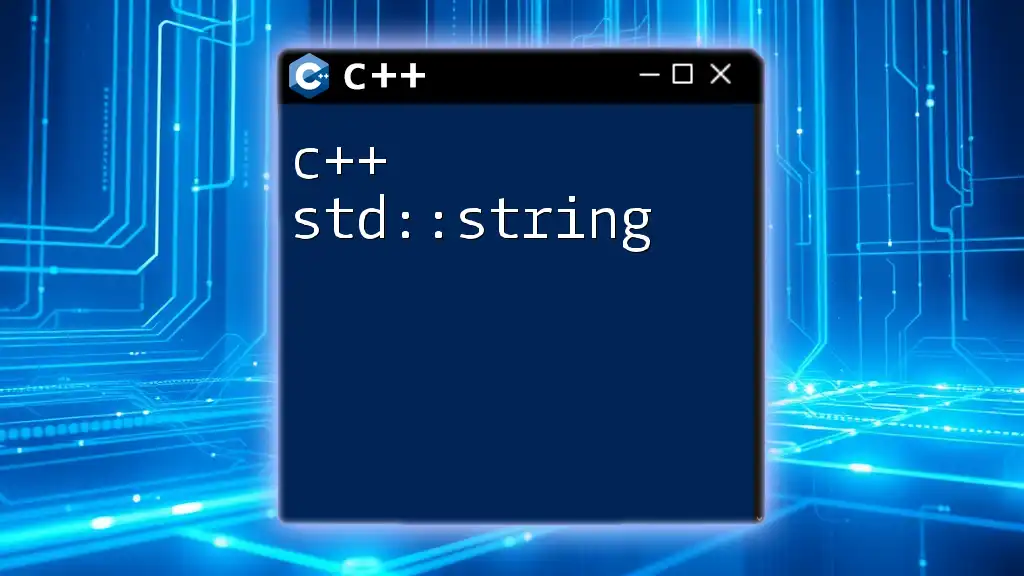
Practical Examples
Example 1: Task Scheduling Using C++ std::queue
Consider a simple task scheduler where each task is represented by an ID. The following program demonstrates how to use the `std::queue` to simulate task scheduling:
#include <queue>
#include <iostream>
class Task {
public:
int id;
Task(int id): id(id) {}
};
int main() {
std::queue<Task> taskQueue;
taskQueue.push(Task(1));
taskQueue.push(Task(2));
while (!taskQueue.empty()) {
std::cout << "Processing Task ID: " << taskQueue.front().id << std::endl;
taskQueue.pop();
}
return 0;
}
In this example, tasks are processed in the order they were added, thus demonstrating the first-in, first-out nature of queues.
Example 2: Customers at a Bank
To illustrate how queues work in real-life applications, we can simulate a bank where customers are served in the order they come in:
#include <queue>
#include <iostream>
int main() {
std::queue<std::string> bankQueue;
bankQueue.push("Customer 1");
bankQueue.push("Customer 2");
while (!bankQueue.empty()) {
std::cout << bankQueue.front() << " is being served." << std::endl;
bankQueue.pop();
}
return 0;
}
In this case, the output shows that customers are served sequentially, just like in a real bank.
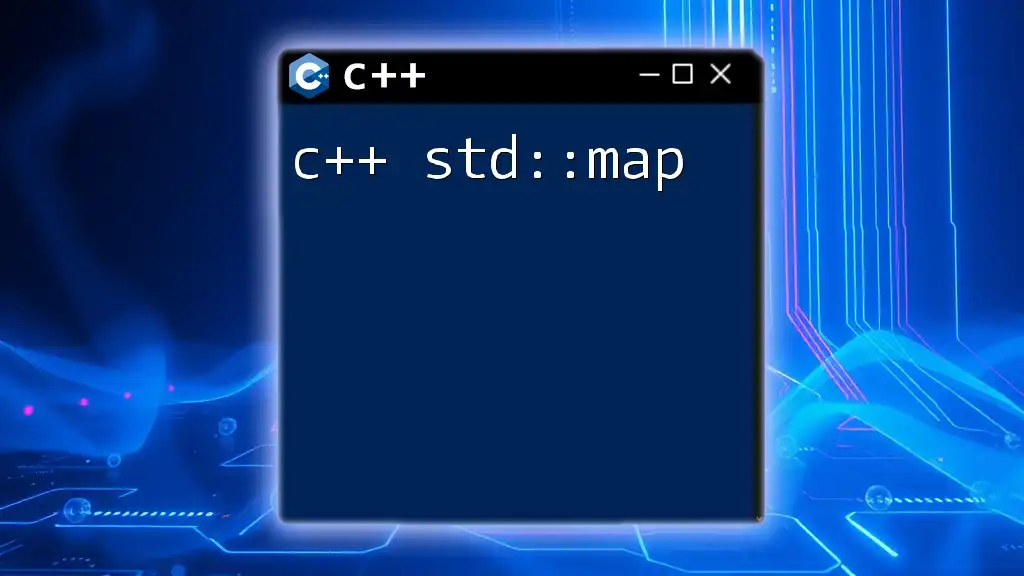
Advanced Usage of std::queue
Empty and Size Methods
Understanding the state of a queue is vital, especially when dealing with dynamic data. The `empty()` and `size()` methods provide useful insights:
if (myQueue.empty()) {
std::cout << "Queue is empty." << std::endl;
}
std::cout << "Size of the queue: " << myQueue.size() << std::endl;
Use `empty()` to check if there are elements to process and `size()` to get the total number of elements currently in the queue.
Custom Data Structures in std::queue
You can utilize `std::queue` with custom data structures, allowing greater practical application. Here’s an example where we enqueue objects of a user-defined class:
#include <queue>
#include <iostream>
#include <string>
class Customer {
public:
std::string name;
Customer(std::string name): name(name) {}
};
int main() {
std::queue<Customer> customerQueue;
customerQueue.push(Customer("Alice"));
customerQueue.push(Customer("Bob"));
while (!customerQueue.empty()) {
std::cout << customerQueue.front().name << " is served." << std::endl;
customerQueue.pop();
}
return 0;
}
This code allows you to manage complex data types effortlessly while leveraging the advantages of `std::queue`.
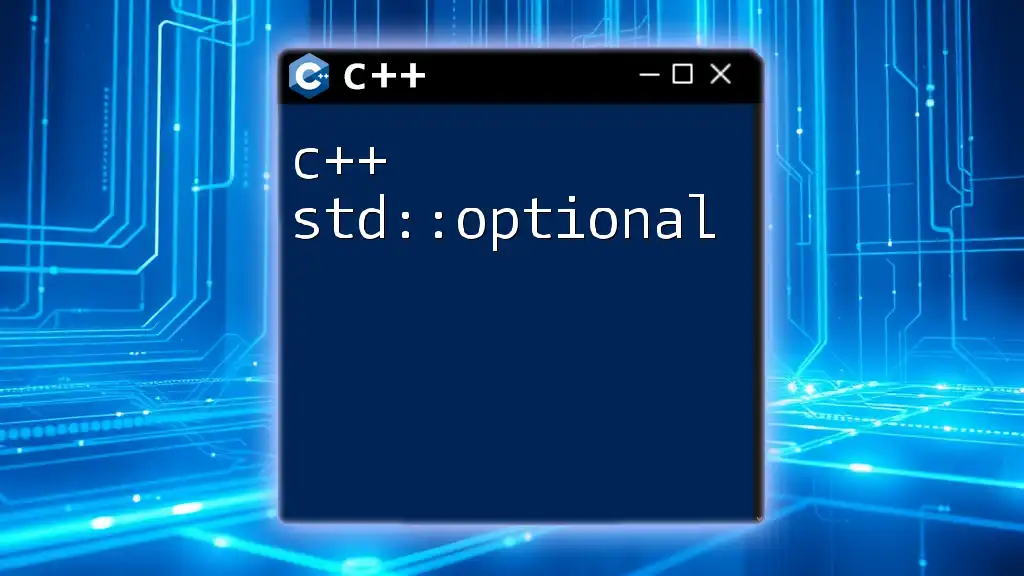
Common Mistakes and Troubleshooting
Memory Management Concerns
While `std::queue` takes care of memory management for you, it is crucial to understand that issues can arise if you attempt to pop elements from an empty queue. Always ensure you check for emptiness before accessing or modifying the queue.
Performance Tuning
Performance considerations should not be overlooked when implementing queues. If you often insert and remove elements, `std::deque` is typically a better choice as the underlying container for `std::queue`, compared to `std::list`.
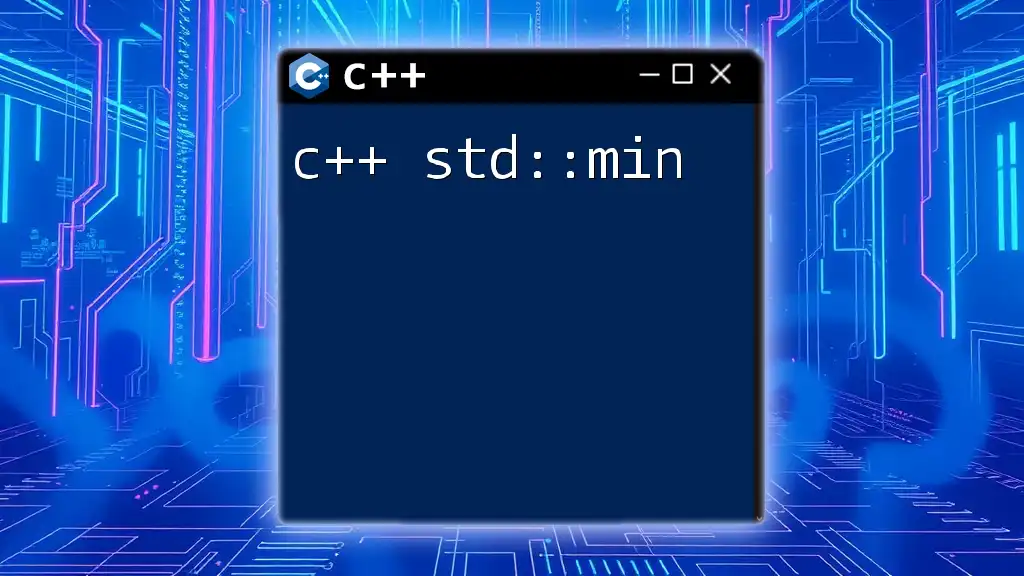
Conclusion
The C++ std::queue class is a powerful and flexible tool for managing collections of data that follow the FIFO principle. By mastering its operations and understanding its features, you can improve the efficiency and clarity of your programming tasks. Whether it's for simple tasks or complex data management scenarios, `std::queue` simplifies the process, allowing you to focus on your application's logic rather than intricate data structure management.
As you continue to explore C++ and its capabilities, practicing the use of `std::queue` in various projects will prove invaluable, enriching your problem-solving toolkit and enhancing your software development skills.
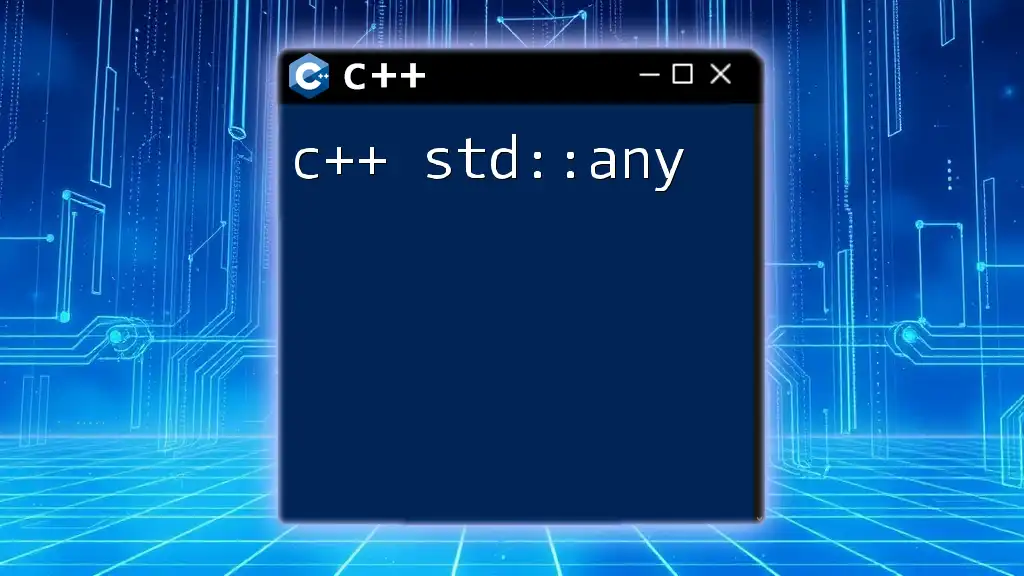
Additional Resources
For further learning and exploration, consider delving into the official C++ documentation and various tutorial resources, which can provide deeper insights and additional examples to enhance your understanding of `std::queue` and its applications.