The `std::copy` function in C++ is used to copy a range of elements from one location to another in a straightforward way.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> source = {1, 2, 3, 4, 5};
std::vector<int> destination(5);
std::copy(source.begin(), source.end(), destination.begin());
for (const auto &elem : destination) {
std::cout << elem << " ";
}
return 0;
}
What is `std::copy`?
`std::copy` is a powerful algorithm provided by the C++ Standard Library. This function is used to copy a range of elements from a source range to a destination range. It is widely utilized for its ability to enhance code clarity and efficiency by abstracting the details of copying.

Why Use `std::copy`?
Using `std::copy` comes with multiple advantages:
- Performance: The implementation of `std::copy` is highly optimized, often outperforming manual copying techniques, especially in large datasets.
- Code Readability: Using this standard function makes your intentions clear to anyone reading the code. It reduces the need for explicit loops and index management.
- Maintainability: By leveraging standard library functions, your code becomes less prone to bugs and easier to modify or extend in the future.
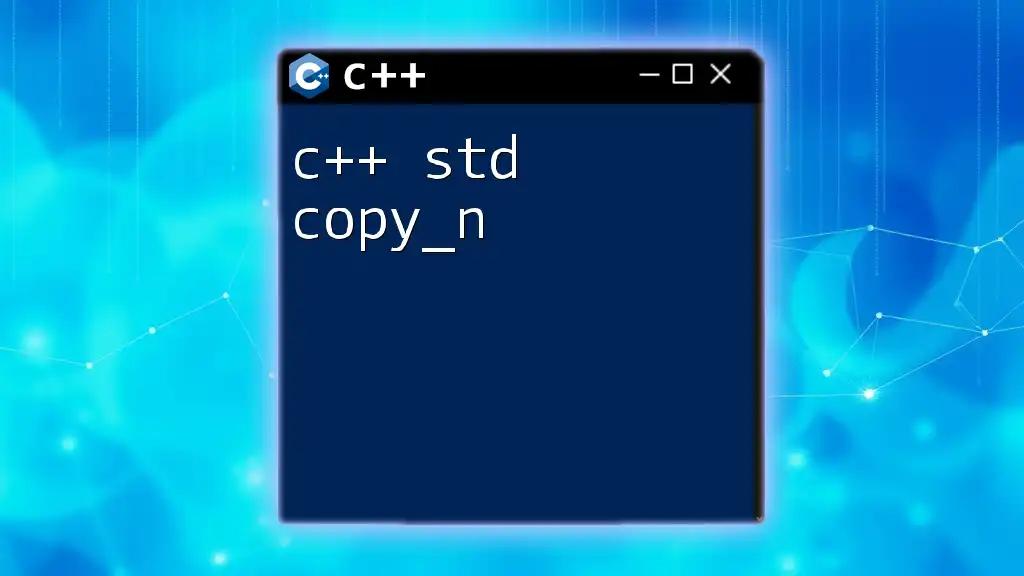
Basic Syntax of `std::copy`
Function Signature
The syntax of `std::copy` can be expressed through its function signature:
template <class InputIt, class OutputIt>
OutputIt copy(InputIt first, InputIt last, OutputIt d_first);
Parameters Explained
- InputIt first: This refers to the beginning of the source range from where elements will be copied.
- InputIt last: This indicates the end of the source range. The last element is not included in the copy operation.
- OutputIt d_first: This points to the beginning of the destination range where the copied elements will be placed.
Return Value
`std::copy` returns an iterator pointing to the end of the destination range, which can be useful for chaining operations or confirming the completion of the copy.
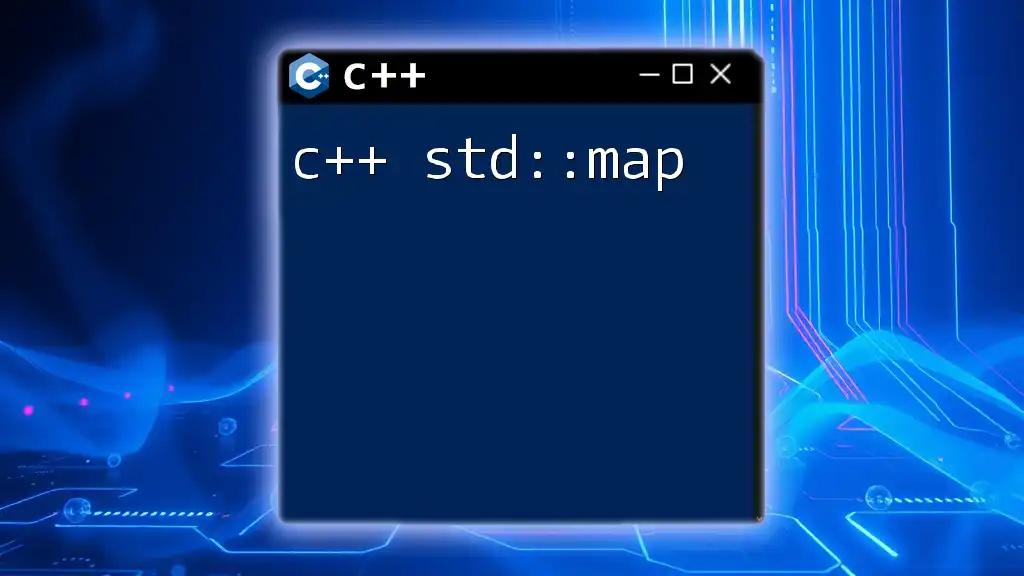
How to Use `std::copy`
Copying from Array to Array
One of the simplest uses of `std::copy` is to copy elements from one array to another.
int src[5] = {1, 2, 3, 4, 5};
int dest[5];
std::copy(src, src + 5, dest);
In this example:
- The source array `src` has five integer elements.
- `std::copy` copies all elements from `src` to `dest`.
- The resulting `dest` array will contain `{1, 2, 3, 4, 5}`.
Copying from Vector to Vector
Another common scenario is copying elements from one `std::vector` to another.
std::vector<int> source = {1, 2, 3, 4, 5};
std::vector<int> destination(5); // Initializing with appropriate size
std::copy(source.begin(), source.end(), destination.begin());
Here:
- The source `std::vector` contains five integers.
- A destination vector is predefined with the same size.
- The `std::copy` function transfers elements from `source` to `destination`, resulting in an identical vector.
Using Iterators with Custom Containers
`std::copy` can also be utilized with custom containers, thereby enhancing flexibility.
class CustomContainer {
public:
std::vector<int> data;
void add(int value) { data.push_back(value); }
};
// Fill sourceContainer with some values
CustomContainer sourceContainer;
sourceContainer.add(1);
sourceContainer.add(2);
sourceContainer.add(3);
CustomContainer destContainer;
std::copy(sourceContainer.data.begin(), sourceContainer.data.end(), std::back_inserter(destContainer.data));
In this example:
- A `CustomContainer` class is created with a vector to store integers.
- After adding values to `sourceContainer`, `std::copy` is used to transfer these elements into `destContainer` using `std::back_inserter`, which appends new elements to the existing vector.
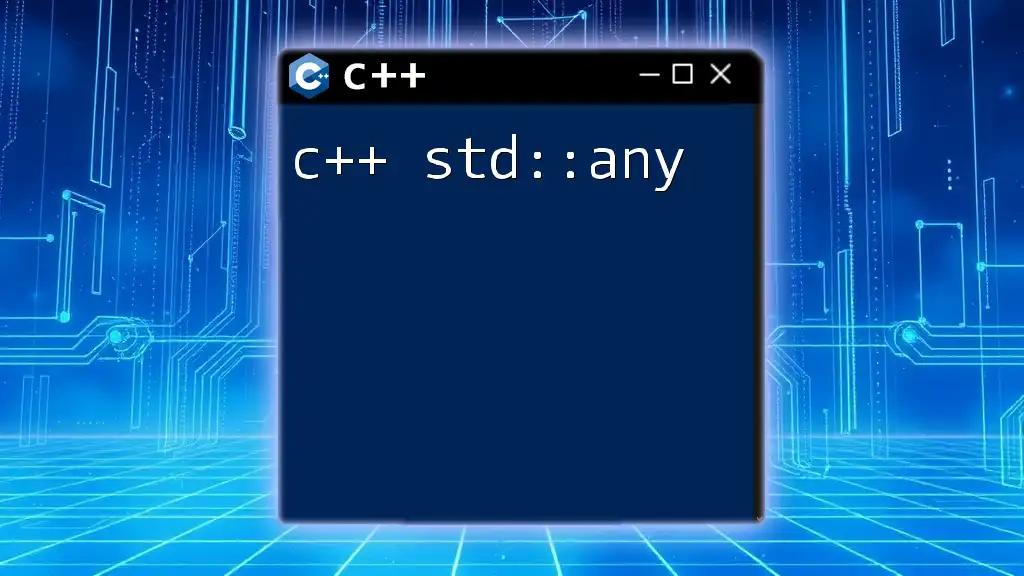
Important Considerations
Overlapping Ranges
When using `std::copy`, it's crucial to be aware of overlapping ranges. If the source and destination areas overlap, it can lead to unexpected behavior or data corruption. In such cases, consider using `std::copy_backward`, which handles overlapping ranges correctly by copying elements from the end towards the beginning.
Types of Elements
`std::copy` requires that the elements being copied are copyable. If you consider elements that are not copyable, such as unique pointers or certain objects, you should use `std::move` in conjunction with iterators to transfer ownership instead:
std::vector<std::unique_ptr<int>> source;
std::vector<std::unique_ptr<int>> destination;
std::move(source.begin(), source.end(), std::back_inserter(destination));
Handling Different Container Types
You can also utilize `std::copy` to copy between different types of containers, such as from an array to a vector.
int arr[] = {1, 2, 3};
std::vector<int> vec(3);
std::copy(std::begin(arr), std::end(arr), vec.begin());
In this example, elements from the array `arr` are copied into the `std::vector` `vec`.
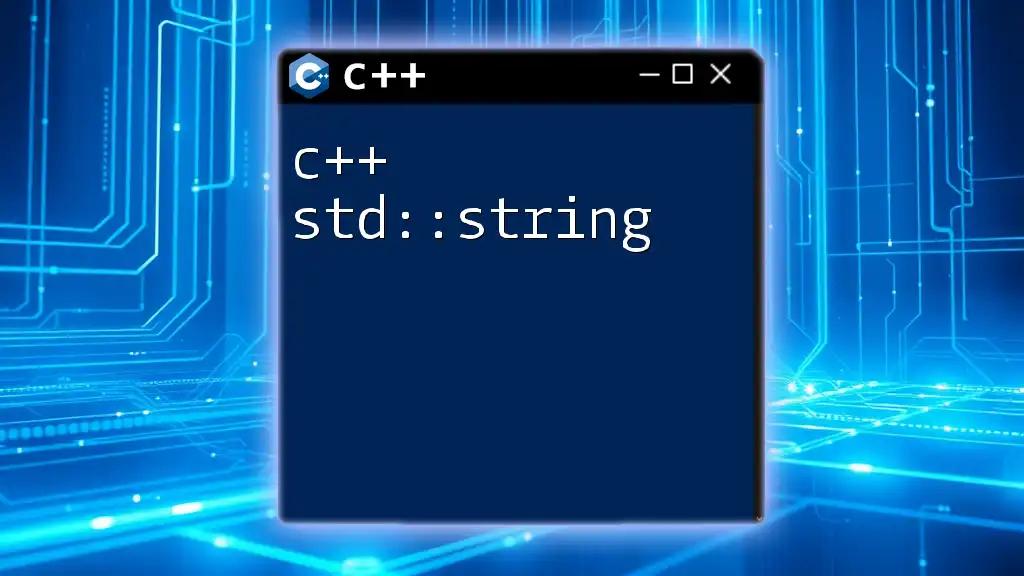
Performance Tips for Using `std::copy`
When to Use `std::copy`
Whenever you need to copy a range of elements in C++, `std::copy` is often the best option due to its optimized performance and clear semantics. It becomes particularly beneficial in algorithms that require data manipulation, such as filtering and transforming collections.
Avoiding Common Pitfalls
Be mindful of:
- Empty containers: If either the source or destination container is empty, ensure proper handling to avoid runtime errors.
- Insufficient destination size: Always ensure that the destination has sufficient space allocated to avoid buffer overruns, which can lead to undefined behavior.
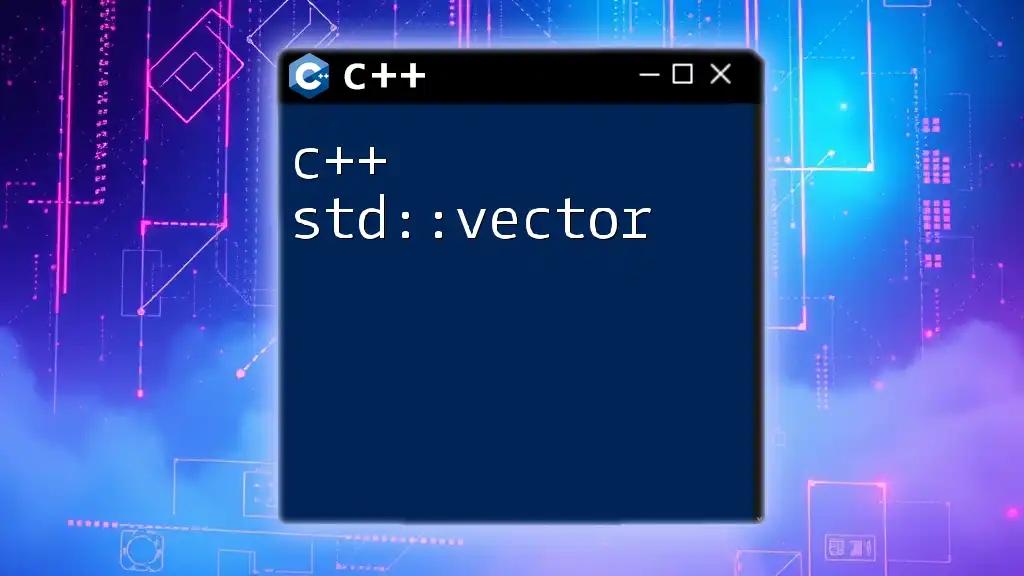
Conclusion
Recap of Key Takeaways
The C++ `std::copy` function serves as a vital tool in the arsenal of any C++ programmer. Understanding how to efficiently copy elements between ranges will elevate your coding proficiency and contribute to building more maintainable and performant applications.
Encouragement to Practice
To master `std::copy`, practice by implementing it in various projects. Experiment with different data structures and containers. Utilize online coding platforms to challenge yourself and assess your understanding of this powerful function.
Call to Action
Share your experiences with `std::copy` in the comments below. What has your experience been like using it in your projects?

Additional Resources
For further reading, consider visiting the official C++ documentation on `std::copy`, exploring coding platforms like LeetCode or HackerRank for hands-on practice, and perusing recommended books that dive deeper into the C++ Standard Library.