In C++, the `copy` algorithm from the Standard Library is used to duplicate elements from one range to another.
Here’s a simple code snippet demonstrating this:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> original = {1, 2, 3, 4, 5};
std::vector<int> copy(5);
std::copy(original.begin(), original.end(), copy.begin());
for (int num : copy) {
std::cout << num << " ";
}
return 0;
}
Understanding Copy Semantics
What is Copy Semantics?
In C++, copy semantics refer to how objects are duplicated in memory. When you create a copy of an object, there are important considerations to determine whether you're making a shallow copy or a deep copy. A shallow copy creates a new object that shares the same memory as the original, which can lead to issues such as dangling pointers if the original object goes out of scope. In contrast, a deep copy creates a completely independent copy, allocating new memory space for the copy's data. Understanding the distinctions between these two types of copying is crucial for effective memory management and avoiding unintended behaviors in programs.
Copy Constructor
The copy constructor is a special constructor in C++ that initializes a new object as a copy of an existing object. It is called when a new object is created from an existing object, as exemplified below:
class MyClass {
public:
int x;
MyClass(int val) : x(val) {}
MyClass(const MyClass &obj) : x(obj.x) {} // Copy constructor
};
MyClass obj1(10);
MyClass obj2 = obj1; // obj2 is a copy of obj1
In this example, `obj2` is a new instance of `MyClass` that is initialized using the value of `obj1`. The copy constructor ensures that the data of `obj1` is used to initialize `obj2`.
Copy Assignment Operator
The copy assignment operator serves a specific purpose when assigning one existing object to another. It must take care of self-assignment and manage resource duplication properly. Here's how to implement a copy assignment operator:
class MyClass {
public:
int x;
MyClass(int val) : x(val) {}
MyClass& operator=(const MyClass &obj) {
if(this != &obj) {
x = obj.x; // Copy the value
}
return *this;
}
};
In this code snippet, the assignment operator checks for self-assignment (i.e., `this` is not the same as `obj`) to prevent inadvertently copying over itself, which could lead to data corruption.
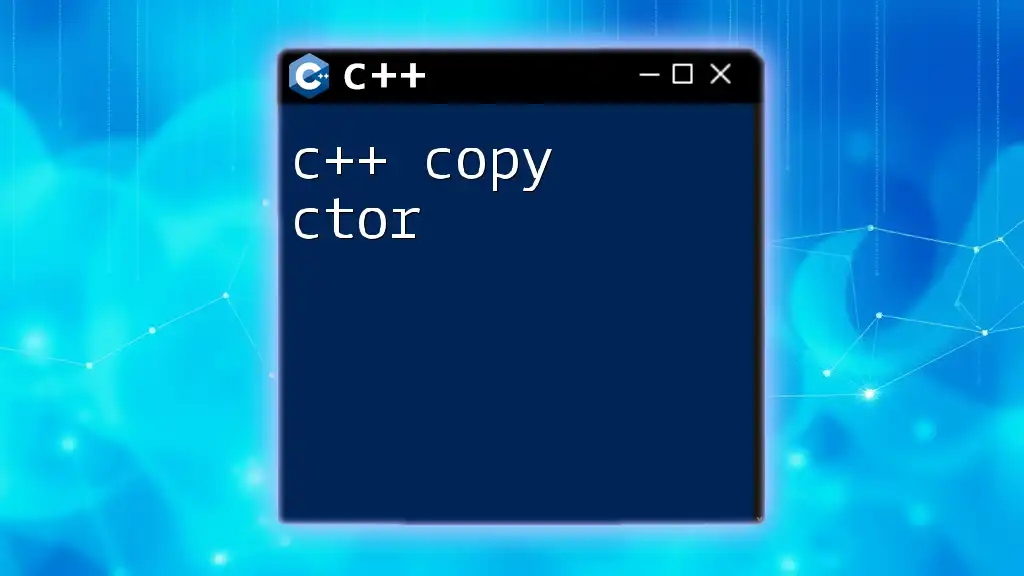
Types of Copy in C++
Shallow Copy vs Deep Copy
Shallow copies can lead to issues because they do not create copies of the data themselves. Instead, both the original and the copied object point to the same memory location. This means that if one object modifies the data, the other is affected as well.
In contrast, deep copies are safer and preferred when dealing with dynamic memory. They ensure that both objects encapsulate their own copies of data, safeguarding against unintentional interferences.
Standard Library Copy Functions
The C++ Standard Library provides various functions designed for copying, such as `std::copy`. This function can be used to copy elements from one container to another. Here's an example of how to use `std::copy` with vectors:
#include <algorithm>
#include <vector>
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2(3);
std::copy(vec1.begin(), vec1.end(), vec2.begin());
In this example, `vec1`'s elements are copied to `vec2`. Utilizing standard library functions not only saves time but also ensures optimized internally managed operations.
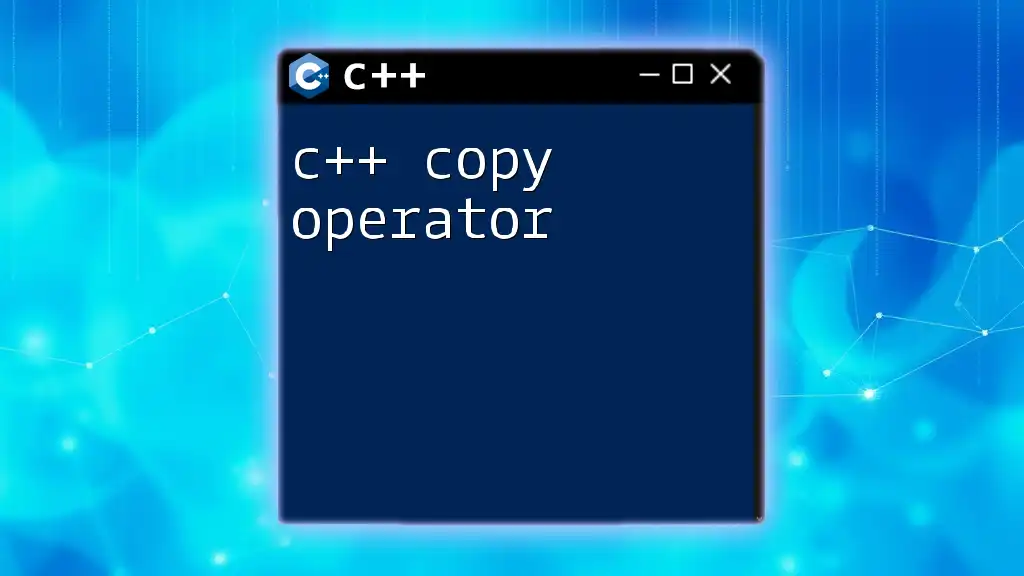
Best Practices for Copying in C++
Rule of Three
The Rule of Three states that if a class defines one of the following: a destructor, a copy constructor, or a copy assignment operator, it should probably define all three. This guideline is vital for ensuring proper resource management, particularly when dealing with dynamically allocated memory. Here’s an example illustrating the Rule of Three:
class Resource {
public:
int* data;
Resource(int value) : data(new int(value)) {}
~Resource() { delete data; }
Resource(const Resource &other) : data(new int(*other.data)) {} // Copy constructor
Resource& operator=(const Resource &other) {
if(this != &other) {
delete data; // Clean up existing resource
data = new int(*other.data); // Deep copy
}
return *this;
}
};
In this class, all three functions are properly defined to manage the dynamic memory allocated for `data`.
Rule of Five
With the introduction of C++11, the Rule of Five expands upon the Rule of Three, adding the concepts of the move constructor and move assignment operator. Move semantics allow resources to be moved rather than copied, improving performance. Here’s a brief example:
class MyClass {
public:
int* data;
MyClass(int value) : data(new int(value)) {}
// Move constructor
MyClass(MyClass&& other) noexcept : data(other.data) {
other.data = nullptr; // Transfer ownership
}
// Move assignment operator
MyClass& operator=(MyClass&& other) noexcept {
if(this != &other) {
delete[] data; // Free current resource
data = other.data; // Move the resource
other.data = nullptr; // Nullify the moved-from object
}
return *this;
}
};
In this case, when an object is moved, ownership of the resource is efficiently transferred, allowing for better performance, especially when dealing with large datasets.
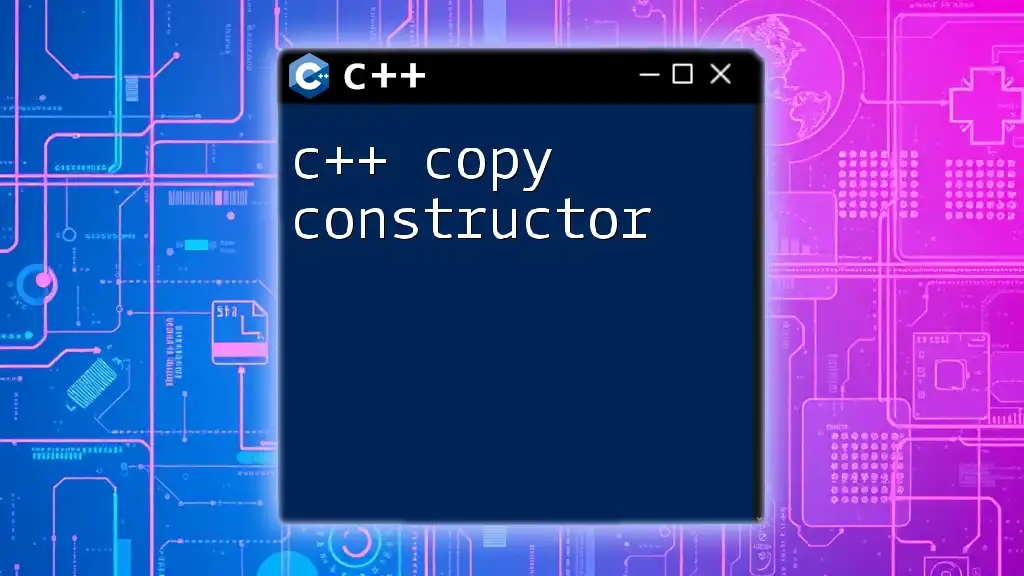
Common Errors and Debugging Tips
Memory Management Issues
Memory management issues can arise from incorrect implementations of copy constructors and assignment operators. Common problems include memory leaks, where allocated memory is never freed, and double deletions, which occur when an object is deleted more than once. To debug such issues, use tools like Valgrind or AddressSanitizer to track memory usage and identify potential leaks.
Performance Considerations
When dealing with large objects or data sets, copying can become costly in terms of performance. Instead of making copies, consider using references or pointers whenever feasible. This helps minimize the overhead associated with unnecessarily copying large amounts of data.
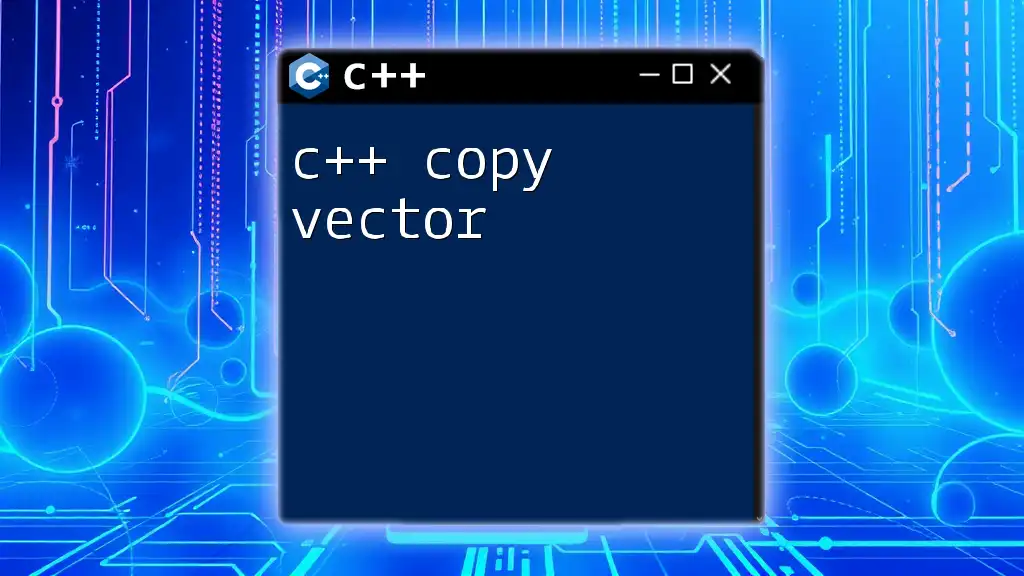
Conclusion
Understanding the intricacies of C++ copy operations is essential for anyone looking to write efficient and effective code. Mastery of the copy constructor, copy assignment operator, and the nuances of shallow and deep copying can prevent common pitfalls in memory management. Remember to practice these concepts to solidify your understanding and improve your programming skills.
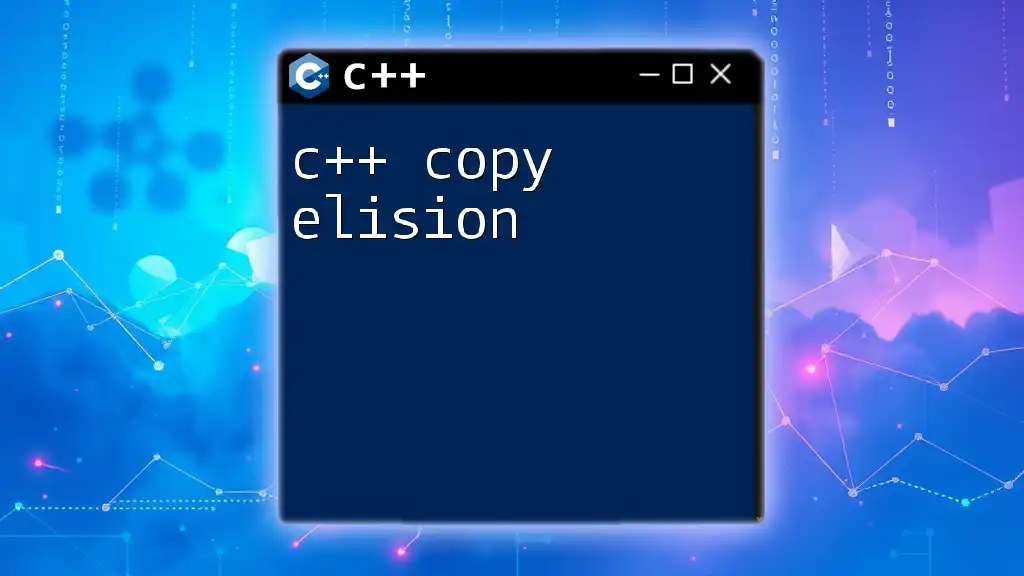
Additional Resources
For further exploration of C++ copying concepts, consider diving into authoritative resources such as books on modern C++, online tutorials specifically covering copy semantics, or courses tailored to deepen your knowledge in object management within C++.