In C++, you can copy a struct by simply assigning one instance of the struct to another, which triggers a shallow copy of its attributes. Here’s a code snippet demonstrating this:
#include <iostream>
struct Point {
int x;
int y;
};
int main() {
Point p1 = {1, 2}; // Original struct
Point p2 = p1; // Copying struct using assignment
std::cout << "Point p2: (" << p2.x << ", " << p2.y << ")" << std::endl; // Output: Point p2: (1, 2)
return 0;
}
Understanding Structs in C++
What is a Struct?
A struct in C++ is a user-defined data type that allows the grouping of related variables together. Structs are particularly useful for organizing complex data and can represent real-world entities, such as a Person or a Car.
While structs and classes are similar, the primary distinction is that structs have public members by default, whereas classes have private members. This makes structs ideal for creating simple data structures without requiring encapsulation.
Basic Syntax of Structs
To define a struct in C++, you use the `struct` keyword followed by its name and a pair of curly braces containing the member variables. Here is a basic example:
struct Person {
std::string name;
int age;
};
In this example, `Person` is a struct containing two members: a string for the name and an integer for the age. You can easily create instances of this struct and access their members.
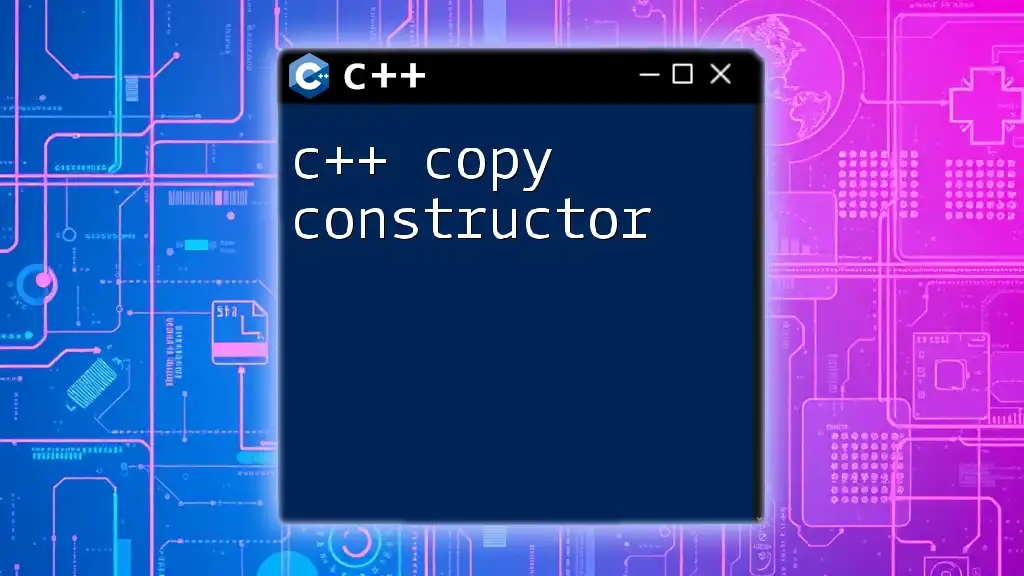
Copy Semantics in C++
What is Copy Semantics?
Copy semantics refer to how objects are copied in C++. When you assign one object to another or pass it to a function, C++ creates a copy of the original object. Understanding copy semantics is vital for managing the resources efficiently, especially when dealing with dynamic memory or complex data structures.
Copying Structs: The Default Behavior
C++ provides a built-in copy constructor and copy assignment operator for structs by default. When you create a new struct as a copy of an existing one, the member variables are copied directly. Consider the following example:
Person person1 = {"John", 30};
Person person2 = person1; // Copying person1 to person2
// Now person2 has a copy of person1's members
In this case, `person2` will have its own individual copy of `name` and `age`. This behavior is known as shallow copy, meaning that the actual values are duplicated rather than referenced.
Shallow vs. Deep Copy
Understanding the difference between shallow and deep copy is essential, especially when your structs contain pointers or refer to dynamic memory.
-
Shallow Copy: Only the pointers are copied, not the actual objects they point to. This can lead to issues such as dangling pointers or double deletion.
-
Deep Copy: A complete copy of the object is created, along with any dynamically allocated memory, ensuring that each object is independent.
Here's an illustration:
struct Node {
int value;
Node* next;
};
Node* shallowCopy(Node* original) {
return original; // Just copying the pointer
}
Node* deepCopy(Node* original) {
Node* newNode = new Node();
newNode->value = original->value;
newNode->next = original->next ? new Node(*original->next) : nullptr; // Create a new copy of the next node
return newNode;
}
In the `shallowCopy` function, the `original` pointer is returned directly, leading to a shared memory situation. In contrast, `deepCopy` ensures that a new `Node` object is created along with its `next` pointer, resulting in separate memory ownership.
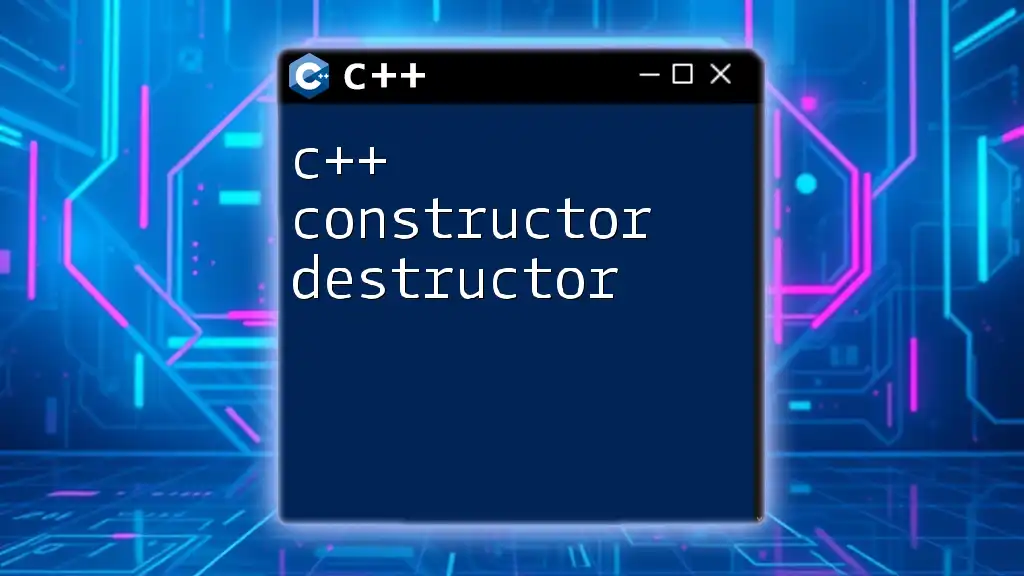
Implementing Custom Copy Semantics
Why Implement Custom Copy Semantics?
In scenarios where structs contain pointers or resources that require specific management, the default copy constructor may not suffice. Implementing custom copy semantics allows you to control how data is copied, ensuring proper memory management and resource allocation.
Writing a Custom Copy Constructor
To implement a custom copy constructor, you need to define a constructor that takes a reference to another instance of the same struct. As an example, consider a struct that manages a dynamic array:
struct DynamicArray {
int* arr;
int size;
// Custom copy constructor
DynamicArray(const DynamicArray& other) {
size = other.size;
arr = new int[size];
std::copy(other.arr, other.arr + size, arr); // Deep copy
}
};
In this `DynamicArray` struct, the custom copy constructor allocates new memory and copies the data from the `other` object's array, providing a deep copy.
Writing a Custom Assignment Operator
Along with the copy constructor, it's essential to implement the copy assignment operator to handle assignment correctly. The key considerations are to check for self-assignment and to avoid memory leaks:
DynamicArray& operator=(const DynamicArray& other) {
if (this != &other) { // Check for self-assignment
delete[] arr; // Free existing resource
size = other.size;
arr = new int[size];
std::copy(other.arr, other.arr + size, arr); // Deep copy
}
return *this;
}
In this code, the existing memory is freed before allocating new memory and copying the elements from `other`. This technique prevents memory leaks and ensures that each instance has its own data.
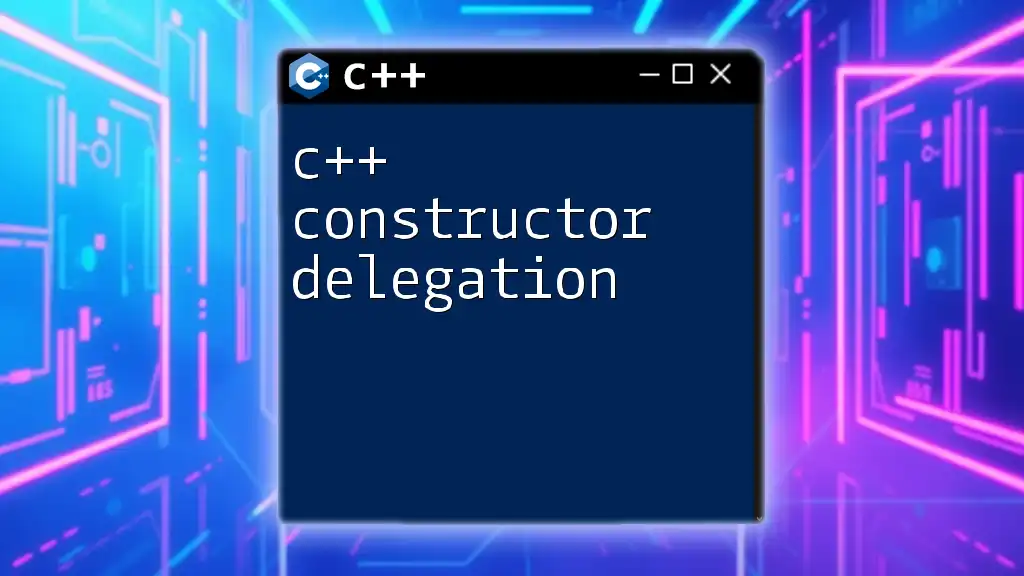
Use Cases and Best Practices
When to Use Copy Semantics
Copy semantics can come into play whenever you need to pass structs by value, return structs from functions, or assign one struct to another. Understanding how your structs behave when copied is crucial in avoiding unexpected behaviors.
Avoiding Common Pitfalls
To ensure smooth operation and memory integrity, follow these best practices:
- Self-Assignment: Always check for self-assignment in your copy assignment operator to prevent unexpected behavior.
- Resource Management: Manage dynamic memory diligently. Use RAII (Resource Acquisition Is Initialization) principles to handle resources effectively.
- STL Containers: When your structs contain STL containers (like `std::vector`), remember that copying these containers automatically handles deep copies of their contents.
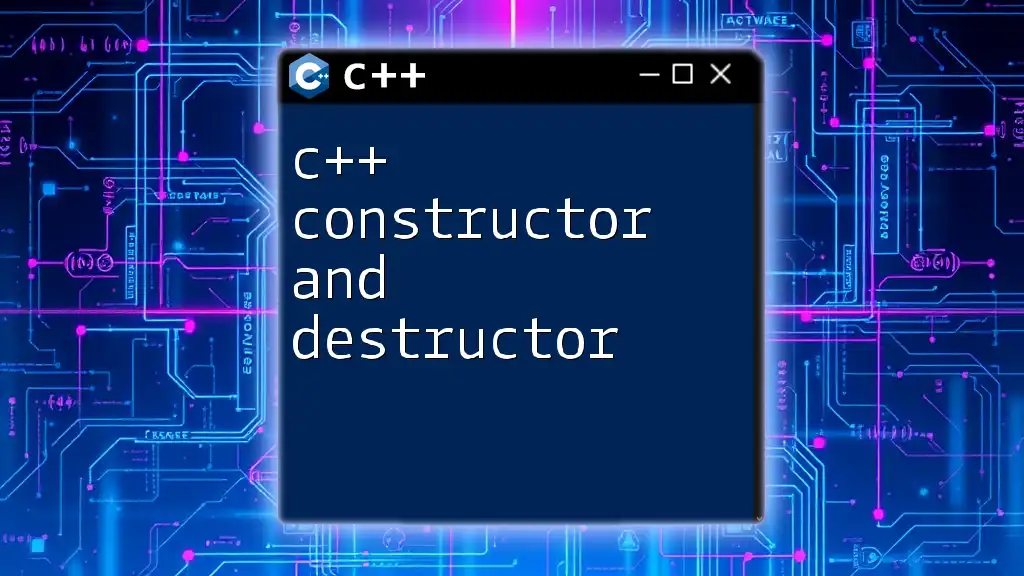
Conclusion
Understanding C++ copy struct semantics enriches your programming skills and aids in writing efficient, error-free code. By mastering the differences between shallow and deep copying, along with customizing your copy constructors and assignment operators, you can ensure that your programs manage resources effectively.
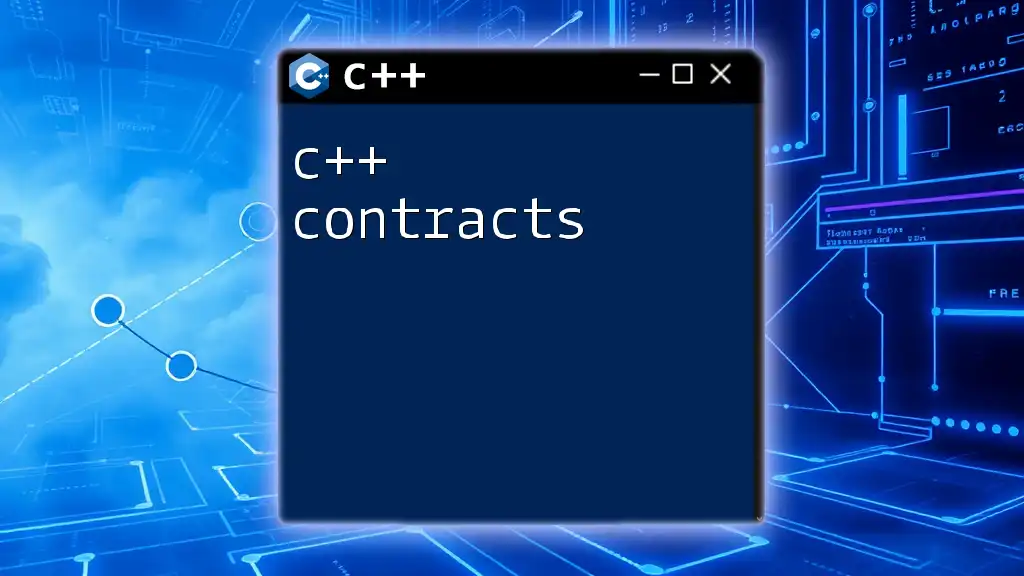
Additional Resources
- Official C++ documentation to explore further concepts.
- Recommended books and online courses for in-depth learning on object-oriented programming and memory management.
FAQs
-
What happens if I don't implement copy semantics?
- If not implemented, the default copy constructor and assignment operator will suffice, but they may not be adequate for complex data types that involve dynamic memory.
-
Can I use structs with STL containers?
- Absolutely! However, you should be cautious about how they are copied. STL containers manage their own memory, so their default copy semantics typically work without additional implementation.
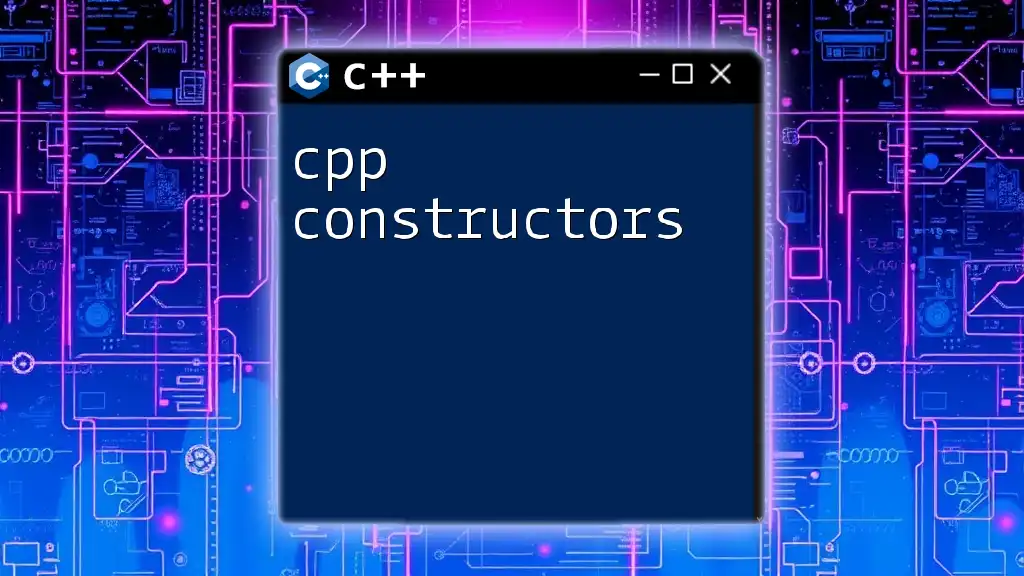
Call to Action
Practice what you've learned by creating your own structs and implementing custom copy constructors and assignment operators. Share your experiences or pose any questions you might have in the comments section! Happy coding!