Constructor delegation in C++ allows one constructor to call another constructor within the same class to avoid code duplication and improve maintainability.
Here's a code snippet demonstrating constructor delegation:
#include <iostream>
class Point {
public:
Point(int x, int y) : x_(x), y_(y) {}
Point(int x) : Point(x, 0) {} // Delegating constructor
void display() {
std::cout << "Point(" << x_ << ", " << y_ << ")\n";
}
private:
int x_, y_;
};
int main() {
Point p1(10, 20); // Calls the first constructor
Point p2(5); // Calls the delegating constructor
p1.display();
p2.display();
return 0;
}
The Basics of C++ Constructors
What is a Constructor?
A constructor is a special member function in C++ used for initializing objects when they are created. It has the same name as the class and does not have a return type. The primary purpose of a constructor is to set initial values for data members of an object.
Types of Constructors
There are several types of constructors in C++, each serving different purposes:
-
Default Constructors: These constructors do not take any parameters. They initialize objects with default values.
-
Parameterized Constructors: Constructors that take arguments to set initial values for data members.
-
Copy Constructors: These constructors create a new object as a copy of an existing object.
-
Move Constructors: Used primarily with C++11 and later, move constructors transfer resources from temporary objects.
How Constructors Work
Constructors allow for an initialization list, a powerful feature that enables the direct initialization of data members before entering the constructor body. This is particularly useful for constant or reference members that cannot be assigned later.
class Example {
public:
Example(int value) : value(value) {} // Initialization list
private:
int value;
};
In this code snippet, the constructor initializes the `value` parameter directly using an initialization list.
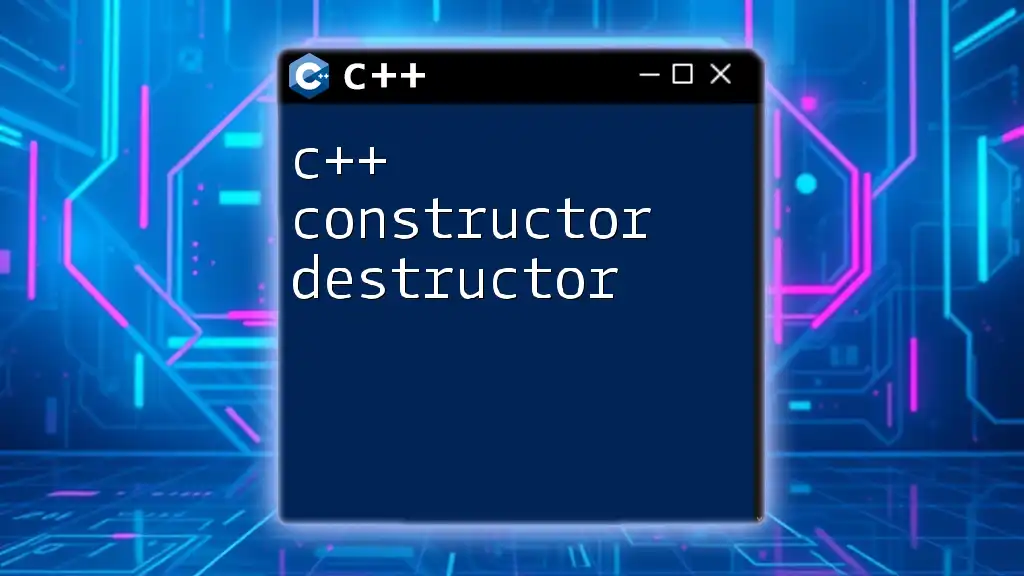
Understanding Delegating Constructors in C++
Defining Delegating Constructors
Delegating constructors are a specific feature in C++ that allow one constructor to call another constructor within the same class. This mechanism facilitates code reuse and can significantly streamline object initialization.
Syntax for Delegating Constructors
The syntax for a delegating constructor is straightforward. It uses the member initializer list to delegate the initialization to another constructor in the same class.
class Example {
public:
Example() : Example(42) {} // Delegates to another constructor
Example(int value) : value(value) {}
private:
int value;
};
In this example, the default constructor `Example()` delegates the initialization to the parameterized constructor `Example(int value)`, thus initializing `value` to 42.
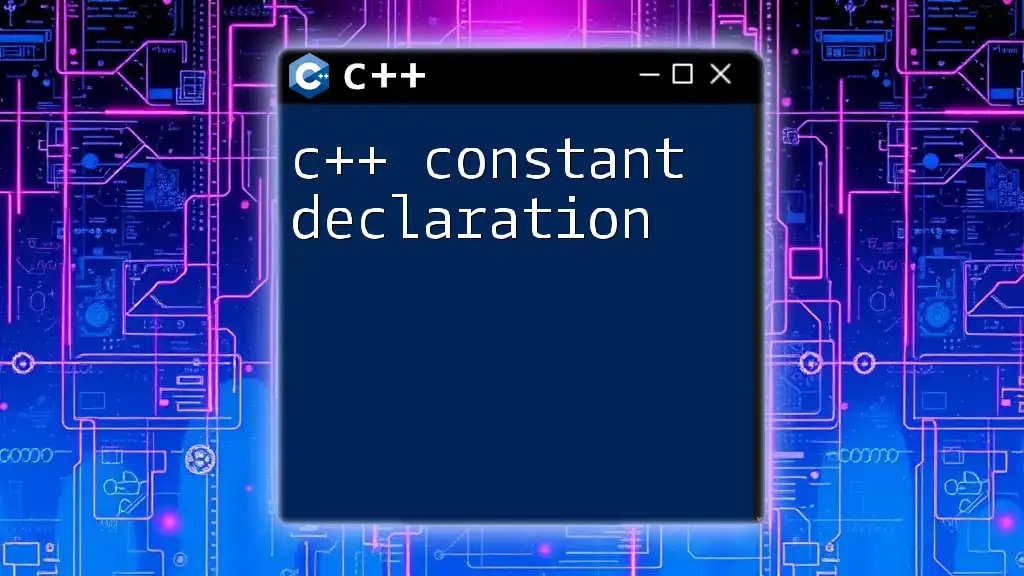
Benefits of Using Delegating Constructors
Code Reusability
One of the primary benefits of c++ constructor delegation is enhanced code reusability. By using delegating constructors, you can avoid repeating initialization logic across different constructors. This not only minimizes redundancy but also makes your code easier to maintain.
Simplifying Complex Initialization
Constructor delegation can help to reduce the complexity of object creation. By breaking down the initialization process into manageable pieces, the overall constructor design becomes simpler and easier to understand. For instance, if your class has multiple constructors that share common initialization logic, delegating can consolidate that logic into one constructor, promoting clarity.
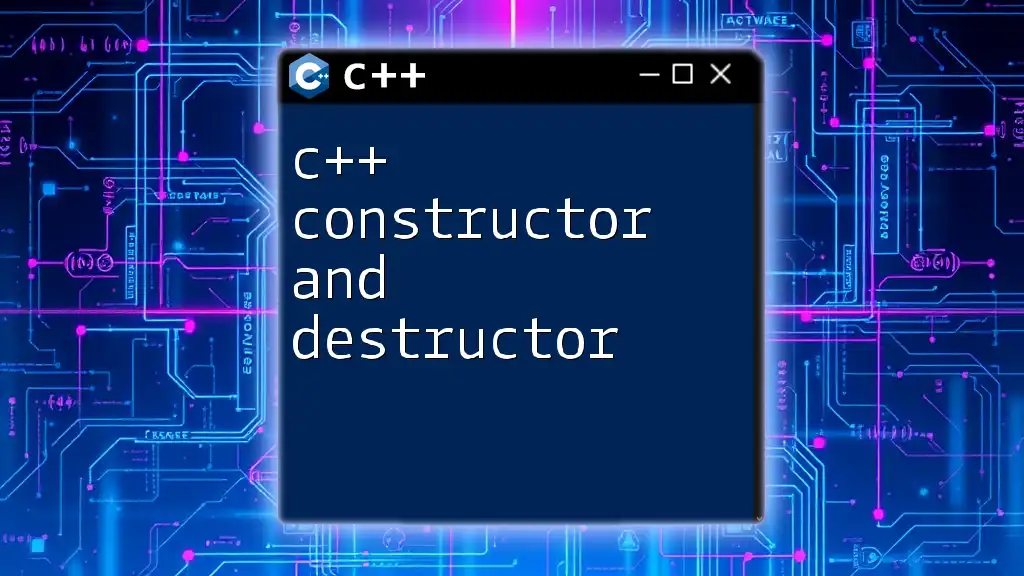
Real-World Use Cases of Delegated Constructors
Example 1: Default Initialization
Consider the following example of a `Point` class:
class Point {
public:
Point() : Point(0, 0) {} // Default to origin
Point(int x, int y) : x(x), y(y) {}
private:
int x, y;
};
In this case, the default constructor initializes `Point` to the origin (0,0) by delegating to the parameterized constructor. This approach minimizes code duplication while clearly expressing intent.
Example 2: Multiple Ways to Construct an Object
Another example is a `Rectangle` class:
class Rectangle {
public:
Rectangle() : Rectangle(1, 1) {} // Default is a 1x1 rectangle
Rectangle(int width, int height) : width(width), height(height) {}
private:
int width, height;
};
Here, the default constructor sets the rectangle’s dimensions to 1x1 by delegating to the parameterized constructor, which keeps the initialization logic neat and easy to follow.
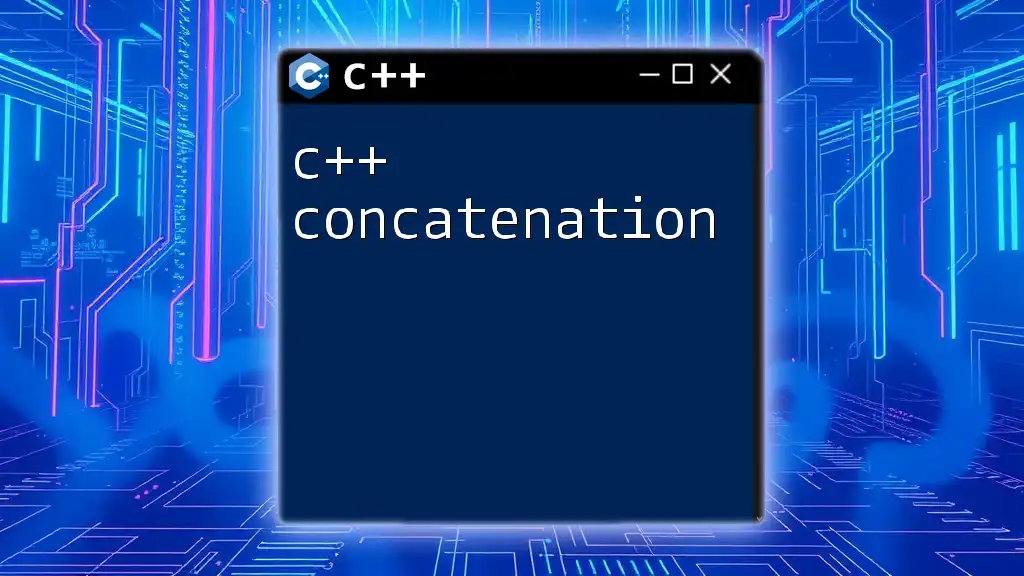
Best Practices for Using Delegating Constructors
Clarity and Readability
When using delegating constructors, it’s imperative to maintain clarity and readability. Choose clear and descriptive names for your constructors to indicate their functionality. This practice not only helps in understanding your code better but also aids in maintaining it over time.
Avoiding Circular Delegation
While delegating constructors can simplify initialization, it’s crucial to avoid circular delegation where one constructor delegates to another, which eventually calls back to the first constructor. This could lead to infinite recursion and runtime errors. Always analyze your constructor calls to ensure that there’s a clear path of initialization.
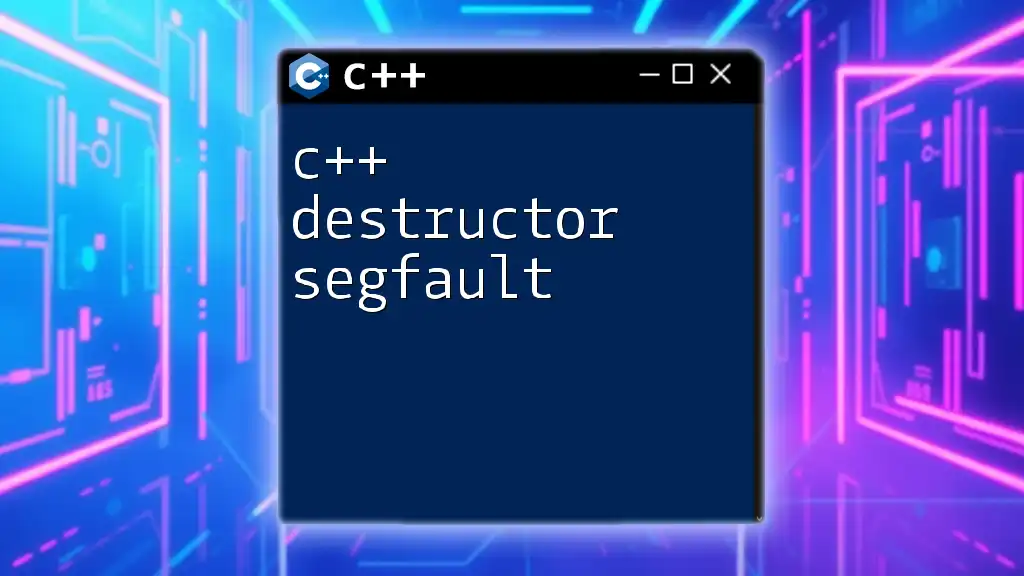
Conclusion
C++ constructor delegation offers a robust mechanism for simplifying object initialization, enhancing code reusability, and improving maintainability. By allowing one constructor to call another within the same class, you can significantly streamline your code structure and reduce redundancy. As you engage with c++ constructor delegation, keep in mind best practices to maximize clarity and effectiveness in your implementations. Experiment with delegating constructors in your own projects to fully grasp their benefits and applications.
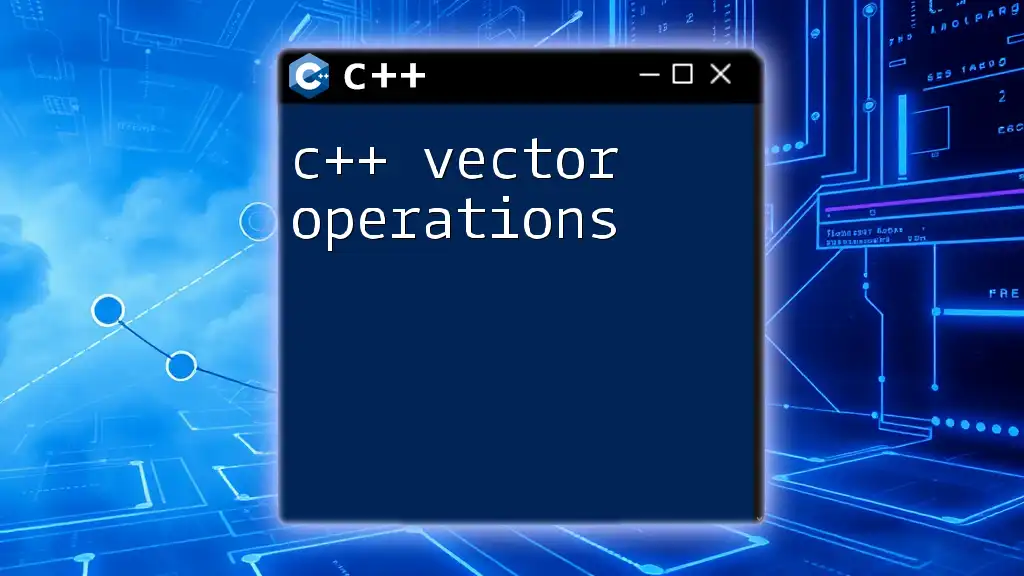
Further Reading
To further deepen your understanding of c++ constructor delegation and related topics, consider exploring the following resources:
- The official C++ documentation
- Online tutorials focused on object-oriented programming in C++
- Literature on advanced C++ concepts and best practices