In C++, a constant reference is a reference that cannot be used to modify the value it refers to, ensuring that the referred object remains unchanged when passed to functions.
#include <iostream>
void printValue(const int& value) {
std::cout << "Value: " << value << std::endl;
}
int main() {
int num = 10;
printValue(num); // num cannot be modified inside the function
return 0;
}
Understanding References in C++
In C++, a reference is an alias for another variable. Unlike pointers, references do not consume additional memory, allowing developers to work with variables without focusing on the underlying memory address. This feature simplifies code while improving readability.
When you pass parameters to functions, you can choose between passing by value or by reference. Passing by reference allows the function to operate directly on the variable it refers to, which is particularly useful for large data structures as it avoids the overhead of copying the object.
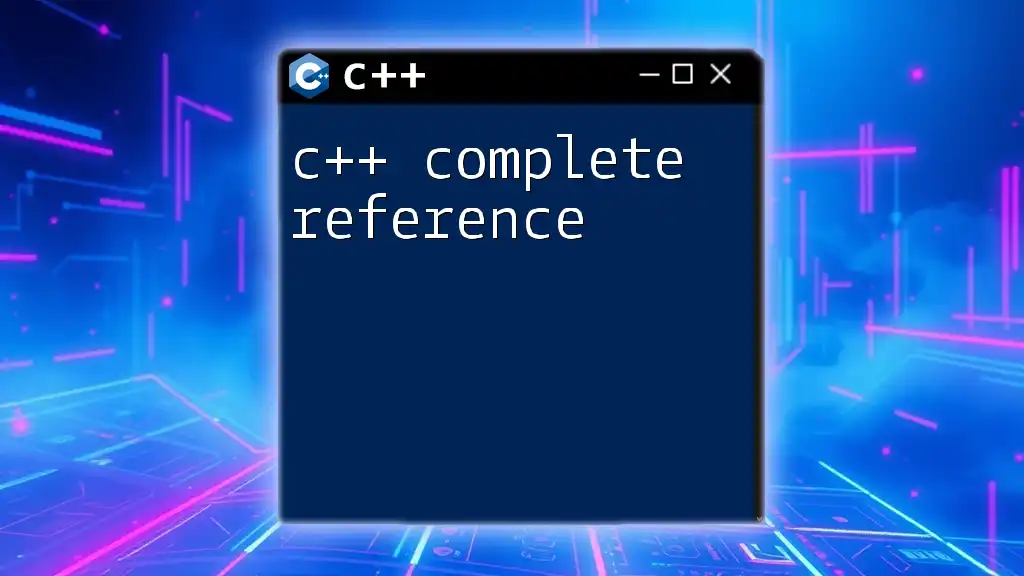
What is a Constant Reference?
A constant reference in C++ is a type of reference that cannot modify the value it refers to. The syntax for declaring a constant reference is straightforward:
const Type& varName;
This declaration signifies that `varName` will be a reference to `Type`, but any attempt to modify the object it references will result in a compilation error.
Important Characteristics of Constant References
-
Immutable Nature: Constant references provide a way to access variables without the ability to modify them, hence they are particularly useful for function parameters or returned values when you don't want them altered.
-
Prevention of Unintended Side Effects: Using constant references helps enforce immutability, making your code less error-prone.
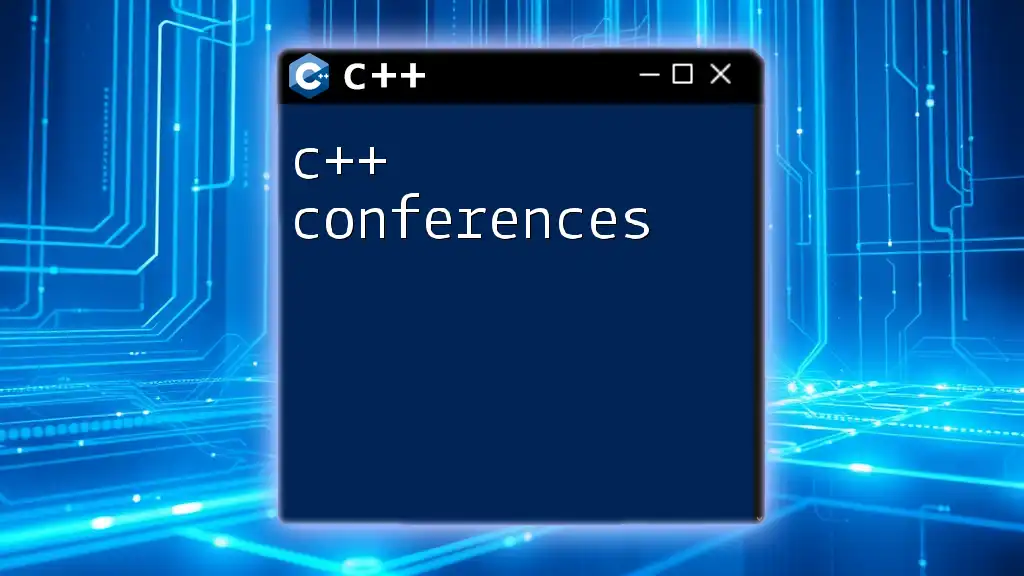
Why Use Constant References?
Utilizing constant references brings various benefits that enhance performance and code clarity.
Performance Benefits
When passing objects, especially large ones, by constant reference instead of by value, you avoid extra copying, which can be resource-intensive. Here are a couple of points to consider:
-
Efficiency: With constant references, the function receives a pointer to the original data instead of a copy, leading to faster execution times.
-
Memory Overhead: Using constant references lowers memory usage since no additional copies are created.
Improved Code Readability and Maintainability
Constant references make your intent explicit in the code. They signal to anyone reading the code that the function will not modify the passed variable, which enhances comprehension and reduces potential bugs.
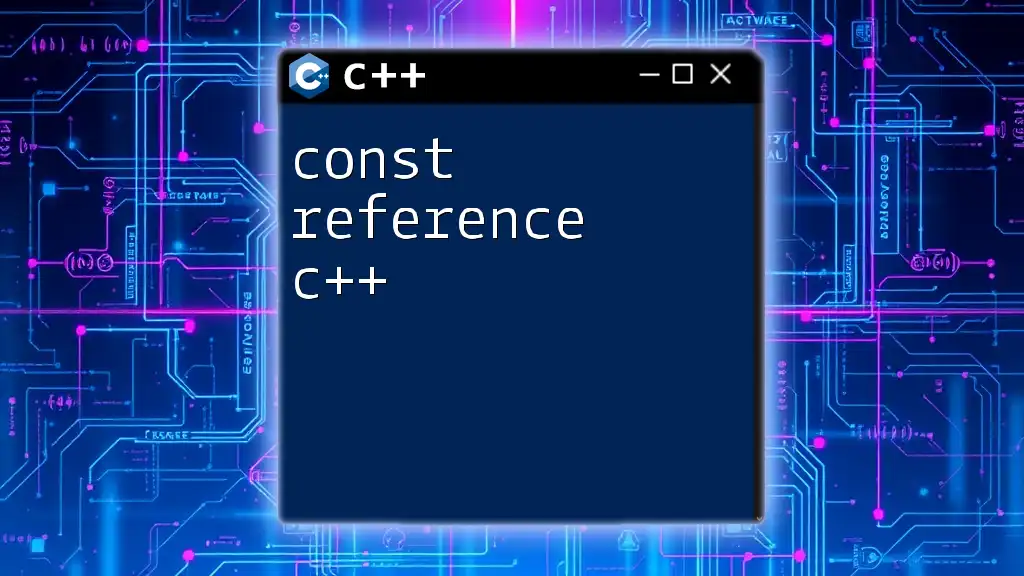
How to Declare and Use Constant References
Declaring Constant References
The declaration of a constant reference follows a simple pattern:
const int& myRef = myVariable;
In this case, `myRef` acts as a constant reference to `myVariable`, meaning any modifications to `myRef` will result in an error.
Using Constant References in Functions
Constant references are often used as function parameters. Here’s a typical syntax illustrating their use:
void displayValue(const int& value) {
std::cout << "Value: " << value << std::endl;
}
In the above example, `displayValue` takes a constant reference to an integer. This method effectively prevents modifications to `value` inside the function, ensuring that the original value remains intact.
Returning by Constant Reference
Returning a constant reference can be useful when you want to provide access to the original object without allowing modifications. For instance:
const std::string& getName(const std::string& name) {
return name; // Return constant reference to name
}
In this example, `getName` returns a constant reference to the string passed in. This method can be advantageous, especially in classes where you might want to expose internal data safely.
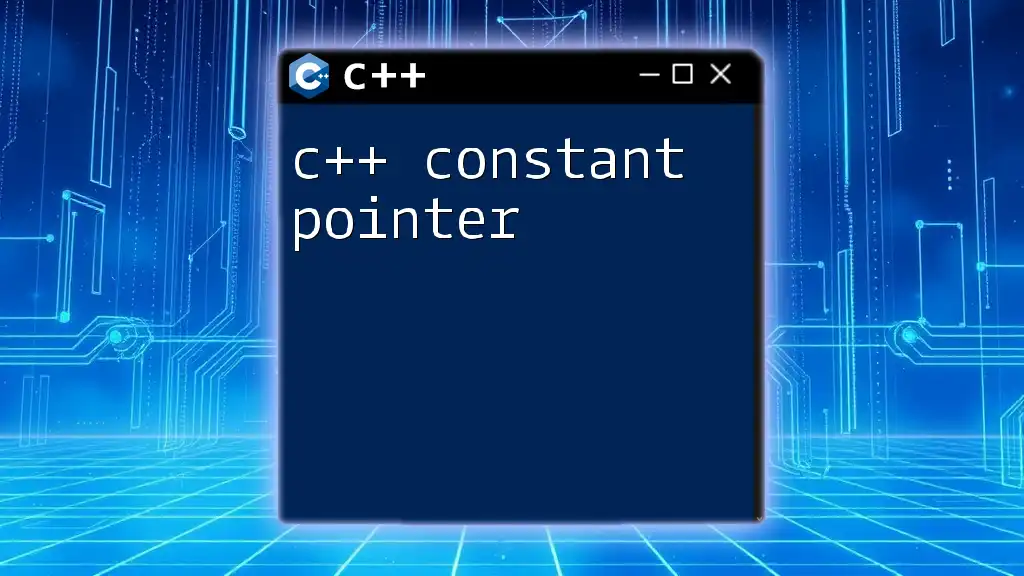
Common Use Cases for Constant References
In Classes and Data Structures
Constant references are often leveraged in class methods to maintain encapsulation while also providing efficient access. For example:
class MyClass {
public:
void print(const std::string& str) const {
std::cout << str << std::endl;
}
};
In `MyClass`, `print` uses a constant reference to accept and display a string without altering it.
Working with Large Objects
When dealing with complex data structures like vectors or maps, using constant references can significantly cut down the performance costs associated with copying. For instance:
void processData(const std::vector<int>& data) {
// Process data without copying
}
This function processes a vector of integers without having to create a duplicate, thus conserving both time and memory.
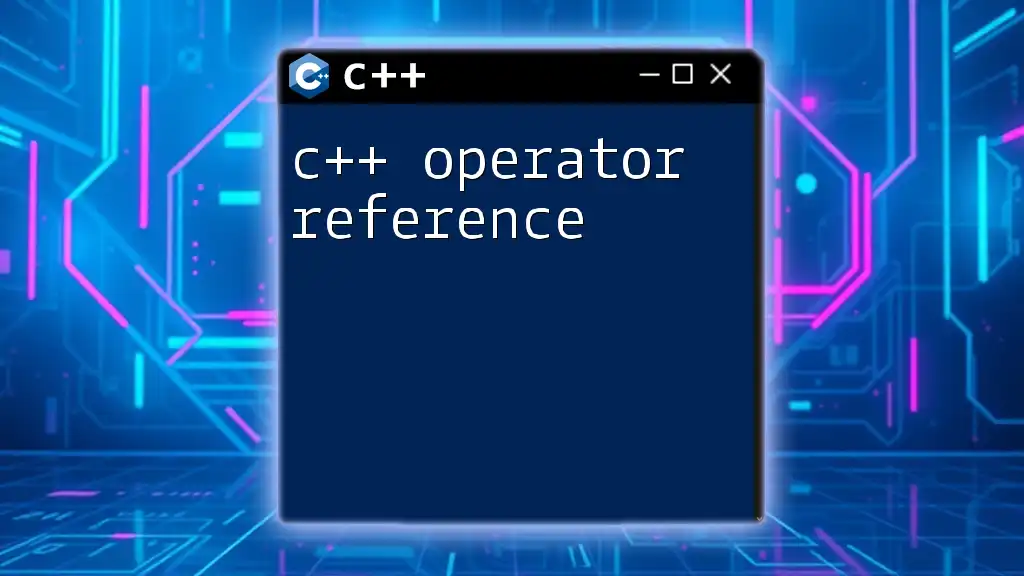
Best Practices When Using Constant References
Effective use of constant references involves knowing when to utilize them appropriately. Here are some key points:
-
When to Use: Always consider constant references for function parameters, especially when dealing with large objects. They provide a smart balance of efficiency and clarity.
-
Avoiding Dangling References: Be mindful when returning constant references to local variables, as this can lead to dangling references. Instead, ensure that the referenced object outlives the reference itself.
Tips for Performance Optimization
Keep in mind that while constant references are efficient, they are not a silver bullet. Always profile your application to ensure that you're using them in performance-critical sections appropriately.
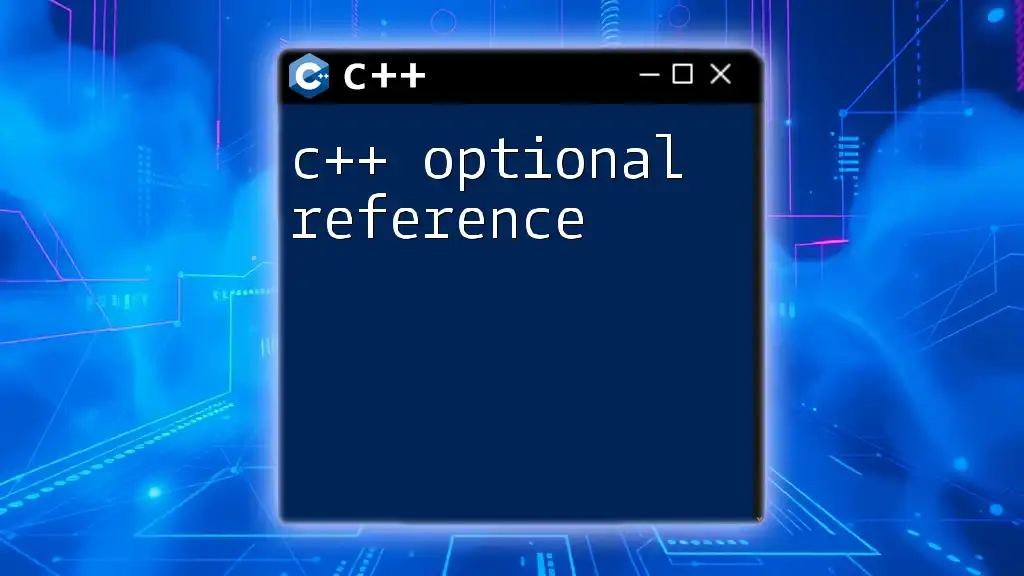
Pitfalls to Avoid with Constant References
There are a few traps that can lead to common mistakes when handling constant references:
-
Dangling References: Be cautious of returning a constant reference to a local variable that goes out of scope. This will lead to undefined behavior, as the reference will point to an invalid location in memory.
-
Misconceptions about Mutability: Many new developers assume that constant references are entirely immutable. It's crucial to understand that you cannot change the reference itself or what it refers to, but you can still mutate the object referred to if it is not itself `const`.
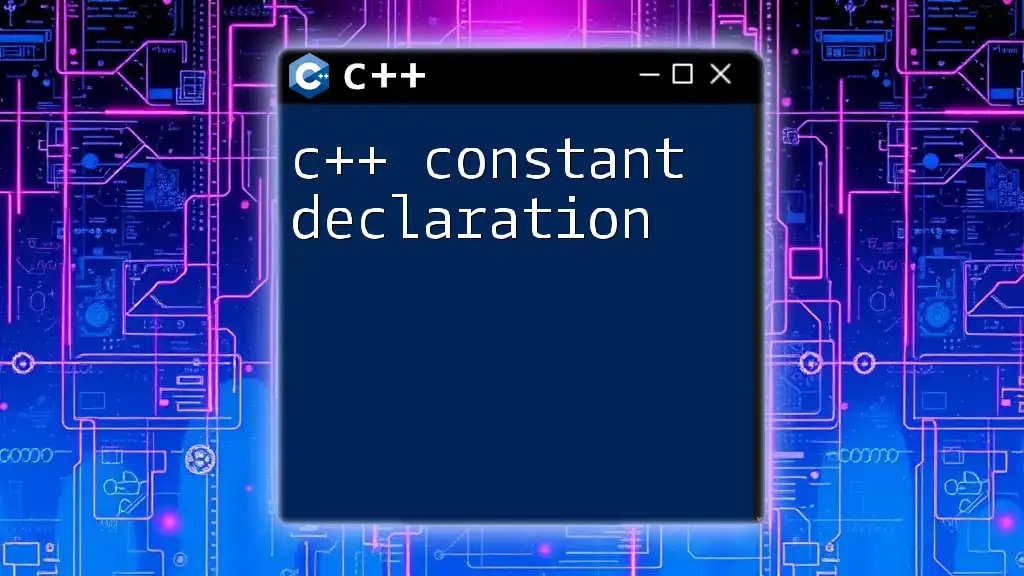
Conclusion
The C++ constant reference is a powerful tool that enhances performance, ensures code clarity, and prevents errors associated with unintended modifications. As you develop your C++ skills, utilizing constant references will help you write cleaner, safer, and more efficient code. Practice integrating constant references into your projects, and you'll see the difference in both readability and performance.
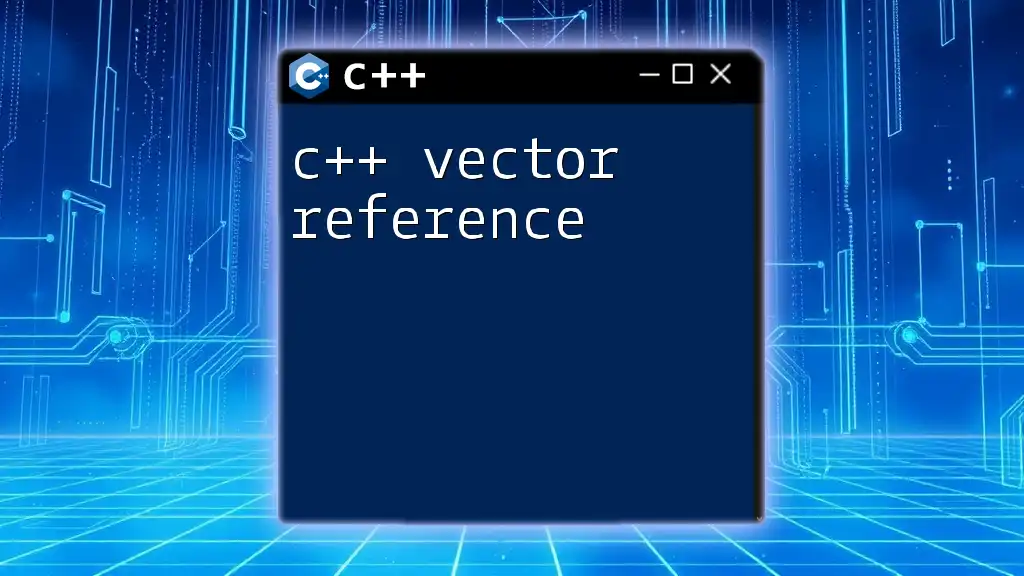
Further Resources
For those looking to deepen their understanding, consider exploring C++ documentation and advanced tutorials surrounding object management and references.
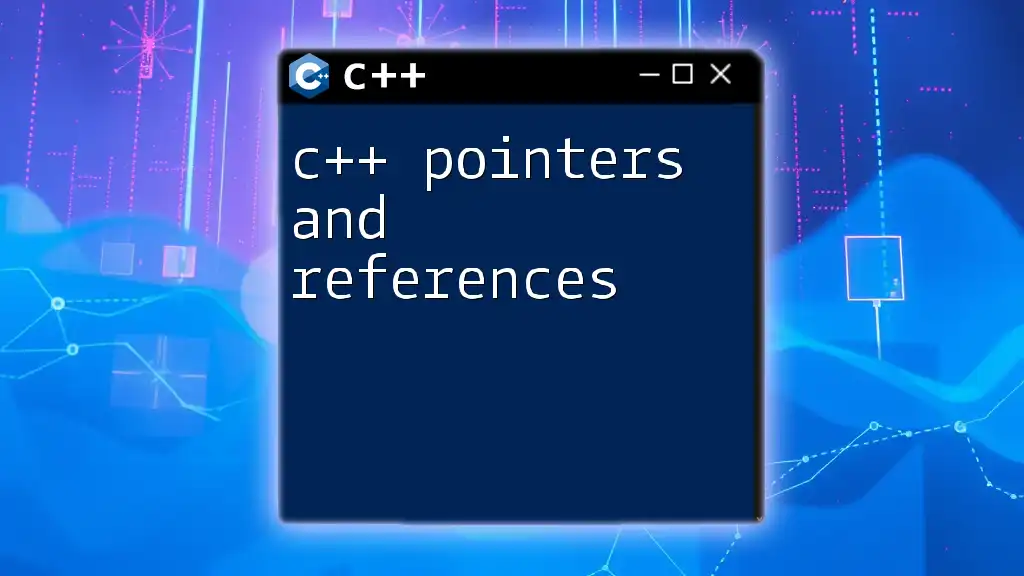
FAQs
-
What happens if you try to modify a constant reference? Attempting to modify a constant reference will result in a compilation error, enforcing immutability in your code.
-
Can you have a constant reference to a mutable object? Yes, you can have a constant reference to a mutable object, meaning that while you cannot change the reference itself, the underlying object can still be modified unless it's declared as `const`.