In C++, a constant declaration allows you to create immutable variables whose values cannot be changed after initialization, using the `const` keyword.
Here's a code snippet demonstrating a constant declaration in C++:
const int MAX_SIZE = 100;
Understanding C++ Constants
What is a Constant in C++?
A constant in C++ is a fixed value that cannot be altered during the execution of a program. Constants play a crucial role in programming as they provide a way to define values that remain unchanged, which enhances code clarity and reduces errors. Unlike variables, which can vary in value, constants maintain their assigned value throughout the program’s lifecycle, making them essential for maintaining integrity in calculations and control flow.
Types of Constants in C++
C++ offers various types of constants:
-
Literal Constants: These are direct values written in the code, such as numbers and characters. For instance, `42`, `3.14`, and `'a'` are literal constants.
-
Defined Constants: These are created using specific keywords, allowing for more flexibility and maintainability in code. They are often defined using `const` or `constexpr`.
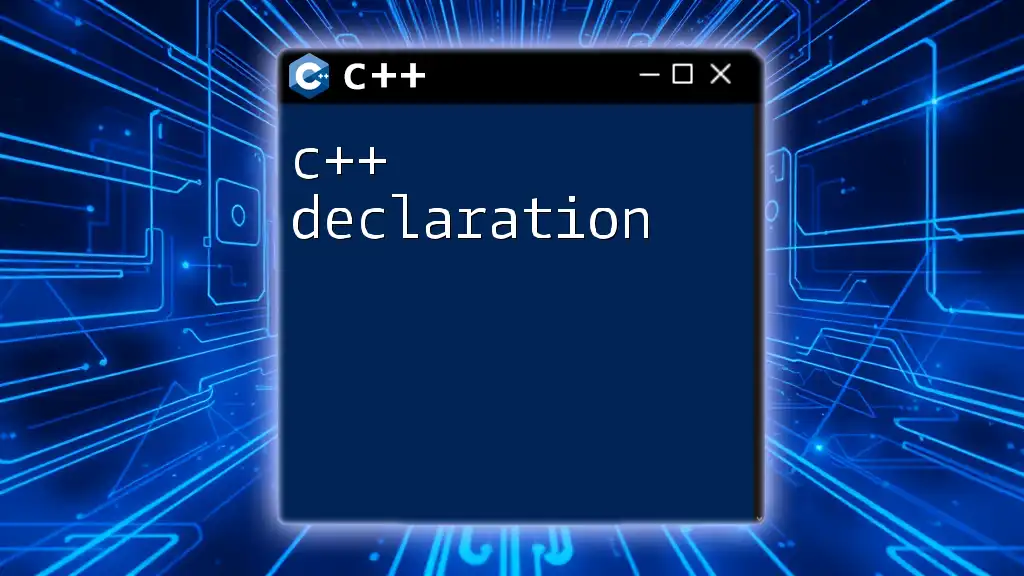
Declaring Constants in C++
How to Declare a Constant in C++
C++ provides two primary ways to declare constants: using the `const` and `constexpr` keywords.
Using the `const` Keyword
To declare a constant variable, you use the `const` keyword followed by the data type and the variable name. Here’s the syntax:
const data_type CONSTANT_NAME = value;
Example of Declaring a Constant Variable
For example, if you want to declare a constant variable for the maximum number of users allowed:
const int MAX_USERS = 100;
In this snippet, we defined `MAX_USERS` as a constant integer set to `100`. This means that throughout your program, the value of `MAX_USERS` will remain `100`, and any attempt to change it will lead to a compilation error.
Using the `constexpr` Keyword
The `constexpr` keyword allows you to define a constant that the compiler can evaluate at compile time. This can be beneficial for performance optimization, especially in larger programs.
Example of Using `constexpr` to Declare a Constant
For instance, if you need to declare the value of Pi:
constexpr double PI = 3.14159;
This line declares `PI` as a constant double with a value of `3.14159`, which can be computed during compilation, thereby improving performance.
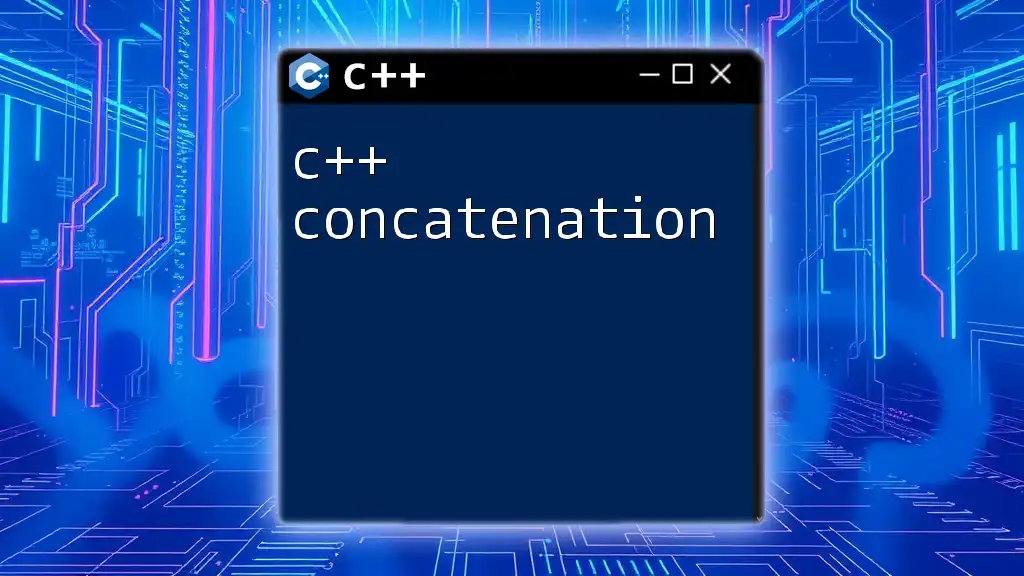
Best Practices for Declaring Constants
Naming Conventions for Constant Variables
When declaring constants, using descriptive names is crucial for code readability. Most programmers follow a standard naming convention for constants, often using all uppercase letters with underscores to separate words. For example, `MAX_BUFFER_SIZE` clearly indicates what the constant represents.
Scope of Constants in C++
Understanding the scope of constants is critical:
- Local Constants: Declared within a function and only accessible in that function.
- Global Constants: Accessible throughout the entire program, declared outside of any function.
Being mindful of the scope helps prevent naming conflicts and improves code organization.
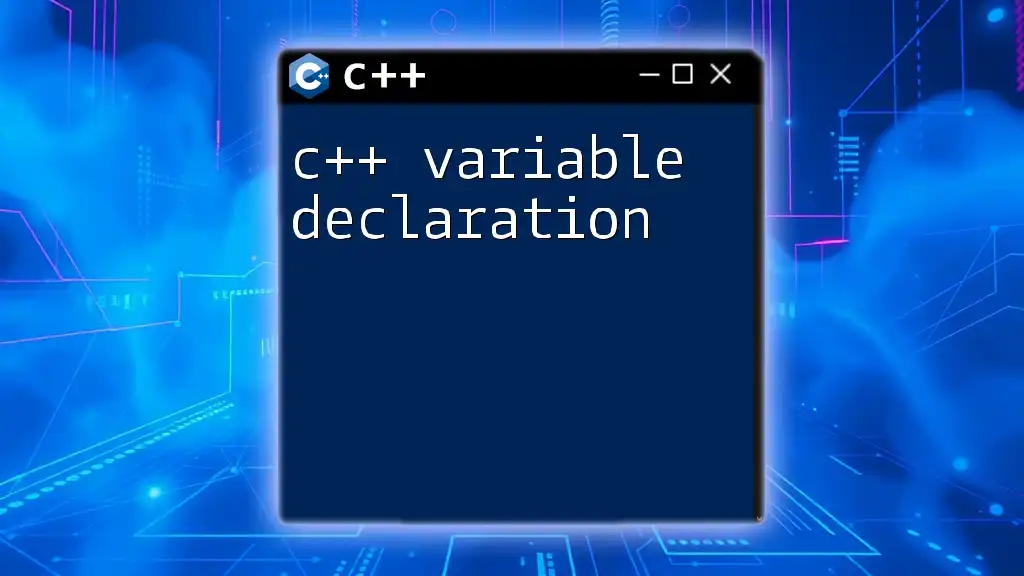
Common Mistakes When Declaring Constants
Forgetting to Use const or constexpr
One common mistake among beginners is neglecting to declare a variable as `const` or `constexpr`. Failing to do so can lead to unintended modifications where values are altered, leading to bugs.
Modifying a Constant Variable
Another frequent mistake is trying to modify a declared constant. For instance:
const int DAYS_IN_WEEK = 7;
// Error: DAYS_IN_WEEK = 8; // This will cause a compilation error
Attempting to assign a new value to `DAYS_IN_WEEK` will trigger a compile-time error, highlighting the necessity of constants in preventing accidental changes.
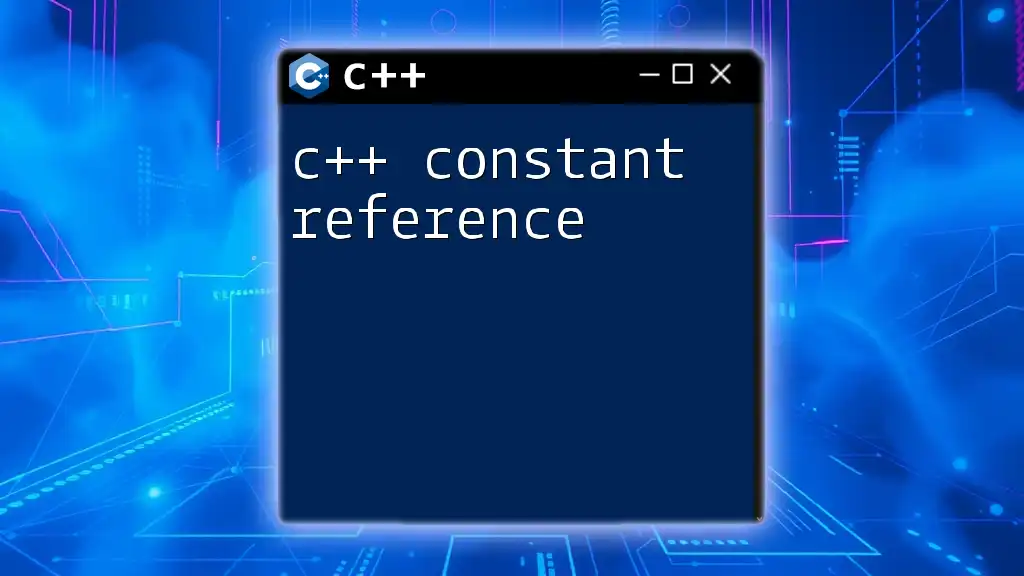
Benefits of Using Constants
Enhancing Code Readability and Maintenance
Using constants greatly contributes to improved code readability. When you encounter a constant like `MAX_CONNECTIONS`, it indicates its purpose without needing to check its definition. This self-documenting nature simplifies maintenance, making it easier for other developers (or yourself in the future) to understand the codebase quickly.
Improving Performance
Constants declared with `constexpr` are evaluated at compile time, enhancing performance, especially in situations where the constant value is repeatedly used in calculations. By letting the compiler handle constant values early in the compilation process, you reduce runtime overhead. For example, using `constexpr` in loops allows the compiler to optimize those loops significantly.
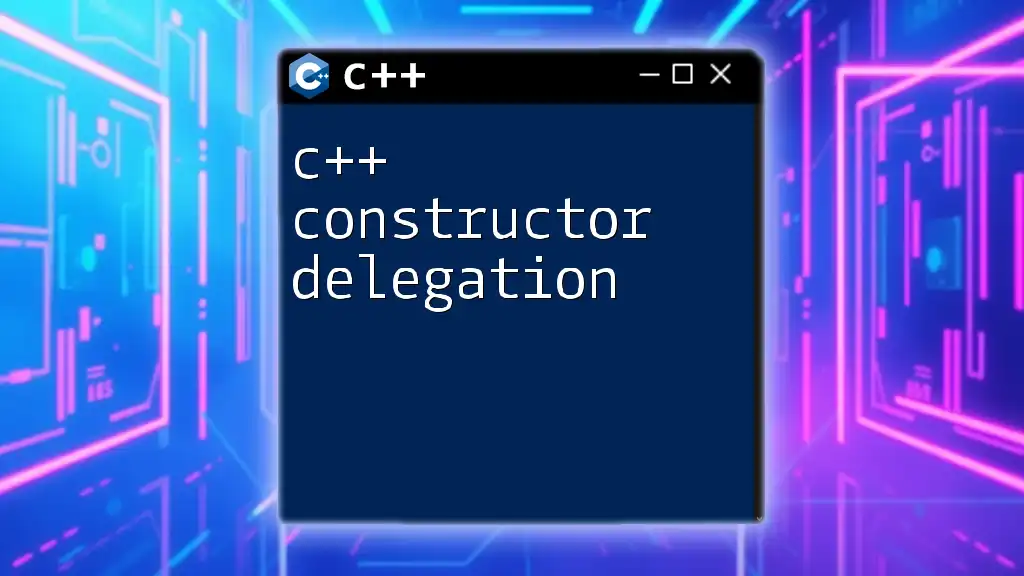
Conclusion
Summary of Key Points
In summary, understanding C++ constant declarations through the `const` and `constexpr` keywords is fundamental for writing robust and efficient code. Proper naming conventions and awareness of scope will elevate the quality and maintainability of your code.
Final Thoughts
By effectively utilizing constants, you not only enhance your programming skills but also foster best practices in C++. Embrace the power of constants in your coding journey, and you'll find your programs becoming cleaner, more efficient, and easier to maintain. Don’t hesitate to explore more about C++ and improve your command over its intricacies!