In C++, a constant is a variable whose value cannot be changed once it is initialized, typically defined using the `const` keyword.
Here’s a simple example:
const int MAX_SIZE = 100; // MAX_SIZE cannot be modified after initialization
Understanding Constants in C++
A C++ constant is a variable whose value cannot be changed once it has been initialized. Using constants promotes clarity and intent in code, helping to prevent accidental modifications and making the code easier to maintain. By utilizing constants, developers can enhance the readability and reliability of their programs.
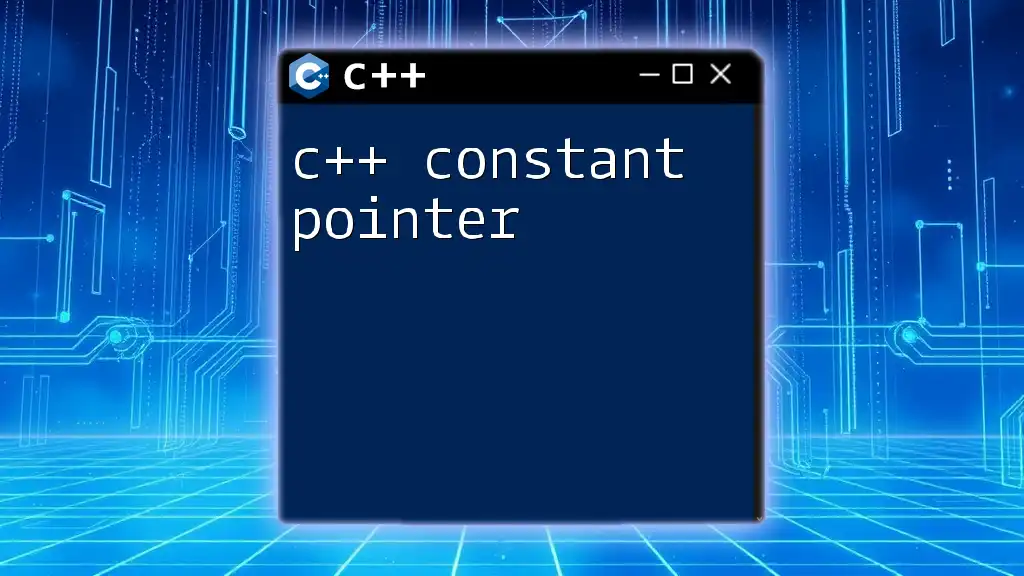
Types of C++ Constants
Built-in Constant Types
Integer Constants
Integer constants are used to represent whole numbers. By declaring an integer constant, you can ensure that its value remains fixed throughout its scope, making your intentions clear.
const int maxScore = 100;
In the above example, `maxScore` is defined as a constant integer set to 100. This value cannot be altered later in the code.
Floating-Point Constants
Floating-point constants represent decimal numbers. Similar to integer constants, they are useful when you need precise values that should not change.
const double pi = 3.14159;
Here, `pi` is declared as a constant double value representing the mathematical constant π.
Character Constants
Character constants are defined for individual symbols. They provide a way to represent single characters in a consistent manner, enhancing code clarity.
const char grade = 'A';
In this instance, `grade` is a character constant storing the letter 'A'.
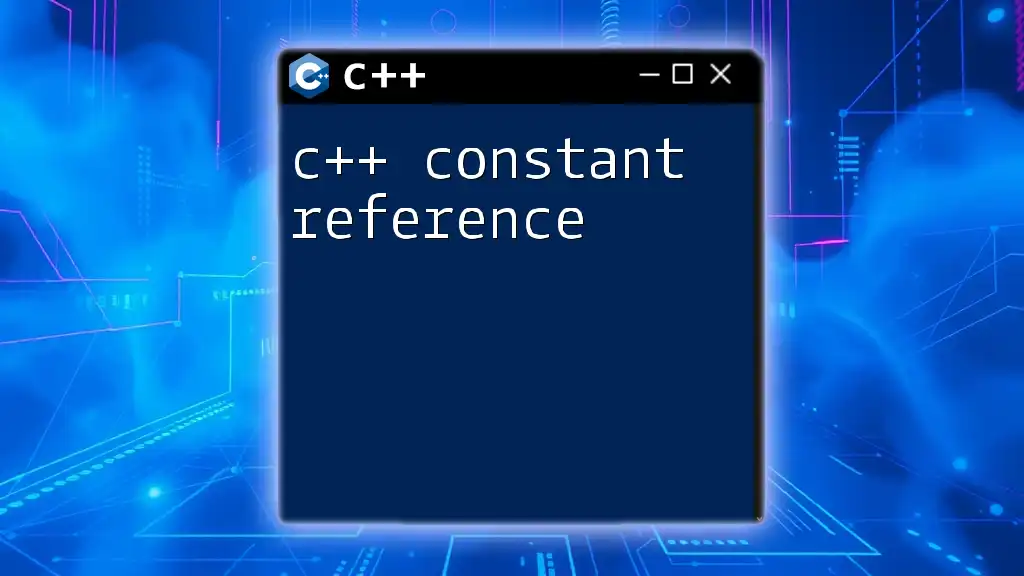
Declaring and Initializing Constants in C++
Declaring a Constant in C++
To declare a constant, simply use the `const` keyword followed by the data type, the identifier name, and the initial value. This provides a clear indication to anyone reading your code that the value is fixed.
Best Practices for Naming Constants
When naming constants, it's a common convention to use uppercase letters separated by underscores. This practice enhances readability, immediately signaling to other developers that the variable should not change.
Examples of Declaring a Constant
Here’s a fundamental example of constant declaration:
const int daysInWeek = 7;
In this case, `daysInWeek` consistently represents the number of days in a week.
You can also declare constants using references for situations where you want to bind a constant to another value:
const double& circumradius = pi * rad;
This ensures that any changes to `rad` reflect in `circumradius`, while the value of `pi` remains intact.
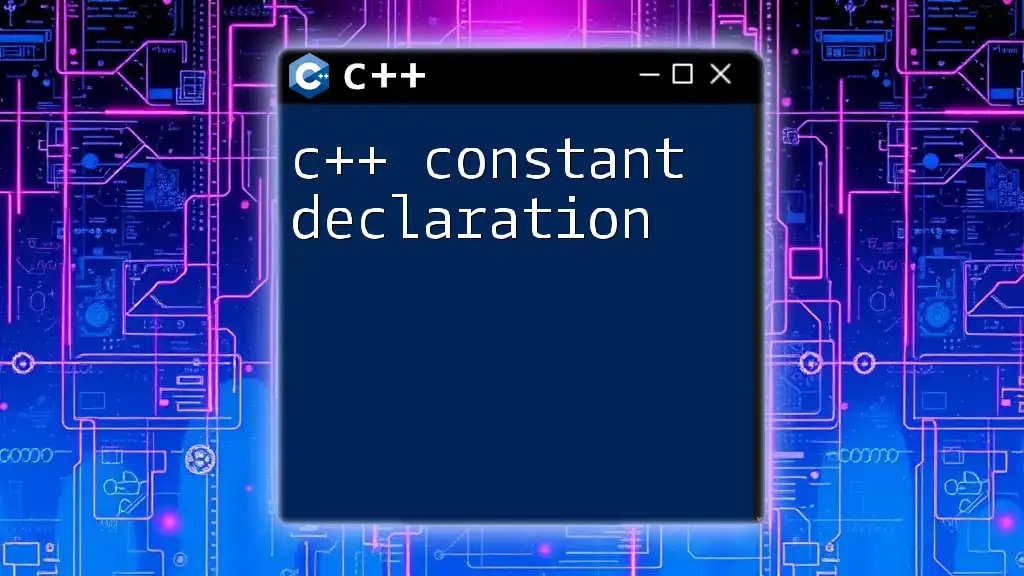
Using Constants in C++
Where to Use Constants
Using constants instead of "magic numbers" or string literals improves your code's maintainability. For example, if you need to change a constant value, you only need to do so in one place.
Example: Using Constants in Functions
Constants can play a pivotal role in functions, particularly as parameters. By using constants in function declarations, you enhance both the clarity and safety of your code.
double calculateArea(const double radius) {
return pi * radius * radius;
}
In this example, the function `calculateArea` receives `radius` as a constant parameter, indicating that it should remain unchanged within the function's execution.
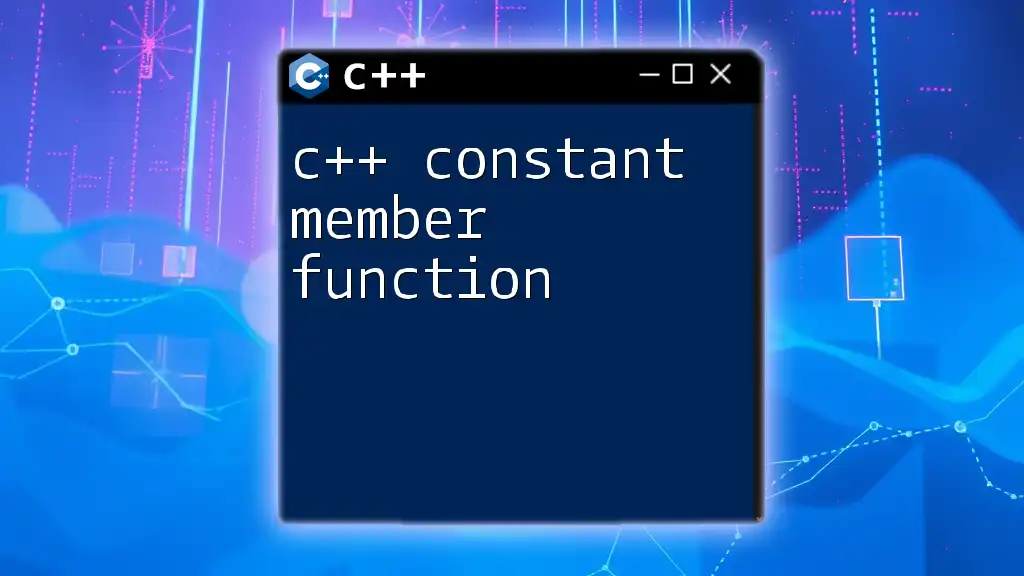
C++11 and Beyond: Improved Constant Functionality
The `constexpr` Keyword
With C++11, the introduction of the `constexpr` keyword allows for more advanced constant declarations and computations at compile time. This can significantly enhance performance in certain scenarios.
constexpr int factorial(int n) {
return n <= 1 ? 1 : n * factorial(n - 1);
}
The `factorial` function here computes the factorial of a number at compile time if possible, resulting in more efficient code execution.
Implicitly Typed Constants with `auto`
Using the `auto` keyword in conjunction with `const` simplifies the declaration process and enhances type inference.
auto const pi = 3.14159;
By employing this style, the type of `pi` is automatically deduced, while ensuring that it remains a constant.
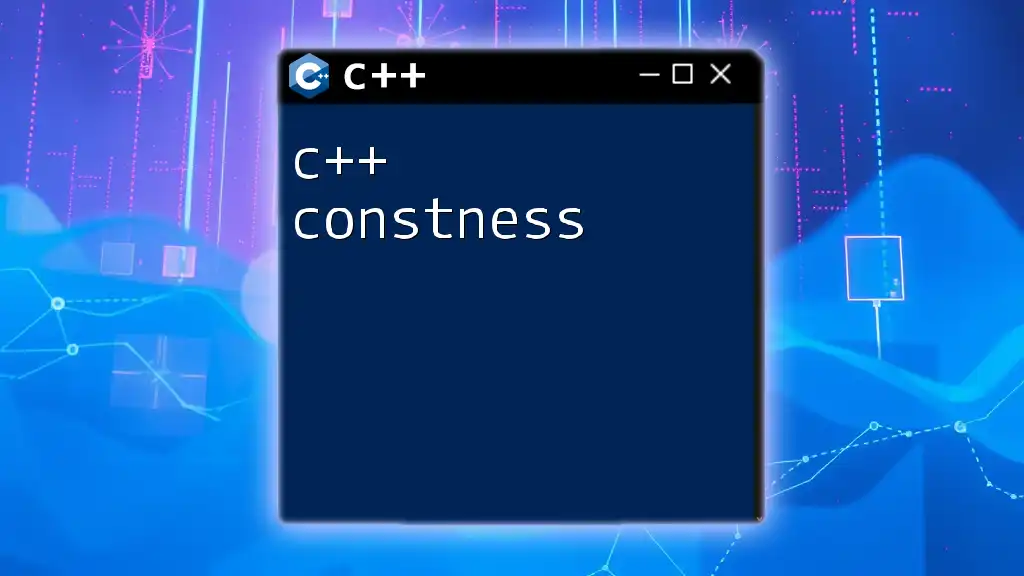
Common Mistakes to Avoid with Constants
Mutability Issues
One common pitfall is attempting to change the value of a constant. This results in a compilation error and a clear indication of poor code practice. For example:
const int maxItems = 10;
maxItems = 20; // Error: cannot assign to variable
Trying to assign a new value to `maxItems` will lead to a compilation error, emphasizing the immutable nature of constants.
Scope of Constants
Another area to be cautious of is the scope of constants. It's vital to understand how constants behave within different scopes, as conflicts may arise if two constants share the same name in disparate scopes.
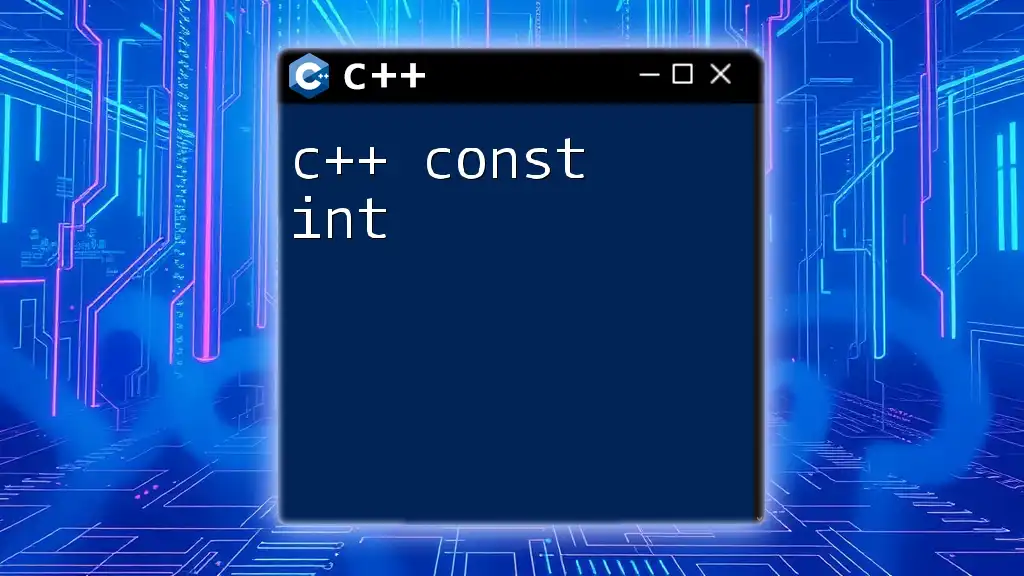
Best Practices for Using C++ Constants
Naming Conventions
To enhance the readability of your code, stick to conventions such as using uppercase letters for constants and keeping names descriptive. For example, prefer `const int MAX_CONNECTIONS = 100;` over a cryptic name.
When to Use Constants vs. Variables
Deciding when to use constants versus variables is essential. Use constants when a value is fixed and should not change during program execution, and variables should be used when values will be modified. This distinction ensures clarity in your code and helps with debugging and maintenance.
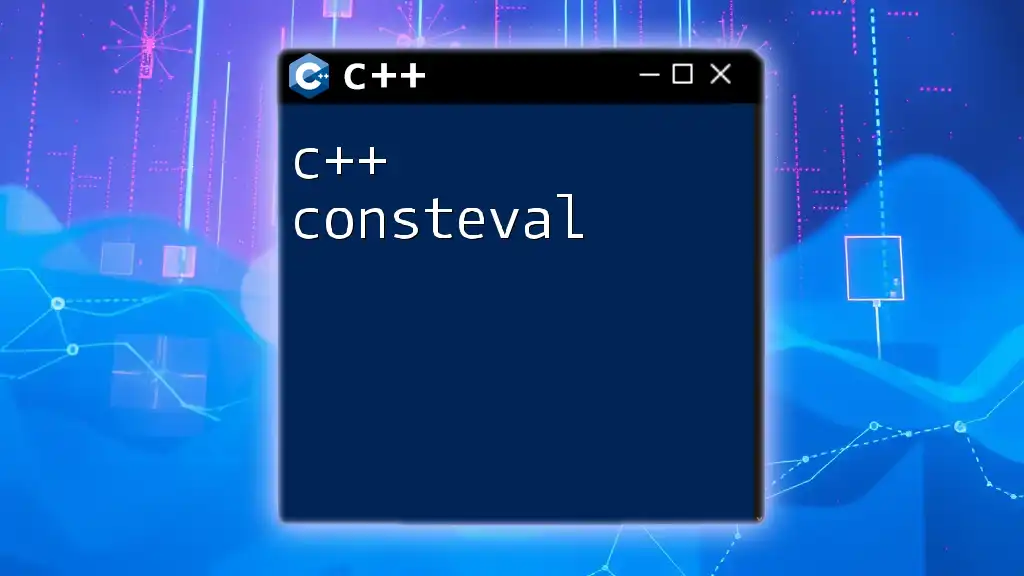
Conclusion
Constants in C++ are powerful tools that improve the reliability and maintainability of your code. By ensuring that certain values remain unchanged throughout your program, you can write cleaner, more understandable, and less error-prone C++. Incorporating constants effectively into your programming practices will lead to better coding habits and a deeper understanding of C++.
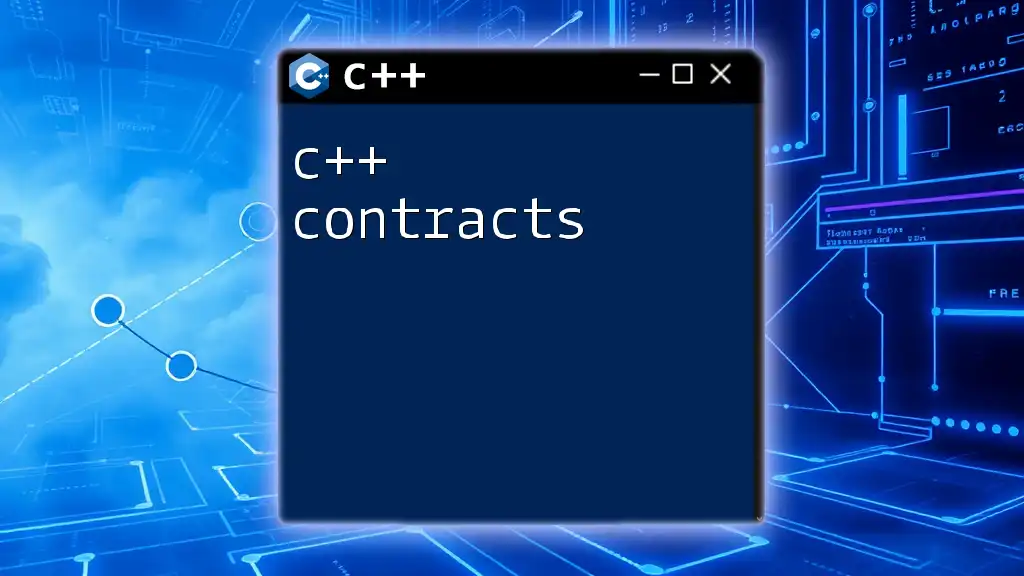
Additional Resources
To further deepen your comprehension of C++ constants, consider exploring additional resources such as textbooks, online courses, and official documentation that provide in-depth discussions and practical exercises catered to both novices and experienced developers in C++.