The `instanceof` operator in C++ is used to check if an object is an instance of a particular class or derived class, although it's worth noting that C++ uses the `typeid` operator and dynamic casting for this purpose, as C++ does not have a direct `instanceof` operator like some other languages.
Here's a code snippet demonstrating this:
#include <iostream>
#include <typeinfo>
class Base {};
class Derived : public Base {};
int main() {
Base* b = new Derived;
if (typeid(*b) == typeid(Derived)) {
std::cout << "b is an instance of Derived" << std::endl;
} else {
std::cout << "b is NOT an instance of Derived" << std::endl;
}
delete b;
return 0;
}
What is "instanceof" in C++?
The terminology "instanceof" is commonly associated with languages like Java. However, in C++, the concept translates into type-checking mechanisms that allow developers to determine the dynamic type of an object during runtime. Understanding how to ascertain an object’s type in C++ is crucial, especially when implementing polymorphism, as it allows for more flexible and reusable code.
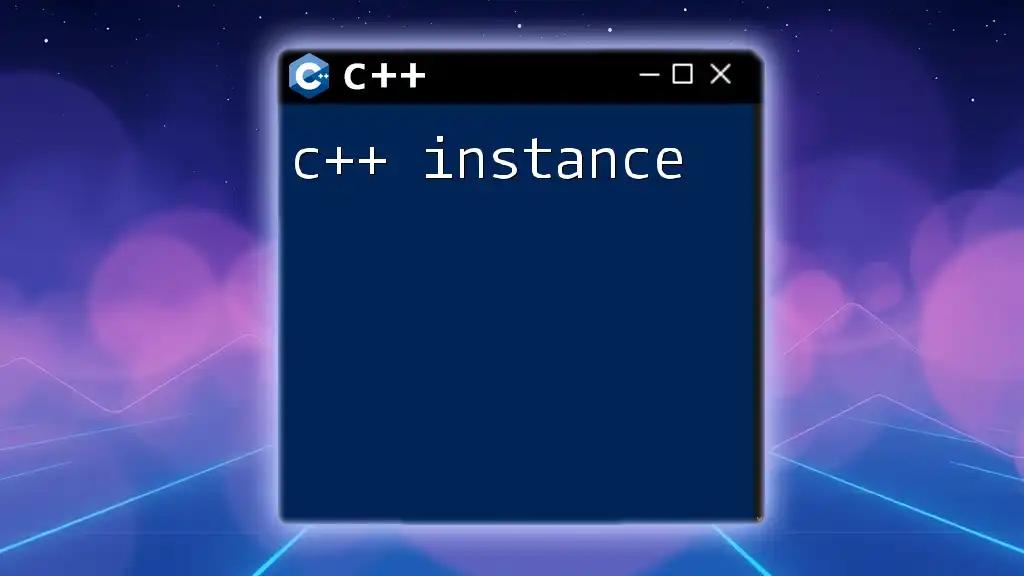
The Basics of Type Checking
Type checking refers to the method by which a programming language verifies the type of variables and expressions. C++ incorporates both static and dynamic type checking.
Static vs Dynamic Type Checking
-
Static Type Checking occurs at compile-time. This means that the type integrity of objects is enforced before the program runs. C++ uses strong static type checking, so if you try to perform operations on mismatched types, the compiler generates errors.
-
Dynamic Type Checking happens during runtime, allowing the program to determine the type of an object as it executes. This is particularly important in situations where types are ambiguous, especially in object-oriented programming.
Understanding the difference between these two forms of type checking is foundational when exploring the nuances of type identification in C++.
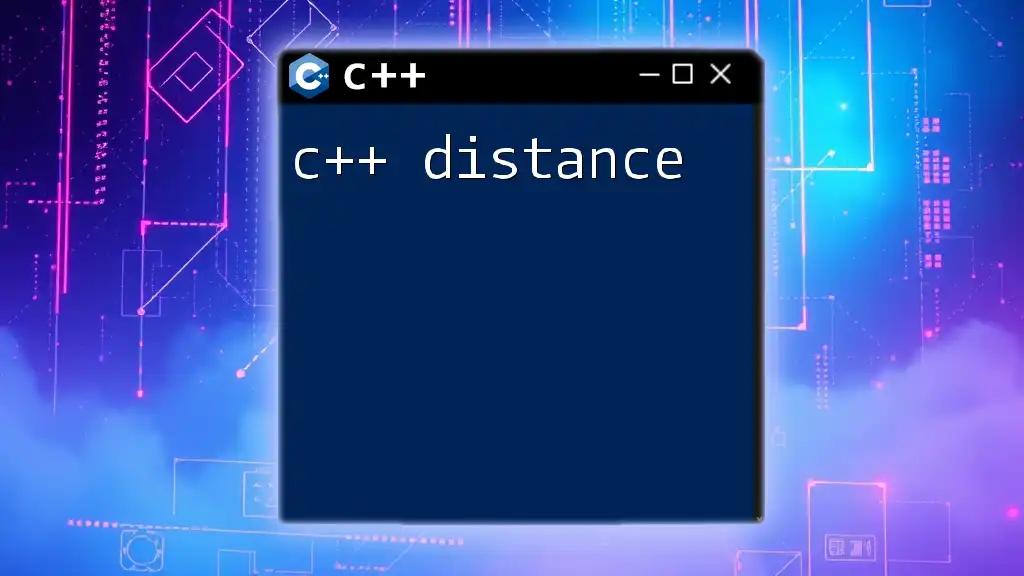
Implementing "instanceof" in C++
In C++, there isn't a direct equivalent to `instanceof`, but similar functionality can be achieved using the `typeid` and `dynamic_cast` operators.
The `typeid` Operator
The `typeid` operator provides information about the type of an expression at runtime. This makes it similar to what "instanceof" does in other programming languages.
Example of Using `typeid`
#include <iostream>
#include <typeinfo>
class Base {};
class Derived : public Base {};
int main() {
Base* basePtr = new Derived();
// Using typeid to check the type
if (typeid(*basePtr) == typeid(Derived)) {
std::cout << "basePtr is pointing to an instance of Derived." << std::endl;
} else {
std::cout << "basePtr is not pointing to an instance of Derived." << std::endl;
}
delete basePtr;
return 0;
}
In this code snippet, we utilize `typeid` to check if `basePtr` points to an instance of `Derived`. This method is direct and effective for runtime type identification.
The `dynamic_cast` Operator
The `dynamic_cast` operator is used for safely downcasting pointers or references to base classes to derived classes. It ensures type safety and returns `nullptr` if the cast fails. It's especially useful in polymorphic contexts where type validation is critical.
Example of Using `dynamic_cast`
#include <iostream>
class Base {
public:
virtual ~Base() {} // Ensure that Base is polymorphic
};
class Derived : public Base {
public:
void derivedFunction() {
std::cout << "Called derivedFunction." << std::endl;
}
};
int main() {
Base* basePtr = new Derived();
// Using dynamic_cast
if (Derived* derivedPtr = dynamic_cast<Derived*>(basePtr)) {
derivedPtr->derivedFunction(); // Success!
} else {
std::cout << "basePtr is not pointing to an instance of Derived." << std::endl;
}
delete basePtr;
return 0;
}
In this example, `dynamic_cast` checks if `basePtr` can be safely cast to a `Derived*`. If successful, we can safely call methods defined in the `Derived` class.
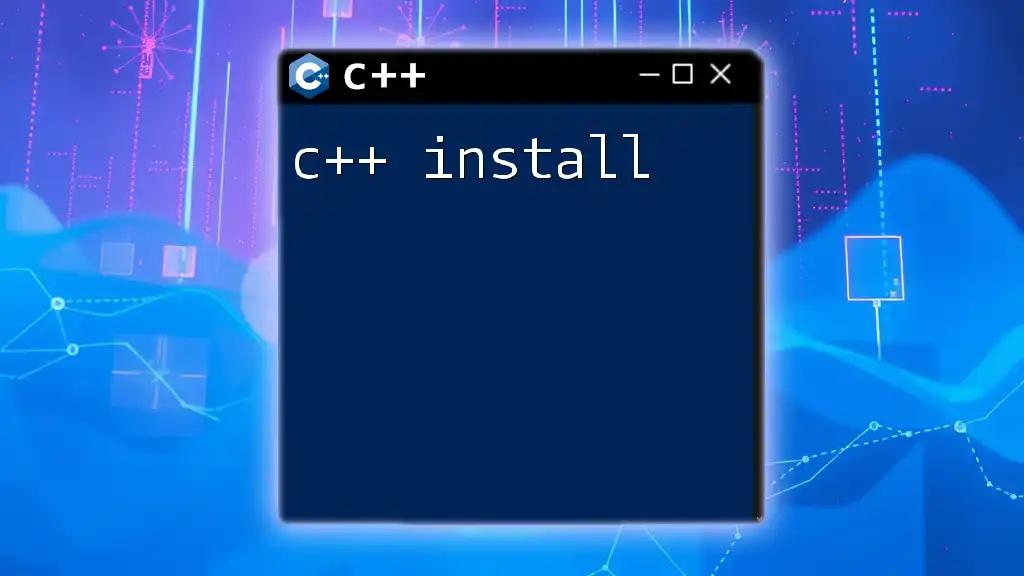
Practical Examples of Type Checking
Example 1: Basic Class Hierarchy
Creating a simple class hierarchy can illustrate how type checking works.
#include <iostream>
class Base {
public:
virtual ~Base() {}
};
class DerivedA : public Base {};
class DerivedB : public Base {};
int main() {
Base* basePtr = new DerivedA();
// Using dynamic_cast to determine the type
if (dynamic_cast<DerivedB*>(basePtr)) {
std::cout << "basePtr is of type DerivedB." << std::endl;
} else {
std::cout << "basePtr is NOT of type DerivedB." << std::endl;
}
delete basePtr;
return 0;
}
In this code, `basePtr` is a pointer to `DerivedA`. The `dynamic_cast` check for `DerivedB` returns `nullptr`, confirming the type of `basePtr`.
Example 2: `typeid` in Action
Using `typeid` in a program lets you perform multiple type checks and handle objects based on runtime type.
#include <iostream>
#include <typeinfo>
class Animal {
public:
virtual ~Animal() {}
};
class Dog : public Animal {};
class Cat : public Animal {};
void identifyAnimal(Animal *animal) {
if (typeid(*animal) == typeid(Dog)) {
std::cout << "It's a Dog!" << std::endl;
} else if (typeid(*animal) == typeid(Cat)) {
std::cout << "It's a Cat!" << std::endl;
} else {
std::cout << "Unknown Animal!" << std::endl;
}
}
int main() {
Dog myDog;
Cat myCat;
identifyAnimal(&myDog);
identifyAnimal(&myCat);
return 0;
}
Here, `identifyAnimal` checks the type of the passed `Animal` pointer and prints a message accordingly.
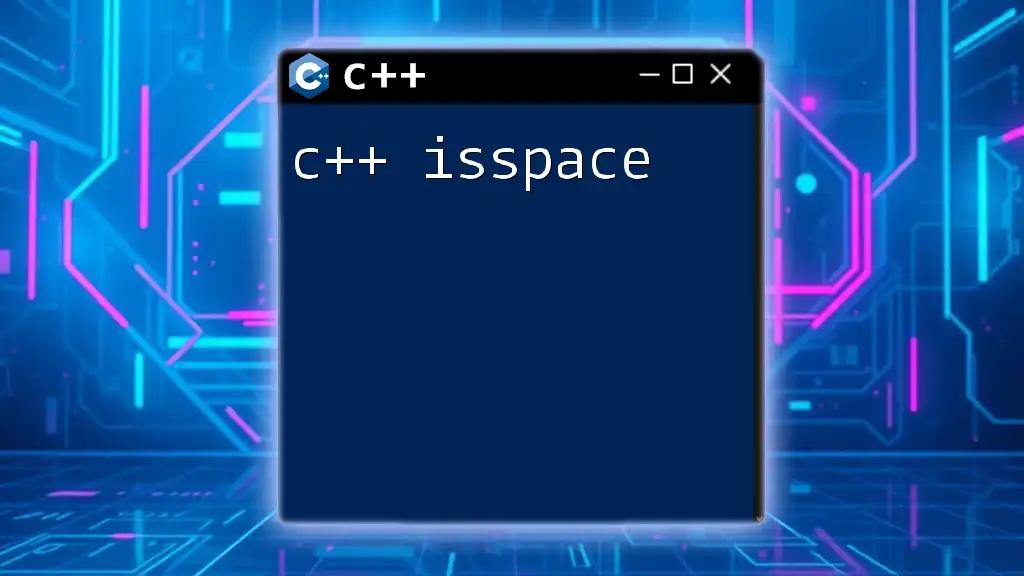
Common Pitfalls and Best Practices
While using `dynamic_cast` and `typeid`, developers should be aware of potential issues.
-
One common pitfall is failing to declare the base class as polymorphic by including at least one virtual function. This should be done to ensure that `dynamic_cast` and `typeid` work correctly.
-
Always check the return value of `dynamic_cast`. If it returns `nullptr`, this means the cast was unsuccessful, often leading to undefined behavior if you attempt to use that pointer again without verification.
When implementing type identification, prefer `dynamic_cast` for polymorphic types to ensure safety over `static_cast`, which does not perform any type checks.
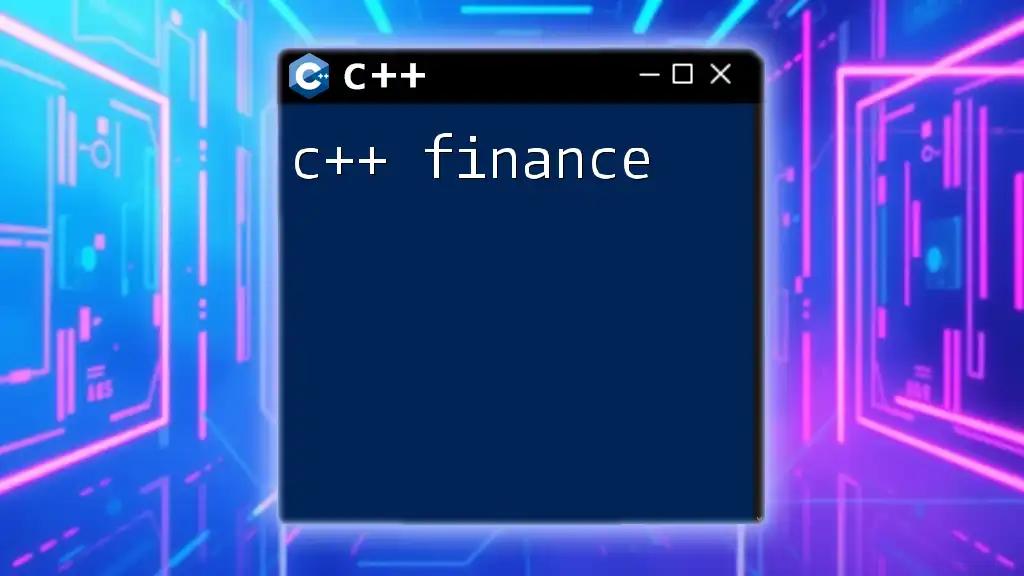
Real World Applications of "instanceof"
Type identification is invaluable in scenarios such as event handling in graphical user interfaces where callbacks or events may be directed to various types of objects. Frameworks like Qt and Boost heavily leverage polymorphism, with type checking facilitating robust event management.
- In event-driven systems, understanding the exact type of an event receiver or handler is essential for executing specific behaviors tied to different classes.
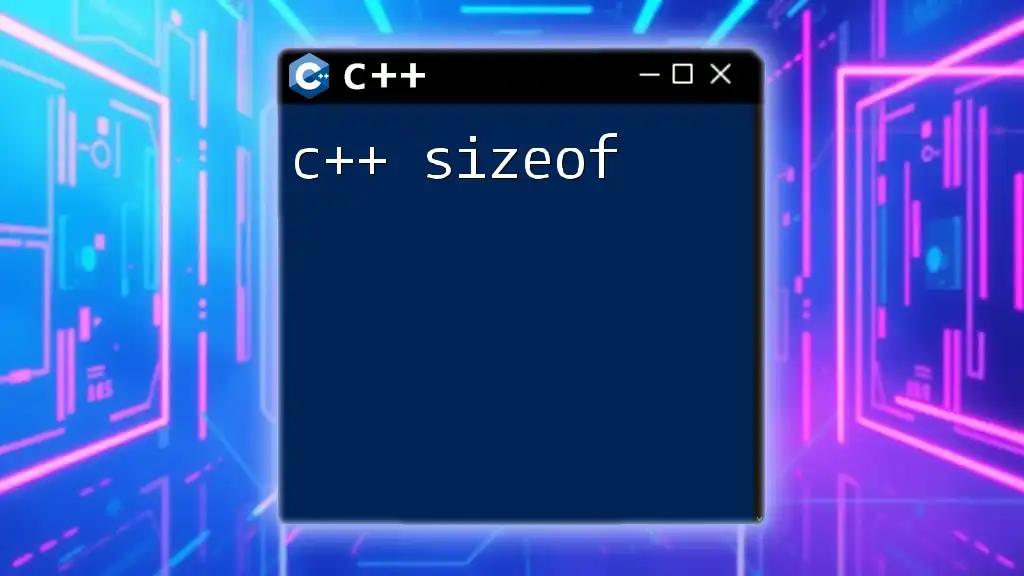
Conclusion
Mastering C++'s type checking mechanisms allows developers to write more robust and maintainable code. By leveraging `typeid` and `dynamic_cast`, you can implement effective type checking similar to "instanceof" in other languages. Understanding when and how to use these techniques empowers you to manage polymorphic behavior and ensures type safety in your applications.
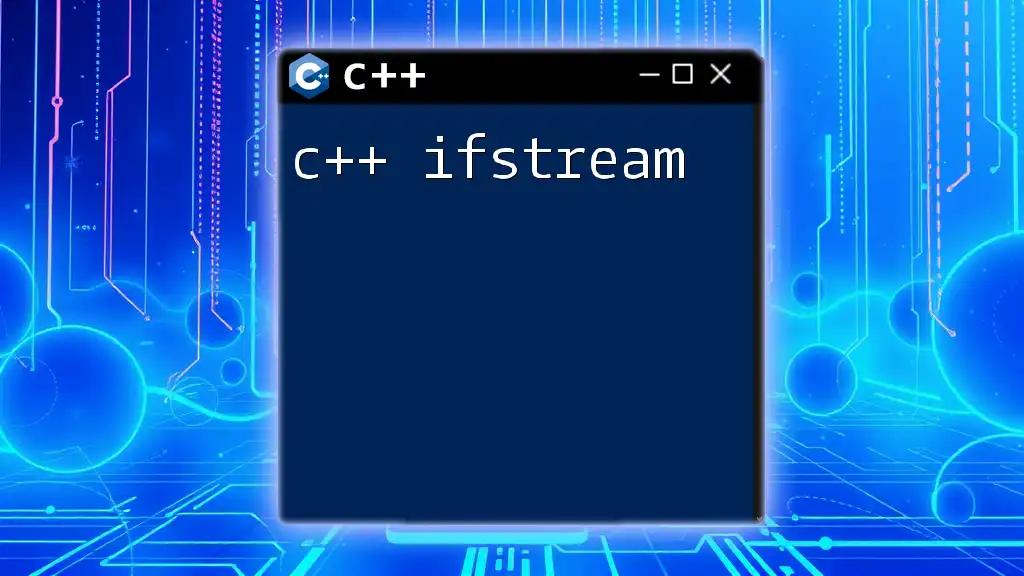
FAQs
Is "instanceof" a standard keyword in C++?
No, C++ does not have an `instanceof` keyword like Java. Instead, it provides `typeid` and `dynamic_cast` for similar functionality.
When should I use `dynamic_cast`?
Use `dynamic_cast` when you are unsure of an object's type in a polymorphic hierarchy and need to safely downcast to a derived type.
Are there alternatives to `typeid`?
Yes, alternatives include designing with RTTI (Runtime Type Information) and implementing various design patterns like Visitor or State, which can help circumvent the need for explicit type checking.