A C++ sandbox is an isolated environment that allows developers to experiment with C++ code snippets without the risk of affecting the broader system or software.
Here’s a simple example of a C++ program that prints "Hello, World!" to the console, which can be run in a sandbox environment:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is a C++ Sandbox?
A C++ sandbox is an isolated environment where programmers can experiment with C++ code without the risk of affecting their main development projects. It serves as a perfect playground for learning, testing, and honing skills in C++ programming. The importance of a C++ sandbox cannot be overstated, especially for beginners who need a safe space to explore the intricacies of the C++ language. By utilizing a sandbox, developers can quickly iterate on ideas and troubleshoot issues without the overhead of complex setups or serious consequences of erroneous code.
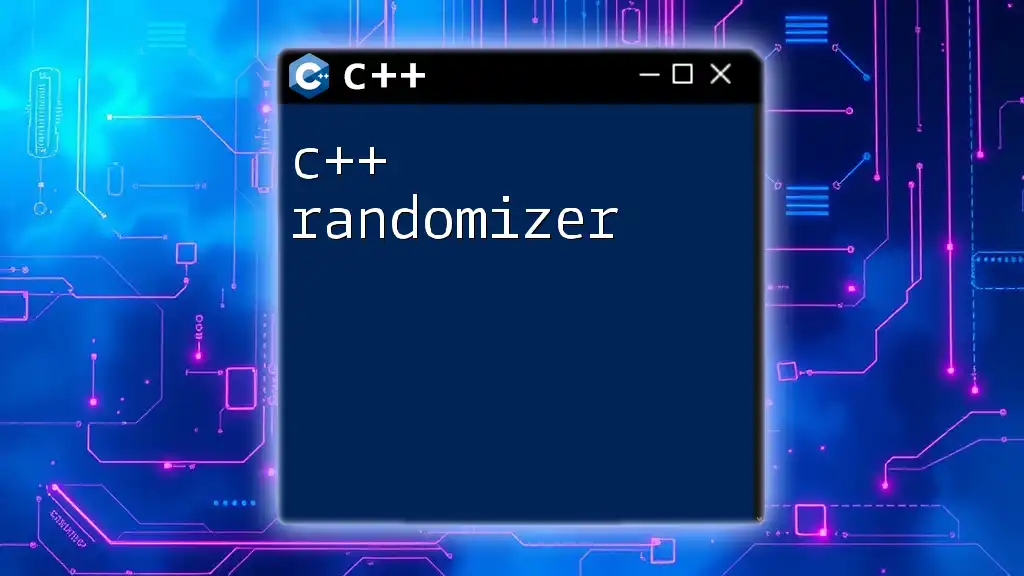
Setting Up Your C++ Sandbox
Choosing the Right Environment
When selecting a sandbox, developers must choose between local and online options.
Local Sandboxes are installed on personal computers and allow for full control over the setup. They often offer advanced features suited for heavy development work and debugging.
Online Sandboxes provide instant access to coding environments via web browsers, making them ideal for quick tests or introductions to C++ without installation. Popular online options include Repl.it and OnlineGDB.
Installing the Necessary Tools
Setting up a local C++ development environment typically involves choosing a compiler and an Integrated Development Environment (IDE).
Recommended C++ Compilers:
- GCC (GNU Compiler Collection)
- Clang (C Language Family Frontend)
- MSVC (Microsoft Visual C++)
Popular IDEs include:
- Visual Studio
- Code::Blocks
- CLion
These tools facilitate code writing, debugging, and project management, streamlining the developer's workflow.
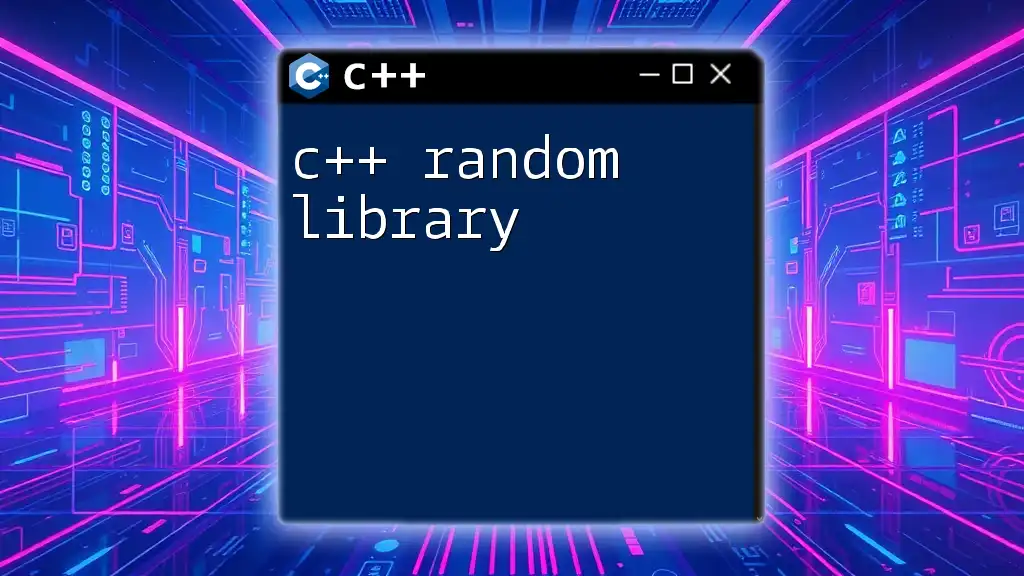
Working with Online C++ Sandboxes
Popular Online C++ Sandboxes
Repl.it is a versatile online IDE that supports multiple programming languages, including C++. Its user-friendly interface enables developers to write, run, and share C++ code seamlessly. A typical workflow on Repl.it involves writing code directly in the browser and executing it with the press of a button.
Example of using Repl.it for C++ code execution:
#include <iostream>
int main() {
std::cout << "Welcome to Repl.it!" << std::endl;
return 0;
}
OnlineGDB is another powerful option, featuring an integrated debugging tool that provides a step-by-step execution view. This is especially beneficial for learning as it highlights errors in real-time.
Example code snippet demonstrating usage:
#include <iostream>
int main() {
int a = 5, b = 10;
std::cout << "Sum: " << (a + b) << std::endl;
return 0;
}
Compiler Explorer stands out by allowing developers to write code and see the compilation results and assembly output instantly. This helps in understanding how C++ code translates into machine instructions.
Example code showing output and assembly generation:
#include <iostream>
int main() {
std::cout << "Compiler Explorer is a great tool!" << std::endl;
return 0;
}
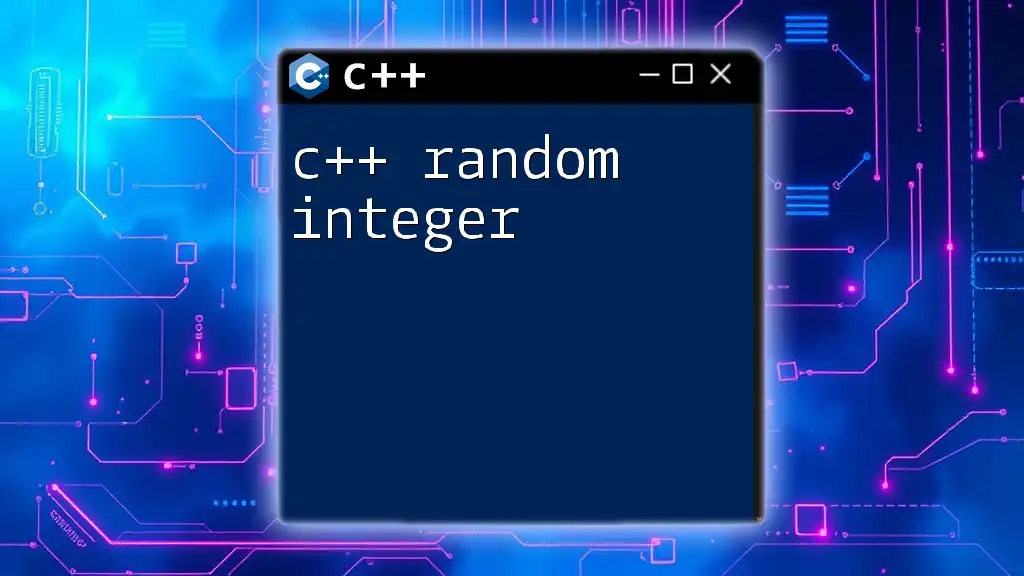
Creating a Local C++ Sandbox
Setting Up Your Local Environment
The first step in creating a local sandbox is installing the necessary tools. Installation guides vary by operating system (Windows, macOS, Linux) but generally, they involve downloading the compiler and IDE of choice and setting them up through configuration wizards.
Writing Your First Program
Once the environment is set up, it’s time to write a simple program. A classic "Hello, World!" example establishes the foundation of C++ syntax.
Hello World Example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code:
- `#include <iostream>` imports the input-output stream library.
- `int main()` defines the main function where program execution begins.
- `std::cout` prints text to the output console.
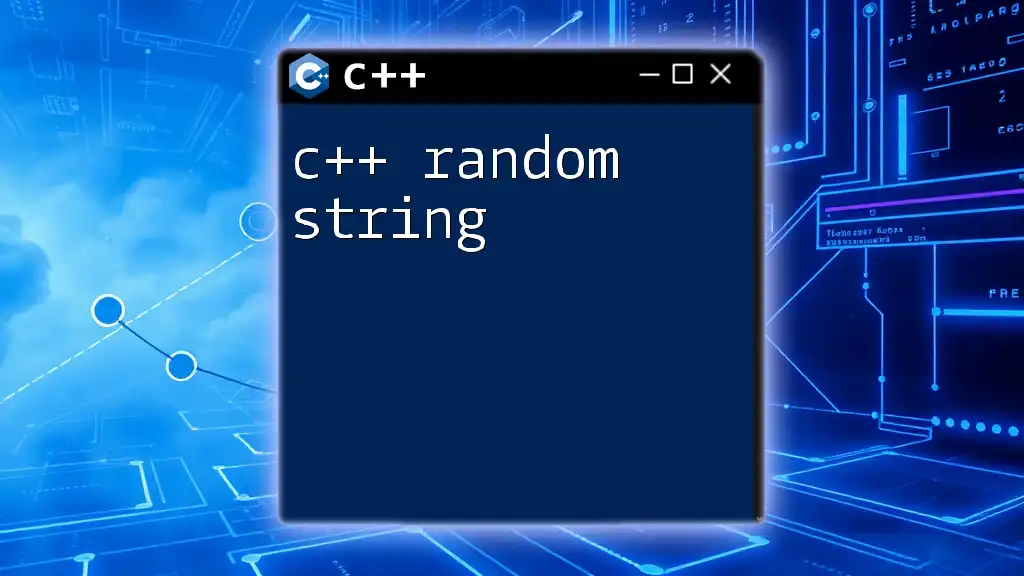
Exploring C++ Syntax and Features in the Sandbox
Basic Syntax
Understanding C++ syntax is crucial. This includes variables, data types, and operators.
Variables are identifiers used to store data values. For example:
int age = 30;
float height = 5.9;
char initial = 'A';
Data Types include:
- `int` for integers
- `float` for floating-point numbers
- `char` for characters
C++ also provides various operators for arithmetic, comparison, and logical operations, enabling developers to perform complex calculations with ease.
Control Structures
Control structures like conditionals and loops are vital for managing program flow.
Conditionals (if, else) allow for decisions in programming. Here’s an example demonstrating conditionals:
int x = 10;
int y = 20;
if (x > y) {
std::cout << "x is greater" << std::endl;
} else {
std::cout << "y is greater or equal" << std::endl;
}
In this case, the program evaluates the values of `x` and `y` before deciding which message to print.
Loops (for, while) are essential for performing repeated tasks. A basic example of a for loop is shown below:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
Functions and Classes
Defining Functions allows for code reusability and better organization. An example of a simple function is:
void greet() {
std::cout << "Hello, User!" << std::endl;
}
This function can be called multiple times, promoting modular design.
Class Basics introduce the object-oriented features of C++. Consider this basic class representing a Dog:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
In this case, the class encapsulates a behavior (barking) that can be instantiated and executed.
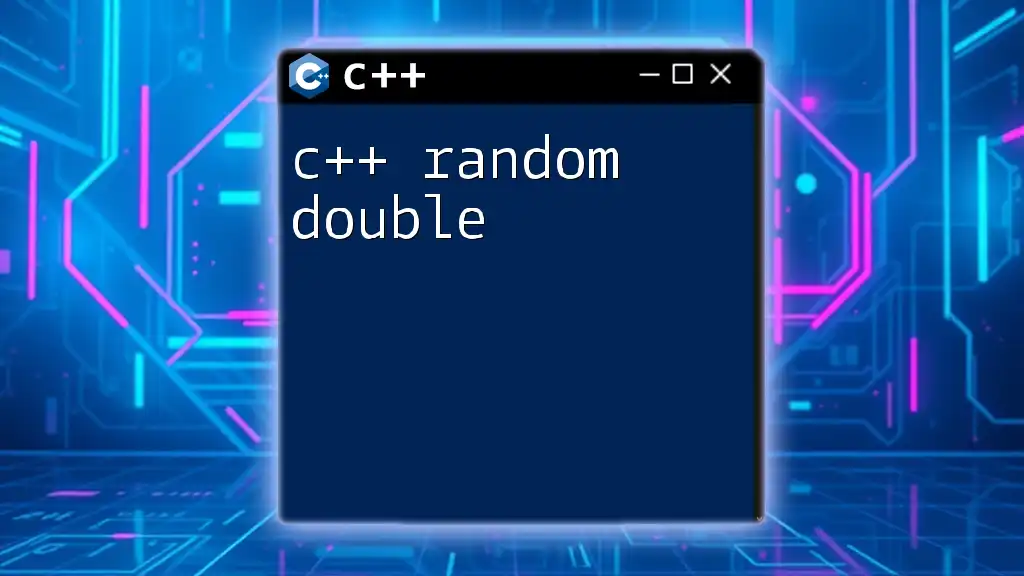
Best Practices in Using a C++ Sandbox
Code Organization
Good code organization is essential for maintainability. Modular Programming encourages the separation of functionality into distinct modules or files, making it easier to navigate large projects. For example, dividing code into `main.cpp`, `utilities.cpp`, and `classes.cpp` can lead to clearer structures.
Debugging Techniques
Familiarity with debugging tools is crucial for developers. Common tools like GDB (GNU Debugger) or built-in IDE debuggers allow the inspection of variables, stack traces, and program flow, guiding developers through diagnosing issues effectively.
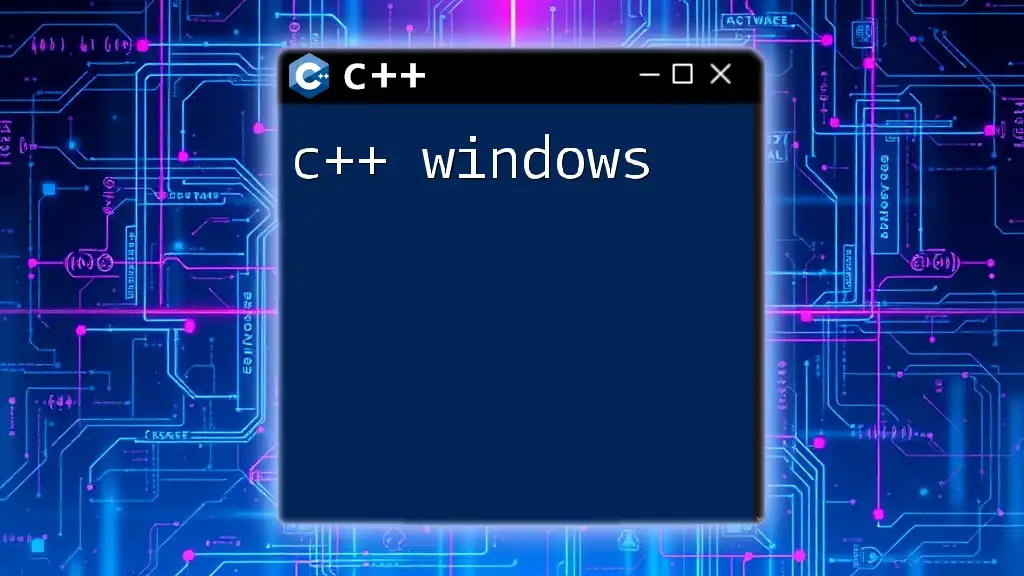
Conclusion
Using a C++ sandbox offers an unparalleled opportunity to refine coding skills in a risk-free environment. It encourages experimentation, providing immediate feedback that is vital for learning. To truly master C++, one must embrace the sandbox experience, practicing regularly and challenging oneself with new concepts.
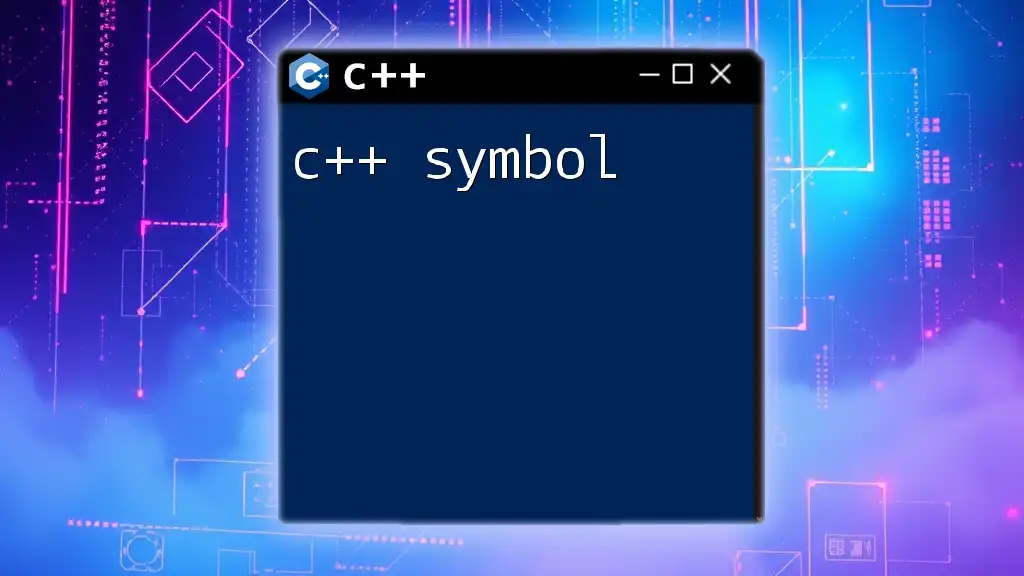
Additional Resources
For those looking to deepen their understanding of C++, resources abound. Books and online courses, such as those on platforms like Udemy, Coursera, and edX, offer structured learning pathways.
Engaging with community forums on websites like Stack Overflow or Reddit can also provide invaluable support and encouragement, helping you connect with fellow programmers on your journey to mastering C++.