In C++, the `std::exception` class serves as the base class for all standard exceptions, allowing developers to handle errors in a uniform manner.
Here's a simple example demonstrating how to use `std::exception`:
#include <iostream>
#include <stdexcept>
int main() {
try {
throw std::runtime_error("An error occurred");
} catch (const std::exception& e) {
std::cerr << "Caught an exception: " << e.what() << std::endl;
}
return 0;
}
Understanding std::exception
Exceptions in C++ are a fundamental aspect of error handling, allowing developers to manage unexpected situations in a controlled manner. The `std::exception` class, part of the C++ Standard Library, serves as the base class for all standard exceptions. Understanding its structure and usage is crucial for writing robust C++ applications.
What is std::exception?
The `std::exception` class is a polymorphic class designed to handle exceptions in a generic manner. It provides a base interface for standard library exceptions, enabling polymorphic behavior and consistent handling across various exception types. Each derived class represents a category of error, making it easier for developers to catch specific exceptions and respond appropriately.
Common std::exception Derived Classes
C++ provides several standard exception types derived from `std::exception` that capture different error scenarios. Some of the most frequently used derived classes include:
std::runtime_error: This exception indicates that an error occurred during program execution. Common examples include issues like resource allocation failures and unexpected behaviors.
Example of `std::runtime_error`:
try {
throw std::runtime_error("Runtime error encountered.");
} catch (const std::exception& e) {
std::cerr << "Caught: " << e.what() << std::endl;
}
std::logic_error: This exception signifies that a logical error has occurred, typically due to incorrect program logic or input. An example of this might be providing an invalid argument that violates program assumptions.
Example of `std::logic_error`:
try {
throw std::logic_error("Logic error occurred.");
} catch (const std::exception& e) {
std::cerr << "Caught: " << e.what() << std::endl;
}
std::out_of_range: This exception is thrown to indicate that an attempt was made to access an element outside the valid range of a container, like accessing an index in a vector that does not exist.
Example of `std::out_of_range`:
std::vector<int> vec = {1, 2, 3};
try {
int value = vec.at(5); // This will throw
} catch (const std::out_of_range& e) {
std::cerr << "Caught: " << e.what() << std::endl;
}
std::invalid_argument: Raised when a function receives an invalid argument. This error usually indicates that while the argument was of the correct type, it wasn't appropriate for the specific context.
Example of `std::invalid_argument`:
try {
throw std::invalid_argument("Invalid argument provided.");
} catch (const std::exception& e) {
std::cerr << "Caught: " << e.what() << std::endl;
}

Throwing Exceptions
Understanding how to throw exceptions is essential for proper error management in C++. The syntax is straightforward—use the `throw` keyword followed by an instance of an exception class.
Basic syntax of throwing exceptions:
throw std::runtime_error("An error occurred.");
Best practices for throwing exceptions include throwing exceptions in response to adverse conditions rather than simply returning error codes. This approach enhances code readability and maintainability, facilitating easier debugging and error handling.

Catching Exceptions
Once exceptions are thrown, they need to be caught to handle them gracefully. This is achieved using `try` and `catch` blocks.
The basic structure looks like this:
try {
// Code that may throw an exception
} catch (const std::exception& e) {
std::cerr << "Exception caught: " << e.what() << std::endl;
}
C++ allows you to have multiple `catch` blocks, enabling you to handle various exception types differently.
Example demonstrating multiple catch blocks:
try {
throw std::out_of_range("Out of range error.");
} catch (const std::runtime_error& e) {
std::cerr << "Caught runtime error: " << e.what() << std::endl;
} catch (const std::out_of_range& e) {
std::cerr << "Caught out of range error: " << e.what() << std::endl;
}
When you catch exceptions, keep in mind whether to catch by value or reference. Catching by reference is generally preferred as it avoids unnecessary copies of the exception object, ensuring better performance.

Custom Exceptions
Sometimes, the built-in exceptions may not suffice for your specific error conditions. In such cases, creating custom exceptions can be beneficial. Custom exception classes can provide additional context about the error condition.
To create a custom exception, derive your class from `std::exception` and override the `what()` method.
class MyCustomException : public std::exception {
public:
const char* what() const noexcept override {
return "My custom exception occurred";
}
};
Consider using custom exceptions when you want to represent errors specific to your application’s domain. This enhances clarity and provides better debugging information.

Exception Safety
Understanding exception safety is crucial to writing reliable code. There are three levels of exception safety:
-
Basic Guarantee: Ensures that if an exception is thrown, object invariants are preserved, but some operations may not have been completed.
-
Strong Guarantee: If an exception is thrown, the program state remains unchanged, allowing for safe rollback of operations.
-
No Guarantee: There is no specific assurance; an exception may leave program objects in an invalid state.
Implementing exception safety in your code often involves applying RAII (Resource Acquisition Is Initialization) principles and using smart pointers. These tools help manage resources and ensure that they are released properly, even if an exception occurs.
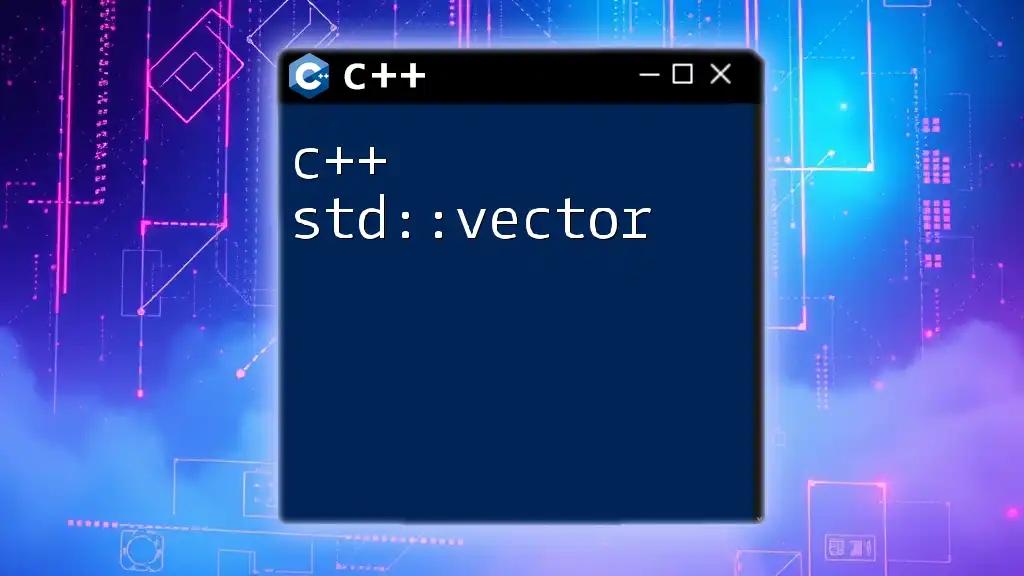
Practical Use Cases of C++ Exceptions
In real-world applications, exceptions are crucial for managing unpredictable behaviors in libraries and frameworks. For instance, when performing file I/O operations, you might need to handle various exceptions that arise from file access issues or permissions.
Using exceptions simplifies error handling by allowing you to separate error-handling logic from the main code flow, leading to cleaner and more understandable code.
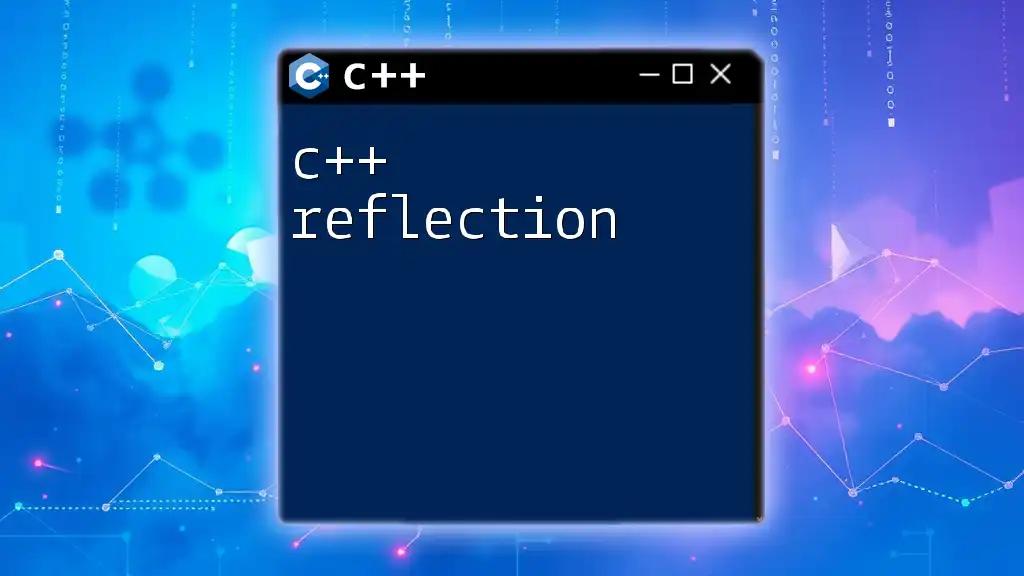
Conclusion
Understanding the C++ std exception is essential for effective error handling in C++. By effectively using standard exceptions, creating custom exceptions, and implementing exception safety principles, developers can write resilient applications. Emphasizing the importance of exception handling will help create more robust, maintainable, and user-friendly software solutions.
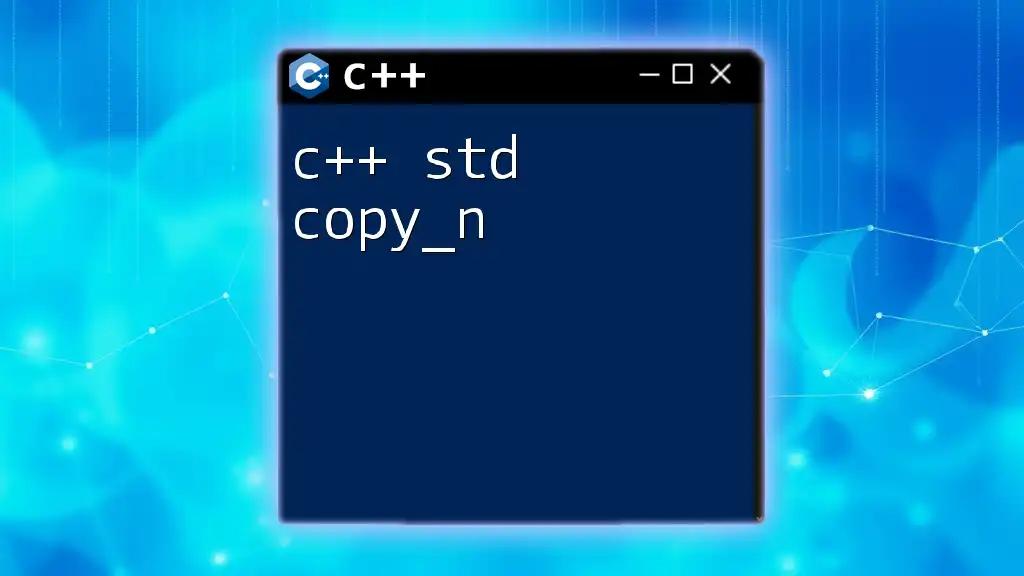
Additional Resources
For further exploration of C++ exceptions, consider consulting resources such as C++ programming books, online documentation, and community forums that focus on advanced exception handling techniques. These will deepen your understanding and improve your skills in managing exceptions within your applications.