In C++, an exception class is a user-defined class derived from `std::exception` that allows you to create custom exceptions to handle specific error scenarios in your programs.
#include <iostream>
#include <exception>
class MyException : public std::exception {
public:
const char* what() const noexcept override {
return "This is a custom exception!";
}
};
int main() {
try {
throw MyException();
} catch (const MyException& e) {
std::cout << e.what() << std::endl;
}
return 0;
}
What is an Exception Class?
An exception class is a critical component of C++ that allows developers to handle errors and exceptional situations gracefully during the execution of a program. Exception classes enable structured error management, enhancing the robustness of applications by separating error-handling code from regular code flow. An exception class typically includes a structure to represent the error, properties that describe it, and methods to manage it.
Exception handling is built upon three major elements: throwing an exception when an error occurs, catching that exception in a controlled way, and propagating it up the call stack. This model provides a cleaner and more efficient manner of managing errors compared to traditional error-checking mechanisms.

Built-in Exception Classes in C++
C++ comes equipped with several built-in exception classes that are part of the standard library. These classes provide a predefined way of handling common issues, allowing developers to manage exceptions more effectively.
-
`std::exception`: This is the base class for all standard exceptions. It has a virtual method `what()`, which returns a C-style character string describing the exception.
-
`std::runtime_error`: Inherits from `std::exception`. This exception should be used for errors that can only be detected during runtime, such as invalid input or failure in the logic of the program.
-
`std::logic_error`: Also inherits from `std::exception`, but is intended for errors related to the program's logic or incorrect assumptions used in the program.
Example: Standard Exception Usage
Here’s how you can use the built-in `std::runtime_error` exception in a program:
#include <iostream>
#include <stdexcept>
int main() {
try {
throw std::runtime_error("A runtime error occurred!");
} catch (const std::exception& e) {
std::cout << e.what() << std::endl;
}
}

Creating Custom Exception Classes
While C++ provides built-in exception classes, there are instances where creating a custom exception class is beneficial. Custom exception classes allow developers to better represent specific error conditions pertinent to their application domain.
To create a custom exception class, inherit from `std::exception` and override the `what()` method to return a description of the error. This practice enhances clarity and maintainability of the code, making it easier to handle exceptions effectively.
Example: Creating a Custom Exception Class
Here’s a practical example of how to implement a custom exception class:
#include <iostream>
#include <exception>
#include <string>
class MyException : public std::exception {
public:
MyException(const std::string& message) : msg_(message) {}
virtual const char* what() const noexcept override {
return msg_.c_str();
}
private:
std::string msg_;
};
int main() {
try {
throw MyException("This is a custom exception!");
} catch (const std::exception& e) {
std::cout << e.what() << std::endl;
}
}
In this example, `MyException` provides a specific error message when the exception is thrown, offering clarity regarding the nature of the problem encountered.
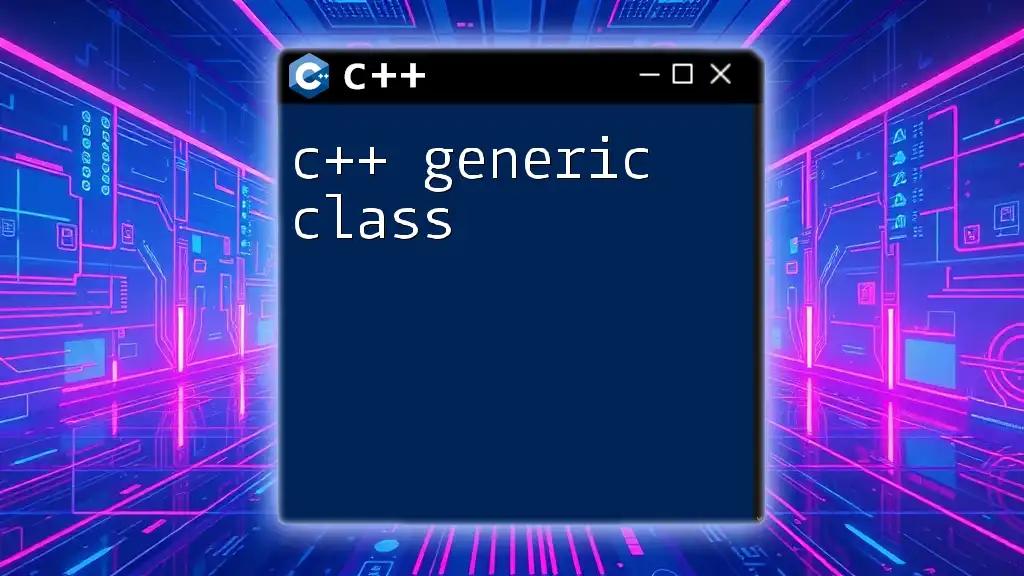
How to Use Exception Classes in C++
Implementing exception classes in your code involves the use of try-catch blocks. The code that may potentially throw an exception is enclosed in a try block. If an error occurs, the control is passed to the corresponding catch block, which handles the exception.
Best practices dictate that you should only throw exceptions for errors which you are not able to process, allowing your program to recover from these situations effectively. When catching exceptions, be specific about the type of exception you are catching in order not to obscure other potential issues.
Example: Using Try-Catch with Exceptions
Here’s how to use try-catch blocks to handle exceptions:
#include <iostream>
void riskyFunction() {
throw std::runtime_error("Something went wrong!");
}
int main() {
try {
riskyFunction();
} catch (const std::exception& e) {
std::cout << "Error caught: " << e.what() << std::endl;
}
}
The above code demonstrates the separation of error handling from the main logic, reinforcing the maintainability of both sections.

Performance Considerations with Exception Classes
When considering performance, it’s crucial to understand that while exceptions provide a powerful mechanism for error handling, they can introduce some overhead. Throwing exceptions involves stack unwinding, which can slow down execution.
Developers should also consider the impact of excessive use of exceptions in performance-sensitive applications. However, the benefits of clearer code structure and maintainability often outweigh the slight performance costs. It is wise to use them judiciously, particularly in performance-critical paths.

Best Practices for Exception Handling in C++
To effectively manage exceptions in your programs, adhere to the following best practices:
- Use exceptions for exceptional conditions: Only throw exceptions for conditions that the normal control flow cannot handle.
- Be specific when catching exceptions: Catch specific exception types rather than using a catch-all. This allows for targeted error handling.
- Avoid throwing exceptions in destructors: If a destructor throws an exception, it can cause the program to terminate unexpectedly.
- Don’t use exception specifications: They are deprecated and do not guarantee behavior in a predictable manner.

Common Mistakes in Exception Handling
Despite the elegance of exception handling, developers often fall into common pitfalls, such as:
- Catching exceptions too broadly: This can hide specific actionable responses to different errors.
- Failing to handle exceptions: Not having catch blocks can lead to unhandled exceptions, which will crash the program.
- Neglecting resource management: If exceptions occur, resources may not be released properly unless managed with RAII (Resource Acquisition Is Initialization) principles.
Ensuring careful design around exceptions can vastly improve the stability and maintainability of your code.
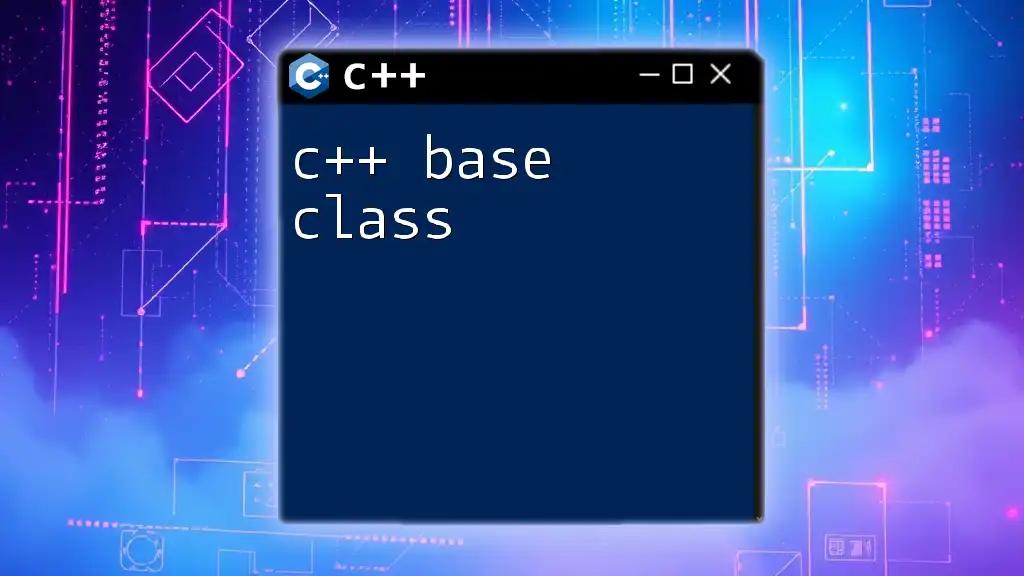
Conclusion
Understanding the C++ exception class is vital for effective error handling in modern C++ programming. By leveraging built-in and custom exception classes, structuring your code with try-catch blocks, and following best practices, you can create applications that are resilient to errors. The ability to handle errors gracefully significantly enhances user experience and system reliability.
Exploring exception handling deeply will empower you to write more robust and error-resistant C++ applications. Embrace the power of exception classes to elevate your coding skills and project outcomes.

Additional Resources
To further your understanding of C++ exceptions and error handling, consider exploring the following resources:
- Official C++ documentation for exception handling
- Online tutorials focusing on advanced C++ error handling techniques
- Recommended books that delve deeper into C++ programming concepts
By engaging with these resources, you will build a stronger foundation in handling errors and exceptions in your C++ applications.