In C++, the question mark is used in the ternary conditional operator, which provides a shorthand way to execute a simple if-else logic in a single line.
int a = 10, b = 20;
int max = (a > b) ? a : b; // max will be 20
What is the C++ Question Mark Operator?
The C++ question mark, commonly known as the ternary operator, is a unique conditional operator that allows for a more compact way to express conditional logic in a single line of code. Unlike traditional if-else statements, which can often lead to lengthy blocks of code, the ternary operator delivers a succinct alternative.
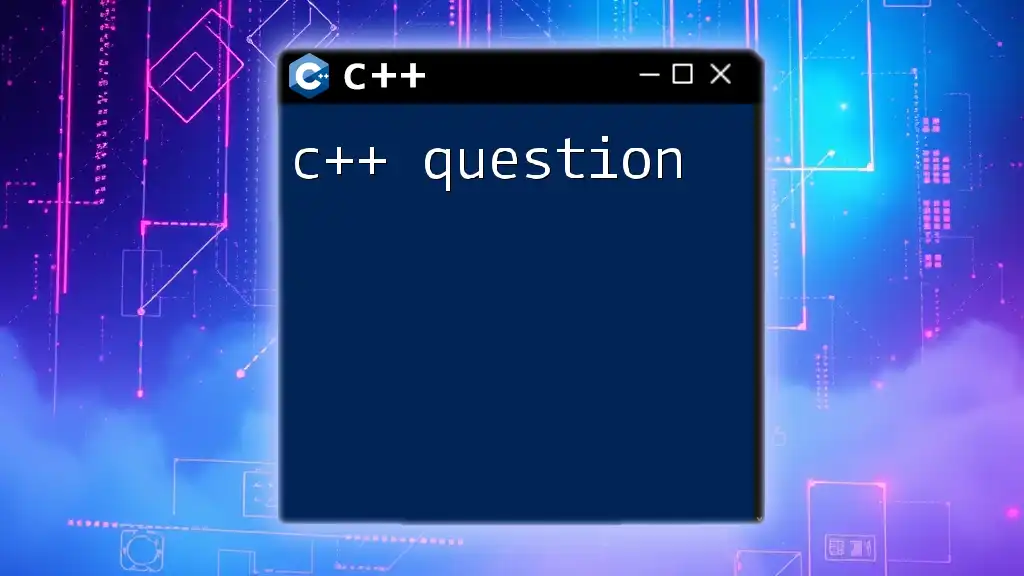
Syntax of the C++ Question Mark Operator
The syntax for using the question mark operator is simple:
condition ? expression1 : expression2;
In this structure:
- condition is a Boolean expression that evaluates to either true or false.
- expression1 is the value returned if the condition is true.
- expression2 is the value returned if the condition is false.
This compact arrangement allows for efficient decision-making in code.
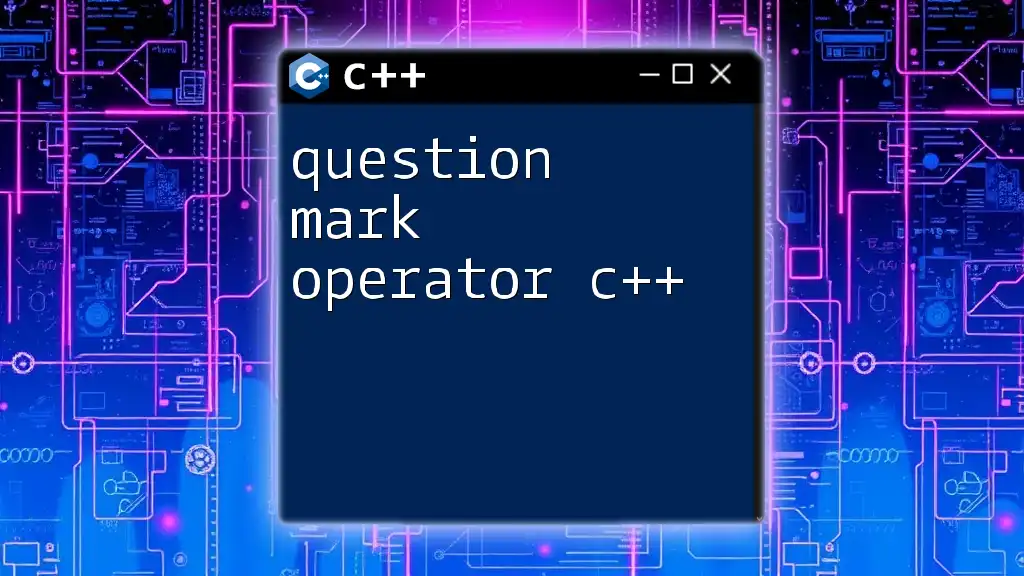
How the Ternary Operator Works
Explanation of the Logic
To fully grasp the functionality of the C++ question mark operator, it's essential to understand its logical flow. When the condition is evaluated, the operator determines which expression to execute:
- True case: If the condition is true, the operator will execute and return expression1.
- False case: Conversely, if the condition is false, it will move to expression2 and return that value.
For example:
bool isAdult = (age >= 18) ? true : false;
In this snippet, if `age` is 18 or older, `isAdult` will be assigned `true`; otherwise, it will be `false`.
Practical Examples
Here are a couple of practical examples that demonstrate the utility of the ternary operator.
In a simple case of finding the maximum of two numbers, you might write:
int a = 10, b = 20;
int max = (a > b) ? a : b;
// max will hold the value of 20
This example succinctly determines that `b` has the greater value and assigns it to `max`.
In another scenario, utilizing the ternary operator for string output can simplify code that checks a score:
std::string result = (score >= 50) ? "Pass" : "Fail";
Here, if `score` meets the threshold, "Pass" is assigned; otherwise, "Fail" is indicated.
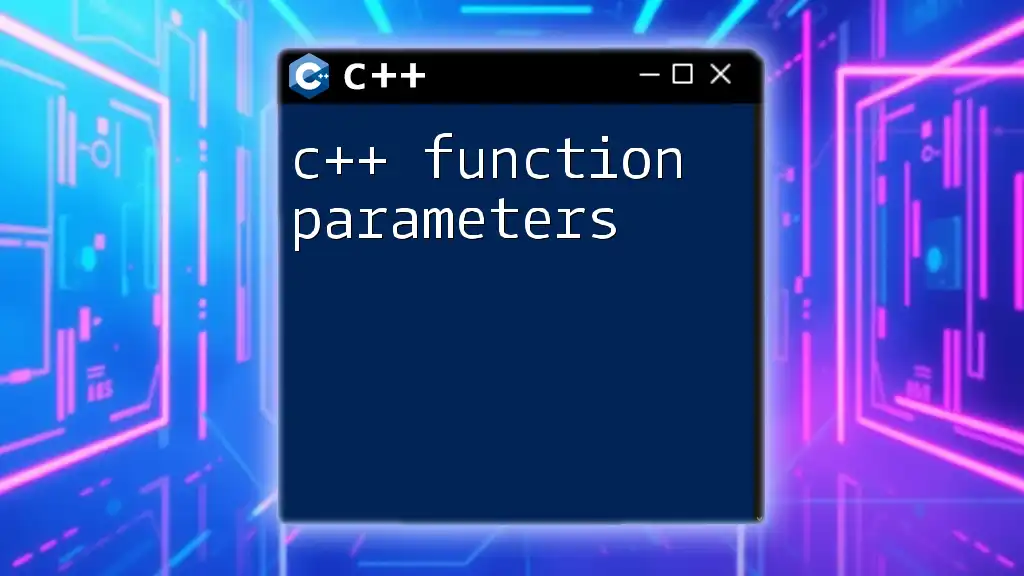
Advantages of Using the Ternary Operator
Conciseness and Clarity
One of the primary advantages of the C++ question mark is its ability to condense code into fewer lines. This compact representation can streamline programming, reducing visual clutter in straightforward conditions.
Enhanced Readability
When used appropriately, the ternary operator can improve readability, allowing other programmers to quickly understand a quick decision being made within the code. However, it’s essential to balance conciseness with clarity, especially in more complex scenarios.
Situations Ideal for Ternary Operator
The ternary operator shines in certain situations, such as:
- Variable assignment based on a simple condition.
- Inline decision making for functions that return values.
It's advisable to avoid usage in scenarios where nested conditions are involved, as they can lead to confusing code that becomes difficult to decipher.
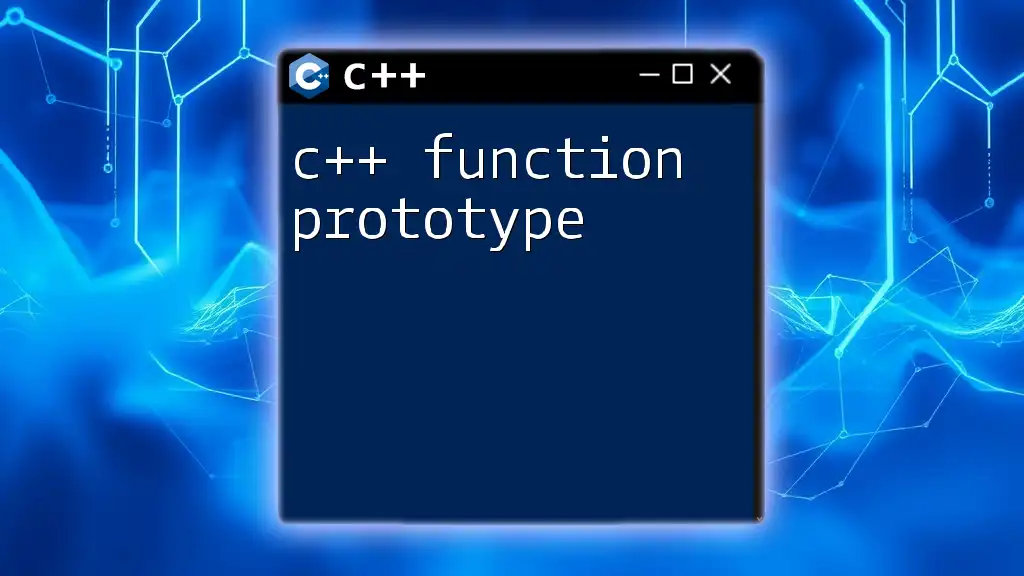
Common Use Cases for the C++ Question Mark Operator
Variable Assignment
Frequently, developers use the ternary operator for conditional variable assignment. Consider the following example:
int age = 16;
std::string status = (age >= 18) ? "Adult" : "Minor";
Here, the `status` variable will be either "Adult" or "Minor" based on the evaluation of `age`.
Function Returns
The ternary operator can also be integrated within function definitions for efficient return statements:
std::string checkEvenOdd(int num) {
return (num % 2 == 0) ? "Even" : "Odd";
}
In this case, when `checkEvenOdd` is called, the function evaluates if `num` is even or odd and returns the appropriate string.
Conditional Expressions in Output
Incorporating the ternary operator in output expressions can enhance clarity as well:
std::cout << ((number > 0) ? "Positive" : "Negative or Zero");
This statement succinctly indicates the status of `number` within a single line, simplifying the output process.
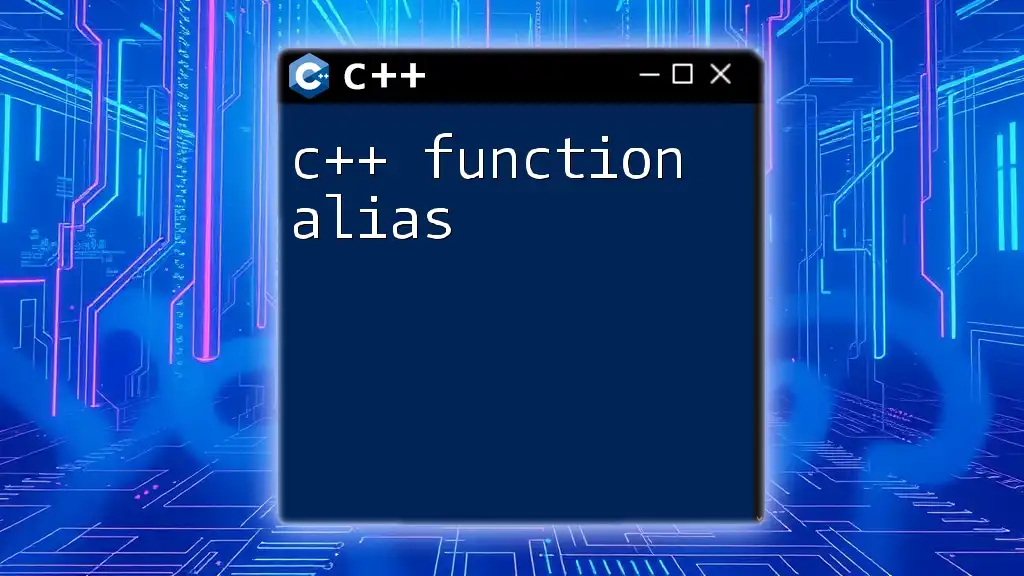
Potential Pitfalls of the Ternary Operator
Readability Concerns
While the ternary operator can make code elegant, it can also lead to reduced readability, especially when nested. For instance:
int num = 20;
std::string result = (num > 0) ? "Positive" : (num < 0) ? "Negative" : "Zero";
In this example, while it’s compact, it requires careful scrutiny to fully understand the logic at a glance.
Debugging Difficulty
Another potential drawback is the challenge in debugging complex ternary expressions. When you encounter issues, deciphering which result emerged from the chained conditions can be cumbersome. For this reason, it’s crucial to regularly consider whether employing the ternary operator contributes more clarity than it takes away.
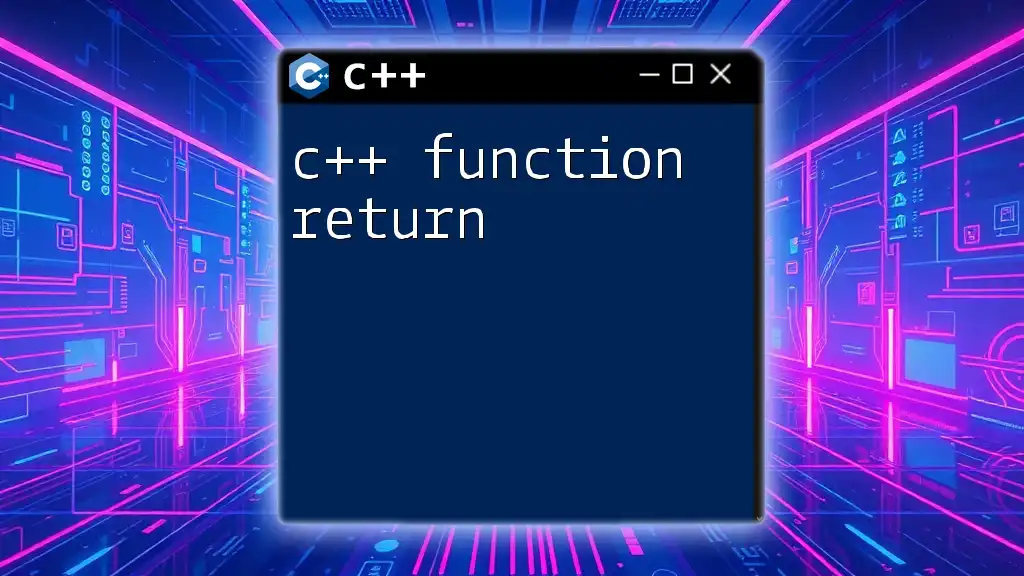
Conclusion
The C++ question mark operator is a powerful aspect of programming that, when used wisely, can simplify decisions and enhance the expressiveness of your code. With its succinct syntax and functional utility, it serves as an essential tool in a programmer's toolkit.
By understanding its functionality, advantages, and potential pitfalls, you can leverage the ternary operator effectively in your C++ programming. As you hone your skills, consider practicing its implementations in various scenarios to develop a more intuitive grasp of this powerful operator.
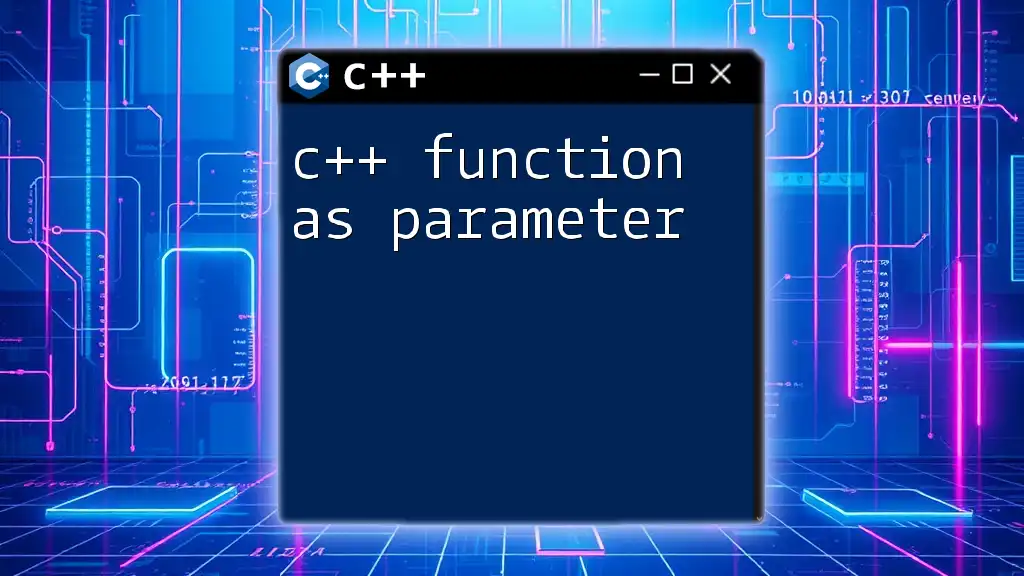
Further Reading and Resources
For those eager to deepen their understanding of the C++ question mark operator, consider exploring recommended books, engaging in online courses, and examining tutorials dedicated to mastering C++. Additionally, reviewing the official C++ documentation will further illuminate the nuances of this operator along with other related functionalities in C++.
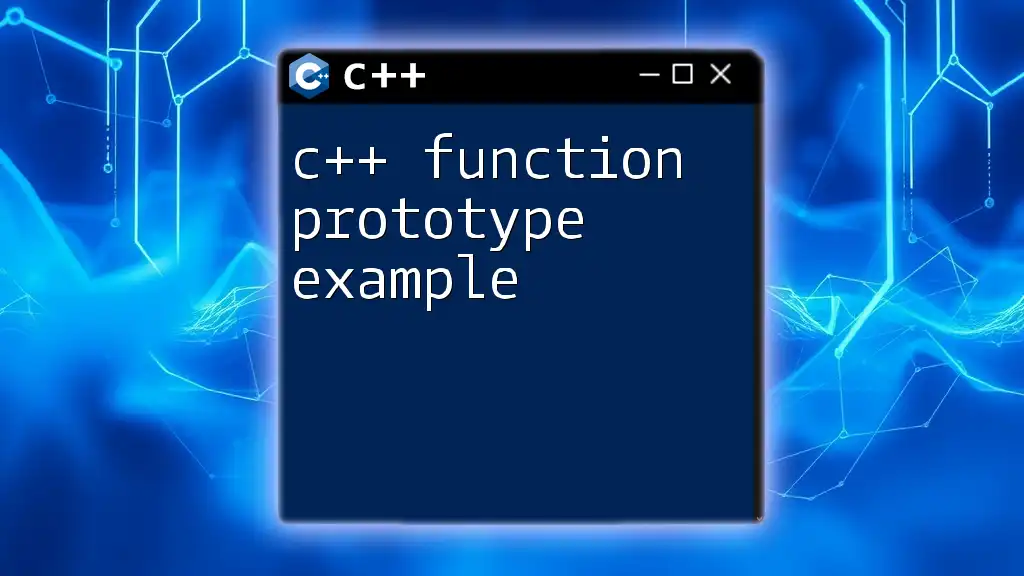
Call to Action
If you're interested in mastering C++ commands, join our course! With focused learning on operators such as the question mark operator, you'll enhance your programming capabilities and elevate your coding proficiency.