C++ JSON parsing involves the use of libraries to deserialize JSON data into C++ objects, enabling developers to easily manipulate and access the data within their applications.
#include <iostream>
#include <nlohmann/json.hpp>
int main() {
// Sample JSON string
std::string jsonString = R"({"name": "John", "age": 30})";
// Parse JSON
nlohmann::json jsonData = nlohmann::json::parse(jsonString);
// Access data
std::cout << "Name: " << jsonData["name"] << ", Age: " << jsonData["age"] << std::endl;
return 0;
}
Understanding JSON Structure
What is JSON?
JSON, or JavaScript Object Notation, is a lightweight data interchange format that is easy for humans to read and write, and easy for machines to parse and generate. It primarily uses two structures: objects (key-value pairs) and arrays (ordered lists). These structures allow for the representation of complex data sets in a simple format.
Example of JSON Data
Consider the following sample JSON data structure, which illustrates common types found in JSON:
{
"name": "John Doe",
"age": 30,
"is_student": false,
"courses": ["Math", "Science"]
}
In this example, we have an object with multiple key-value pairs, showcasing a string, a number, a boolean, and an array.
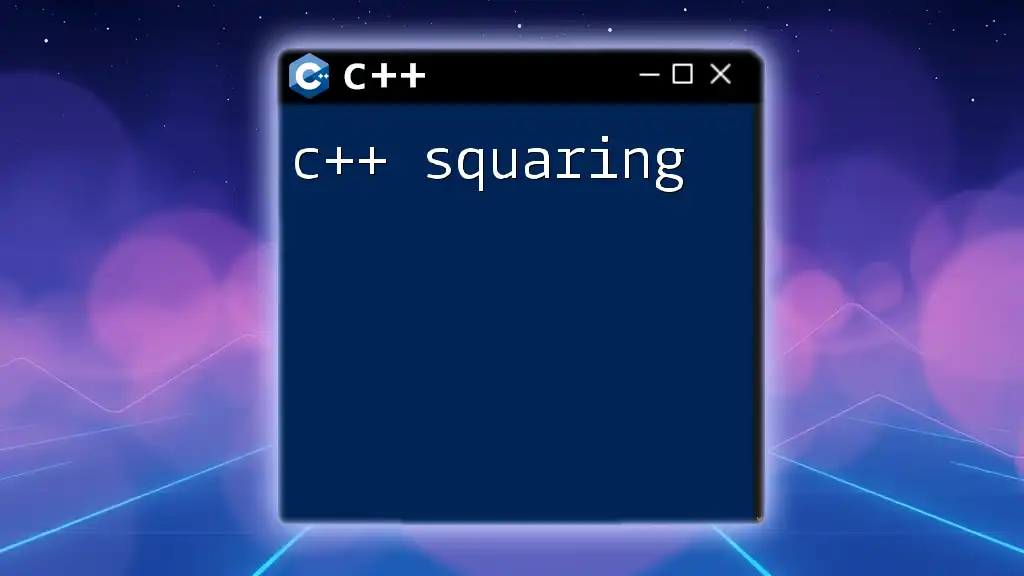
Why Use a JSON Parser for C++?
Advantages of Using a JSON Parser
Utilizing a JSON parser in C++ significantly enhances the ease of handling data formatted in JSON. Here are some key advantages:
- Simplified Data Handling: Parsers abstract away the complexities of manually interpreting JSON strings.
- Prevention of Common Parsing Errors: Libraries throw helpful errors and exceptions when malformed data is encountered, making debugging easier.
- Improved Code Readability: With parsers, your code becomes much more intuitive and maintainable, as they provide logical ways to access and manipulate JSON data.
Common Challenges without JSON Parsers
Without a JSON parser, developers face numerous challenges, especially when dealing with complex, nested data structures. Writing custom parsing logic can lead to frequent errors, making the code less reliable and challenging to maintain.
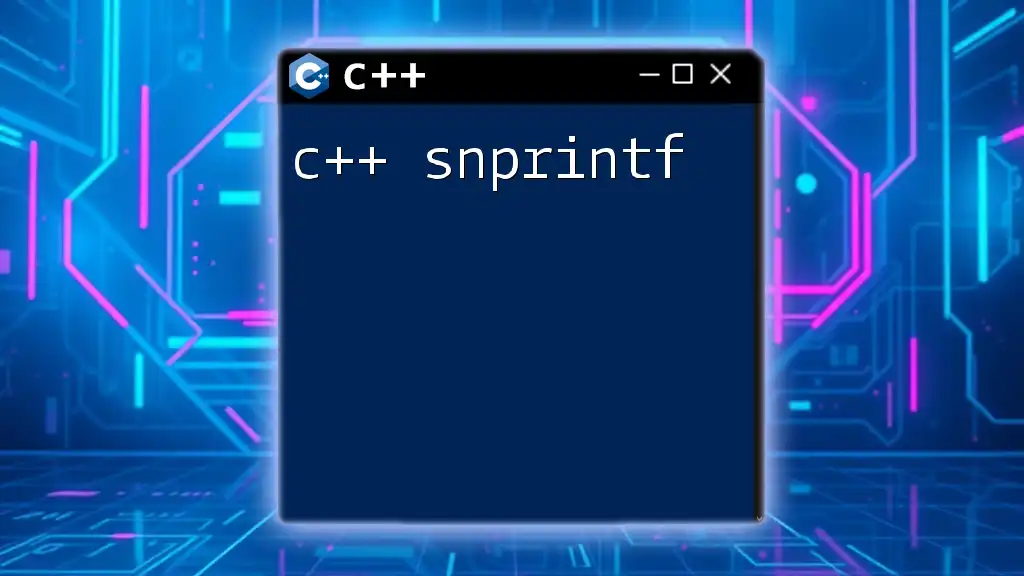
Choosing a C++ JSON Parser Library
Popular JSON Parser Libraries for C++
When it comes to C++ JSON parsing, several libraries stand out due to their performance and ease of use:
-
nlohmann/json: This is arguably the most popular and user-friendly JSON library in C++. It allows for intuitive JSON creation, manipulation, and parsing, closely resembling how native C++ syntax works.
-
RapidJSON: This library is known for its speed and efficiency, making it ideal for applications where performance is critical. It features a powerful DOM-style API as well as SAX-style parsing.
-
jsoncpp: While not as widely adopted as the previous two, jsoncpp remains a solid option for basic JSON functionalities. It offers a straightforward interface for manipulating JSON data.
Installation Instructions
Here’s how you can install the nlohmann/json library with CMake:
git clone https://github.com/nlohmann/json.git
cd json
mkdir build
cd build
cmake ..
make
sudo make install
This will help set up the library for use in your C++ projects.
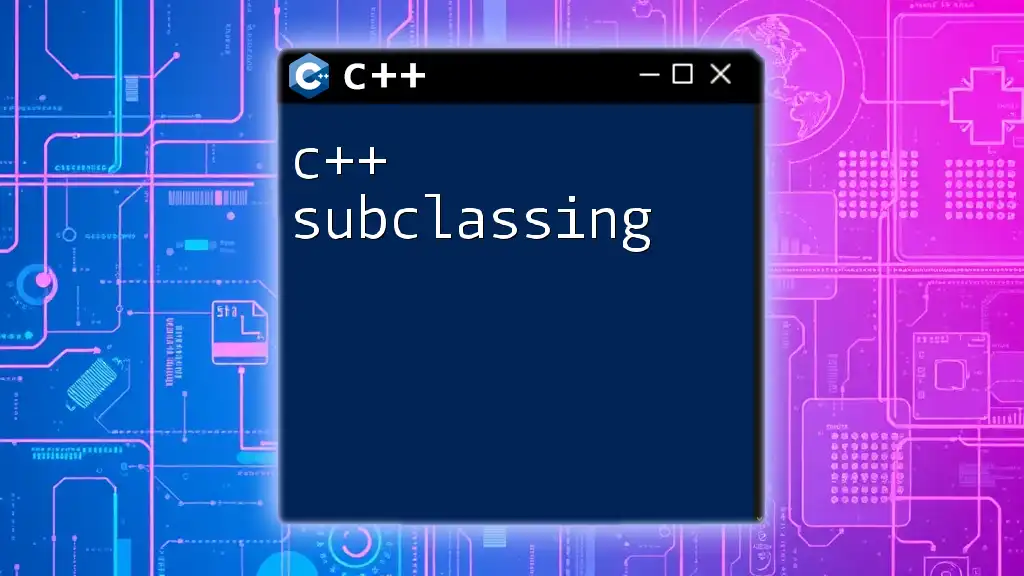
Basic Usage of a C++ JSON Parser
Initializing the JSON Parser
The first step in using a C++ JSON parser is to create and initialize JSON objects. Using the nlohmann/json library, you can do this as follows:
#include <nlohmann/json.hpp>
using json = nlohmann::json;
json j = {
{"name", "John Doe"},
{"age", 30},
{"is_student", false}
};
In this code, we define a JSON object `j` that holds a mix of data types.
Parsing JSON Data
You may also need to parse JSON data from strings. Here’s an example of how to accomplish this:
std::string json_string = "{\"name\":\"Jane Doe\",\"age\":25}";
json parsed_json = json::parse(json_string);
In this snippet, we convert a JSON string into a structured JSON object, making it easy to access its contents.
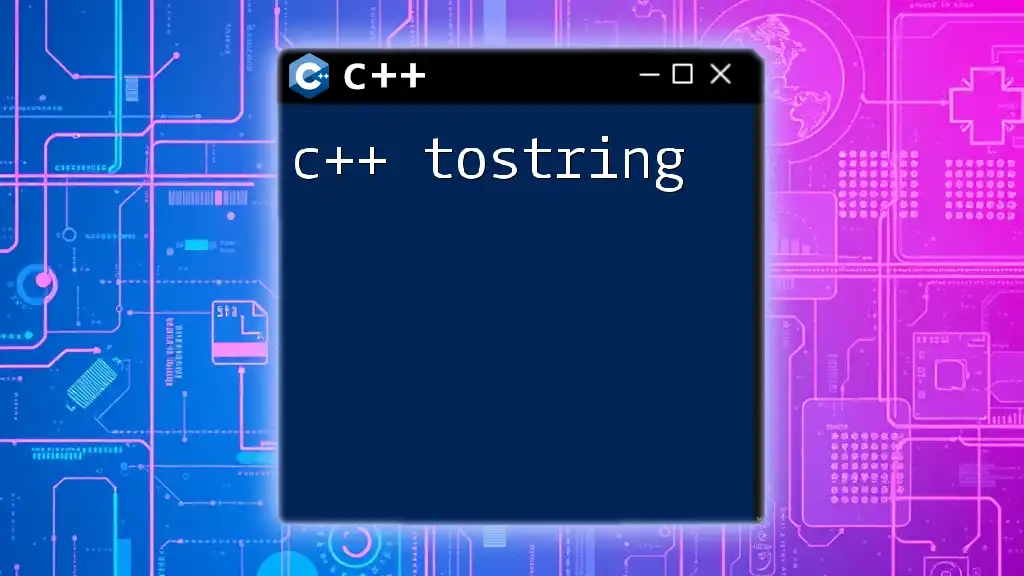
Accessing and Modifying JSON Data
Accessing Values in JSON
Accessing data stored in a JSON object is straightforward. You can retrieve values using their respective keys:
std::string name = parsed_json["name"];
int age = parsed_json["age"];
Here, we are extracting the `name` and `age` from the `parsed_json` object.
Modifying JSON Objects
You can also easily add or update key-value pairs in a JSON object:
parsed_json["courses"] = {"History", "Literature"};
In this example, we add a new array of courses to the existing JSON object.
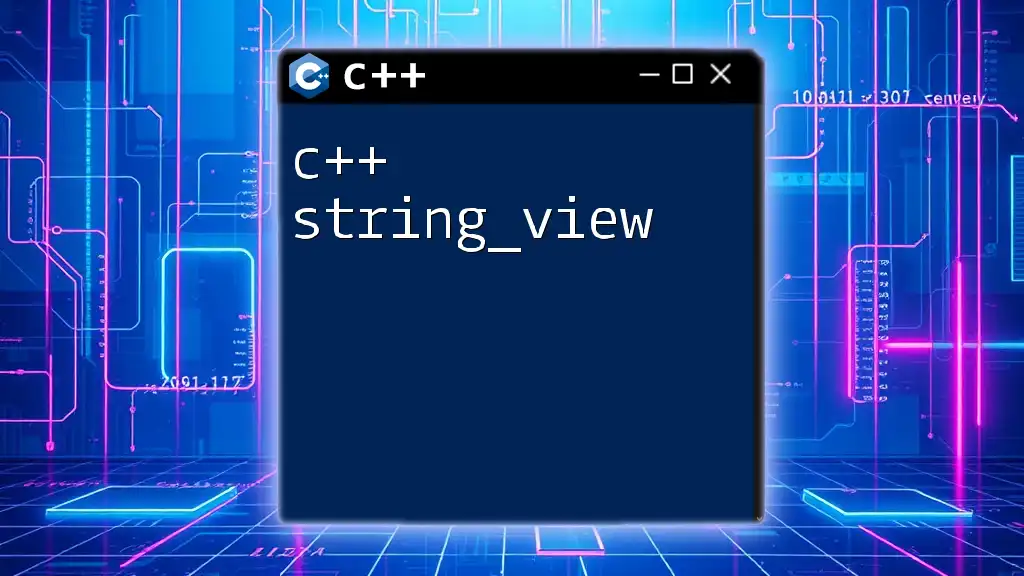
Serializing and Deserializing JSON Data
Serializing JSON to String
When you want to convert a JSON object back to a string representation, you can use the `dump` method:
std::string serialized = parsed_json.dump();
The `serialized` variable will now contain a JSON string that can be saved or transmitted as needed.
Deserializing JSON from File
To read JSON data from a file, you can use the following code:
std::ifstream file("data.json");
json file_json;
file >> file_json;
This code snippet reads JSON data from a file and parses it directly into a JSON object for further manipulation.
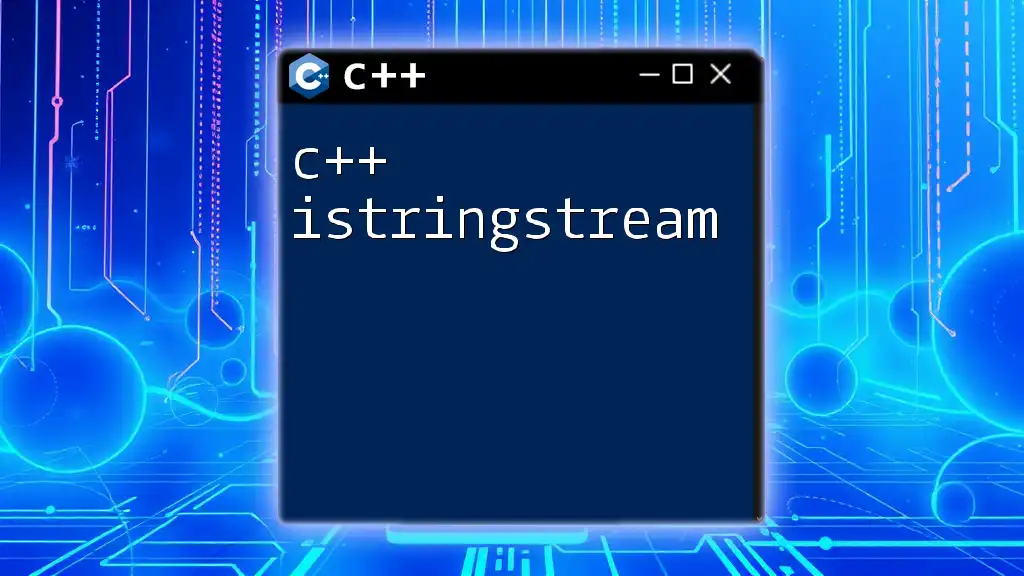
Error Handling in C++ JSON Parsing
Common JSON Parsing Errors and Solutions
Parsing errors may occur when the JSON format is not adhered to. Using the exceptions from the library helps to catch these errors gracefully:
try {
json invalid_json = json::parse("invalid json");
} catch(const json::parse_error& e) {
std::cout << "Parse error: " << e.what() << std::endl;
}
In this example, we attempt to parse an invalid JSON string, catching the `parse_error` and printing an error message if parsing fails.
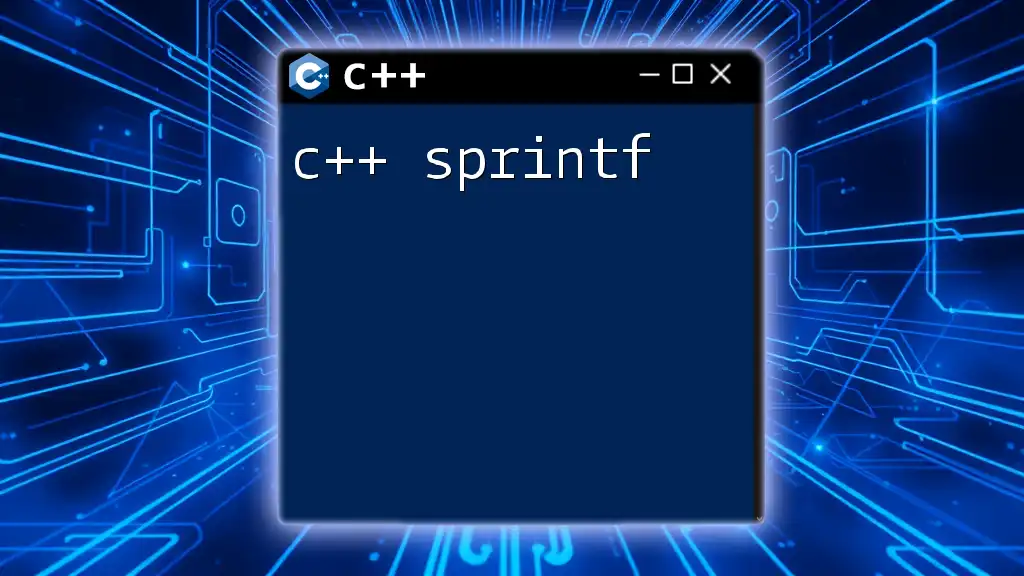
Best Practices for C++ JSON Parsing
Tips for Efficient JSON Handling
To make the most of C++ JSON parsing, consider the following best practices:
- Keep JSON data flat when possible: This minimizes complexity, making your data structures easier to manage.
- Avoid excessive nesting: Deeply nested structures can become cumbersome to navigate and manage.
Performance Considerations
It’s essential to consider performance when choosing a JSON library. RapidJSON, for instance, is particularly well-suited for applications where speed is a priority, while nlohmann/json shines in terms of usability.
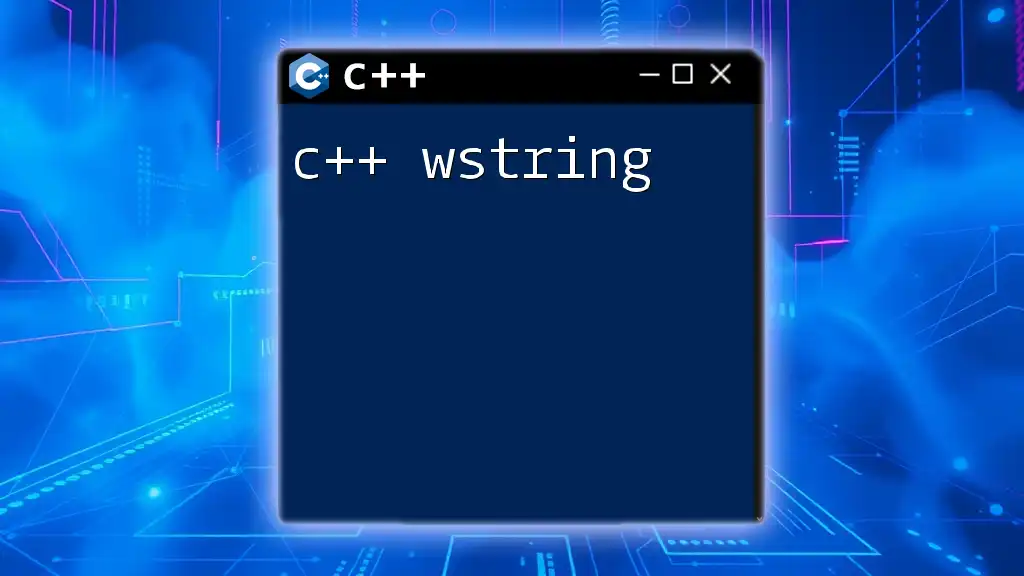
Conclusion
In this guide, we covered the essentials of C++ JSON parsing, highlighting the importance of using a JSON parser library. By providing clear examples and best practices, we have shown how to work effectively with JSON in C++. Embracing these techniques will help simplify your data handling process and enhance the reliability of your applications.
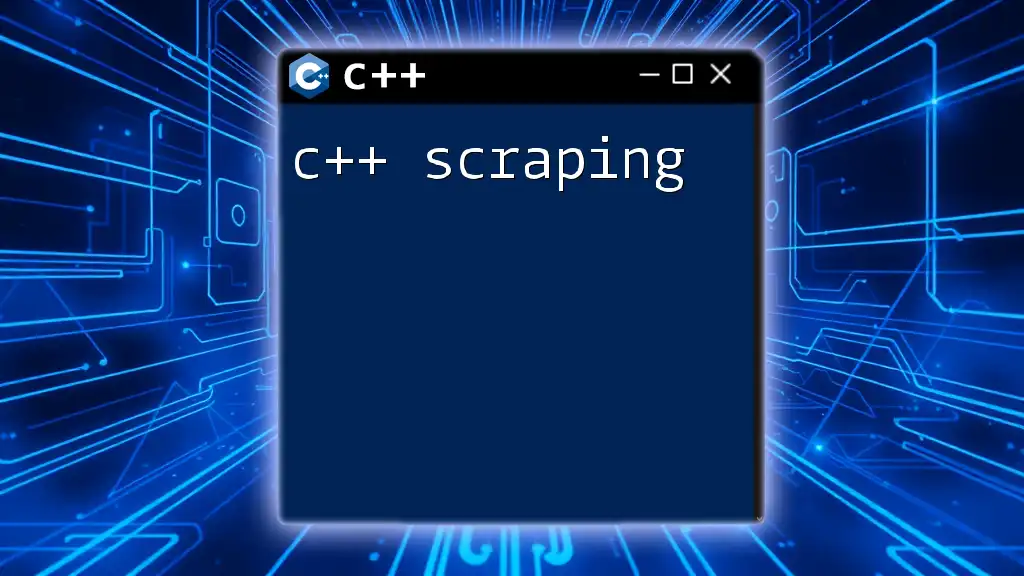
Additional Resources
For further exploration, check the documentation of the libraries discussed and recommended readings to deepen your understanding of C++ JSON parsing techniques.