In C++, `std::wstring` is a wide string type used to store strings composed of wide characters (typically UTF-16) for handling internationalization and supporting a larger character set.
#include <iostream>
#include <string>
int main() {
std::wstring wideStr = L"Hello, World!";
std::wcout << wideStr << std::endl;
return 0;
}
Introduction to `std::wstring`
`std::wstring` is a powerful feature of C++ that deals with wide character strings. Unlike the more commonly used `std::string`, which operates with single-byte characters, `std::wstring` is designed for use with multi-byte and wide character representations. This makes `std::wstring` particularly useful for applications that require character sets beyond ASCII, such as internationalization and localization, where characters might be represented in UTF-16 or UCS-2 encoding.
Differences Between `std::string` and `std::wstring`
The fundamental difference between `std::string` and `std::wstring` lies in their data types. While `std::string` utilizes `char` data types (typically 1 byte per character), `std::wstring` employs the `wchar_t` data type, which typically requires 2 bytes per character. This allows `std::wstring` to encompass an extensive range of characters, accommodating languages such as Chinese, Japanese, and Korean, which include characters that cannot fit into a standard byte.
Importance of Wide Character Strings
In today’s globalized world, software applications must process multiple languages and character sets. Hence, understanding how to manipulate wide strings (`std::wstring`) is crucial for developers who want to ensure their applications run smoothly in diverse cultural contexts.
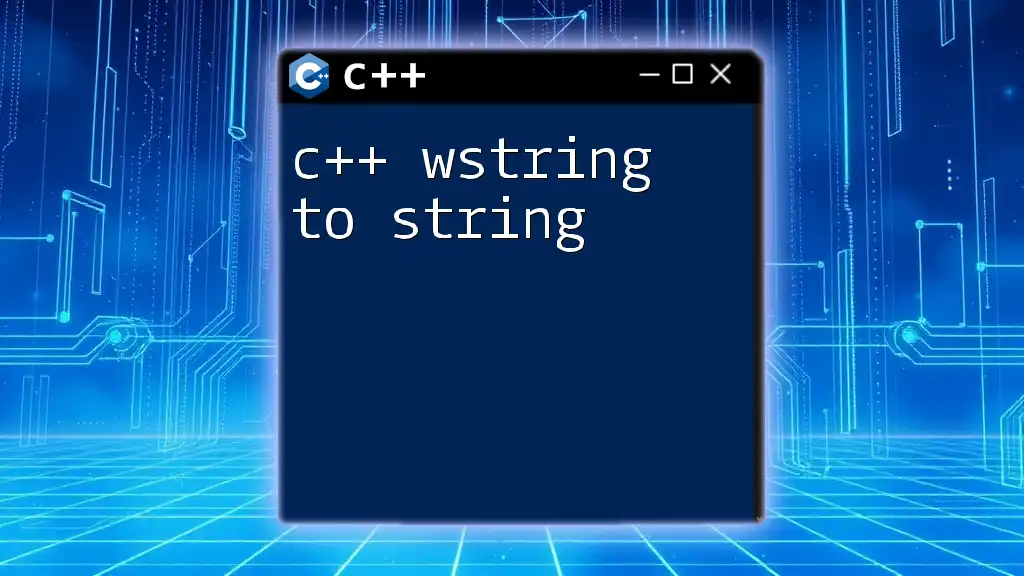
Setting Up the Environment
To begin working with `std::wstring`, you should include the relevant headers in your C++ program. The following header file is necessary:
#include <string>
When developing, ensure your IDE or compiler settings support wide characters. Various IDEs like Visual Studio, Code::Blocks, or even g++ in terminal environments can be configured to handle wide strings.
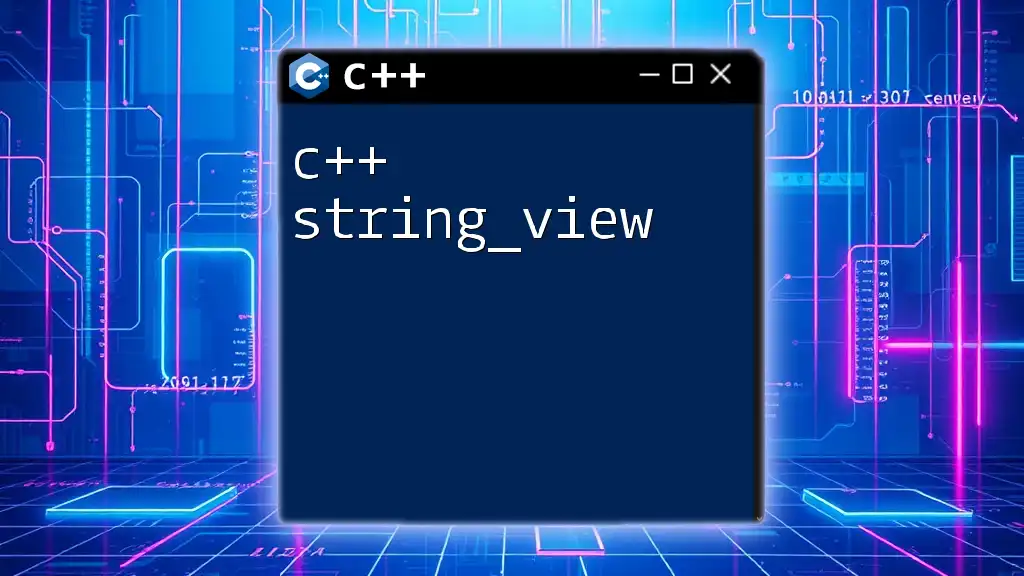
Understanding Wide Character Types
What Are Wide Characters?
Wide characters, represented by `wchar_t`, serve the purpose of enabling the representation of larger character sets. This is particularly relevant in modern programming, where developers are tasked with supporting multiple languages and scripts.
Size Differences
When comparing `char` and `wchar_t`, you'll notice that `char` typically consumes 1 byte while `wchar_t` usually takes up 2 or 4 bytes, depending on the platform. While this may lead to increased memory consumption, the trade-off is the ability to represent a vastly larger array of characters.
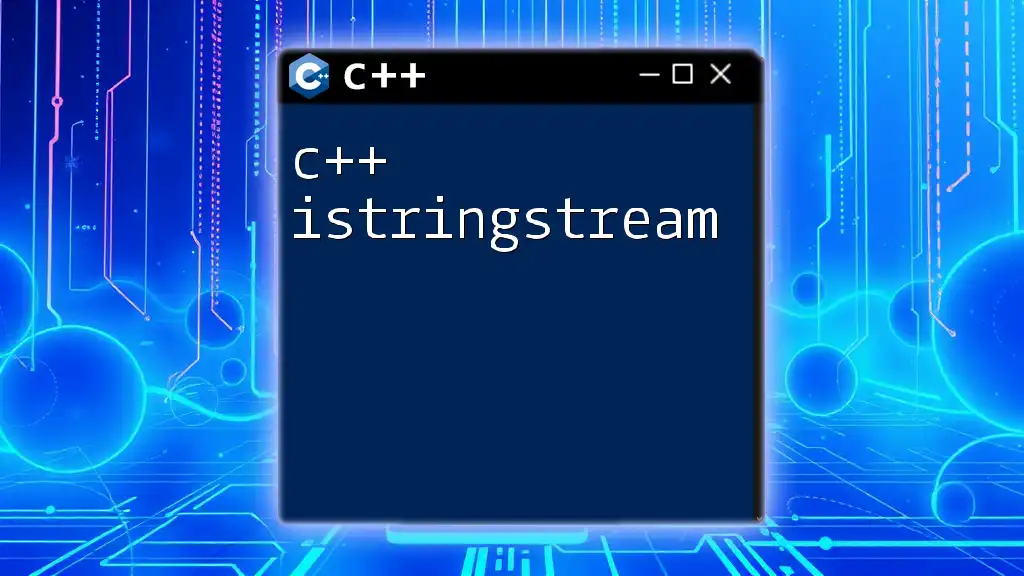
Creating and Initializing `std::wstring`
Default Initialization
Initializing a `std::wstring` without any content sets it to an empty state, meaning:
std::wstring myString;
At this point, `myString` has no characters, and its length is 0.
Initializing with a String Literal
Initializing wide strings requires prefixing string literals with `L`. For example:
std::wstring greeting = L"Hello, World!";
This notation clearly indicates that the string is intended to store wide characters.
Copying and Assigning
Wide strings can be copied and assigned similar to regular strings. You can use the copy constructor:
std::wstring copy = myString;
The assignment operator behaves just as it would for `std::string`:
myString = L"New Value";
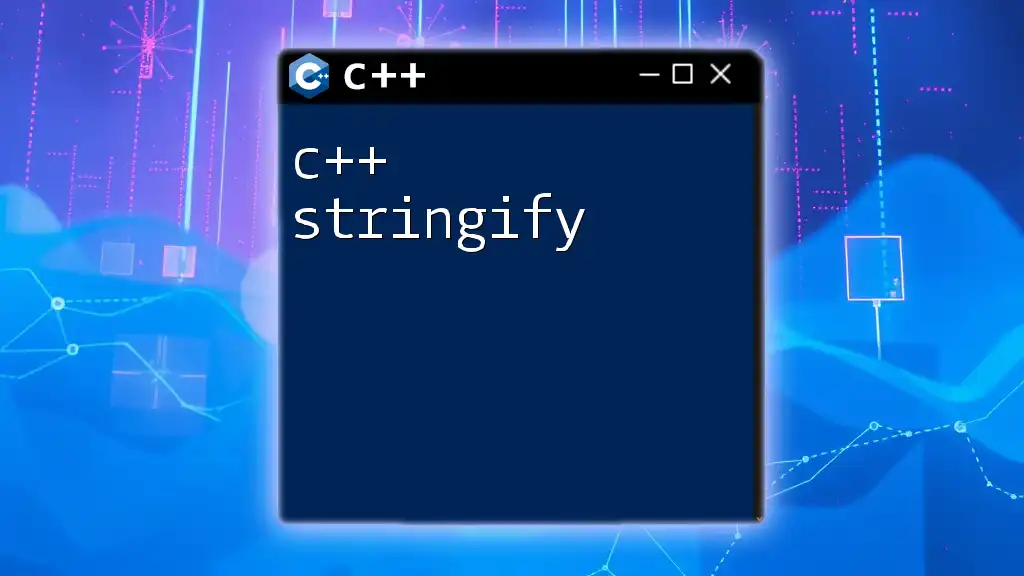
Common Operations on `std::wstring`
Length and Capacity
To check the size of a wide string, use either the `length()` or `size()` methods:
size_t length = myString.length();
Both methods return the same result, indicating the number of wide characters in the string.
Concatenation
You can combine wide strings using the `+` operator:
std::wstring fullString = myString + L" More text.";
Alternatively, the `append()` method is useful for adding content:
myString.append(L" appended text!");
Accessing Characters
The characters within a `std::wstring` can be accessed just like an array. For example:
wchar_t firstChar = myString[0];
To ensure bounds checking, prefer the `at()` method:
wchar_t secondChar = myString.at(1);
Comparison of Wide Strings
Comparing wide strings can be done using typical comparison operators:
if (myString == L"Check this") { /* ... */ }
However, for more nuanced comparisons (like lexicographical order), use the `compare()` method:
int result = myString.compare(L"Another String");
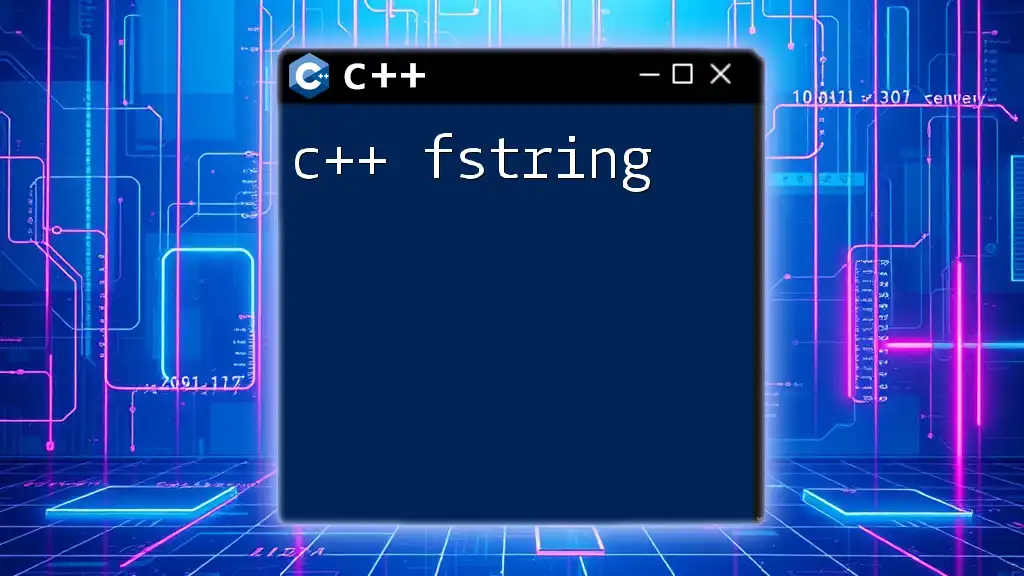
Important Member Functions of `std::wstring`
`find()`
To locate a substring within your wide string, you can use the `find()` method:
size_t pos = myString.find(L"search");
If the substring is found, `pos` will contain the starting index; otherwise, it will return `std::wstring::npos`.
`substr()`
Extracting a portion of a wide string can be done as follows:
std::wstring sub = myString.substr(5, 10);
This retrieves 10 characters starting from the index 5.
`replace()`
To replace a portion of your string, the `replace()` method comes handy:
myString.replace(5, 10, L"replacement");
This will replace 10 characters starting from index 5 with the new substring.
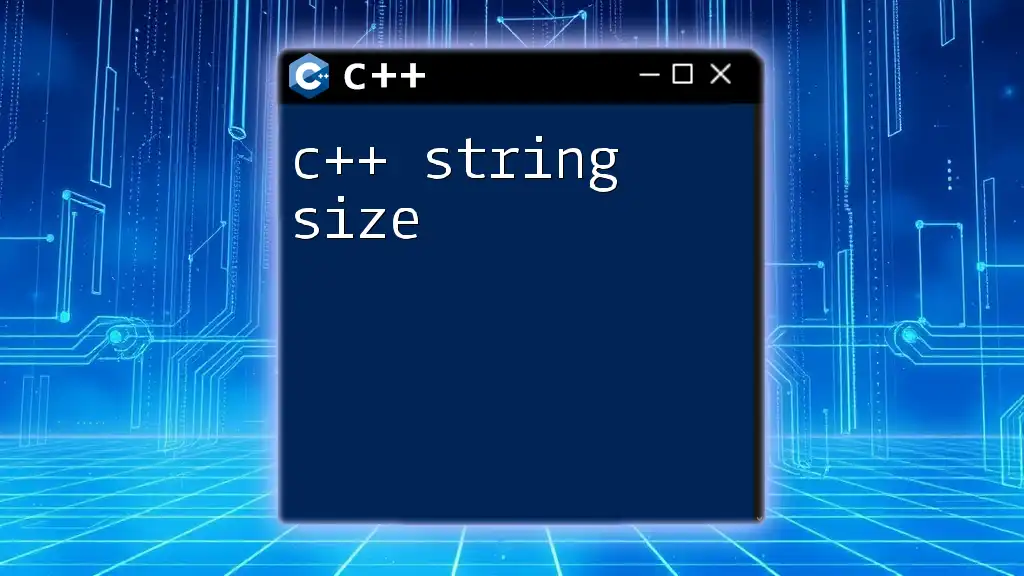
Working with Input/Output
Outputting `std::wstring` to Console
When printing `std::wstring` to the console, utilize `std::wcout`:
std::wcout << myString << std::endl;
This ensures that the wide string is displayed correctly.
Reading from Input
For reading wide strings, you can use `std::wcin`:
std::wcin >> myString;
This allows user input to be directly assigned to the `std::wstring`.
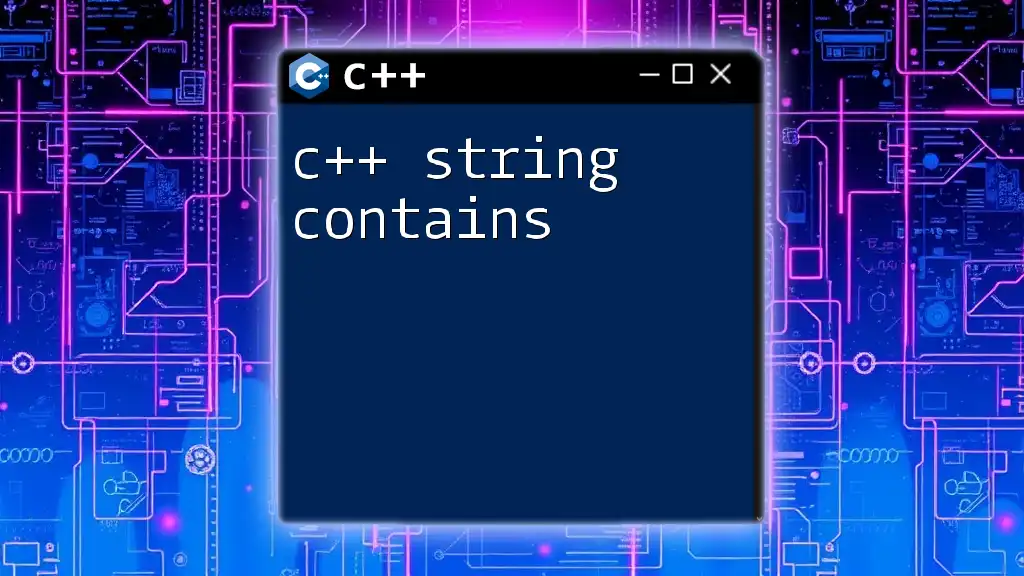
Converting Between `std::string` and `std::wstring`
Converting `std::string` to `std::wstring`
You can convert a narrow string to a wide string using `std::mbstowcs`. Here's a basic example:
std::string str = "Hello";
std::wstring wstr(str.begin(), str.end());
This method takes advantage of the iterator to initialize the wide string.
Converting `std::wstring` to `std::string`
Conversely, to convert a wide string back to a narrow string, you can use `std::wcstombs` as follows:
std::wstring wstr = L"Hello";
std::string str(wstr.begin(), wstr.end());
This is a straightforward way to handle conversion between the two types.
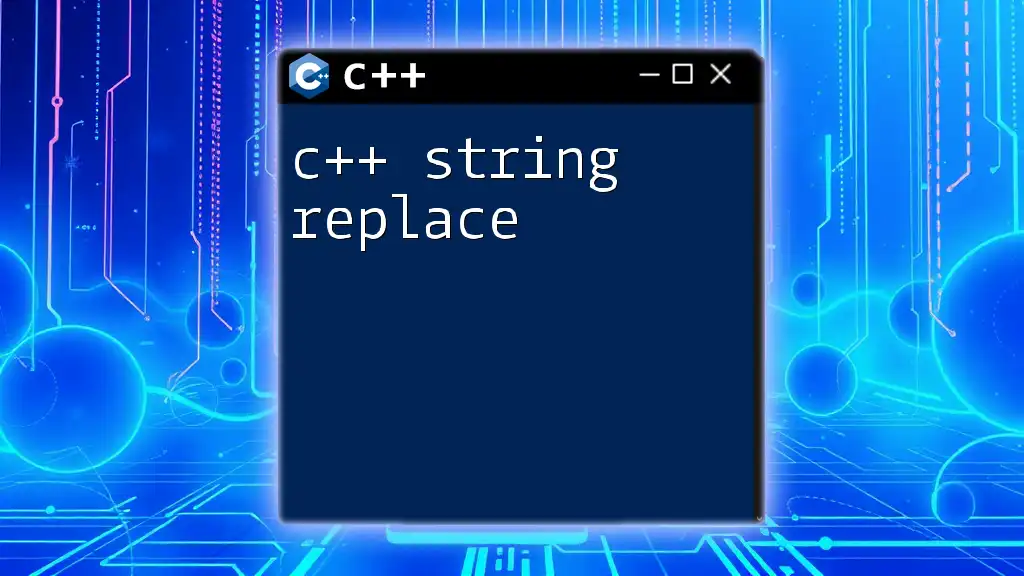
Practical Applications of `std::wstring`
Use Cases in GUI Applications
Wide strings are vital when working with graphical user interface (GUI) libraries like Qt or wxWidgets. These libraries heavily support Unicode and wide characters, making `std::wstring` indispensable for text rendering and user interaction on diverse platforms.
File Handling and Encoding
When dealing with text files in various encodings, `std::wstring` can interact seamlessly with streams capable of handling wide characters. This enhances file operations, ensuring that international text is read and written without data loss.
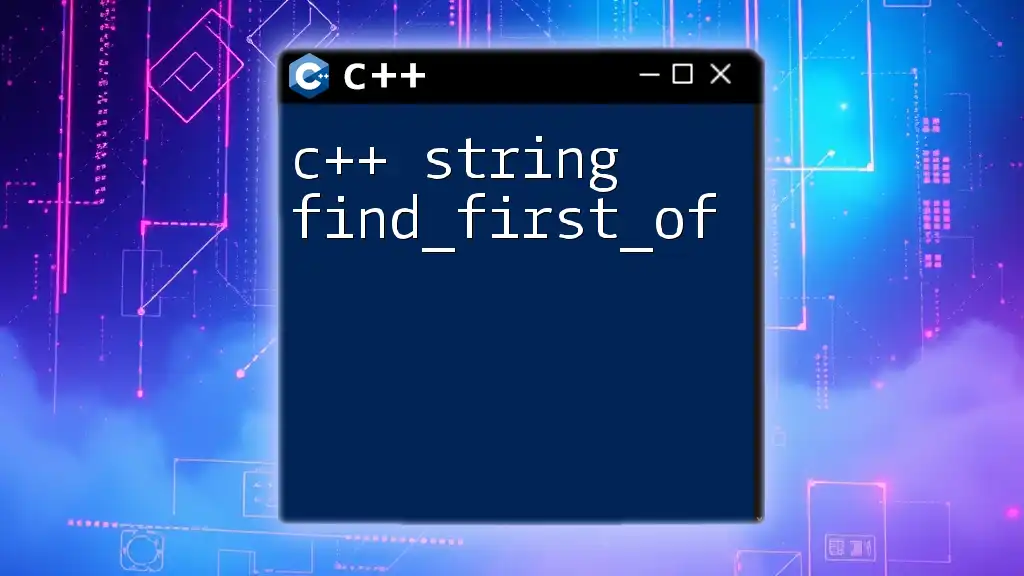
Best Practices for Using `std::wstring`
Memory Management
When dealing with wide strings, it's crucial to manage memory effectively to avoid leaks. Ensure proper usage of scopes and initialization to guarantee that resources are released appropriately. Consider utilizing move semantics when applicable to enhance performance when transferring ownership of wide strings.
Performance Tips
While `std::wstring` provides many benefits, it is essential to evaluate whether your application truly requires it. If you are dealing solely with ASCII characters, `std::string` may offer better performance. Understanding the context of character use is vital in making this decision.
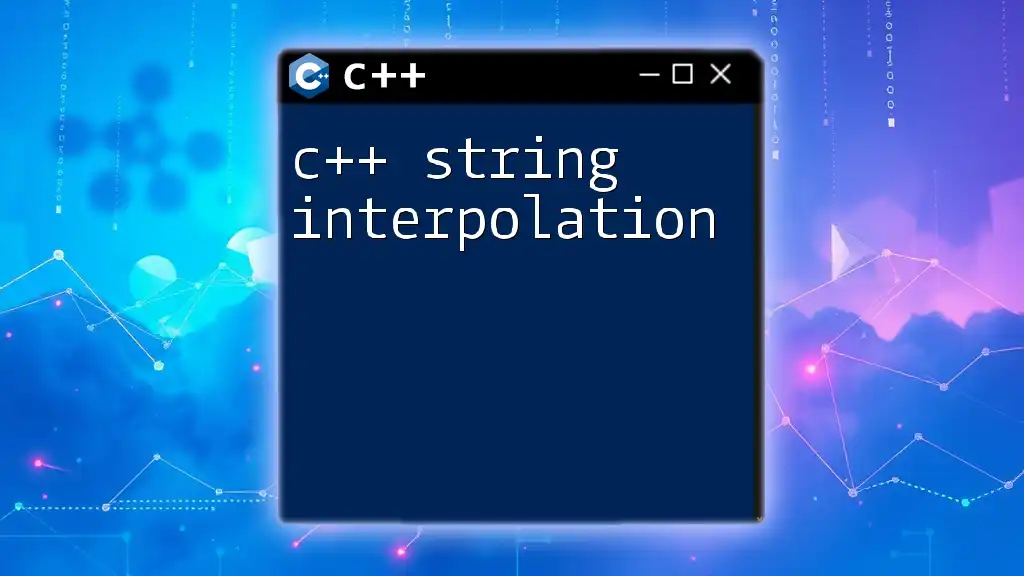
Conclusion
Understanding and utilizing `std::wstring` is crucial for modern C++ applications that require handling wide character sets. With its rich set of functions and compatibility with international text, `std::wstring` opens up robust solutions for various software development needs. By practicing the code examples and leveraging this guide, you’ll be well on your way to mastering wide strings in C++.