To convert a `std::wstring` to a `std::string` in C++, you can use the `std::wstring_convert` class along with a `std::codecvt_utf8<wchar_t>` facet for handling wide characters.
#include <string>
#include <codecvt>
#include <locale>
std::wstring wstr = L"Hello, World!";
std::wstring_convert<std::codecvt_utf8<wchar_t>> converter;
std::string str = converter.to_bytes(wstr);
What is wstring?
Definition of wstring
In C++, `wstring` is a wide-character string type that stores characters in a UTF-16 format. It is designed to handle larger character sets, which makes it possible to use non-ASCII characters effectively. This is particularly important when developing applications that need to support multiple languages or character symbols, ensuring that all characters are represented accurately.
Creating a wstring
Creating a `wstring` is straightforward. You can do so using the `L` prefix to indicate that the string is wide. Here’s an example:
#include <string>
std::wstring myWideStr = L"Hello, Wide World!";
In this example, `myWideStr` is initialized with a wide string that contains the phrase "Hello, Wide World!". The `L` prefix signifies that the string literals are wide-character strings.
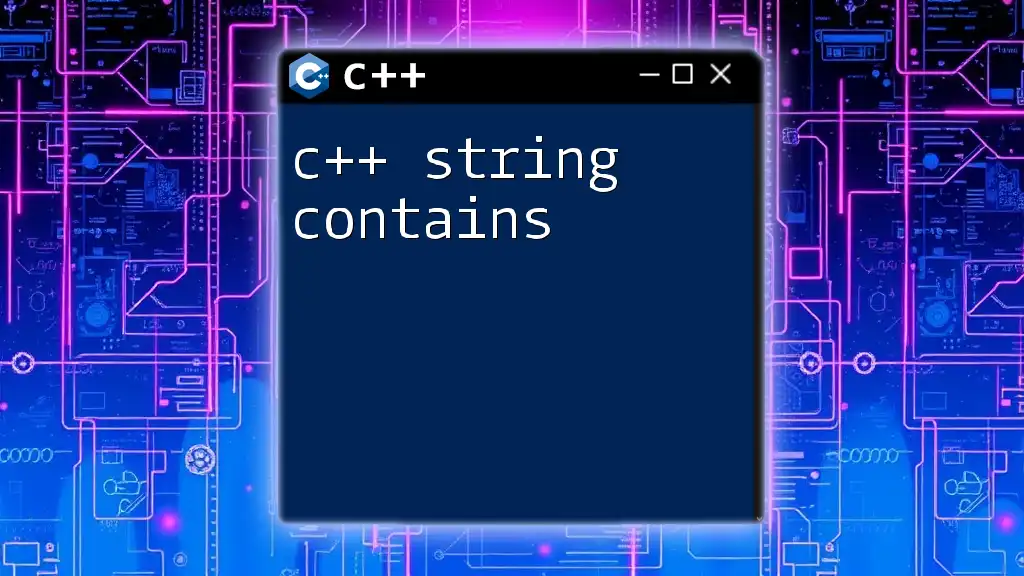
What is string?
Definition of string
The `string` type in C++ is the standard string representation used for handling text. It utilizes a single-byte character set (usually ASCII or UTF-8, depending on the implementation) and is suitable for most textual data handling needs. This makes `string` easy to use for general-purpose string manipulation and is often employed in user interfaces and file handling.
Creating a string
A `string` can be created using standard syntax without needing a prefix. Here’s how you can initialize it:
#include <string>
std::string myStr = "Hello, World!";
In this case, `myStr` is a typical C++ string that contains the text "Hello, World!".
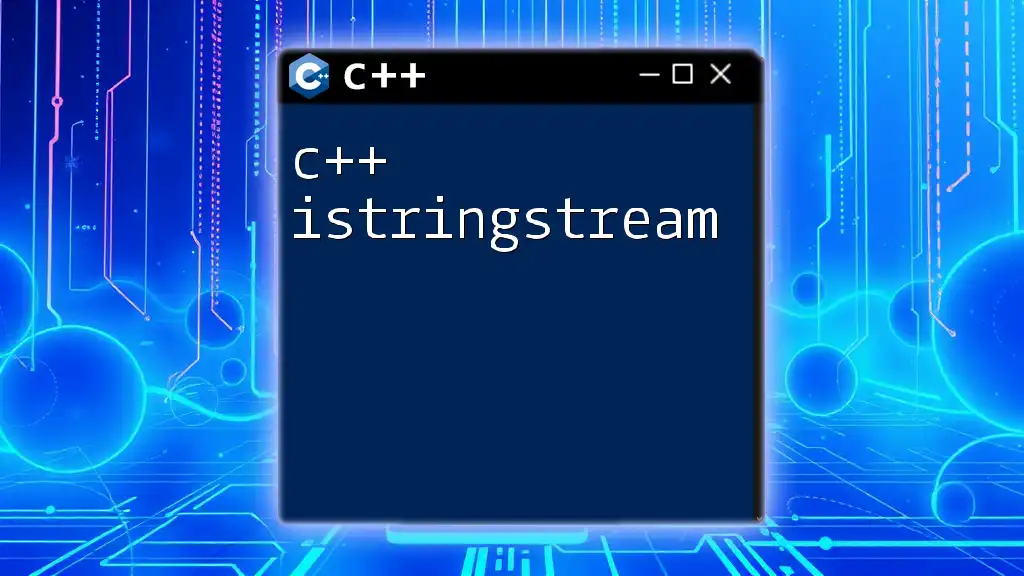
Key Differences Between wstring and string
Character Representation
One of the most significant differences between `wstring` and `string` is how they represent characters. A `string` typically uses single-byte characters, making it suitable for English and other Western languages. In contrast, `wstring` uses wide characters (usually two or four bytes) to accommodate characters from various languages, enabling developers to work with a broader range of inputs effectively.
Memory Consumption
Memory usage is another area where `wstring` and `string` differ. `wstring` consumes more memory per character, which can lead to enhanced memory requirements when dealing with large volumes of text data. Consequently, while `wstring` is more versatile for internationalization, it may lead to performance overheads in memory-limited applications.
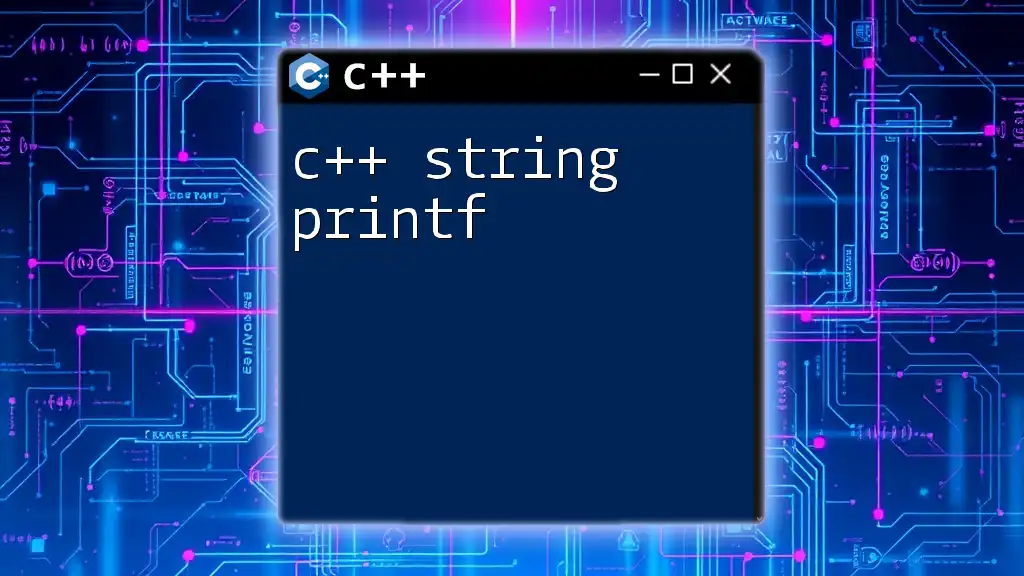
Converting wstring to string
Why Convert wstring to string?
The conversion from `wstring` to `string` is often essential in C++ applications where functions and libraries are designed to work with `string` rather than `wstring`. When dealing with display, file output, or interfacing with APIs that require standard strings, converting `wstring` to `string` becomes necessary. However, developers must pay attention to character encoding to ensure that no information is lost during this process.
Methods to Convert wstring to string
Using C++ STL
One of the simplest ways to convert a `wstring` to a `string` is by leveraging the built-in STL constructs. This method utilizes the `std::string` constructor, which can take iterators as arguments.
std::wstring wideStr = L"Hello, World!";
std::string narrowStr(wideStr.begin(), wideStr.end());
This code snippet converts `wideStr` into `narrowStr` by constructing a new `string` from the range provided by `wideStr`. It should be noted that this method only works effectively for ASCII characters; non-ASCII characters may not be represented correctly, leading to data loss.
Using Widechar to Multi-char Conversion
For scenarios where precise character encoding is critical, using the `wcstombs` function can be a better approach. This function converts a wide-character string to a multibyte character string, considering the current locale.
#include <cwchar>
#include <cstdlib>
std::wstring wideStr = L"Hello, World!";
char narrowStr[50];
wcstombs(narrowStr, wideStr.c_str(), sizeof(narrowStr));
In this example, `wcstombs` converts the `wideStr` to a standard `char` array. This method also allows for better handling of character sets, but developers should always check the return value to detect conversion errors.
Using std::wstring_convert (C++11 and above)
C++11 introduced `std::wstring_convert`, which simplifies the conversion between `wstring` and `string`. Utilizing codecs makes it flexible regarding various character types.
#include <locale>
#include <codecvt>
std::wstring_convert<std::codecvt_utf8<wchar_t>> converter;
std::string narrowStr = converter.to_bytes(wideStr);
In this code example, `to_bytes` is invoked on the `converter` object, converting the `wstring` to a `string`. It ensures that the conversion process adheres to UTF-8 encoding. However, it is essential to note that `std::wstring_convert` has been marked for deprecation in later versions of C++, and developers should consider alternative libraries like `Boost.Locale` or C++20 text transformation functions.
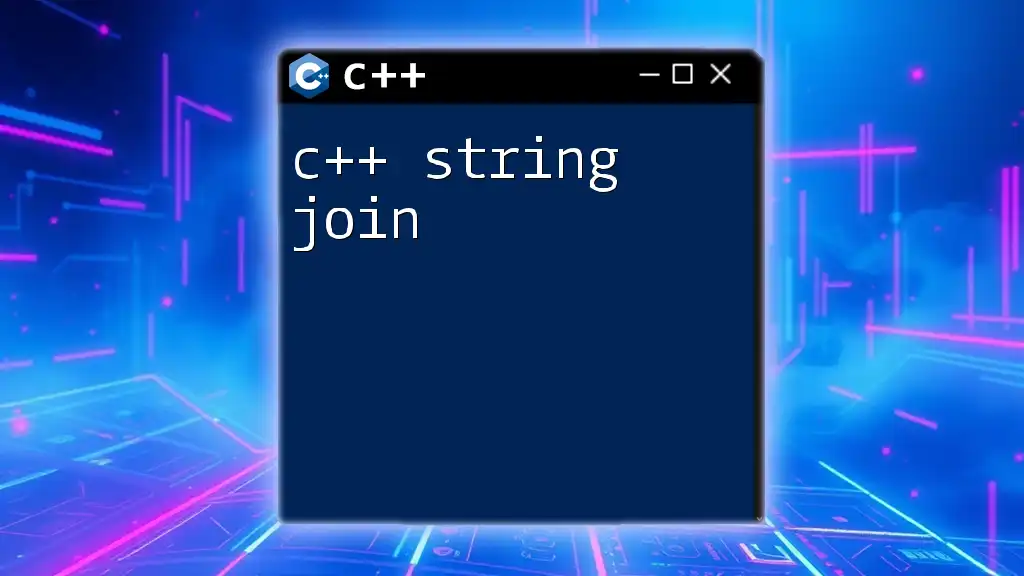
Handling Encoding Issues
Common Pitfalls During Conversion
While converting `wstring` to `string`, developers may face several pitfalls, primarily related to character mismatching. Non-ASCII characters may not be encoded correctly in `string`, resulting in corrupted output or lost data.
Troubleshooting Conversion Problems
To solve conversion issues, always check the locale settings of your application. Use functions like `setlocale` to ensure that your environment is appropriately configured to handle multilingual character sets. Common error messages during conversion often relate to buffer overflows or misconfigured locales; understanding and diagnosing these issues is critical for robust applications.
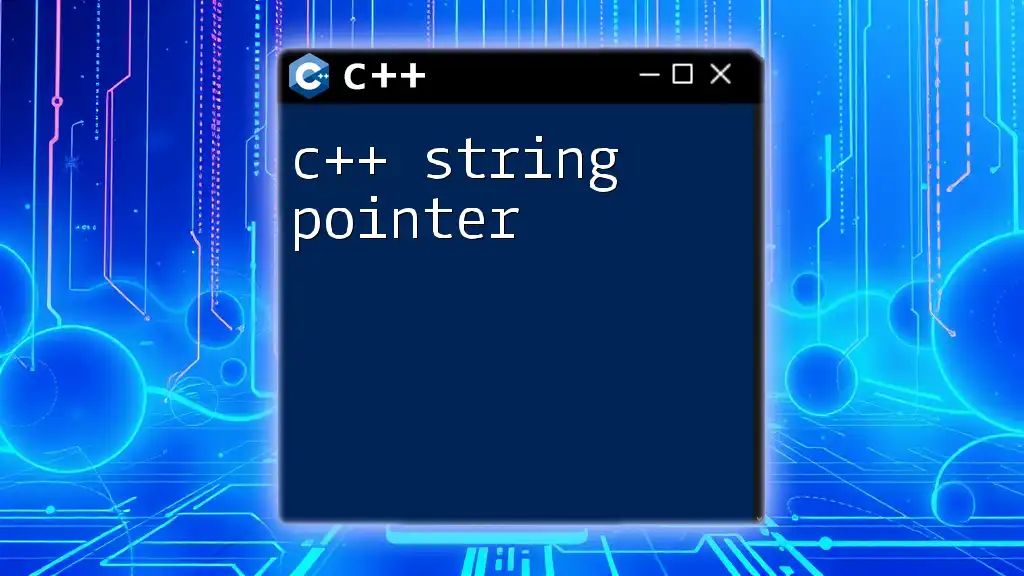
Performance Considerations
Impact of Conversion on Performance
Converting between `wstring` and `string` can impact performance, especially with large strings. Frequent conversions can lead to unnecessary memory allocations and management overhead, causing scalability concerns in performance-sensitive applications.
Best Practices for Managing wstring and string
To optimize string handling, consider these best practices:
- Use `string` when you know your application will handle only ASCII characters.
- Reserve space in your strings when you know the expected length of text to avoid reallocations.
- Limit conversions between `string` and `wstring` to minimize performance overhead.
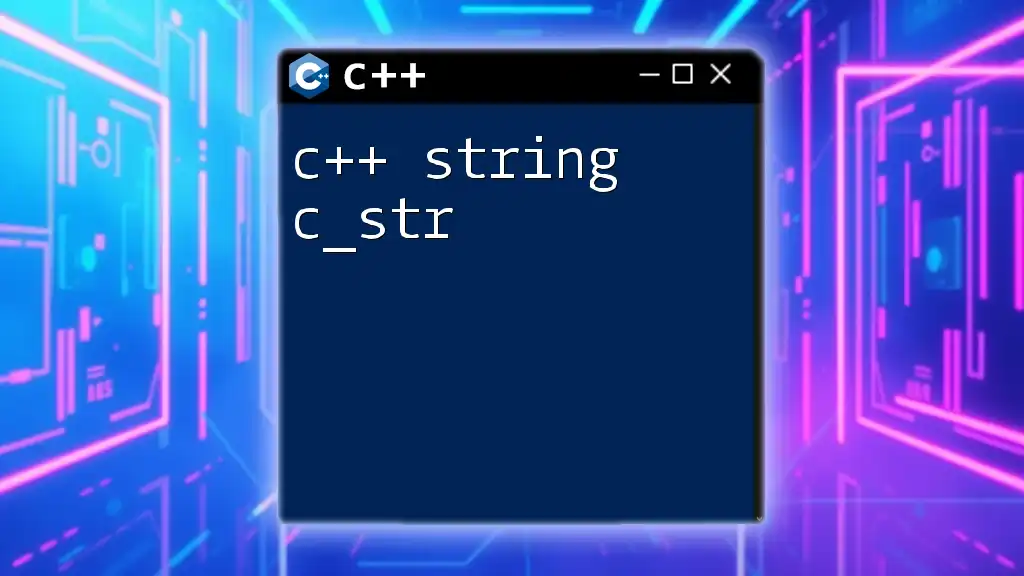
Conclusion
Understanding the transformation of C++ `wstring` to `string` is crucial for building versatile applications that cater to various language requirements. By employing efficient conversion methods, handling encoding issues, and keeping an eye on performance, developers can ensure that their C++ applications can interact seamlessly across different character sets.
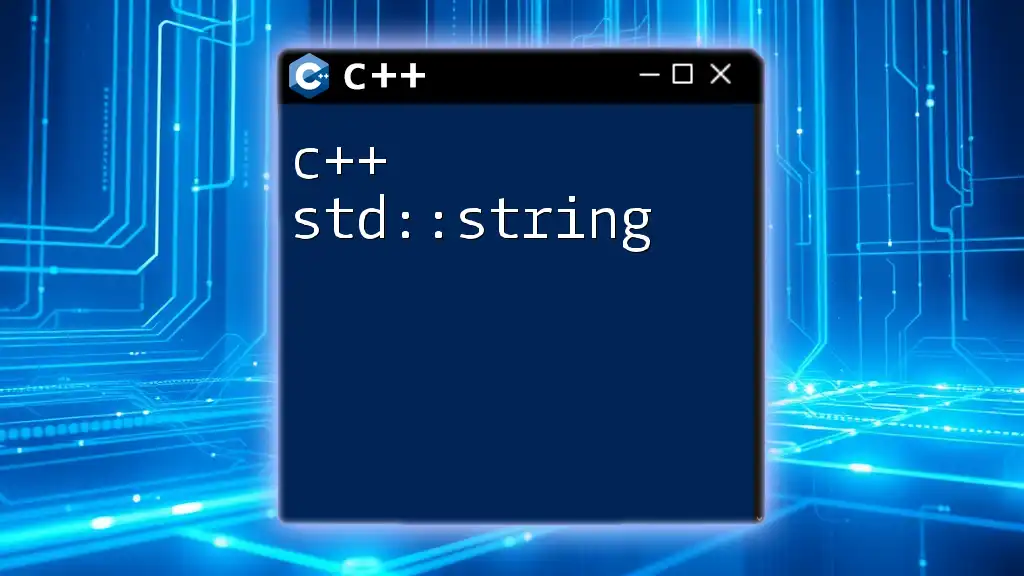
Additional Resources
For those interested in expanding their knowledge, several valuable resources exist:
- C++ Standard Library documentation for string handling and character types.
- Online communities such as Stack Overflow for real-time questions and support.
- Books and articles focusing on C++ development practices.
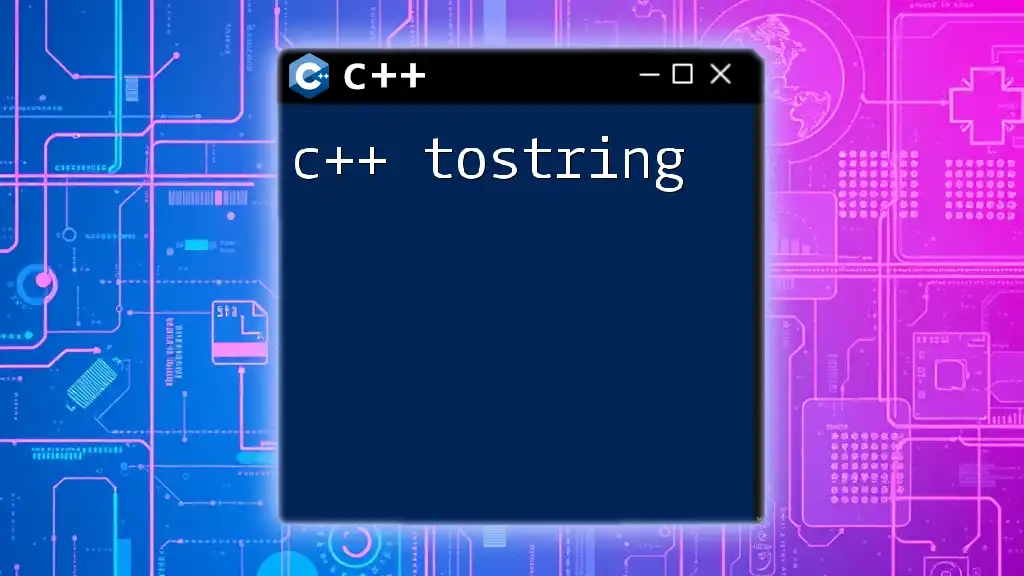
Frequently Asked Questions (FAQ)
How do I directly compare a wstring with a string?
Directly comparing a `wstring` with a `string` requires both to be of the same type. Convert one to the other before comparison.
Can I mix wstring and string in the same application?
While you can mix `wstring` and `string`, doing so can introduce complications. Consider using type-safe conversions to minimize errors.
What are the best libraries for handling strings in C++?
Some popular libraries for advanced string handling include `Boost.String` and `ICU (International Components for Unicode)`, both offering powerful internationalization capabilities.