In C++, the `printf` function can be used to format and print strings to the console, similar to how it's used in C, utilizing format specifiers to control the output.
Here's a code snippet demonstrating its use:
#include <cstdio>
int main() {
const char* name = "Alice";
int age = 30;
printf("Name: %s, Age: %d\n", name, age);
return 0;
}
Understanding the printf Function
What is printf?
The `printf` function is a core component inherited from the C programming language, widely used for formatted output. Its syntax consists of a format string followed by a variable number of arguments that match the format specifiers within the string. The versatility of `printf` allows for the integration of various data types into a single formatted output line, enabling programmers to create tailored console messages.
How printf Works with C++ Strings
In C++, there are primarily two ways to represent strings: C-style strings, which are arrays of characters terminated by a null character, and `std::string`, a part of the C++ Standard Library that offers more functionality and ease of use compared to C-style strings.
When using `printf`, developers must be cautious, as `printf` functions expect C-style strings. Hence, a seamless conversion or proper type handling becomes essential when dealing with C++ `std::string` objects.
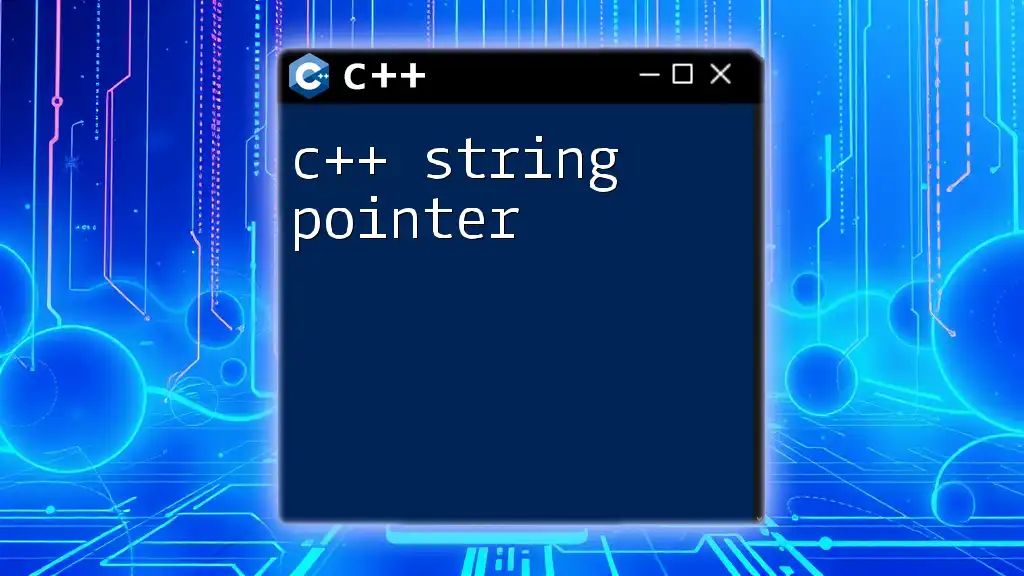
C++ printf String Basics
Using printf with C-Style Strings
C-style strings can be directly passed to the `printf` function. Here’s a basic example demonstrating its usage:
#include <cstdio>
int main() {
const char* name = "World";
printf("Hello, %s!\n", name);
return 0;
}
This example illustrates how you can declare a C-style string and pass it to `printf`. The `%s` format specifier tells `printf` to expect a string and substitute it appropriately in the output.
Transitioning to C++ Strings
Using `std::string` in conjunction with `printf` is not direct since `printf` doesn't accept `std::string` as an argument. Instead, you can convert a C++ string to a C-style string with the `.c_str()` method. This function returns a pointer to a null-terminated character array, making it possible to seamlessly integrate `std::string` with `printf`:
#include <iostream>
#include <string>
#include <cstdio>
int main() {
std::string name = "World";
printf("Hello, %s!\n", name.c_str());
return 0;
}
This code snippet illustrates how to convert a C++ `std::string` into a format that `printf` can handle, ensuring that the output is formatted correctly.
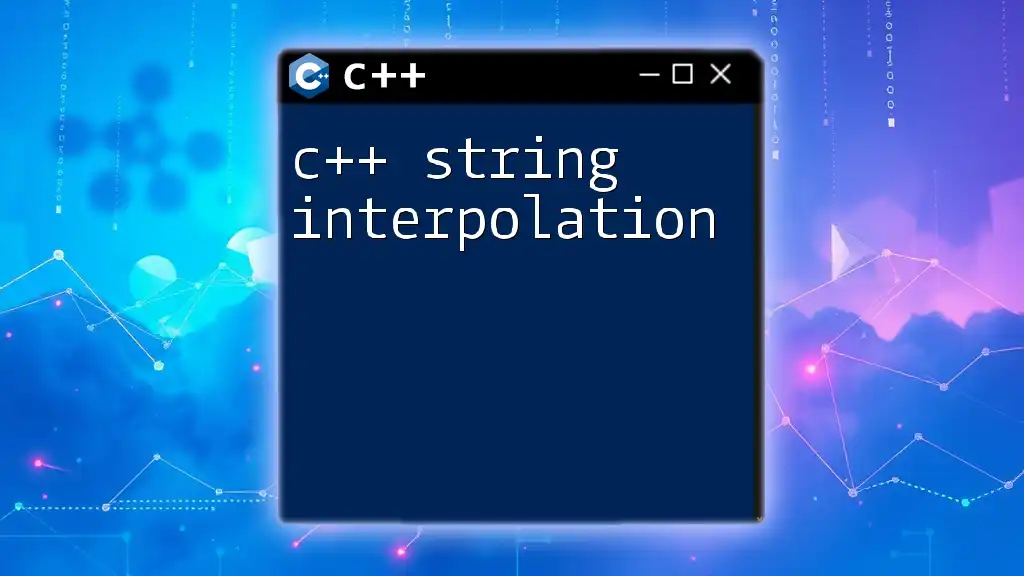
Formatting C++ Strings with printf
Converting std::string to C-Style Strings
The `.c_str()` method is integral when you want to utilize `printf` with `std::string`. When calling `.c_str()`, you must ensure that the lifetime of the `std::string` object remains valid throughout the execution of the `printf` function to avoid unexpected behavior or access violations.
Variable Argument Lists and printf
The `printf` function supports a variable number of arguments, allowing for diverse output formatting. Understanding the various format specifiers is key to effective usage. Here’s a breakdown of some common format specifiers:
- %s: For strings
- %d: For decimal integers
- %f: For floating-point numbers
- %x: For hexadecimal representation
These specifiers enable the creation of sophisticated and informative output messages. Here’s an example using several specifiers:
#include <cstdio>
#include <string>
int main() {
std::string firstName = "John";
std::string lastName = "Doe";
int age = 30;
float height = 5.9;
printf("Name: %s %s, Age: %d, Height: %.1f ft\n", firstName.c_str(), lastName.c_str(), age, height);
return 0;
}
This example illustrates how to combine different data types in one formatted output statement, ensuring clarity and precision.
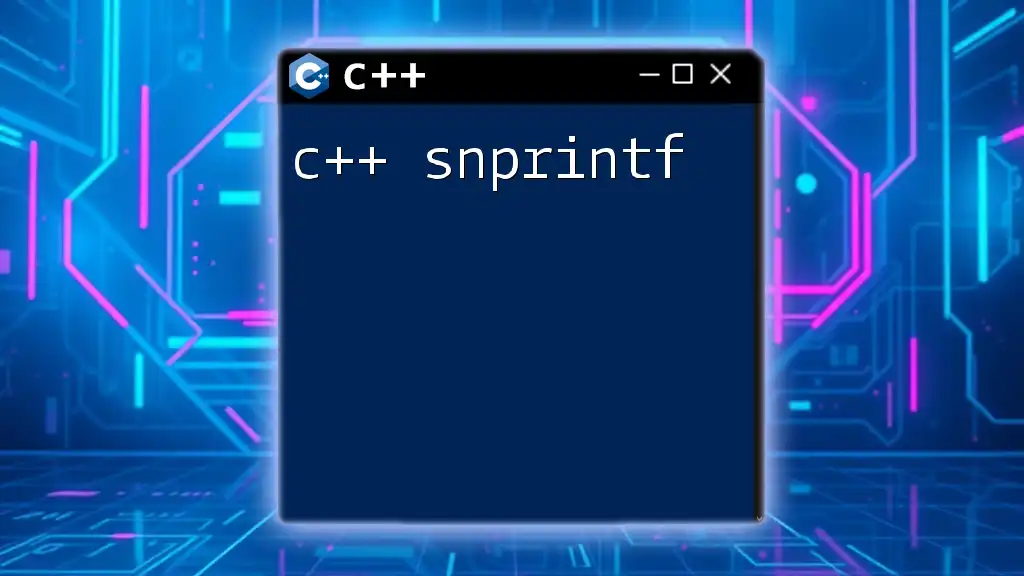
Advanced C++ String Formatting Techniques
Combining Multiple Strings
Utilizing `printf` seamlessly with multiple strings can enhance the clarity of outputs. When dealing with various types of data, it's important to remember to convert each non-C-style string to the proper format. The example provided showcases how multiple strings can be formatted in a single `printf` statement, enhancing readability:
#include <cstdio>
#include <string>
int main() {
std::string firstName = "John";
std::string lastName = "Doe";
printf("Name: %s %s\n", firstName.c_str(), lastName.c_str());
return 0;
}
Applying Format Specifiers
When formatting output, understanding and using format specifiers correctly is crucial. Each specifier serves a unique purpose. For instance:
- `%d` is used for decimal integers with automatic formatting.
- `%f` offers floating-point precision and can be modified to display a specific number of decimal places.
- `%x` converts integers into hexadecimal format.
This knowledge allows you to portray data precisely as needed, adapting to the needs of your output with ease.
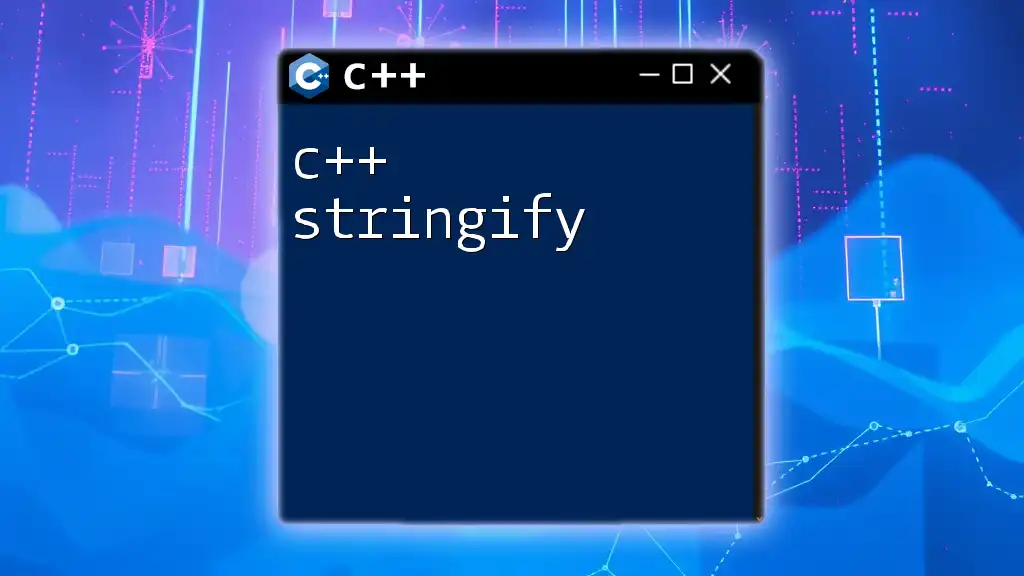
Best Practices for Using printf with C++ Strings
Avoiding Common Pitfalls
When working with `printf`, be cautious of memory issues, especially when handling C-style strings. Passing a pointer to a string that goes out of scope can lead to undefined behavior. Moreover, since `printf` is not type-safe, mismatched format specifiers can lead to runtime errors.
When to Use printf vs. std::cout
While `printf` provides flexibility, C++'s `std::cout` offers type safety and better integration with C++ features. For example, using `std::cout` with `std::string` doesn't require conversion and is more idiomatic in C++:
#include <iostream>
#include <string>
int main() {
std::string name = "World";
std::cout << "Hello, " << name << "!" << std::endl;
return 0;
}
Consider using `std::cout` for scenarios where type safety and C++ features are priorities, reserving `printf` for cases requiring specific format control or when replicating legacy C code.
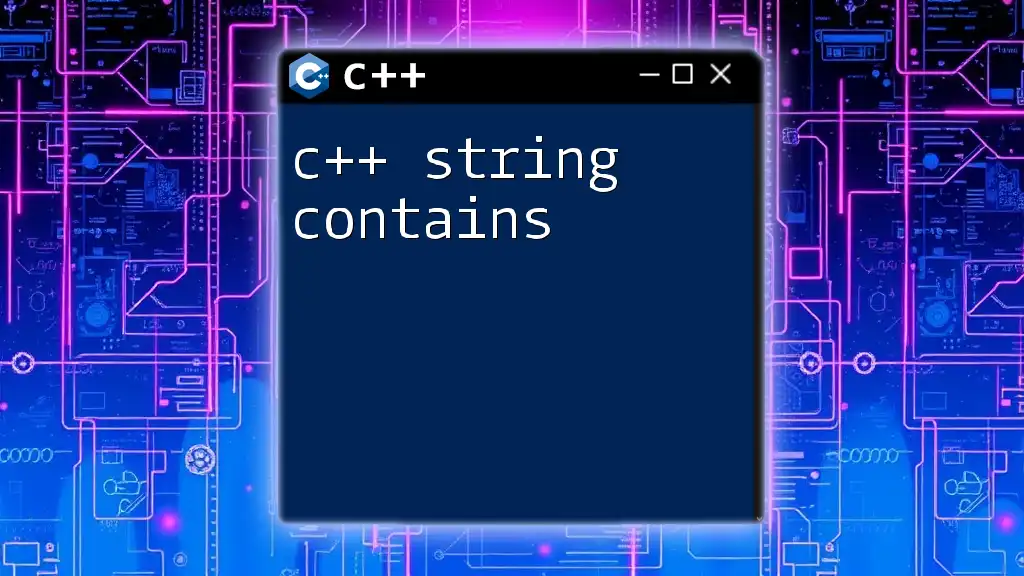
Conclusion
In summary, understanding how to effectively utilize the `printf` function with C++ strings can significantly enhance your ability to output formatted data. By mastering the conversion methods, format specifiers, and best practices, you can approach string formatting with confidence in your C++ programming endeavors. Always remember the distinctions between C-string handling and `std::string` to ensure seamless integration and to maximize the potential of formatted output in your applications.