A C++ string builder allows efficient concatenation of strings, reducing the overhead associated with repeated string additions by utilizing `std::ostringstream`.
Here's a simple example of using a string builder in C++:
#include <iostream>
#include <sstream>
int main() {
std::ostringstream stringBuilder;
stringBuilder << "Hello, " << "world!" << " This is a string builder example.";
std::string result = stringBuilder.str();
std::cout << result << std::endl;
return 0;
}
Understanding C++ String Builder
The Need for a String Builder in C++
In C++, string manipulation often involves concatenating multiple strings together. This can lead to performance issues, especially when the size of the strings or the number of operations grows. Each time you concatenate a string using `std::string`, a new memory allocation may occur, which is inefficient. A C++ string builder addresses this by allowing for a more scalable and efficient way to handle dynamic strings.
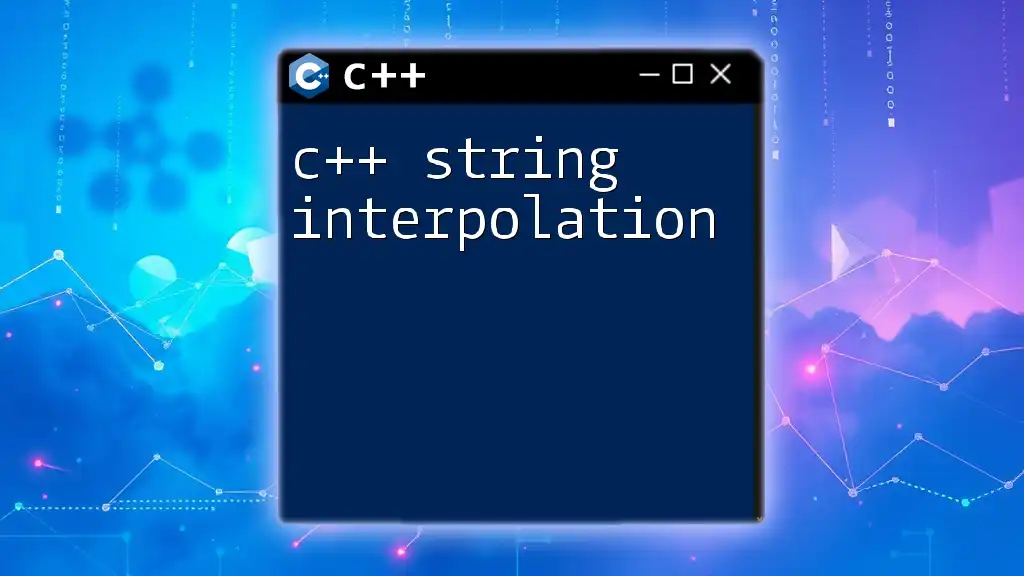
The Basics of Strings in C++
Standard String Class Overview
The `std::string` class in C++ is a powerful and versatile way to work with strings. It provides various functionalities, such as basic string manipulation, handling character arrays, and offering rich APIs for searching, comparing, and formatting. However, when it comes to building complex strings, `std::string` can fall short.
Limitations of `std::string`
Even though `std::string` simplifies many tasks, it is not immune to issues, particularly when concatenating strings repetitively. The typical method of using `+=` or `append` creates temporary copies of the strings, leading to excessive memory allocations. This can severely degrade performance in performance-critical applications.
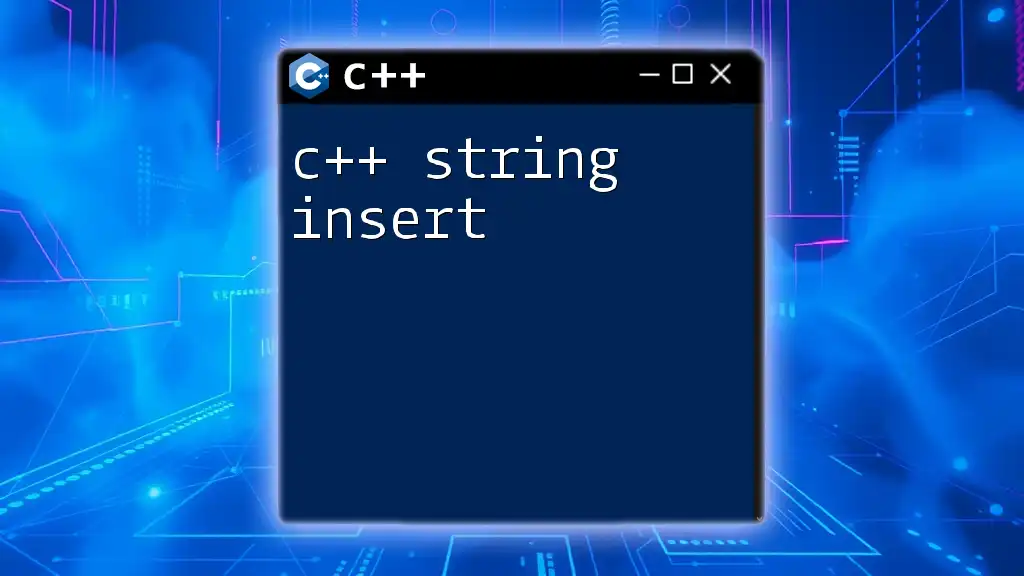
Creating a Simple C++ String Builder
Basic Structure of a String Builder Class
To create a `C++ string builder`, we first need to define a class that will store the strings internally. This class will contain essential member variables, such as a string buffer and its capacity.
class StringBuilder {
private:
std::string buffer;
public:
// Constructor, methods, etc.
};
Important Member Functions
Appending Strings
One of the primary functions of a string builder is appending strings. Here, we can implement an `append` method that efficiently adds strings to the buffer without repeatedly reallocating memory.
void append(const std::string& str) {
buffer += str;
}
This method uses `+=`, but within a controlled context, reducing the number of reallocations significantly.
Clearing the Buffer
Another important function is `clear()`, which allows for the memory used by the buffer to be freed when it is no longer needed.
void clear() {
buffer.clear();
}
Clearing the buffer is essential for memory management and can prevent memory leaks in long-running applications.
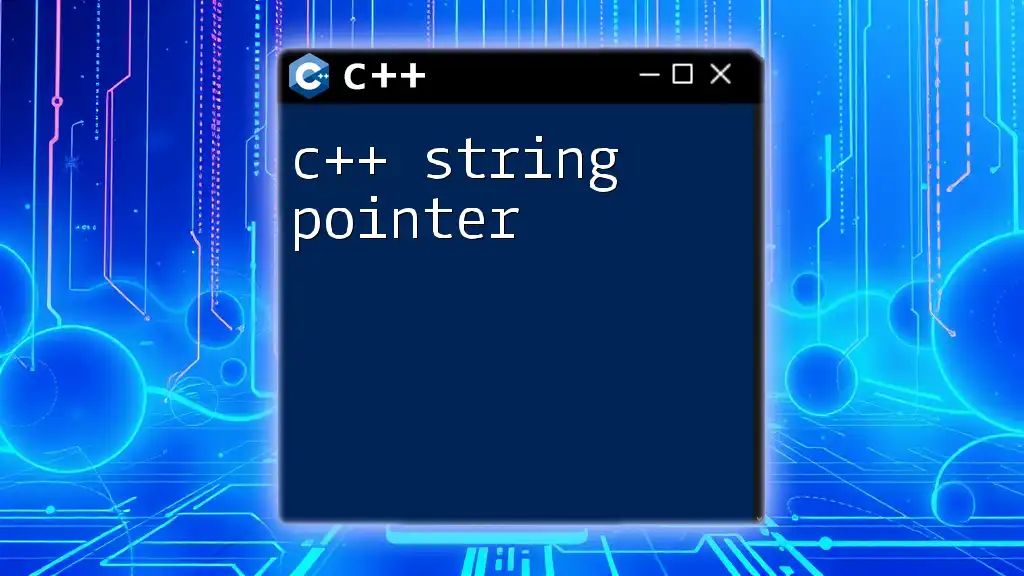
Advanced String Builder Functionality
Insert Functionality
In addition to appending, the string builder should allow for inserting strings at specific positions in the buffer.
void insert(size_t index, const std::string& str) {
buffer.insert(index, str);
}
This method expands the capabilities of our string builder, making it more versatile for various use cases.
Formatting Strings
A string builder can also incorporate methods for formatting strings. Here, we can leverage `std::ostringstream` to format strings that need to incorporate different data types.
void format(const std::string& str, int value) {
std::ostringstream oss;
oss << str << value;
buffer += oss.str();
}
This allows developers to build complex strings easily, integrating different data types in a seamless manner.
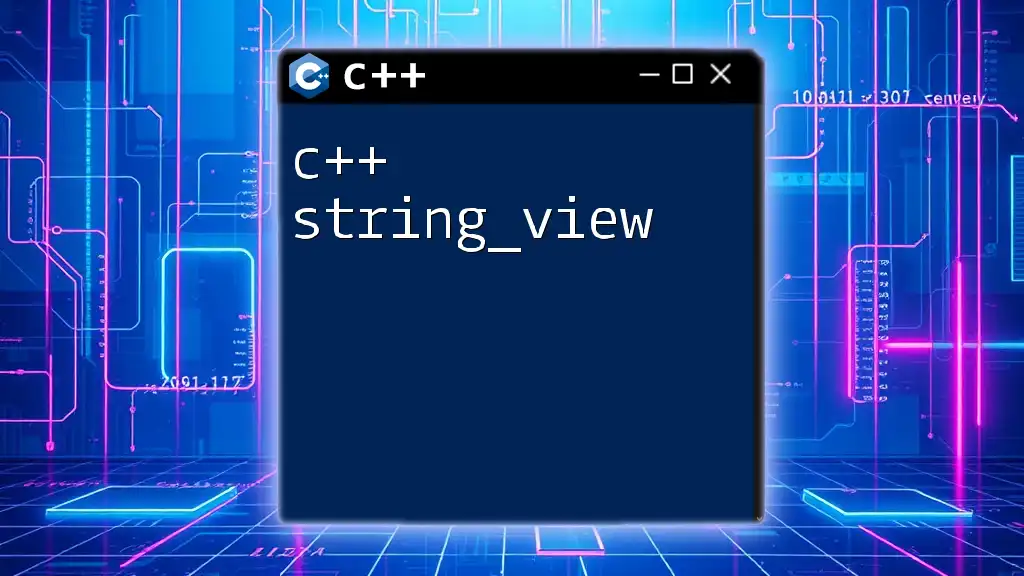
Performance Considerations
Advantages of Using a String Builder
The primary advantage of utilizing a string builder is the efficiency it brings. By minimizing memory reallocation and copying, we can significantly enhance performance, especially when dealing with very large strings or numerous concatenation operations.
Performance Tips
It is essential to recognize when to prefer a string builder over `std::string`. In scenarios involving heavy string concatenation within loops or in performance-sensitive applications, a string builder stands out as the superior choice. Benchmarks have shown that a well-implemented string builder can outperform standard string concatenation practices markedly.
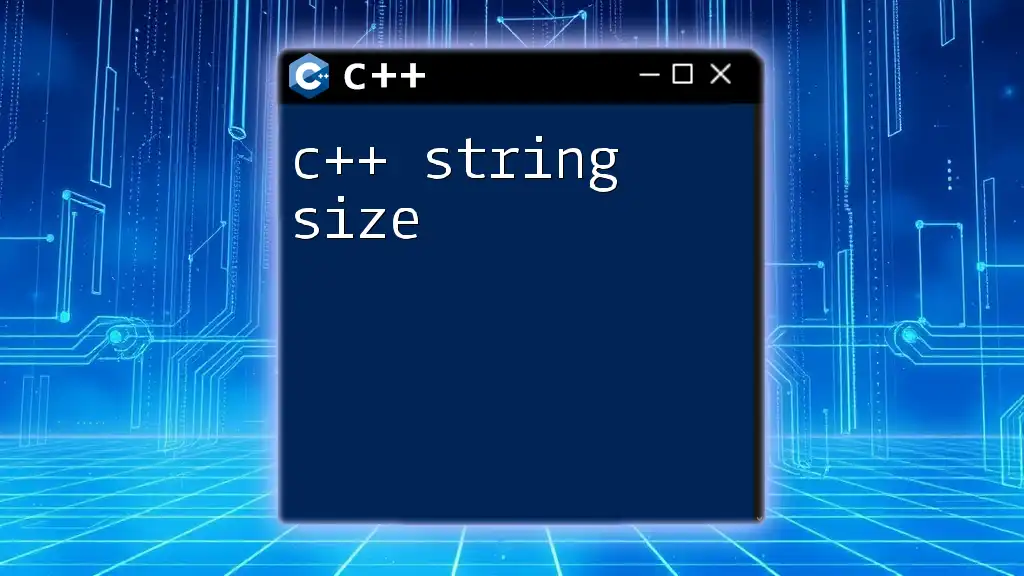
Practical Usage of C++ String Builder
Common Scenarios for String Builders
A string builder is particularly useful in constructing dynamic strings, such as generating HTML outputs, building complex log messages, or assembling database queries.
For instance, consider constructing a SQL query string:
StringBuilder query;
query.append("SELECT * FROM users WHERE age > ");
query.append(std::to_string(minAge));
This example illustrates how a string builder can simplify creating statements dynamically, enhancing both readability and reliability.
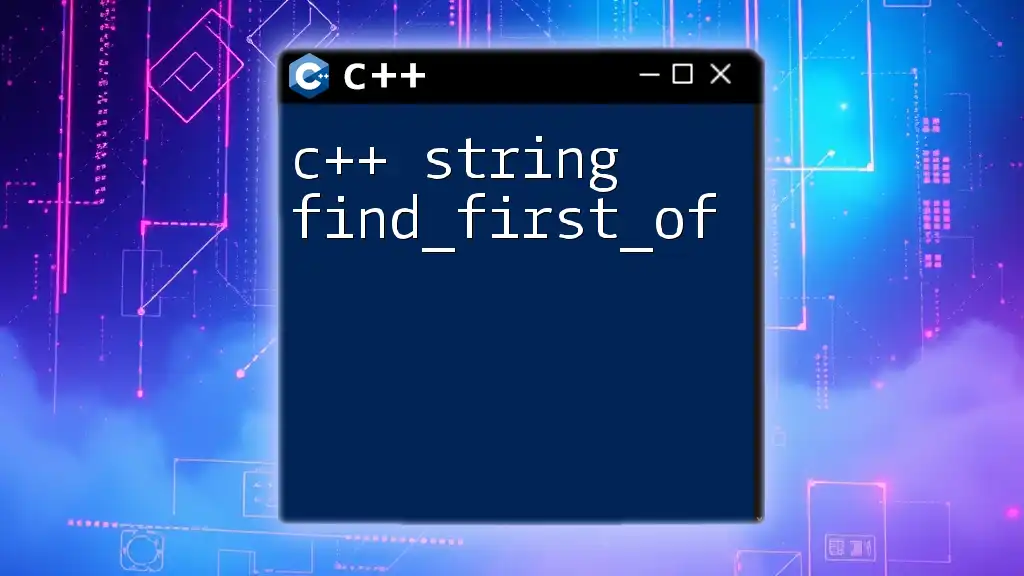
Comparing StringBuilder in C++ with Other Languages
String Builder in Java vs. C++
While C++ does not have a dedicated string builder class in the standard library, Java provides a built-in `StringBuilder` class for similar purposes. Java's string manipulation often defaults to its StringBuilder, which manages a mutable sequence of characters. The core difference lies in memory management—C++ developers have to manually handle memory, while Java automates this.
Other Languages with Similar Concepts
Many programming languages incorporate their own variants of string builders, including Python with its `str.join()` method or C#’s `StringBuilder` class. Each language has its unique approach, yet they all aim to improve the efficiency of string manipulations.
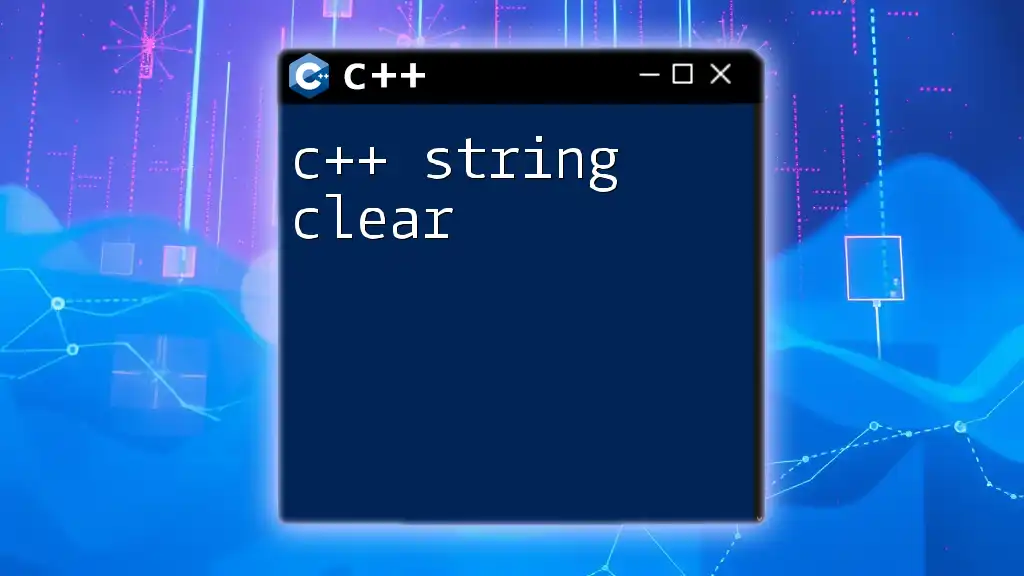
Conclusion
In conclusion, the C++ string builder is an invaluable tool that enhances performance and readability when dealing with strings. By understanding its capabilities and scenarios for use, developers can significantly improve their applications' efficiency. Implementing best practices, such as utilizing a string builder in performance-critical applications, can lead to a more elegant solution for string handling in C++. As you explore C++ string manipulation further, consider the principles outlined here to ensure you are employing the right tools for the task at hand.
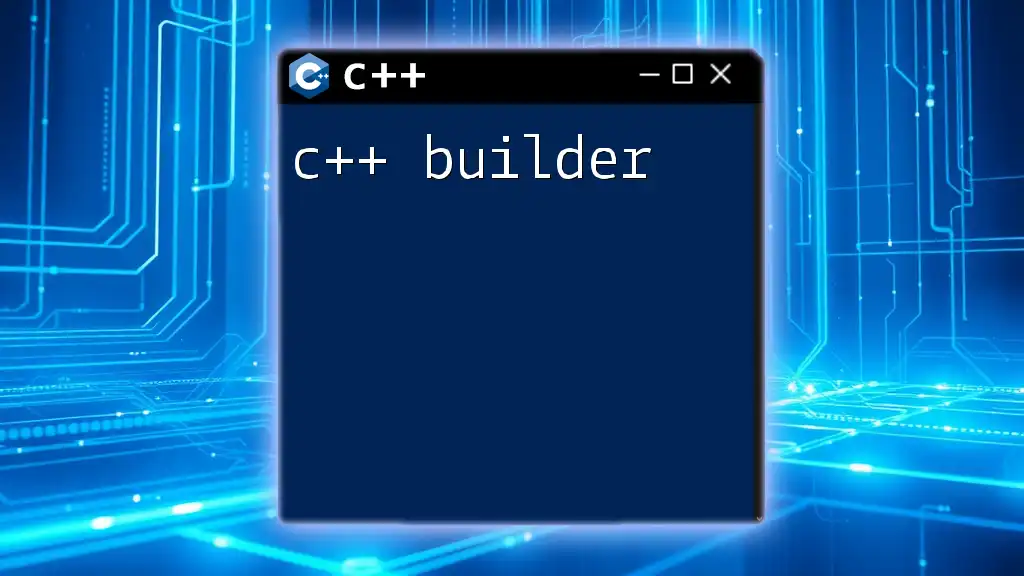
Additional Resources
For those interested in deepening their understanding of C++ string handling, a wealth of resources is available, including comprehensive books and online courses. Libraries that extend string functionalities are also beneficial, providing additional flexibility for developers faced with complex string manipulation tasks.