In C++, a print buffer temporarily holds data before it is output to the console, allowing for efficient I/O operations.
Here’s a simple example of using the print buffer with `std::cout` in C++:
#include <iostream>
#include <sstream>
int main() {
std::ostringstream buffer;
buffer << "Hello, ";
buffer << "world!";
std::cout << buffer.str() << std::endl;
return 0;
}
Understanding Print Buffers in C++
What Is a Print Buffer?
A print buffer in C++ is a temporary storage area that holds data intended for output. When you use output streams like `std::cout`, instead of writing directly to the console, C++ collects the output in a buffer. This ensures that multiple outputs can be processed efficiently, allowing the program to execute more quickly.
Buffers are critical because they minimize the number of input/output operations, which can be expensive in terms of time. Writing data to IO devices, such as screens or files, takes significantly longer than writing to memory. Therefore, by using a buffer, the program can delay actual output operations until the buffer is full or explicitly flushed, making the output process more optimized.
Types of Buffers in C++
In C++, there are primarily two types of buffers relevant to this discussion: output buffers and input buffers.
- Output Buffers: These are used by output streams like `std::cout` to store data before it is sent to the screen or file. The operating system manages these buffers, deciding when to send the content they hold.
- Input Buffers: The counterpart to output buffers, used by input streams like `std::cin` to store incoming data before it is read and processed by the program.
Furthermore, understanding the distinction between unbuffered and buffered I/O is vital. Buffered I/O leverages the print buffer concept, storing data temporarily, while unbuffered I/O sends data directly without waiting. Unbuffered I/O might be necessary in real-time applications where immediate feedback is required, but buffered I/O is more common in standard applications for its efficiency.

How C++ Handles Output Streams
The Role of `std::cout`
`std::cout` is the primary output stream used in C++. Behind the scenes, it uses a print buffer to collect output before actually displaying it on the screen. When you execute commands such as `std::cout << "Hello, World!"`, the string is placed in a buffer rather than written immediately to the console. This delay can improve performance, especially when multiple pieces of data are being output sequentially.
Buffering Strategies
When dealing with output buffers, C++ employs several buffering strategies:
-
Line Buffering: In this mode, the buffer is flushed whenever a newline character is encountered. This is particularly useful in interactive applications, where immediate feedback is required. Here’s a small example demonstrating line buffering:
#include <iostream> int main() { std::cout << "This will appear immediately on the screen.\n"; std::cout << "This will also appear shortly after.\n"; return 0; }
-
Fully Buffering: In this strategy, the data is not sent to the output device until the buffer is full. This method is efficient for programs that produce significant output, as it minimizes the number of write operations. However, it may lead to delayed output if the buffer isn't emptied at strategic points.
-
No Buffering: In cases where immediate output is necessary, you might disable buffering altogether. This is commonly used for debugging or real-time applications where latency is unacceptable.
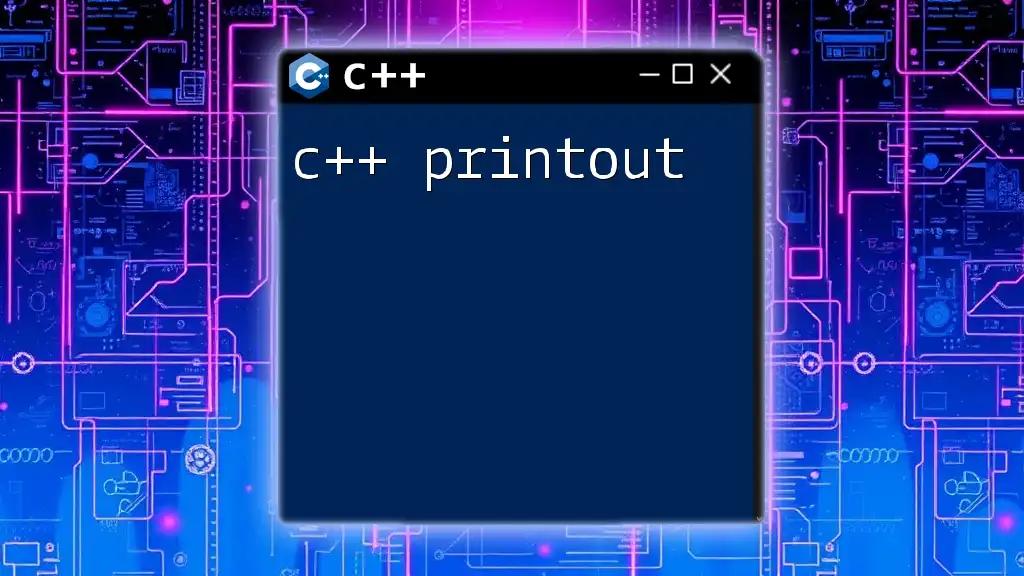
Modifying Buffer Behavior
Flushing the Output Buffer
Flushing a buffer means that you are forcing any buffered data to be written out. This is often done when you need to ensure that previous output is visible to the user without waiting for the buffer to fill up.
Flushing can be performed using the `flush()` function. Consider this example where flushing guarantees timely output:
#include <iostream>
int main() {
std::cout << "Hello, ";
std::cout.flush(); // Ensure immediate output
std::cout << "World!" << std::endl;
return 0;
}
In this example, the first part of the output is immediately displayed because we explicitly flushed the buffer.
Controlling Buffer Size
C++ allows programmers to control buffer sizes, which can lead to more efficient memory use, especially for applications requiring high performance. By defining a custom buffer, you can specify how large that buffer will be.
Implementing a custom stream buffer involves subclassing `std::streambuf`. Here’s a simplified example:
#include <iostream>
#include <streambuf>
class CustomBuffer : public std::streambuf {
// Implement the custom stream buffer features here
};
int main() {
CustomBuffer myBuffer; // Creating an instance of the custom buffer
std::ostream myOutput(&myBuffer);
myOutput << "Hello, Custom World!"; // Output directed to custom buffer
return 0;
}
This example sets up a custom buffer, which enables greater control over how and when data is actually sent to the output stream.
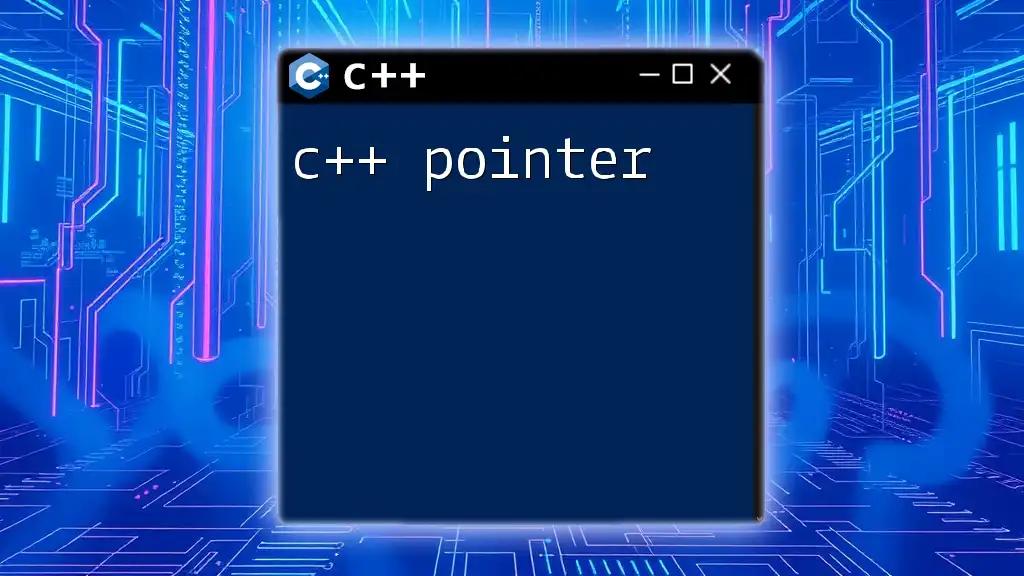
Best Practices for Print Buffers
When to Use Print Buffers
Leveraging print buffers is advantageous particularly in scenarios where you expect to generate large amounts of output. They can help reduce the overhead associated with frequent output operations. For example, in logging systems or data processing applications that output large datasets, utilizing buffers can lead to substantial performance improvements.
Common Pitfalls
One of the most frequent mistakes when working with print buffers is neglecting to flush them when necessary. Failing to flush can result in incomplete output, which can be critical in user-facing applications. Additionally, mismanaging buffer sizes can lead to inefficient memory use, especially in systems with limited resources. Be careful to balance your buffer size based on the needs of your application.

Real-World Applications of Print Buffers
Logging Systems
Print buffers play a vital role in logging systems, where large amounts of data are processed and recorded. By buffering log messages until a certain threshold or condition is met, these systems can ensure efficient writing to files without overloading the system’s I/O capabilities. For instance, many applications implement a background logging system that periodically flushes buffered logs to persistent storage rather than writing each log entry immediately.
Performance Tuning in Applications
Proper control over your print buffers can lead to significant performance tuning in applications. Measuring response times before and after optimizing buffer behavior can demonstrate noteworthy improvements. For instance, applications that rely heavily on console output for debugging can benefit from adjusting buffer sizes or using different buffering strategies to achieve a balance of speed and reliability.

Conclusion
Recap of Key Takeaways
In summary, understanding the concept of C++ print buffers is crucial for optimizing output operations in your applications. It helps you control how data is managed in memory during output operations, leading to more efficient applications that perform well, even under heavy load.
Further Learning Resources
For readers keen on diving deeper into the world of C++ I/O and buffering techniques, various books and online resources are available that delve into advanced programming concepts, best practices, and emerging trends in C++ development.

Call to Action
Now that you're equipped with a foundational understanding of C++ print buffers, I encourage you to implement these strategies in your projects. Feel free to reach out with any questions or share your experiences as you explore the nuanced world of C++ print buffers!