To print a binary representation of an integer in C++, you can utilize the `std::bitset` class from the `<bitset>` header, as shown in the following code snippet:
#include <iostream>
#include <bitset>
int main() {
int number = 42; // Change this number to test different values
std::bitset<8> binary(number);
std::cout << binary << std::endl; // Prints the binary representation
return 0;
}
What is C++?
C++ is a powerful, versatile programming language that combines both high-level and low-level programming capabilities. It is widely used in various domains such as systems programming, game development, and application software. Understanding how to manipulate data effectively is crucial in C++, notably how to represent and display data in various formats, including binary.
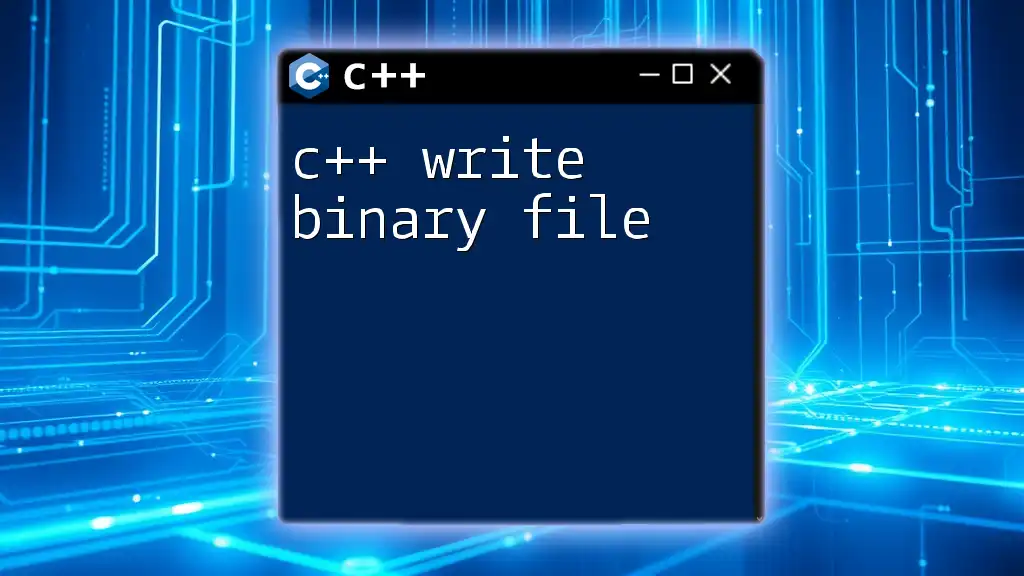
Understanding Binary Representation
Binary representation is the fundamental language of computers. It uses only two symbols: 0 and 1. Each digit in a binary number is referred to as a "bit." Since computers operate on binary data, knowing how to print binary in C++ can be essential for debugging and understanding underlying data structures.
Why Know How to Print Binary?
Printing numbers in binary format can greatly enhance your understanding of how data is stored and manipulated at a low level. This knowledge is invaluable for optimizing performance and debugging issues related to bit manipulation in C++.
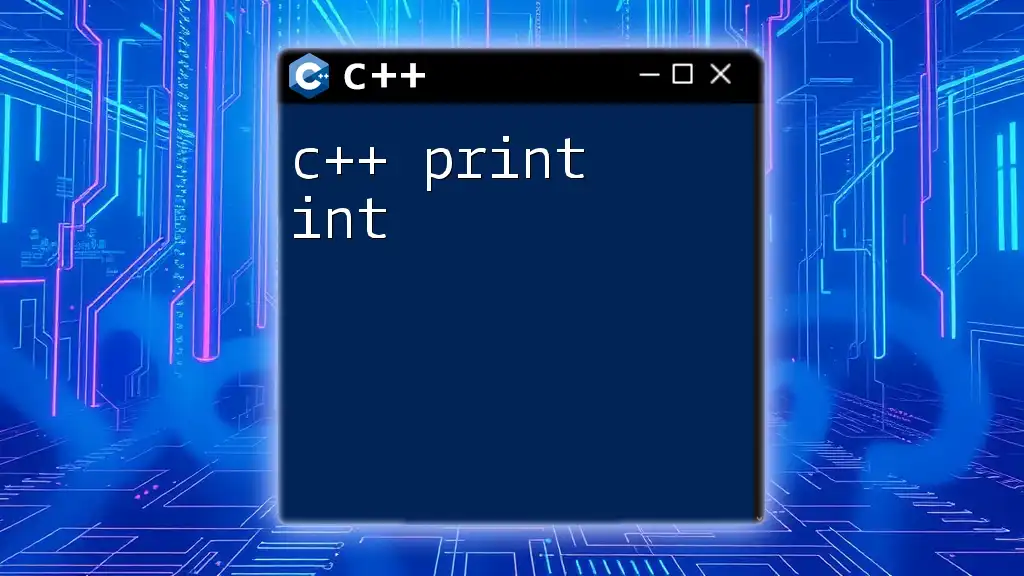
Setting Up the Environment for C++
Choosing a Compiler
Before delving into C++ programming, it’s vital to have a working environment. Popular compilers include GCC (GNU Compiler Collection), MSVC (Microsoft Visual C++), and Clang. Each has its own installation process and features, so choose the one that best suits your needs and operating system.
Writing Your First C++ Program
The basic structure of a C++ program consists of key components: headers, main function, and statements. Here's a simple "Hello World" program to get you started:
#include <iostream>
int main() {
std::cout << "Hello World!" << std::endl;
return 0;
}
This snippet demonstrates how to include the `iostream` library, which provides functionalities for input and output operations.
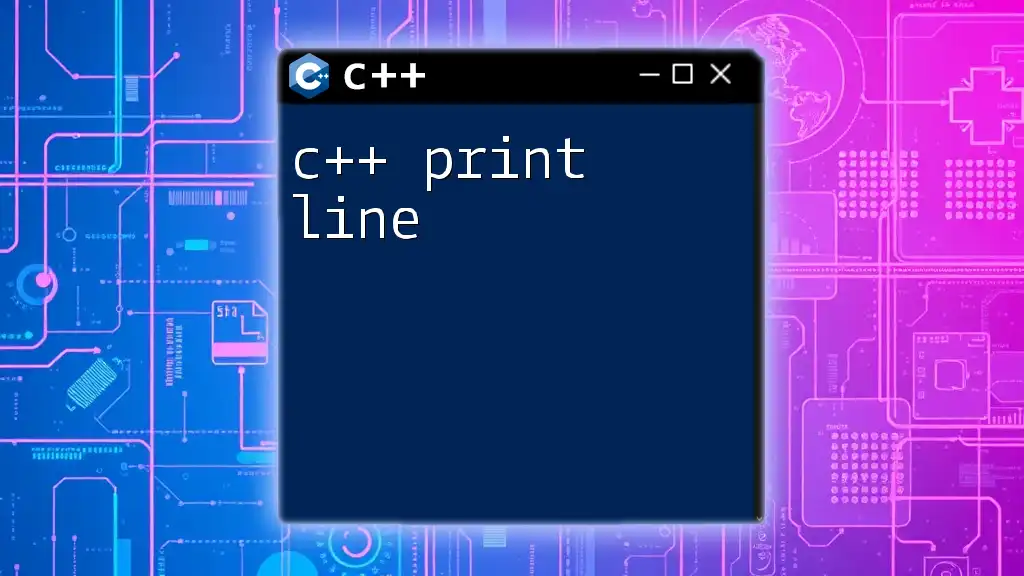
Printing Binary in C++
Using Standard Output Streams
Overview of Output Streams
In C++, the standard output stream (`std::cout`) allows you to print data to the console. Before using it for printing binary numbers, make sure to include the required header.
Basic Example of Printing Integers
To print an integer, you can directly use `std::cout`. Here’s a simple snippet:
#include <iostream>
int main() {
int number = 5;
std::cout << "Decimal: " << number << std::endl;
return 0;
}
This code prints the decimal representation of the number.
Custom Function to Print Binary
Creating a Binary Conversion Function
To print a number in binary format, you can create a custom function. The function below uses recursion to convert a decimal integer to binary:
void printBinary(int n) {
if (n > 1)
printBinary(n >> 1);
std::cout << (n & 1);
}
Using the Function in a Program
Now, let’s integrate this function into a complete program:
#include <iostream>
void printBinary(int n) {
if (n > 1)
printBinary(n >> 1);
std::cout << (n & 1);
}
int main() {
int number = 5;
std::cout << "Binary: ";
printBinary(number);
std::cout << std::endl;
return 0;
}
This program will output the binary representation of the number 5, which is "101".
Formatting Output for Better Readability
In many situations, particularly with larger numbers, you might want to present the binary in a way that’s easier to read. Grouping bits into clusters (e.g., sets of four) can help achieve this clarity. You could modify the `printBinary` function to achieve this formatting.
Using Bitset for Binary Representation
Overview of `std::bitset`
C++ provides the `std::bitset` class, which represents a fixed-size sequence of bits. It simplifies the process of printing binary numbers and offers various functionalities. To use it, you need to include the appropriate header:
#include <bitset>
Example of Using Bitset
Here’s how you can use a `bitset` to print a number in binary:
#include <iostream>
#include <bitset>
int main() {
int number = 5;
std::bitset<8> binary(number);
std::cout << "Binary using bitset: " << binary << std::endl;
return 0;
}
In this example, the `bitset` allows for easy representation and manipulation of binary numbers, automatically handling the conversion and display.
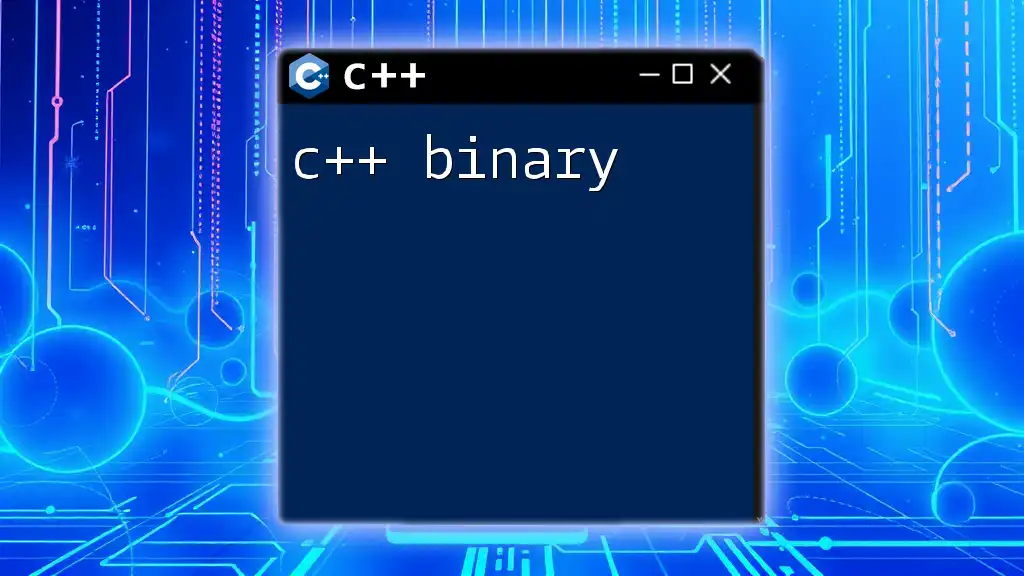
Advanced Techniques for Printing Binary
Customizing Output for Different Data Types
C++ allows for multiple integral types, including `int`, `short`, `long`, and `unsigned`. It’s beneficial to know how to print binary representations for these varying types. You may also create overloaded functions for each type if specific formatting is required.
Function Templates for Flexibility
Function templates are a great way to create a binary printing function that can handle multiple data types flexibly. Here’s a brief snippet demonstrating a template function:
template <typename T>
void printBinary(T n) {
// Implementation here
}
Using templates simplifies code maintenance and enhances code reusability.
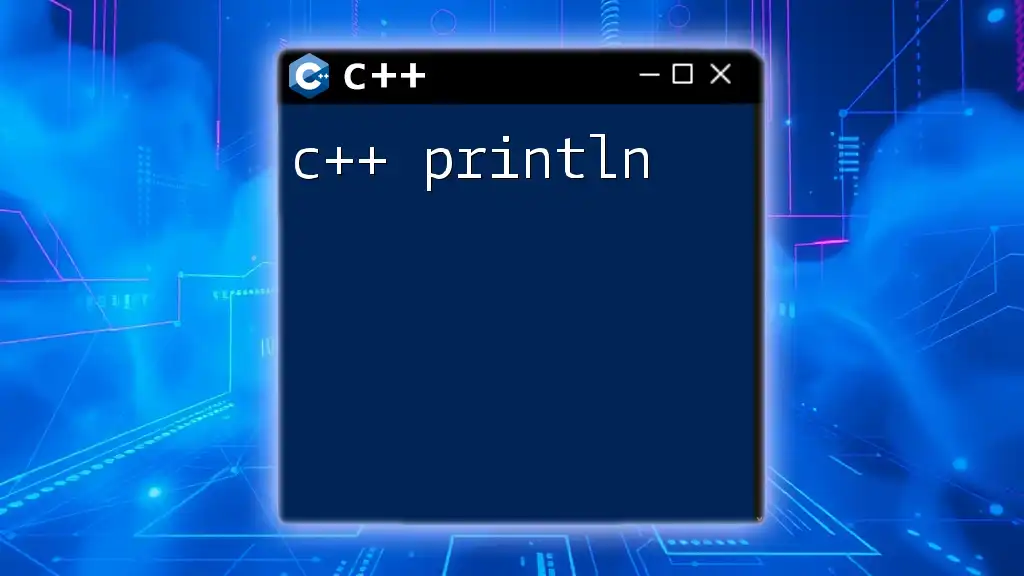
Practical Applications of Printing Binary
Debugging and Verification
Printing binary can be an invaluable tool for debugging and verifying the integrity of data in your applications. For example, when manipulating bits or performing bitwise operations, you may want to confirm how your data is structured at a binary level to ensure correctness.
Learning and Educational Uses
Understanding binary representation is crucial for anyone learning C++. Implementing and practicing binary conversions can enhance problem-solving skills and deepen comprehension of how data is processed and stored by computers.
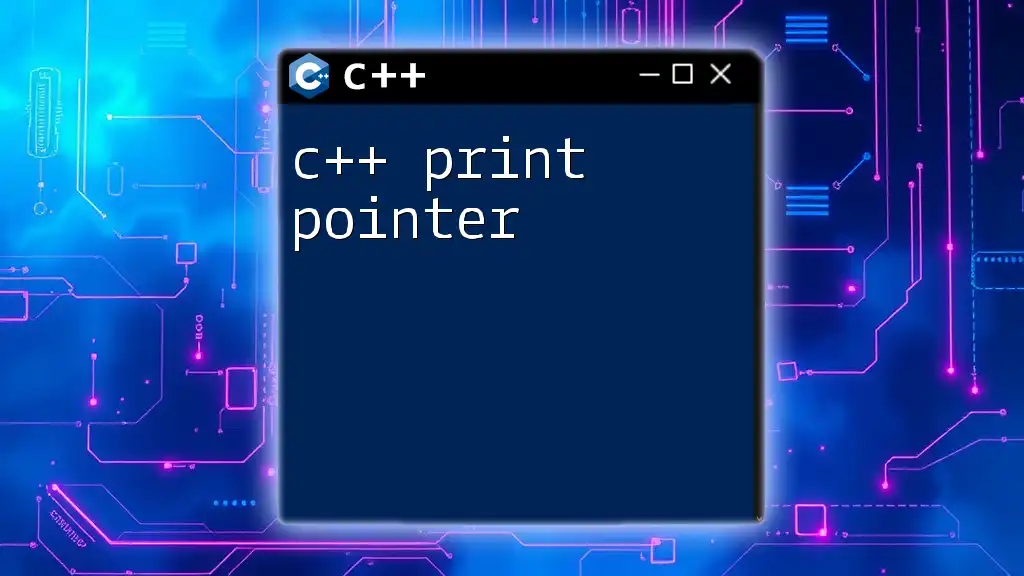
Conclusion
In this guide, we’ve explored the various methods to print binary in C++. From the basic use of output streams to custom functions and `std::bitset`, there are many ways to represent numbers in binary format.
To further enhance your learning experience, consider implementing these concepts in your projects and experimenting with different data types and binary representations. Engaging with this topic will solidify your understanding of the essential role binary plays in computing.
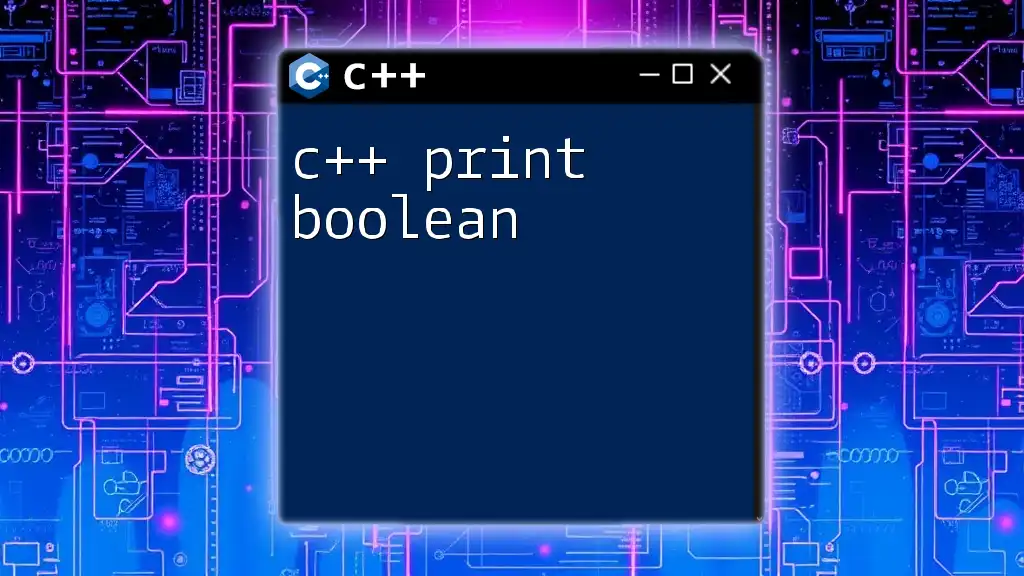
Call to Action
We invite you to share your experiences with printing binary in C++. What challenges have you faced? What tips can you offer? Explore our other articles on C++ and continue your journey into the fascinating world of programming!