In C++, you can write binary data to a file by opening a file stream in binary mode and using the `write` function, as shown in the following example:
#include <fstream>
int main() {
std::ofstream outFile("example.bin", std::ios::binary);
int data = 42;
outFile.write(reinterpret_cast<char*>(&data), sizeof(data));
outFile.close();
return 0;
}
What is a Binary File?
Definition
A binary file is a file that contains data in a format that is not meant to be read as plain text. Binary files can include various types of data, such as images, audio, and compiled programs. Unlike text files, which store data as sequences of readable characters, binary files store data in a way that is efficient for a machine to process. This makes them ideal for applications where performance and size matter.
Characteristics of Binary Files
- Data Format and Structure: Binary data is often structured in blocks or records that can be accessed more quickly than text. Each piece of data may be variable length or fixed length, depending on how it is constructed.
- Advantages of Using Binary Files: Binary files tend to be smaller in size compared to their text counterparts because they do not contain extra formatting characters. Additionally, reading and writing binary files is generally faster. This is significant in applications where large amounts of data need to be processed efficiently.
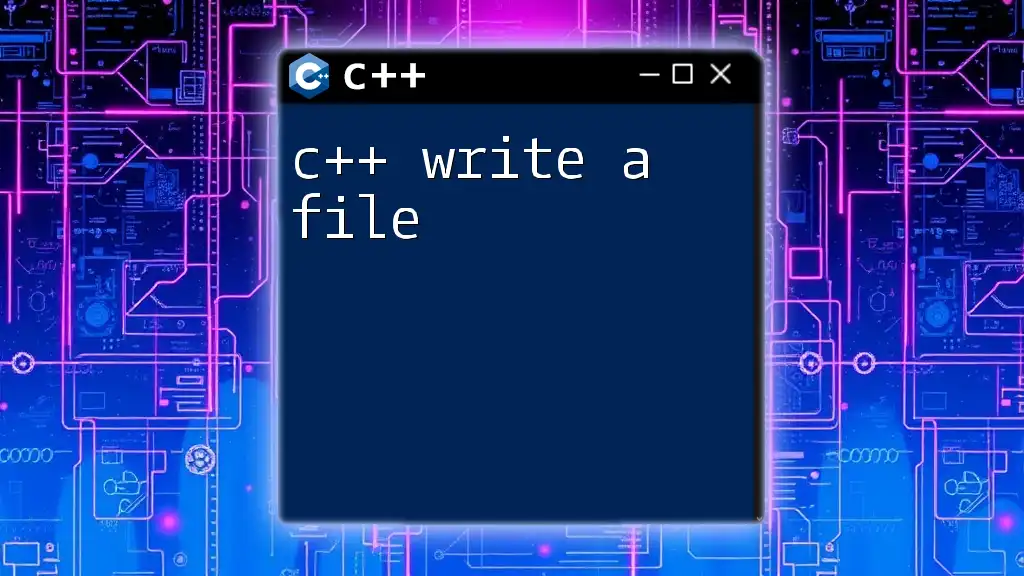
Setting Up Your C++ Environment
Necessary Libraries
To work with binary files in C++, you will need to include two essential libraries:
#include <iostream>
#include <fstream>
- `<iostream>`: This library is used for input and output stream functionalities.
- `<fstream>`: This library is specifically designed for file handling, enabling both reading and writing operations on files.
Compiling Your Program
When compiling your C++ program in an environment where binary file operations are implemented, you can use standard commands:
g++ -o my_program my_program.cpp
Make sure to replace `my_program.cpp` with the name of your actual file.
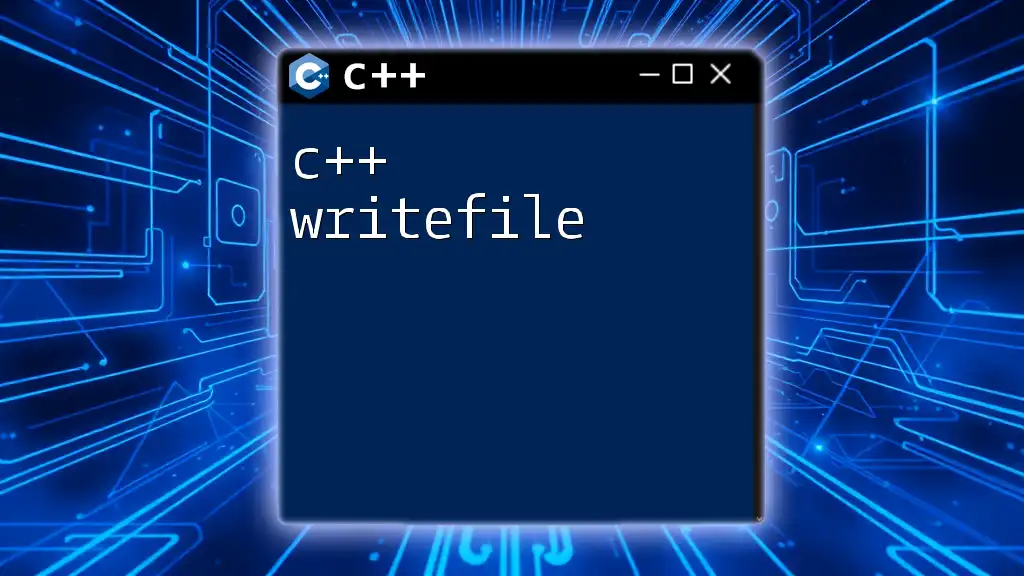
Writing a Binary File in C++
Step-by-Step Process
Opening a File
When writing to a binary file, the first step is to open the file with the appropriate mode using the `ofstream` class. It is crucial to open the file in binary mode to ensure that data is written correctly.
std::ofstream outFile("example.bin", std::ios::out | std::ios::binary);
- `std::ios::out`: This indicates that you are opening the file for output operations.
- `std::ios::binary`: This flag specifies that you are working with a binary file.
Writing Data to a File
When you write data to a binary file, you need to understand how data types are represented in binary format. The `write` method of the `ofstream` class allows you to write the raw byte representation of data to the file.
Here is how you can write an integer to a binary file:
int number = 42;
outFile.write(reinterpret_cast<char*>(&number), sizeof(number));
- `reinterpret_cast<char>(&number)`**: This casts the address of the integer `number` to a `char`, making it suitable for binary writing.
- `sizeof(number)`: This specifies the number of bytes to write (which, in this case, will be 4 bytes for an `int`).
Closing the File
It is essential to close the file after writing to ensure that all data is flushed and not remaining in the output buffer. Closing the file can be done using:
outFile.close();
Failing to close the file can lead to data loss or corruption.
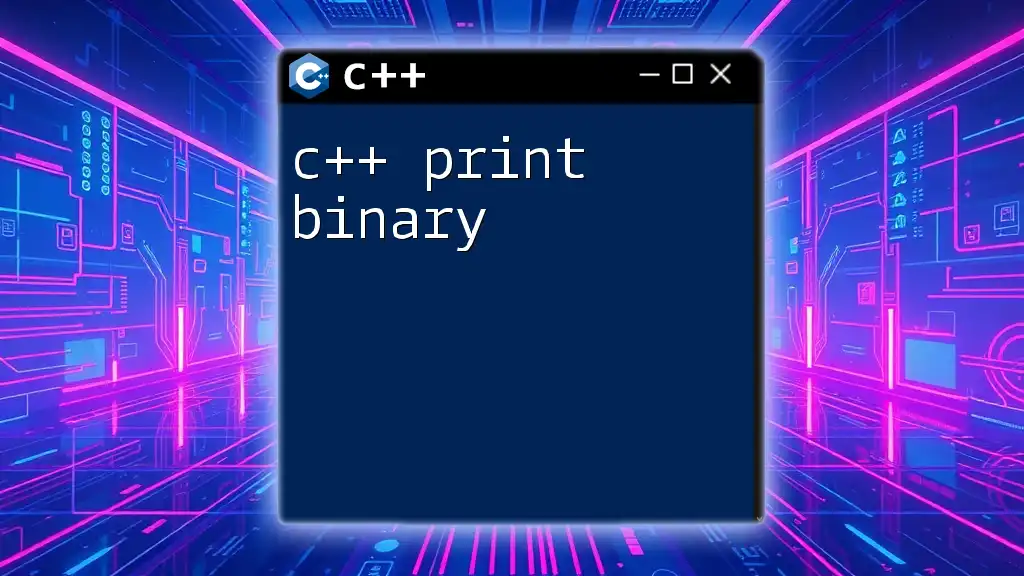
Example: A Complete C++ Program to Write a Binary File
Overview of the Example Program
In this section, we will look at a complete C++ program that writes an integer to a binary file named "data.bin."
Code Snippet of the Complete Program
#include <iostream>
#include <fstream>
int main() {
std::ofstream outFile("data.bin", std::ios::out | std::ios::binary);
if (!outFile) {
std::cerr << "Error opening file!" << std::endl;
return 1;
}
int number = 42;
outFile.write(reinterpret_cast<char*>(&number), sizeof(number));
outFile.close();
return 0;
}
Explanation of the Example Code
- The program begins by including necessary libraries.
- It opens the "data.bin" file in binary write mode. The condition that checks if the file is successfully opened is crucial; if it fails, it will output an error message and terminate the program.
- An integer `number` is defined and written to the binary file using the `write` method.
- Finally, the file is closed to ensure all buffers are flushed.
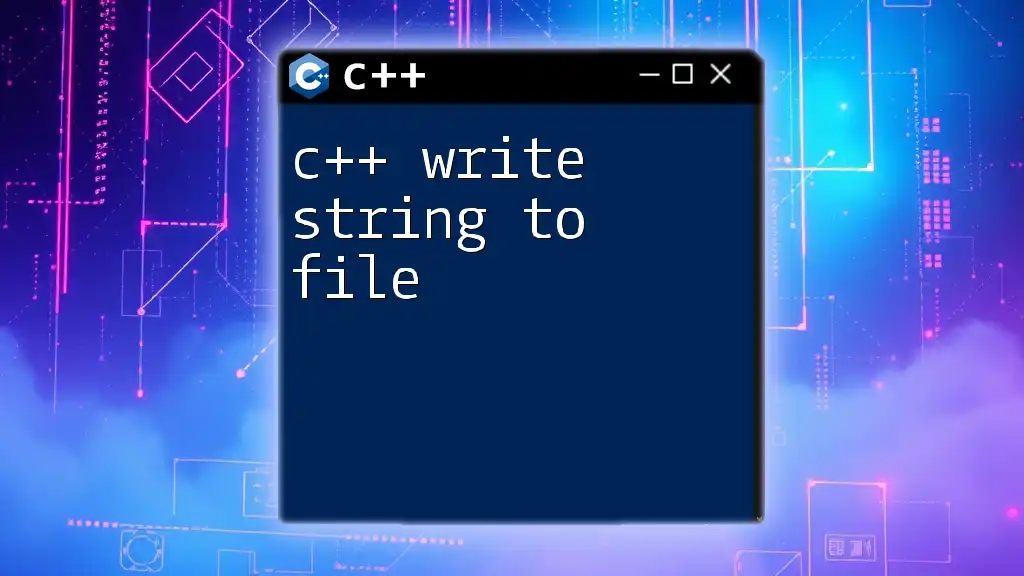
Common Mistakes to Avoid
Not Opening Files in Binary Mode
A frequent error is neglecting to specify the binary mode when opening a file. This oversight can result in data corruption, particularly if you are storing complex data types.
Not Checking for File Open Success
Always check if the file has opened successfully. This simple step can save you from debugging headaches later.
Forgetting to Close the File
Closing files is a best practice to ensure that system resources are freed and data integrity is maintained.
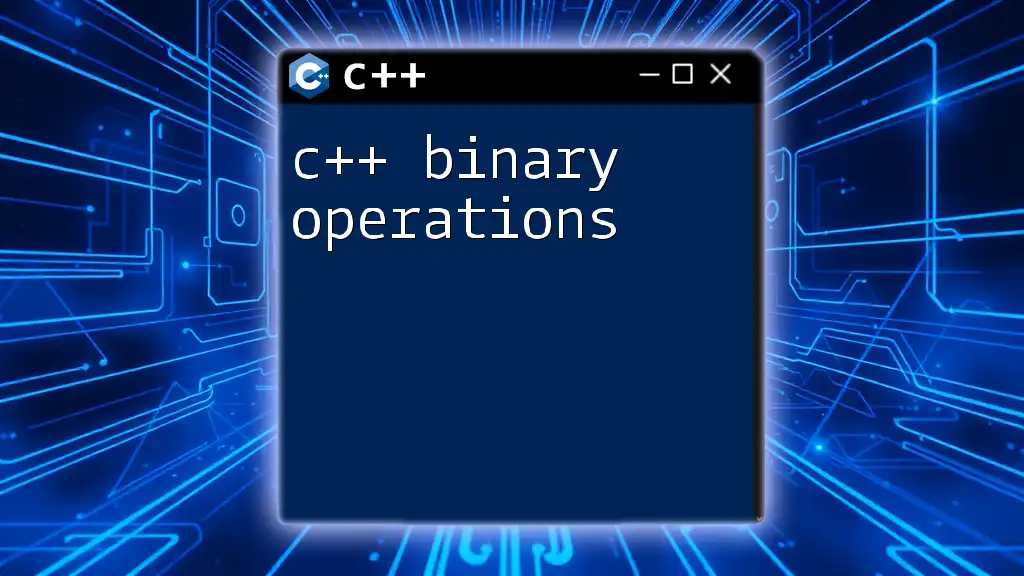
Reading from a Binary File (Optional)
Brief Overview of Reading Binary Data
Understanding how to read binary data complements your ability to write to files. Users often need to retrieve stored data, and this section will outline the basics of reading data from a binary file.
Code Example for Reading Data
Here is how you can read the integer value back from the binary file we created earlier:
std::ifstream inFile("data.bin", std::ios::in | std::ios::binary);
int num;
inFile.read(reinterpret_cast<char*>(&num), sizeof(num));
inFile.close();
This code snippet opens the binary file in read mode, retrieves the integer data, and safely closes the file afterward.
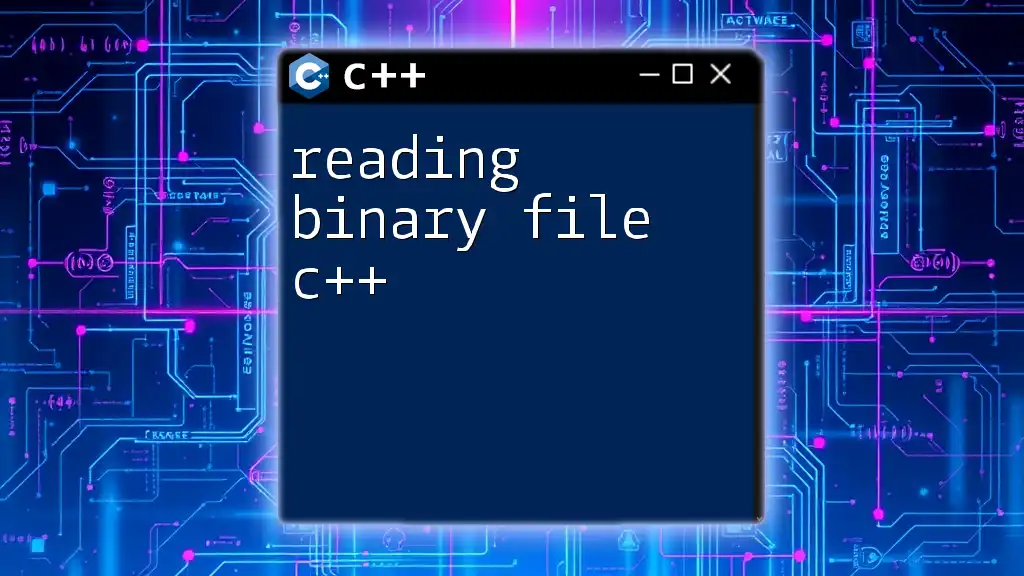
Conclusion
In summary, being able to c++ write binary file is an invaluable skill for any programmer. Using binary files allows for efficient storage and retrieval of data, particularly in applications that require performance optimization. Make sure to practice these concepts with different data types to become proficient in handling binary files in C++. Your journey toward mastering binary file operations is only just beginning!
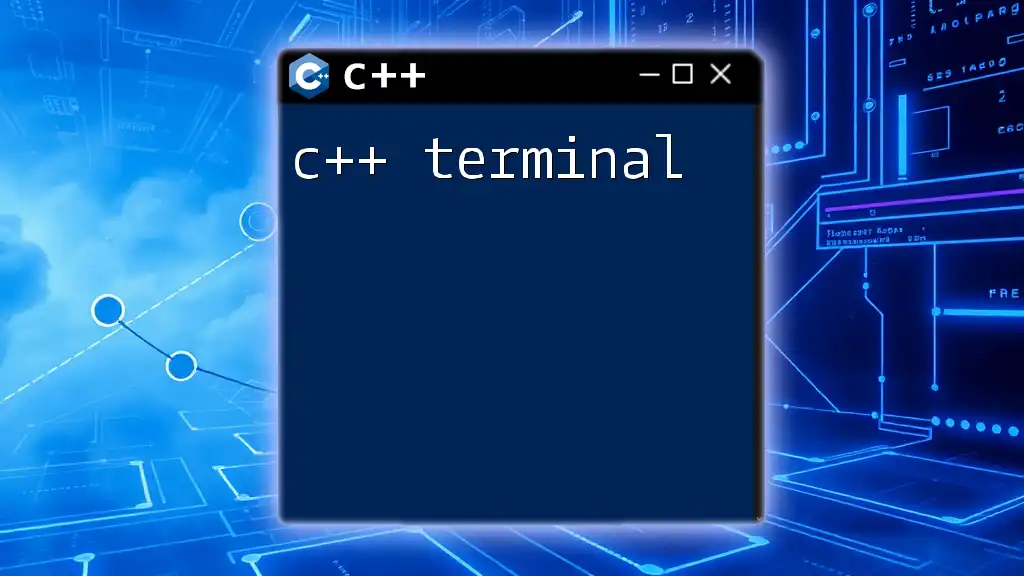
Additional Resources
For further exploration, consider looking into the official C++ documentation regarding file handling or accessing additional tutorials on advanced binary file operations. Happy coding!