An `initializer_list` in C++ allows you to create a list of values for initializing a container or passing multiple arguments to a function in a convenient way.
Here’s an example:
#include <initializer_list>
#include <iostream>
#include <vector>
class MyVector {
public:
MyVector(std::initializer_list<int> list) {
for (auto& element : list) {
vec.push_back(element);
}
}
void display() {
for (const auto& element : vec) {
std::cout << element << ' ';
}
std::cout << std::endl;
}
private:
std::vector<int> vec;
};
int main() {
MyVector mv = {1, 2, 3, 4, 5};
mv.display(); // Output: 1 2 3 4 5
return 0;
}
Understanding Initializer Lists
How Initializer Lists Work
In C++, an initializer list is a feature that allows you to initialize objects using a comma-separated list of values enclosed in braces. This mechanism offers a cleaner and more concise way to initialize objects compared to traditional constructor syntax. Unlike the conventional approach where you would need to specify each member assignment inside the constructor body, initializer lists allow you to initialize members directly as part of the constructor's parameter list.
For example, consider the following use of a class constructor:
class Person {
public:
std::string name;
int age;
Person(std::string n, int a) {
name = n;
age = a;
}
};
// Traditional approach
Person p("John", 30);
Using an initializer list approach would look like this:
class Person {
public:
std::string name;
int age;
// Initializer list in constructor
Person(std::string n, int a) : name(n), age(a) {}
};
// Using initializer list
Person p{"John", 30};
The use of initializer lists here makes the code clearer and more elegant.
Syntax Overview
The syntax for using an initializer list is straightforward. When you define a constructor for a class, you specify the member variables to be initialized in the constructor's parameter list, followed by a colon (`:`) and then the initializations themselves enclosed in braces. For instance:
class MyClass {
public:
int a;
int b;
MyClass(int x, int y) : a(x), b(y) {}
};
// Creating an object using the initializer list syntax
MyClass obj{1, 2};
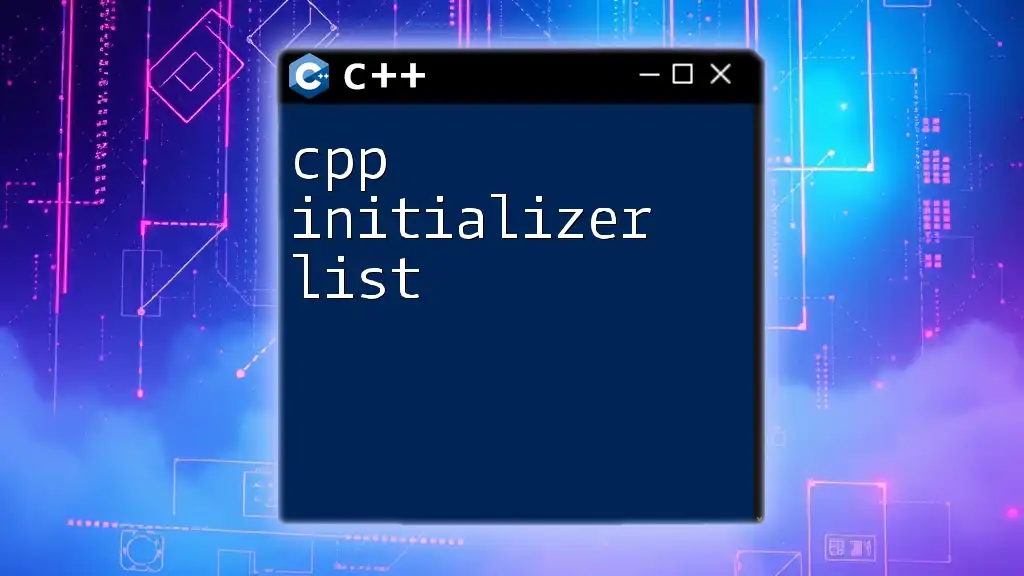
Use Cases for Initializer Lists
Initializing STL Containers
One of the most common use cases for initializer lists is initializing Standard Template Library (STL) containers. This feature significantly simplifies the syntax of initializing collections of data.
For example, using an `std::vector` with initializer lists:
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5}; // Initializing vector with initializer list
for (auto& val : vec) {
std::cout << val << " ";
}
return 0;
}
This approach is not only cleaner but can also help to reduce errors related to size or type mismatches.
Custom Classes and Structs
You can also enable initializer lists for your custom classes by defining constructors that accept `std::initializer_list`. This allows users of your class to initialize it similarly to how they would initialize a container.
Here’s an example of creating a custom class that uses an initializer list to set its member variables:
class Point {
public:
int x;
int y;
Point(std::initializer_list<int> list) {
auto it = list.begin();
x = *it++;
y = *it;
}
};
// Using initializer list
Point p = {3, 4}; // sets x=3, y=4
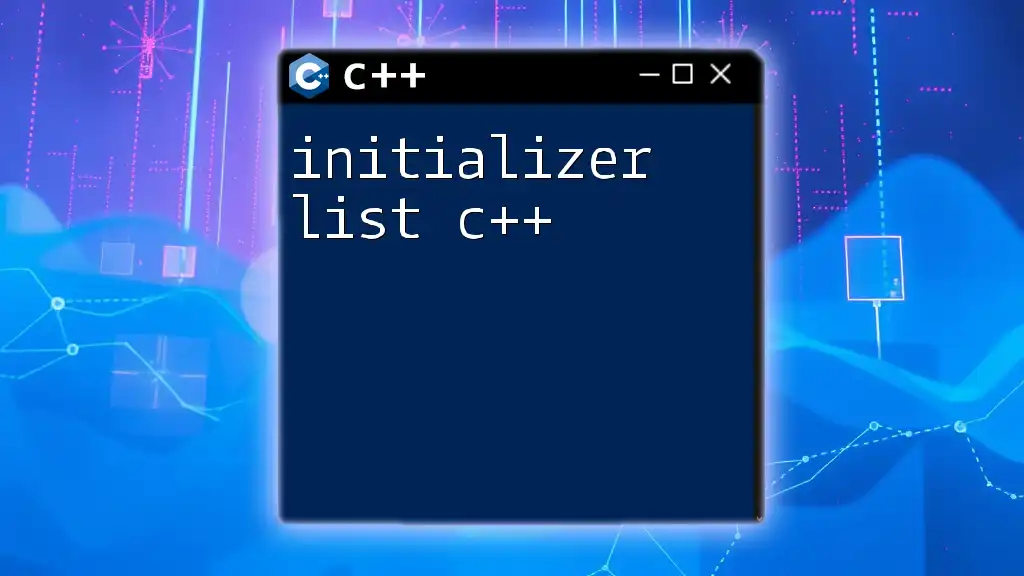
Creating and Using Initializer List in C++
Defining a Class with Initializer List Constructor
When you define a constructor using an initializer list, you guarantee that your class members are initialized before the constructor body is executed. This is particularly advantageous for constant or reference members, which must be initialized at construction.
class Rectangle {
public:
int width;
int height;
Rectangle(int w, int h) : width(w), height(h) {} // Using initializer list to initialize
int area() const { return width * height; }
};
// Using initializer list
Rectangle rect{10, 20}; // width=10, height=20
This guarantees that both `width` and `height` are assigned values right at the moment of construction, allowing for proper and reliable object state right from the start.
Benefits of Using Initializer Lists
Using initializer lists offers several benefits:
-
Improved Readability: The syntax is often cleaner and more straightforward than traditional constructor assignments, making the code easier to read and understand.
-
Performance Advantages: Initializer lists can result in better performance because they allow for direct initialization of member variables, often eliminating unnecessary default constructions followed by assignment.
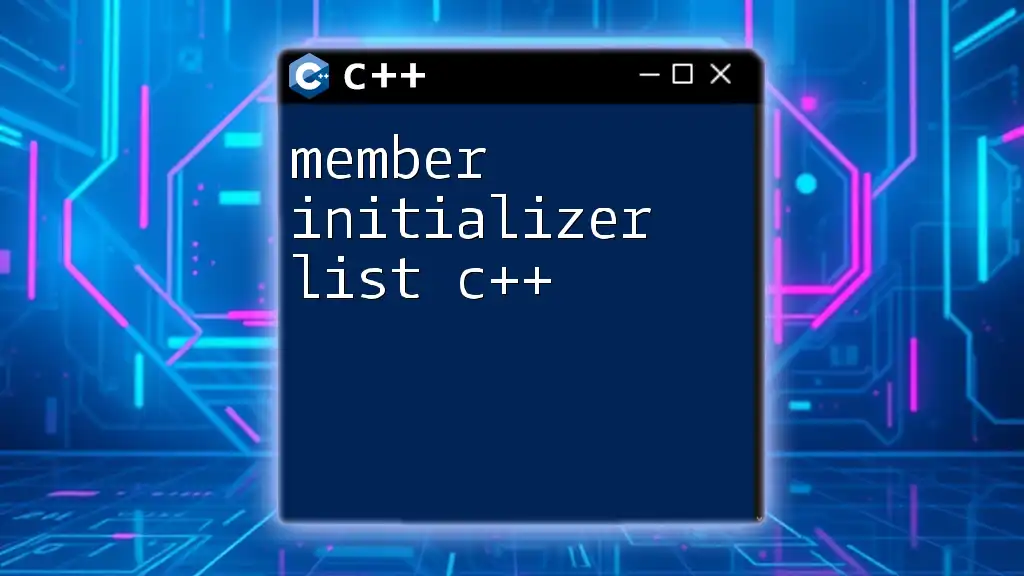
Common Pitfalls
Misusing Initializer Lists
While initializer lists can simplify your code, they also come with potential pitfalls, especially for novice developers. A common mistake is forgetting to provide the correct number of values.
Point p = {3}; // Error: Point expects two values for x and y
This error occurs because the `Point` constructor expects an initializer list with exactly two integers. Such discrepancies can lead to compile-time errors, hindering project progress.
Limitations of Initializer Lists
Despite their advantages, initializer lists have certain limitations. For instance, they cannot be used for types that do not support brace initialization, such as certain class types lacking appropriate constructors. Additionally, care must be taken when using initializer lists with polymorphism, as derived classes must properly implement constructors accepting initializer lists.
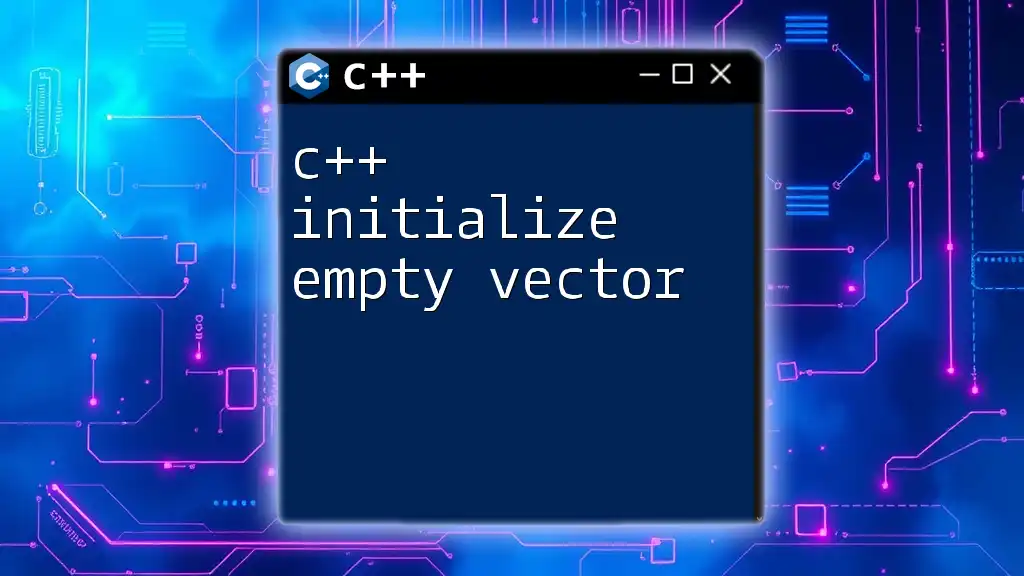
Conclusion
In summary, the C++ initializer_list feature is a powerful tool that enhances code clarity, efficiency, and expressiveness. Whether you’re initializing STL containers or user-defined classes, initializer lists offer a concise solution that helps streamline the initialization process. It's important to experiment with this feature in your own projects to fully appreciate its utility and capabilities.
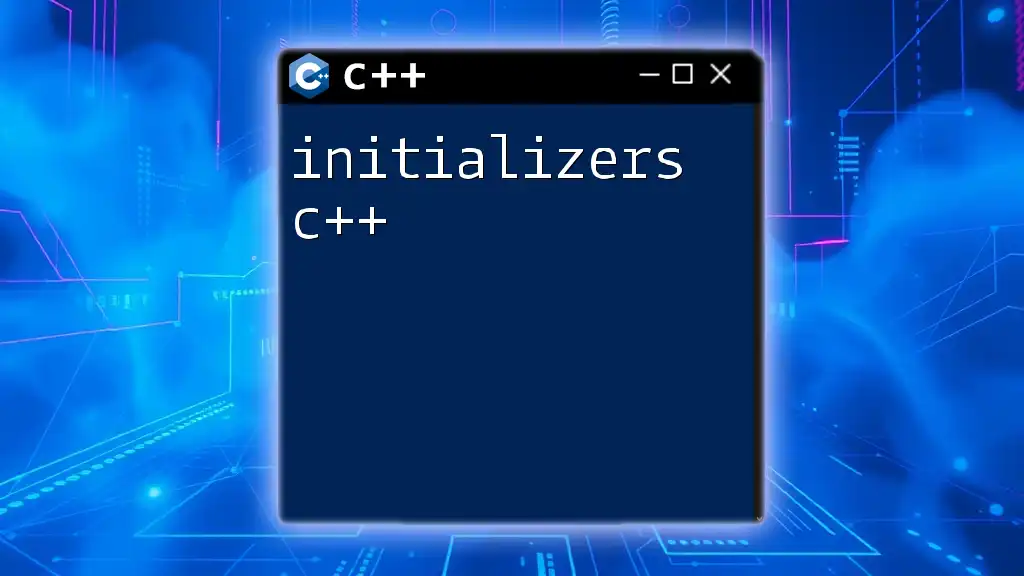
Additional Resources
For those looking to dive deeper into C++ initializer lists and further enhance their knowledge, consider exploring books on modern C++ design, official documentation, and online tutorials that thoroughly cover the nuances of initializer lists and their appropriate usage.
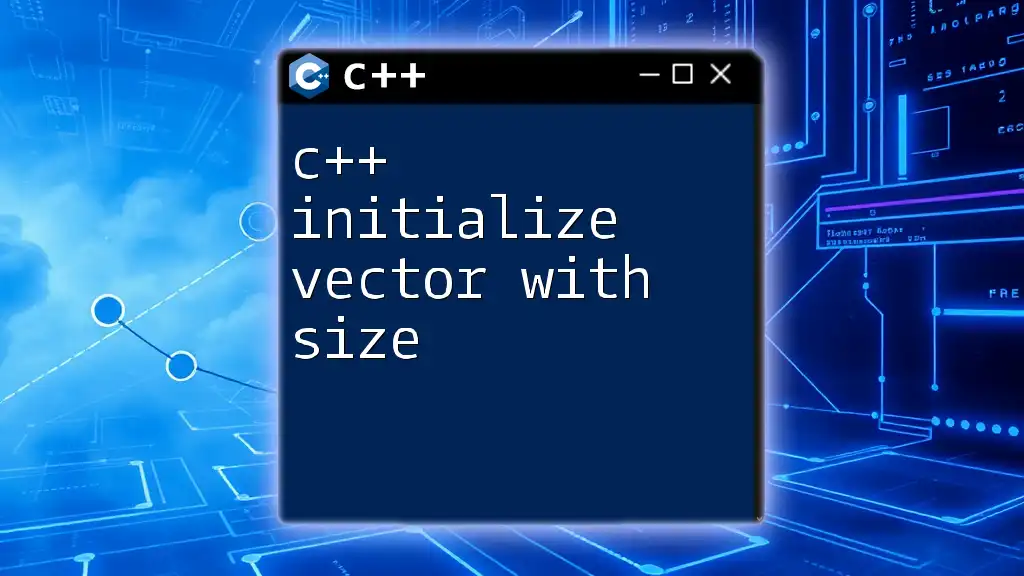
FAQ Section
Frequently Asked Questions about Initializer Lists
-
What is the difference between initializer lists and arrays? Initializer lists are a C++ feature that allows initializing variables directly with a set of values, whereas arrays are data structures that hold multiple items of the same type. Arrays do not have constructors like initializer lists.
-
Can initializer lists be used with inheritance? Yes, initializer lists can be utilized in derived classes. However, constructors in both base and derived classes must correctly handle initialization in their respective initializer lists.
-
How do initializer lists interact with default constructors? Default constructors cannot work with initializer lists directly. If you want to use an initializer list with default parameters, you must define your constructor accordingly in both the base and derived classes.
With this comprehensive guide on C++ initializer_list, you are now well-equipped to implement this powerful feature in your coding endeavors. Happy coding!