In C++, initializers are used to provide initial values to variables at the time of declaration, enhancing clarity and reducing the chance of uninitialized variable errors.
Here’s an example:
int main() {
int x = 5; // Direct initialization
int y(10); // Constructor-style initialization
int z{15}; // Uniform initialization (C++11)
return 0;
}
What Are C++ Initializers?
In C++, initializers are critical constructs that allow you to set values for variables when they are declared. They serve to initialize memory, ensuring that variables begin their lives with known values, which helps prevent undefined behavior in programs. Understanding how to use initializers effectively is crucial for writing robust and maintainable C++ code.
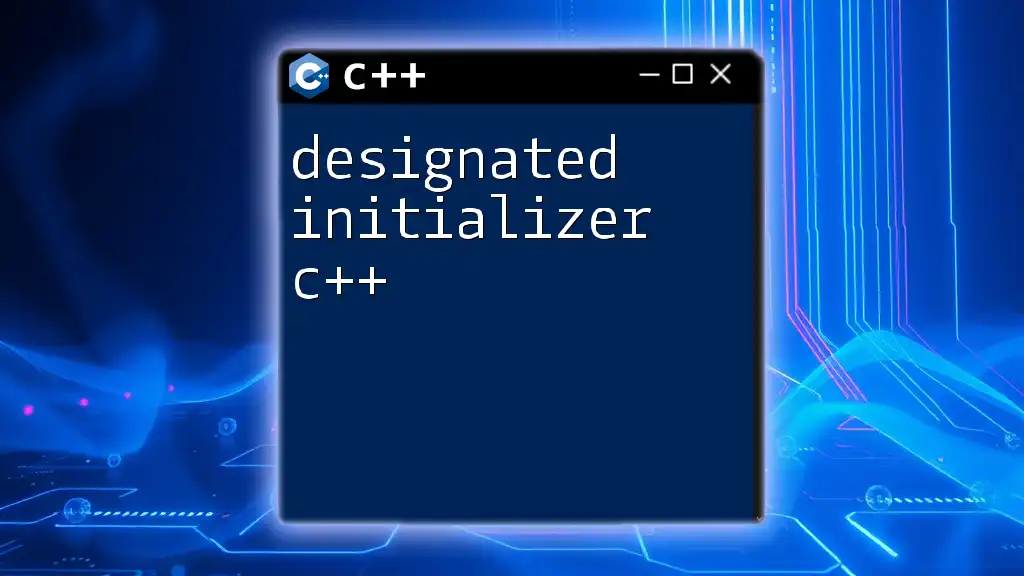
Types of C++ Initializers
Default Initialization
Default initialization occurs when a variable is declared but not explicitly initialized with a value. This means that if the variable is of a built-in type (like `int` or `char`), it remains uninitialized unless it is in an array or static storage duration. Consider the following code snippet:
int x; // x is uninitialized
Here, `x` is declared but does not have a defined value, which can lead to unpredictable results if used before assignment.
Value Initialization
Value initialization happens when a variable is initialized using an empty set of braces. This method guarantees that the variable is set to its default state. For example:
int y{}; // y is initialized to 0
In this case, `y` is guaranteed to start at 0, which eliminates any potential mistakes associated with uninitialized variables.
Copy Initialization
Copy initialization involves assigning a value to a variable at the moment of its declaration using the `=` operator. This method is straightforward but can sometimes lead to unexpected behavior if not used correctly. An example is shown below:
int z = 10; // z is initialized to 10
Here, `z` is a copy of the value `10`, which is quite clear. However, it’s important to note that the copy operation has its own implications, particularly with respect to performance with complex objects.
Direct Initialization
Direct initialization is when you use parentheses to initialize a variable. This method is particularly useful in more complicated scenarios involving non-built-in types like classes. Here’s an example:
int a(5); // a is directly initialized to 5
In this case, `a` receives the value `5` directly, which can sometimes help avoid issues associated with copy initialization, especially in user-defined types.
List Initialization (C++11 and later)
With C++11, list initialization was introduced to provide a more consistent approach to initializing objects. It allows the use of curly braces (`{}`) to initialize variables, enhancing type safety and reducing the risk of narrowing conversions. Example:
int b{10}; // b is initialized to 10
When using list initialization, if you were to attempt to narrow a type (for example, initializing a `double` to an `int`), the compiler would produce an error, providing additional safety.
Aggregate Initialization
Aggregate initialization is a powerful feature for initializing structs or classes with public data members. It allows you to initialize multiple members concisely. Consider the following:
struct Point { int x; int y; };
Point p{1, 2}; // p is initialized with x=1, y=2
In this example, `Point` is initialized directly with `1` and `2`, making the code both readable and efficient.
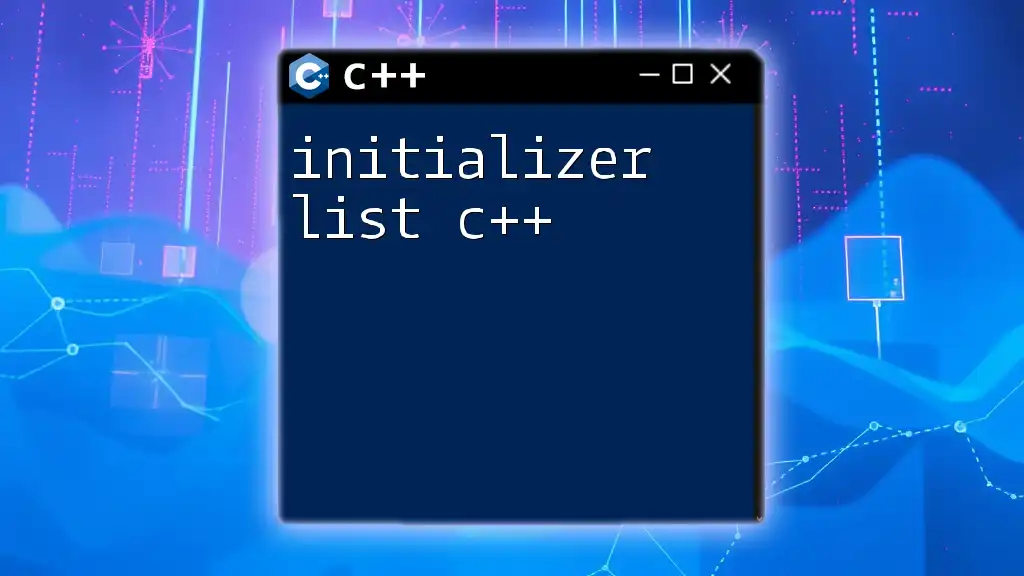
Choosing the Right Initialization Method
When it comes to choosing the right initialization method, it’s crucial to weigh the pros and cons of each approach. Default initialization is simple but risky, while value initialization and list initialization provide safety by ensuring known starting values. Direct initialization is often preferred for objects requiring custom constructors. Choosing wisely boosts code clarity and reduces errors.
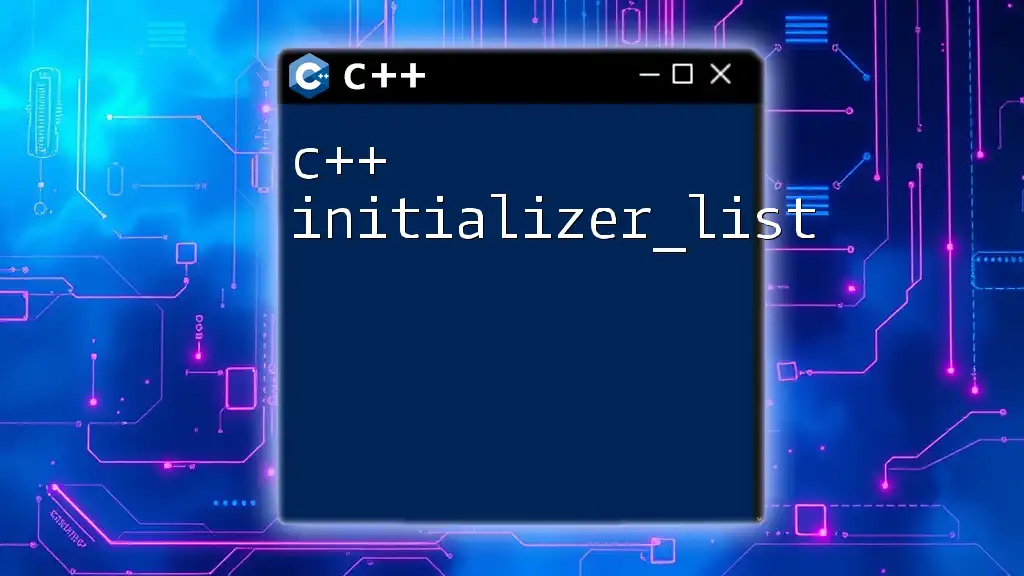
Best Practices for C++ Initialization
Consistency in Style
Maintaining a consistent initialization style throughout your codebase is vital for clarity. Consistency helps other developers (and your future self) quickly understand how variables are being set up. Establishing coding standards that specify when to use which initializer can significantly enhance code quality.
Avoiding Uninitialized Variables
One of the most common pitfalls in C++ programming is the use of uninitialized variables. Uninitialized variables can lead to undefined behavior, which may manifest as crashes or erratic application behavior. Always initialize variables when declaring them, or use tools and compiler settings that alert you to potential risks.
Using `nullptr` for Pointers
Using `nullptr` to initialize pointers is indispensable, as it provides safety for pointer operations. This prevents dereferencing invalid memory addresses. For example:
int* ptr = nullptr; // Safe initialization of a pointer
As a convention, always initialize pointers to `nullptr` unless they can be assigned a valid address immediately. This approach promotes safe memory management practices.
Using `auto` with Initializers
The `auto` keyword can also simplify initialization by allowing the compiler to deduce the variable type based on the assigned value. For instance:
auto myVar = 42; // Type inferred as int
This can enhance readability, but it requires a solid understanding of the intended type to avoid inadvertently introducing bugs.
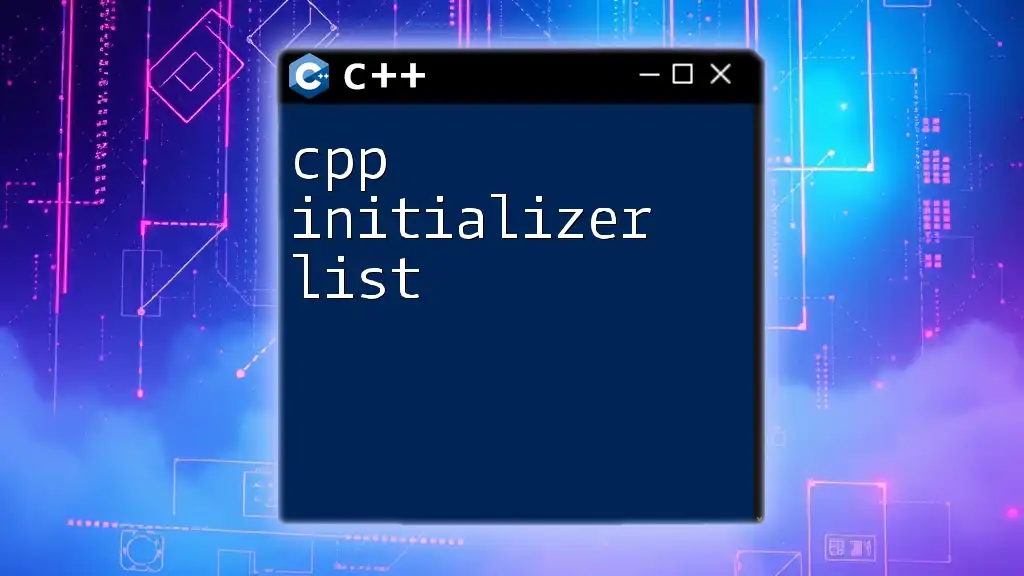
Common Mistakes in C++ Initialization
Over-Initialization
Be wary of over-initialization, which can lead to unnecessarily complex code. When you initialize variables for the sake of being overly explicit or creating redundant assignments, your code can become harder to read and maintain. Always strive for simplicity.
Misunderstanding Copy vs. Move Initialization
Understanding the distinction between copy and move initialization is vital in modern C++. Moving resources can optimize performance and reduce the overhead of copying. Here’s how both appear:
std::string str1 = "Hello"; // Copy initialization
std::string str2 = std::move(str1); // Move initialization
A clear understanding of when to use each concept can drastically affect your program's efficiency, especially when dealing with dynamic memory and temporary objects.
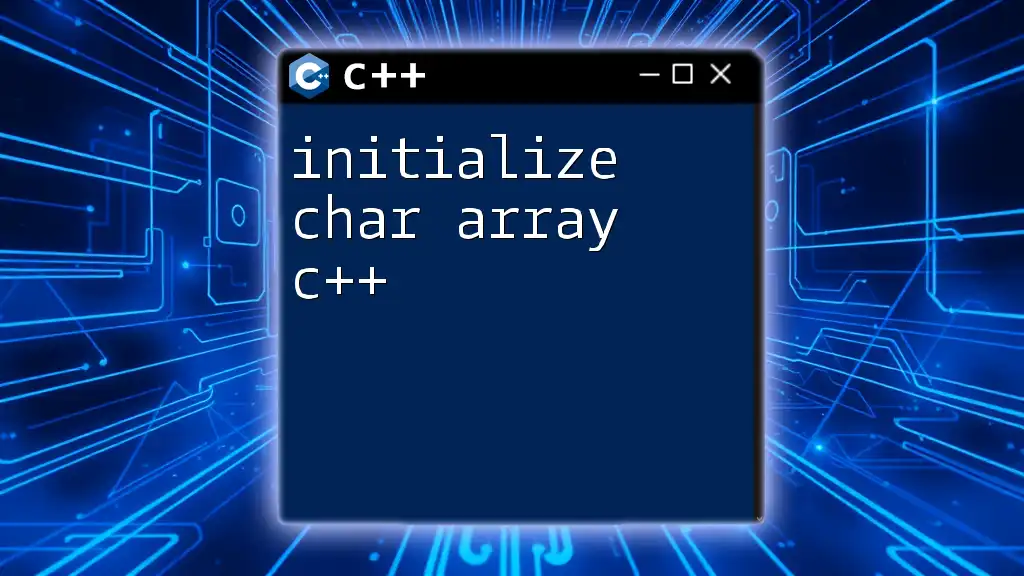
Conclusion
Understanding initializers in C++ is essential for any programmer looking to write efficient, maintainable, and safe code. By mastering the various initialization methods and incorporating best practices, you can prevent common pitfalls associated with uninitialized variables and make informed decisions about your coding style. Practice regularly with these concepts, and explore further into C++ to enhance your programming skills.