A BigInteger in C++ represents an integer type that can handle values larger than the typical data types (like `int` or `long long`), allowing for operations on numbers that exceed standard limits.
Here's a simple example of how you might implement a BigInteger class in C++:
#include <iostream>
#include <vector>
#include <string>
class BigInteger {
public:
BigInteger(const std::string& number) {
for (char digit : number) {
digits.push_back(digit - '0'); // Convert char to int
}
}
void display() const {
for (int digit : digits) {
std::cout << digit;
}
std::cout << std::endl;
}
private:
std::vector<int> digits; // Store digits in a vector
};
int main() {
BigInteger bigNum("123456789012345678901234567890");
bigNum.display(); // Output the big integer
return 0;
}
This example demonstrates the construction of a BigInteger class that can handle very large numbers using a vector to store individual digits.
What is BigInteger?
BigInteger, or biginteger c++, refers to a data type that allows the storage and manipulation of numbers significantly larger than those supported by standard integer types in C++. As C++ offers limited integer types (like `int`, `long`, and `long long`), which can only handle a certain range of values, BigIntegers become essential for applications requiring high-precision arithmetic, such as cryptography and scientific computations.
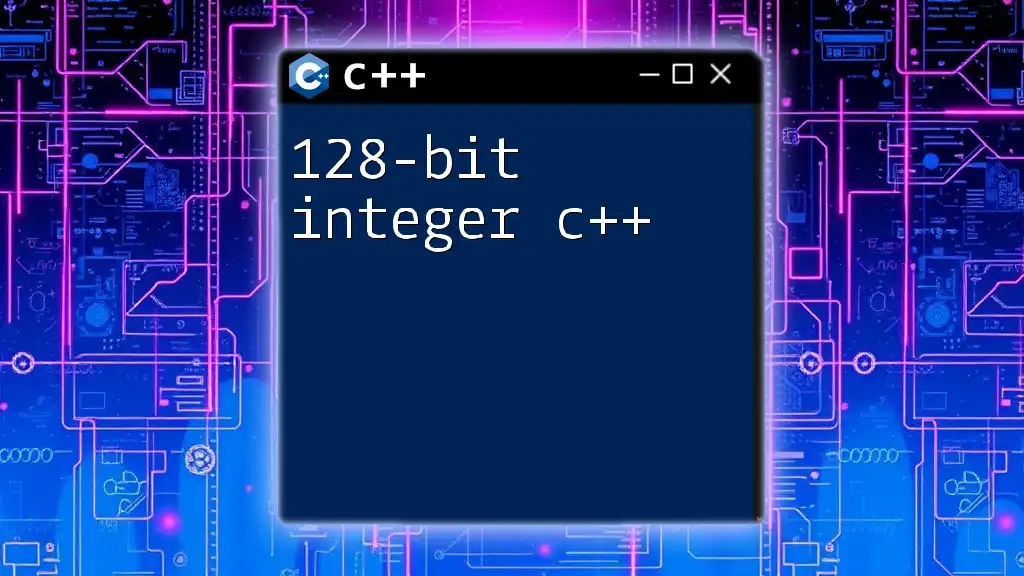
Why Use BigInteger in C++?
In programming, one often encounters integers that exceed standard data type limits. For example, when dealing with cryptographic keys or computations involving very large factorials, one must manipulate numbers far larger than commonly supported by C++. A `long long` type may allow values up to approximately 19 quintillion, but what if you need to calculate something like `100!`? This is where biginteger c++ shines, offering the ability to seamlessly work with virtually limitless integers.
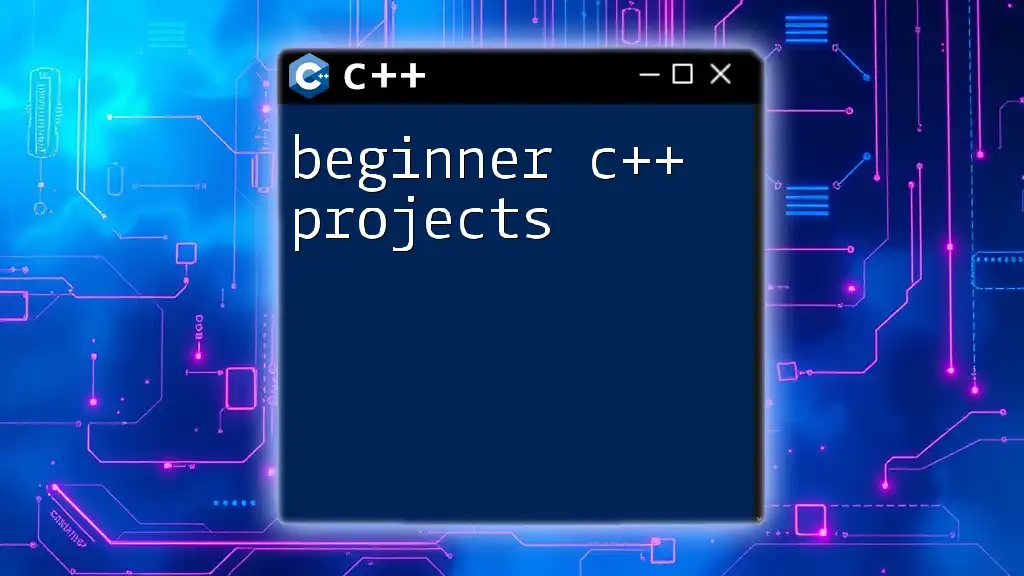
Understanding the Basics of Big Integers
Fundamental Concepts
What is a BigInteger Data Type?
BigInteger significantly expands the capacity of numeric types. It is not a built-in type in C++, but rather a user-defined type implemented through appropriate data structures, typically strings or arrays. This custom type enables operations on extremely large numbers that standard integer types cannot support.
How BigIntegers Represent Large Numbers
BigInteger usually employs a string or an array to represent digits of the number. This allows storage of any number of digits and performs arithmetic operations based on these representations. When adding two BigIntegers, for instance, the algorithm proceeds digit by digit, just like how one would add numbers manually on paper.
BigInteger Libraries Available in C++
Popular Libraries for BigInteger
Several libraries facilitate the implementation of `BigInteger` in C++. Two of the most popular libraries are:
-
Boost.Multiprecision: A powerful library that allows for arbitrary precision arithmetic. It seamlessly integrates with various numeric types and provides excellent performance.
-
GMP (GNU Multiple Precision Arithmetic Library): Offers an extensive set of operations for calculating large numbers with efficient algorithms and data structures.
Each library has its unique advantages, and the choice may depend on the application's specific requirements and performance considerations.
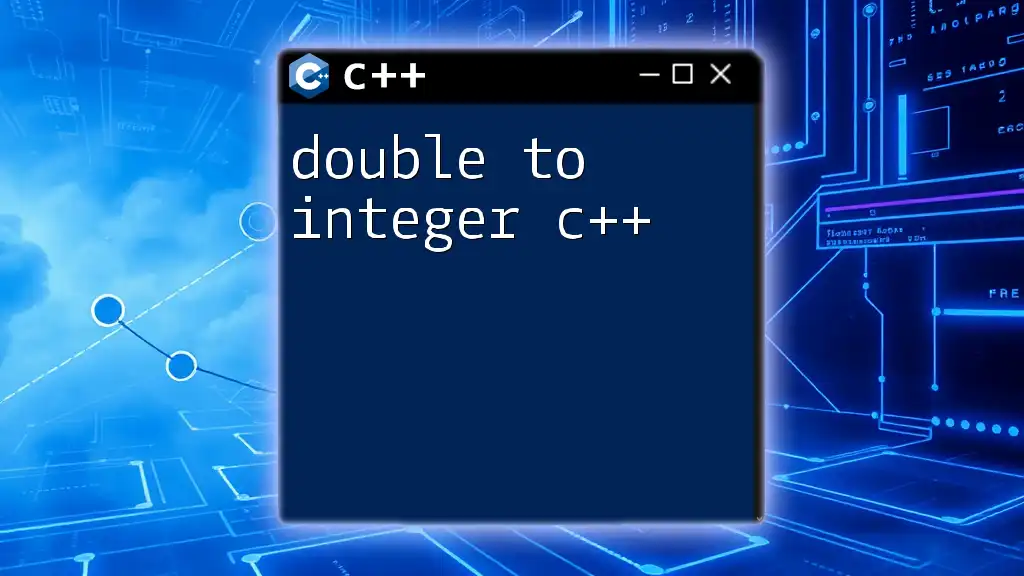
Implementing BigInteger in C++
Setting Up Your Development Environment
Installing Necessary Libraries
To begin working with a BigInteger in C++, you first need to install the required libraries. For Boost, you can download it from [Boost's official website](https://www.boost.org/) or install it using a package manager like `vcpkg`. For GMP, installation can similarly be performed via a package manager or from the [GMP website](https://gmplib.org/).
Once installed, ensure you link your project to these libraries correctly, setting up your compiler options accordingly.
Creating Your Own BigInteger Class
Defining the BigInteger Class
Let’s create a simple `BigInteger` class. This class will encapsulate a number as a string.
class BigInteger {
private:
std::string number; // Member variable for storage
public:
BigInteger(const std::string &num) : number(num) {}
// Method declarations for operations...
};
In this snippet, we define a constructor that initializes the `number` variable using a string.
Constructor and Basic Methods
The constructor allows initializing the `BigInteger` object with a value. To enhance functionality, we can implement methods for key arithmetic operations, comparisons, and conversions.
Arithmetic Operations with BigInteger
Addition
Implementing addition for two `BigInteger` instances requires iteratively summing the digits from the least significant to the most significant.
BigInteger operator+(const BigInteger &other) {
std::string result; // This will hold the result
int carry = 0;
// Your code to add each digit and handle carries...
return BigInteger(result);
}
This code snippet demonstrates how to initiate the addition. The implementation should address digit-by-digit addition, handling carries appropriately.
Subtraction
Subtracting two `BigInteger` instances follows a similar principle, but with careful management of borrows.
BigInteger operator-(const BigInteger &other) {
// Logic for subtraction...
}
Again, a thorough digit-by-digit subtraction logic would need to be crafted.
Multiplication
For multiplication, consider an efficient algorithm such as the Karatsuba algorithm, which splits numbers into parts to reduce the number of necessary multiplications.
BigInteger operator*(const BigInteger &other) {
// Logic for multiplication...
}
This will involve recursive multiplication of the sub-parts.
Division
Division necessitates the implementation of long division manually, which will be more complex than other operations.
BigInteger operator/(const BigInteger &other) {
// Logic for division...
}
It's essential to ensure that the implementation correctly handles cases like division by zero.
Comparison Operations
Implementing Comparison Operators
Comparison operators (`<`, `>`, `==`, etc.) can be overloaded to allow direct comparison of `BigInteger` instances:
bool operator<(const BigInteger &other) {
// Logic for comparison based on string length and actual digits...
}
Continuing this way, other comparison operations may be implemented similarly.
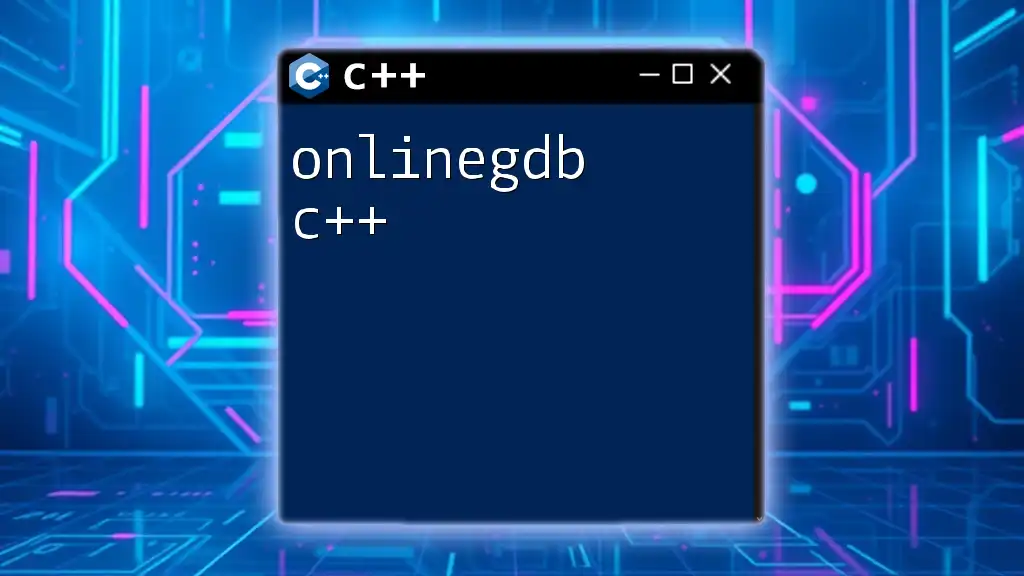
Practical Examples of BigInteger Usage
Real-World Applications
Cryptography
`BigInteger` plays a pivotal role in various cryptographic algorithms, such as RSA, where keys and operands can be extremely large. Using `BigInteger`, developers can perform necessary mathematical operations on these large values securely.
Scientific Computations
In scientific work, researchers frequently encounter vast numbers that require precision. The factorial of a number like 100, for example, results in 158 digits, underscoring the necessity of a BigInteger to store and compute such results.
Example Calculation Using BigInteger
Example: Calculating Factorials
A common application is calculating large factorials using `BigInteger`. Here’s a basic function to accomplish this:
BigInteger factorial(int n) {
BigInteger result("1");
for (int i = 2; i <= n; ++i) {
result = result * BigInteger(std::to_string(i));
}
return result;
}
This function iterates from `2` to `n`, multiplying the result by each integer converted to `BigInteger`.
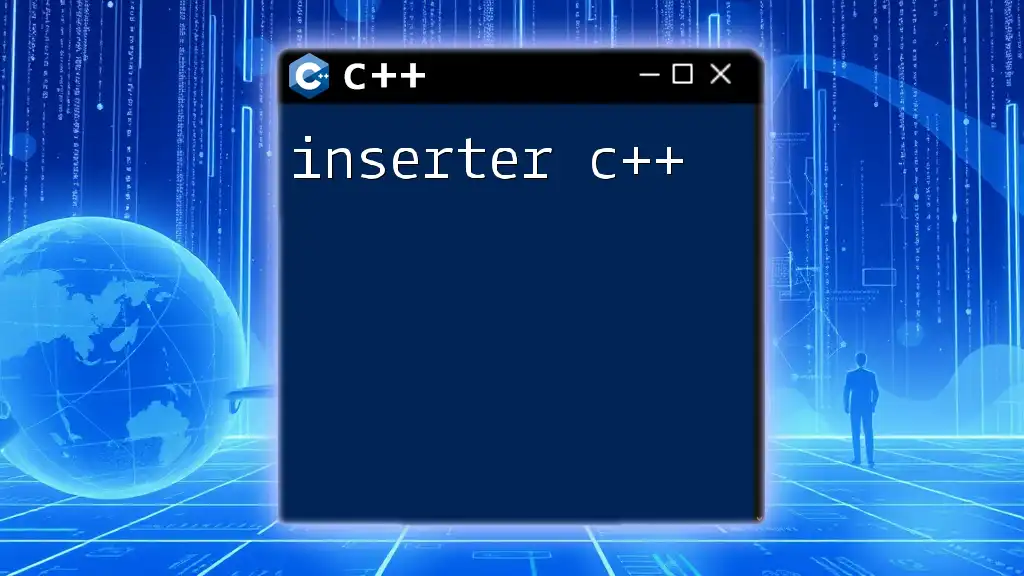
Conclusion
In summary, biginteger c++ is an essential concept for handling operations on very large numbers that exceed standard data types. By understanding the underlying principles of BigInteger and leveraging libraries or implementing custom classes, developers can tackle complex problems in cryptography, scientific fields, and beyond.
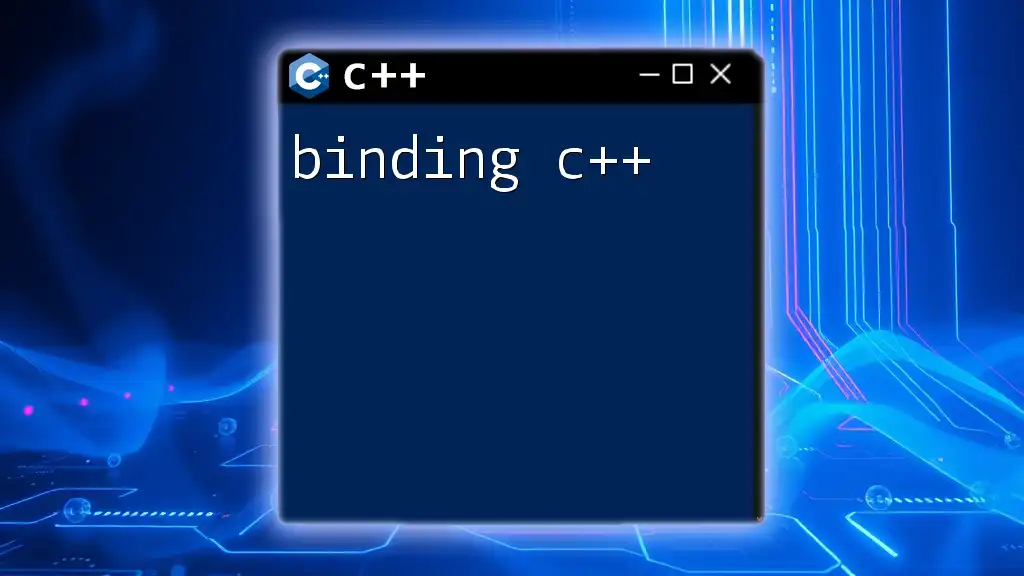
Further Resources
To deepen your knowledge, consider exploring the documentation for libraries like Boost.Multiprecision and GMP. Tutorials and user guides are invaluable for mastering advanced operations and techniques.
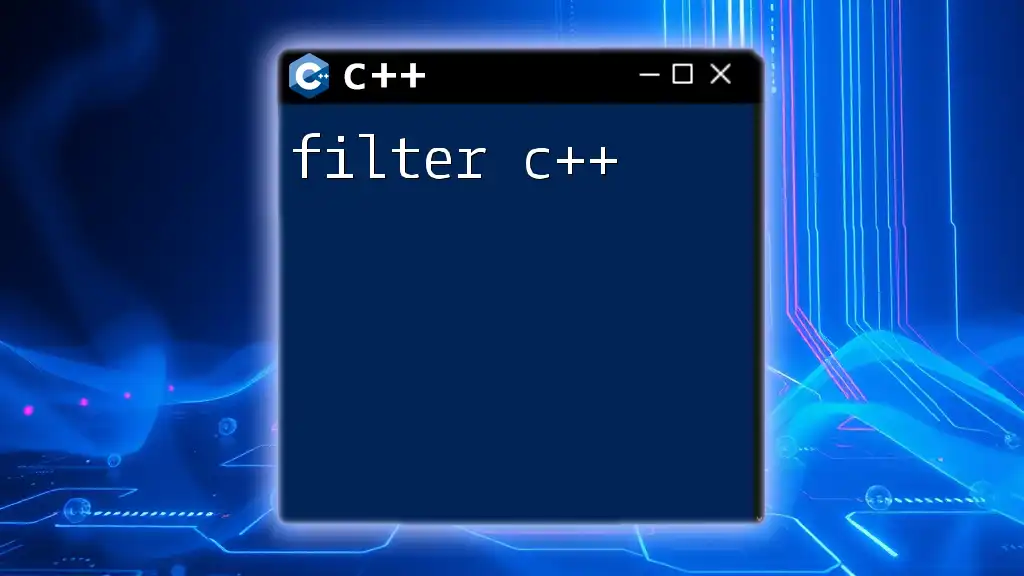
Call to Action
Start your journey with BigInteger in C++ today! Experiment with the code snippets provided and consider downloading sample projects from GitHub to further enhance your understanding and implementation skills.