A double pointer in C++ is a pointer that points to another pointer, allowing for dynamic memory management and multidimensional array access.
Here’s a simple example:
#include <iostream>
int main() {
int value = 42;
int *ptr = &value; // A pointer to an integer
int **doublePtr = &ptr; // A double pointer that points to the pointer
std::cout << "Value: " << **doublePtr << std::endl; // Output: Value: 42
return 0;
}
Understanding Pointers in C++
What is a Pointer?
A pointer in C++ is a variable that stores the memory address of another variable. It acts as a reference to another location in memory, making it essential for dynamic memory management and efficient data handling. Understanding pointers is fundamental for advanced C++ programming.
Types of Pointers
There are several types of pointers you'll encounter in C++:
- Single pointers: Point to a single variable.
- Null pointers: Initialize to `nullptr` or `NULL`, indicating they point to nothing.
- Void pointers: Can point to any data type but need to be typecast before dereferencing.
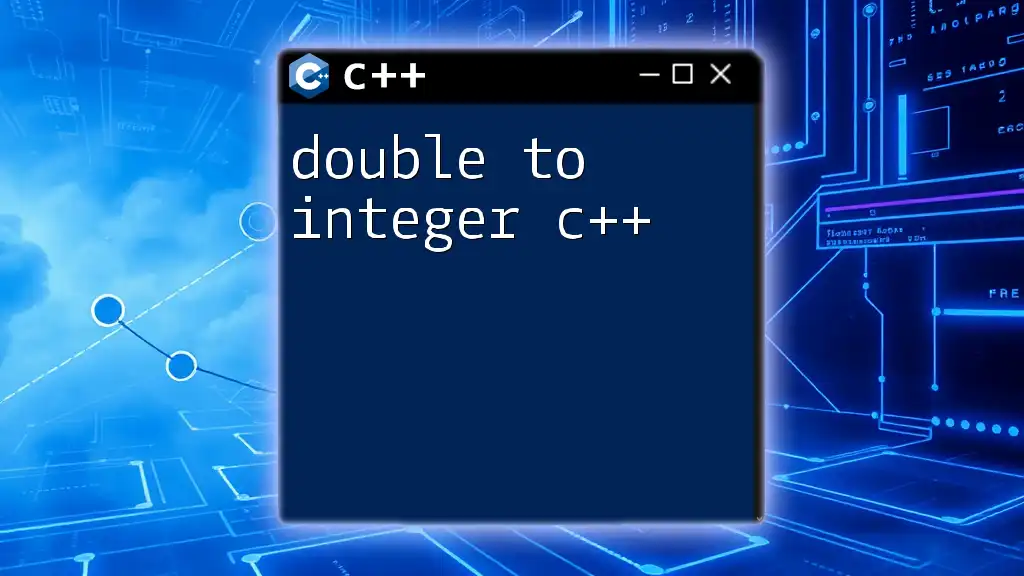
Introduction to Double Pointers
What is a Double Pointer?
A double pointer is a pointer that points to another pointer. Denoted by two asterisks (e.g., `int**`), it allows developers to work with multiple pointers to dynamically allocated arrays or multi-dimensional data structures.
Why Use Double Pointers?
Double pointers are especially useful in various scenarios:
- Managing 2D arrays or matrices where you wish to have a pointer to the pointer of the first element.
- Passing a pointer to a pointer to modify the address that the original pointer holds within a function.
- Facilitating dynamic memory allocation for structures requiring multiple pointers.
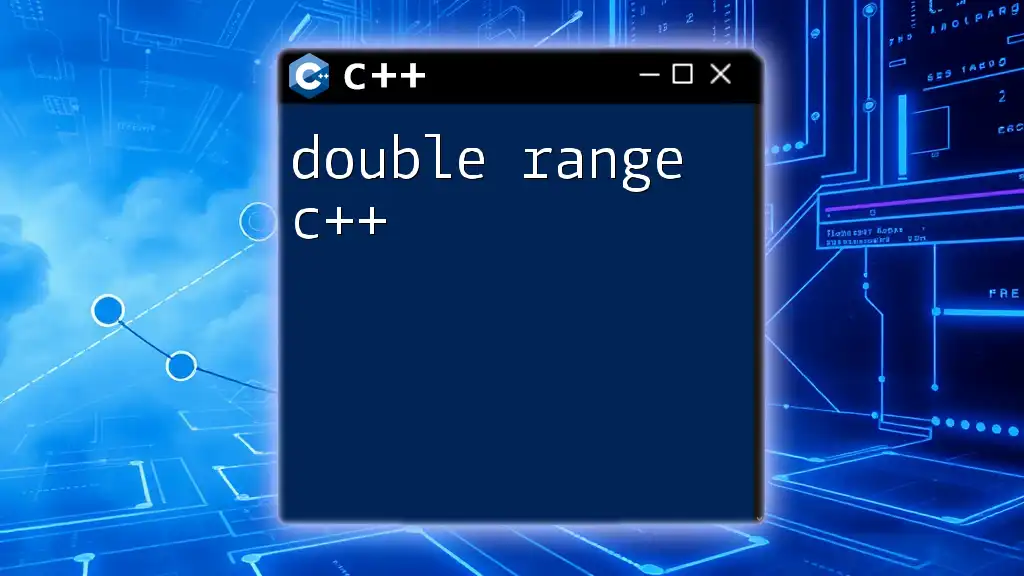
Syntax and Declaration of Double Pointers
Declaring a Double Pointer
To declare a double pointer, you simply append another asterisk to the pointer type, as shown in the syntax below:
int** ptr;
In this declaration, `ptr` is now a pointer that can point to another pointer that points to an integer.
Initializing Double Pointers
Double pointers can be initialized in various ways. Here is a basic setup:
int var = 5; // Regular integer
int* ptr1 = &var; // Pointer to the integer
int** ptr2 = &ptr1; // Double pointer to ptr1
- In this example, `var` holds the integer 5.
- `ptr1` stores the address of `var`.
- `ptr2` now holds the address of `ptr1`, making it a double pointer.
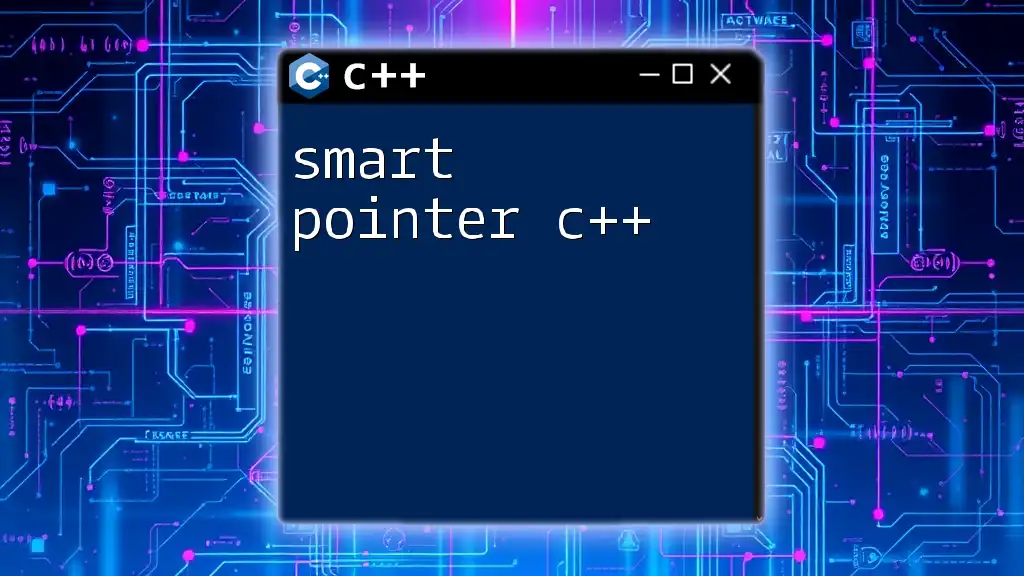
Memory Management with Double Pointers
Dynamic Memory Allocation
Double pointers are commonly used for dynamic memory allocation in multi-dimensional arrays. For example, to allocate memory for a 2D array:
int rows = 3;
int cols = 4;
int** arr = new int*[rows]; // Allocate memory for array of pointers
for(int i = 0; i < rows; i++)
arr[i] = new int[cols]; // Allocate memory for each row
In the above example, `arr` is a double pointer that points to dynamically allocated memory for an array of `int*`, which in turn points to integer arrays.
Deallocating Memory
It is crucial to manage memory effectively to prevent leaks. To deallocate memory allocated for double pointers, you must delete each row and then the array of pointers:
for(int i = 0; i < rows; i++)
delete[] arr[i]; // Deallocate each row
delete[] arr; // Deallocate the array of pointers
This ensures all dynamically allocated memory is appropriately released.
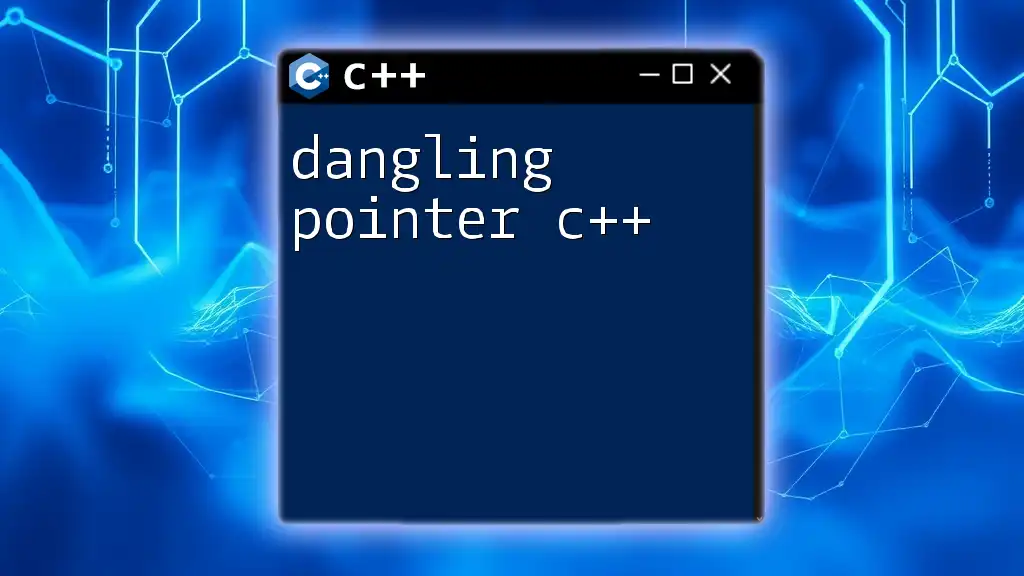
Accessing Values Using Double Pointers
Dereferencing Double Pointers
Accessing the values stored at a double pointer is done through dereferencing. The syntax involves using two asterisks:
**ptr2 = 10; // Sets the value of var to 10
- In this case, the value that `ptr2` points to (which is `ptr1`) is dereferenced to alter `var` directly.
Practical Example
The following example showcases how to use double pointers to modify values in a function:
void updateValue(int** ptr) {
**ptr = 20; // Update the value that ptr points to
}
int main() {
int var = 10; // Initialize an integer
int* ptr1 = &var; // Pointer to var
updateValue(&ptr1); // Pass the address of ptr1
// var is now 20
}
In this example, the function `updateValue` modifies `var` via the double pointer, illustrating how double pointers can facilitate modifications across function boundaries.
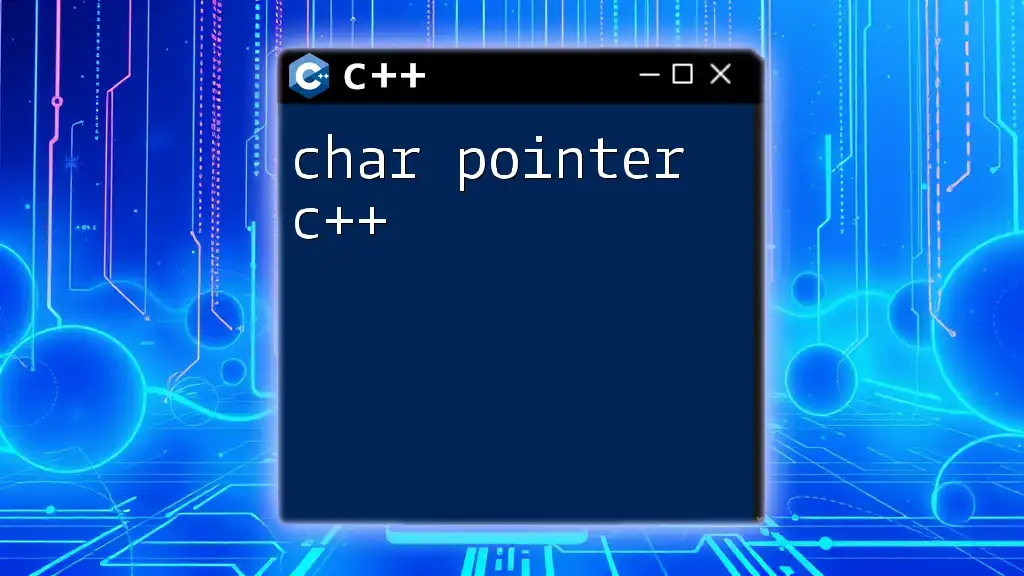
Common Mistakes with Double Pointers
Misunderstanding Dereferencing Levels
When working with double pointers, a common pitfall is misunderstanding dereferencing levels. It's essential to carefully consider how many times you need to dereference. In many instances, programmers forget that they need to dereference twice to access the actual value.
Memory Leaks
Improper management of double pointers can lead to memory leaks. It is vital to `delete` all allocated memory to avoid using up system resources unnecessarily. Always ensure that for every `new`, there is a corresponding `delete` to maintain memory integrity.
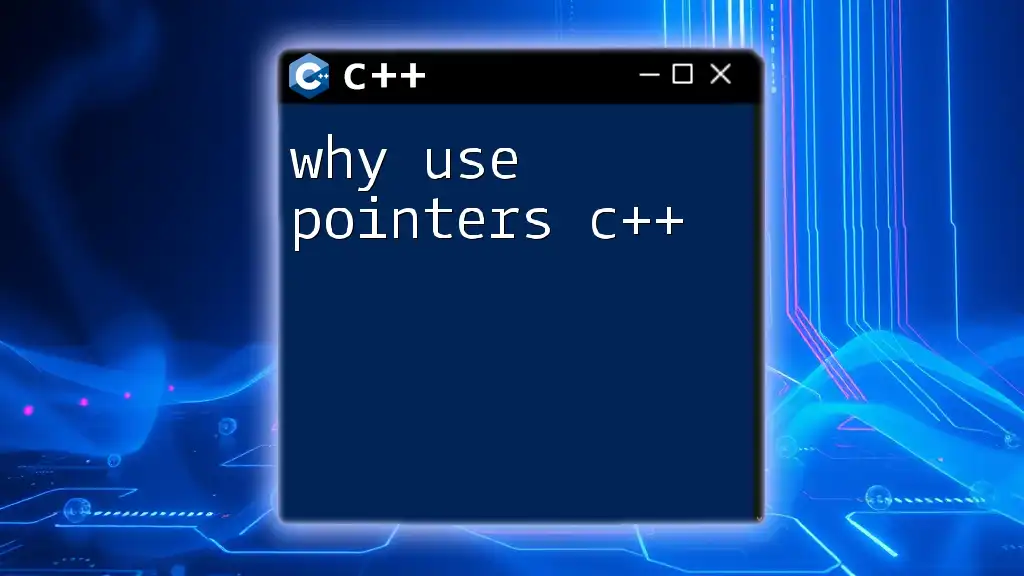
Conclusion
Recap of Double Pointers
In summary, double pointers play a critical role in handling complex data structures and dynamic memory. They empower programmers to manage multi-dimensional arrays, facilitate inter-function pointer management, and optimize memory usage.
Final Thoughts
Mastering the concept of double pointers and their implementation is crucial for enhancing your C++ programming skills. Regular practice, along with tackling complex problems, will significantly strengthen your understanding and application of double pointers in various programming scenarios.
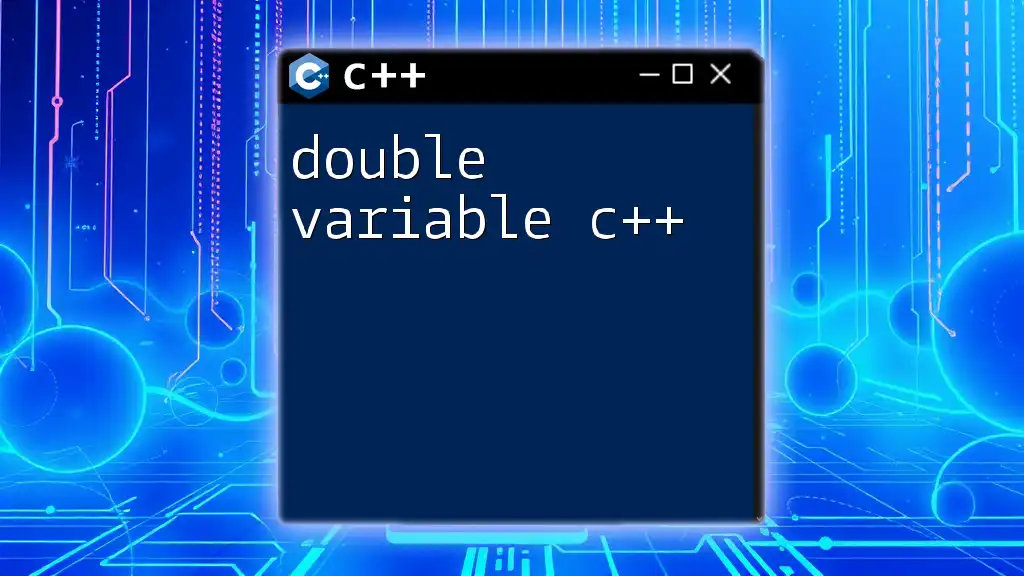
Additional Resources
References
For further reading and deepening your knowledge about double pointers and C++, refer to trusted online documentation, tutorials, and community forums.
Community and Support
Engage with forums and groups dedicated to C++ programming to share insights, ask questions, and collaborate with fellow programmers on mastering complex topics like double pointers.