The `readfile` function in C++ allows you to open and read the contents of a file efficiently, as shown in the following code snippet.
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example.txt");
std::string line;
while (std::getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
return 0;
}
Understanding File Streams in C++
What is File I/O?
Input/Output (I/O) operations are fundamental to programming, allowing applications to communicate with external files. This interaction is crucial for tasks such as storing user data, reading configuration settings, or processing large datasets. Learning how to efficiently utilize file I/O can significantly enhance your application's performance and functionality.
C++ File Streams
In C++, file handling is primarily facilitated through file streams. Understanding these streams enables developers to perform various file operations seamlessly. The primary classes that you will encounter when handling files in C++ include:
- `ifstream`: Used for input file streams, enabling you to read from files.
- `ofstream`: Used for output file streams, suitable for writing to files.
- `fstream`: A combination of both, allowing you to read and write data to the same file.
By utilizing these classes, you can manipulate files in a straightforward manner, leveraging the power of C++ for effective file management.
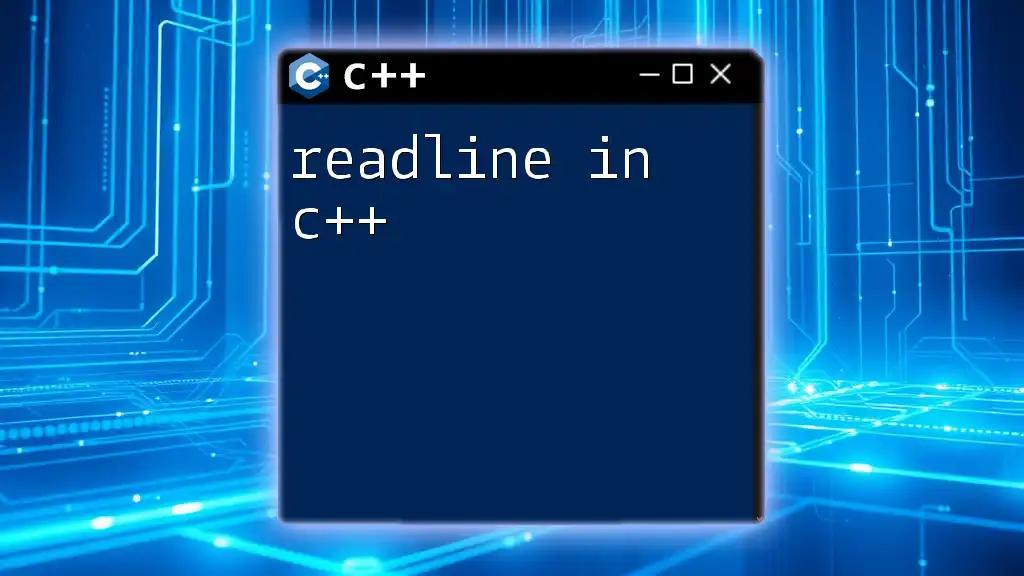
Setting Up Your Development Environment
Required Tools and Libraries
To begin learning about how to read files in C++, you need a development environment. Popular choices include:
- Visual Studio: A comprehensive IDE that supports various languages, including C++. Ideal for beginners due to its user-friendly interface.
- Code::Blocks: An open-source IDE that allows for easy code organization and management.
Make sure to have the C++ compiler installed (such as GCC or MSVC) as it will be essential for compiling and running your C++ programs.
Including Necessary Headers
Before you dive into file operations, remember to include the necessary header files at the beginning of your C++ program. The essential headers for file handling are as follows:
#include <iostream>
#include <fstream>
#include <string>
These headers provide the necessary functionalities for standard input/output operations, file handling, and string manipulations.

The `readfile` Functionality
Opening a File
To open a file for reading, you will utilize the `ifstream` class. The syntax is quite simple but essential for successful file reading:
std::ifstream inputFile("example.txt");
if (!inputFile.is_open()) {
std::cerr << "Error opening file!" << std::endl;
}
This code snippet opens a file named `example.txt` and checks if the file is successfully opened. If it fails, an error message is printed to the console, helping you debug issues promptly.
Reading Data from a File
Once you have opened a file, you can proceed to read its contents. There are a few different methods for reading data, two of the most common being line-by-line reading and word-by-word extraction.
Reading Line by Line
Reading files line by line can be conveniently done using `getline()`. This method is particularly useful for text files, where each line contains separate pieces of information. Here’s a code example:
std::string line;
while (std::getline(inputFile, line)) {
std::cout << line << std::endl;
}
Each time `getline()` is called, it reads up to the newline character and stores the line in the variable `line`. This code prints each line from the file until the end is reached.
Reading Word by Word
If your aim is to read space-separated words from a file, you can utilize the extraction operator (`>>`). Here’s how to implement it:
std::string word;
while (inputFile >> word) {
std::cout << word << std::endl;
}
This snippet reads words directly from the file and outputs them until there are no more words to read.
Closing a File
After finishing the file operations, it’s important to close the file to release system resources. This can be done swiftly using:
inputFile.close();
Always ensure you close your files to avoid memory leaks and other potential issues in your application.

Error Handling in File Operations
Common Errors and How to Handle Them
When working with files, you'll encounter several common issues, such as file not found or permission denied. It’s crucial to incorporate error handling to make your application robust. Here’s an example of how to handle a file opening error:
try {
std::ifstream inputFile("nonexistent.txt");
if (!inputFile) {
throw std::ios_base::failure("File not found");
}
} catch (std::exception& e) {
std::cerr << e.what() << std::endl;
}
This example uses a `try-catch` block to catch exceptions. If the file fails to open, an exception is thrown, and a descriptive error message is displayed.
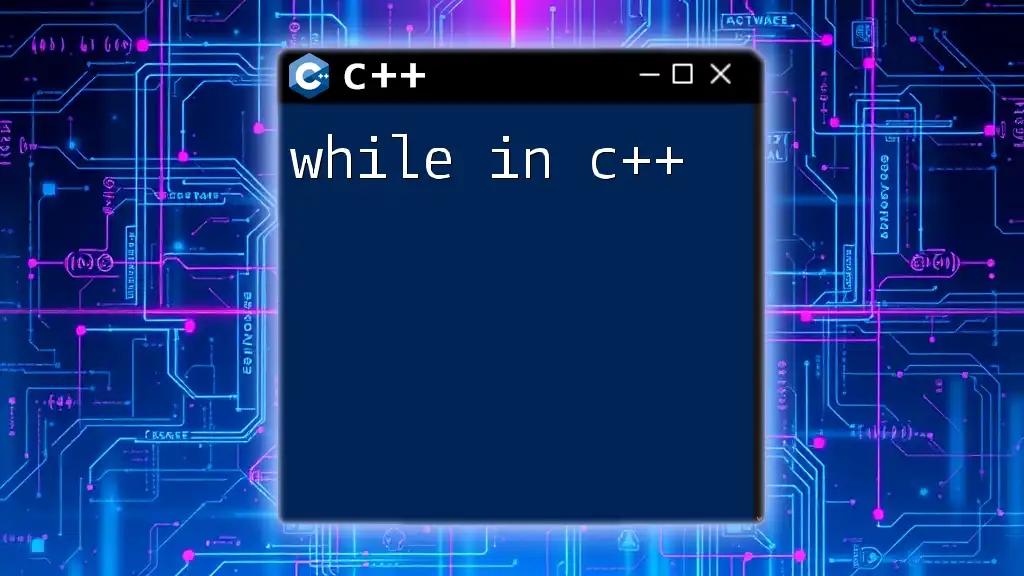
Practical Examples
Example 1: Reading a Text File
Let’s see a practical implementation of how to read a text file and count the number of lines present:
int countLines(const std::string& filename) {
std::ifstream inputFile(filename);
std::string line;
int counter = 0;
while (std::getline(inputFile, line)) {
counter++;
}
return counter;
}
In this function, we open the specified file, read it line by line, and count each line. The function returns the total count of lines, which could be useful in various contexts.
Example 2: Reading Configuration Files
Another common use case involves reading configuration files containing key-value pairs. Here's how you can achieve that:
std::map<std::string, std::string> readConfig(const std::string& filename) {
std::ifstream inputFile(filename);
std::string key, value;
std::map<std::string, std::string> config;
while (inputFile >> key >> value) {
config[key] = value;
}
return config;
}
This function reads a configuration file and stores its key-value pairs into a `std::map`. This is a practical application for many software applications that rely on configuration files.

Best Practices for File I/O in C++
Managing Resources
Following best practices in resource management is vital. Utilizing Resource Acquisition Is Initialization (RAII) helps bind the lifetime of resources (like file handlers) to object lifetimes, improving stability and maintainability of your code. In modern C++, prefer using smart pointers and RAII techniques to manage resources effectively.
Performance Considerations
When reading large files, consider optimizing your reading strategies. Techniques such as buffering or reading files in chunks can help improve performance significantly compared to reading byte by byte or line by line indiscriminately.

Conclusion
Mastering readfile in C++ is a gateway to effective file management in your applications. The ability to handle file I/O proficiently will enable you to build more dynamic and user-friendly software. Practice regularly to enhance your skills and consider exploring additional resources for in-depth understanding.
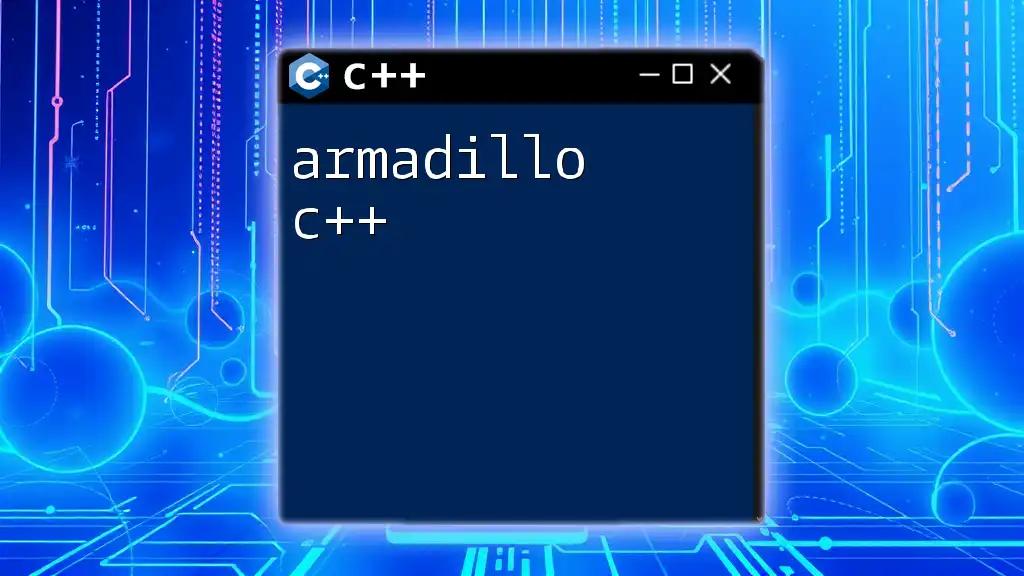
Additional Resources
To further enhance your learning, check out recommended books on C++ programming, online tutorials, and forums. Engaging with the community can provide invaluable insights and support as you develop your file handling skills.
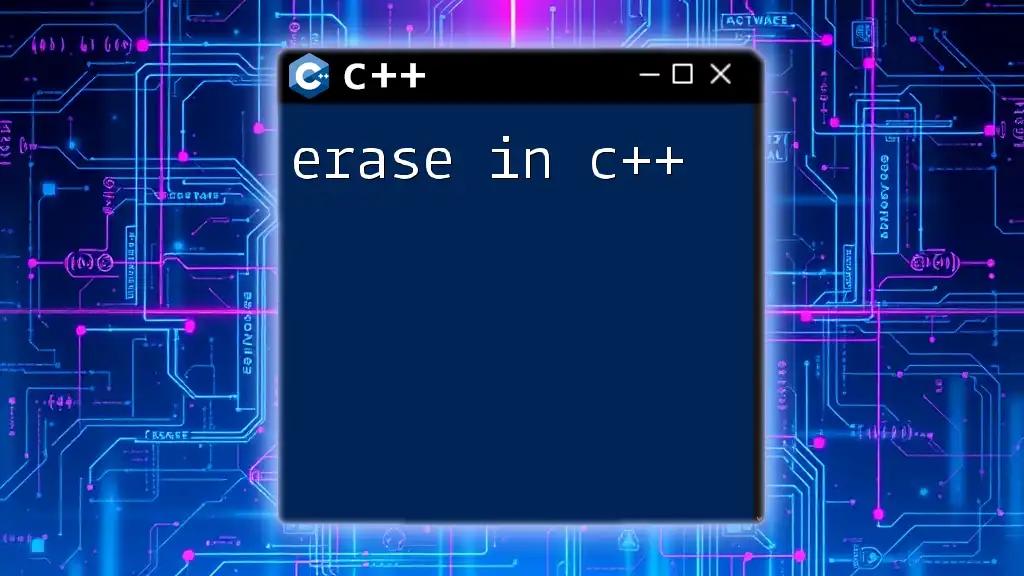
Call to Action
If you have any questions or comments about reading files in C++, feel free to leave them below! Also, consider sharing this article with fellow learners who might benefit from understanding file I/O in C++. Happy coding!