Literals in C++ are constant values directly represented in the code, such as integers, floating-point numbers, characters, and strings.
int integerLiteral = 42; // Integer literal
float floatLiteral = 3.14f; // Floating-point literal
char charLiteral = 'A'; // Character literal
const char* stringLiteral = "Hello"; // String literal
Understanding C++ Literals
Definition of Literals
In C++, literals are fixed values that appear directly in your code. Unlike variables, which can hold different values over their lifetime, literals represent constant data that does not change. Whenever you see something like `42`, `"Hello, World!"`, or `3.14` in your code, you're dealing with literals. Understanding how to use them effectively is crucial for writing clean and efficient code.
Types of Literals
Integral Literals
Integral literals represent whole numbers and can be expressed in various numeral systems.
- Decimal Literals: The most straightforward representation. For example:
int a = 5;
- Octal Literals: Represented by starting the number with a `0`, valid digits are `0-7`.
int b = 07; // This assigns the decimal value 7
- Hexadecimal Literals: Starting with `0x` or `0X`, these can include digits `0-9` and letters `A-F`.
int c = 0x1A; // This assigns the decimal value 26
Each integral literal can also have suffixes to denote type, such as `U` for unsigned and `L` for long.
Floating-Point Literals
Floating-point literals are used for decimal or fractional numbers.
- Standard Notation: These can represent numbers with a decimal point.
float pi = 3.14;
- Scientific Notation: A more compact form useful for expressing very large or small numbers:
double e = 2.718e1; // Represents 2.718 x 10^1, which is 27.18
Remember that floating-point literals can also have suffixes like `f` for float (e.g., `3.14f`) and `l` for long double.
Character Literals
Character literals are individual characters enclosed in single quotes and can also include escape sequences to represent special characters.
- Basic character example:
char letter = 'A';
- Escape sequences allow for non-printable characters.
char newLine = '\n'; // Represents a newline character
String Literals
String literals consist of a sequence of characters enclosed in double quotes. They are widely used for text manipulation and display.
- A basic string could look like this:
std::string greeting = "Hello, World!";
- C++ also allows the use of raw string literals for text that spans multiple lines:
std::string longText = R"(This is a long text that spans multiple lines.)";
Boolean Literals
Boolean literals represent truth values, either `true` or `false`. They play a critical role in control flow and conditions.
bool isCodingFun = true;
This simple declaration uses the boolean literal `true` to set the variable `isCodingFun`.
Pointer Literals
In C++, a pointer literal can represent a null pointer using `nullptr`, which indicates that the pointer does not point to any object or function.
int* ptr = nullptr; // The pointer 'ptr' is initialized to null

User-Defined Literals
What are User-Defined Literals?
User-defined literals allow developers to create custom literals that enhance readability and expressiveness in code. This feature adds flexibility by allowing specific suffixes that convert literals into more meaningful types.
How to Create User-Defined Literals
Creating user-defined literals involves defining an operator function that specifies the conversion.
For instance, you can create a literal for measuring distance:
constexpr long double operator"" _km(long double km) {
return km * 1000; // Converts kilometers to meters
}
// Usage
long double distance = 5.0_km; // This is interpreted as 5000.0 meters
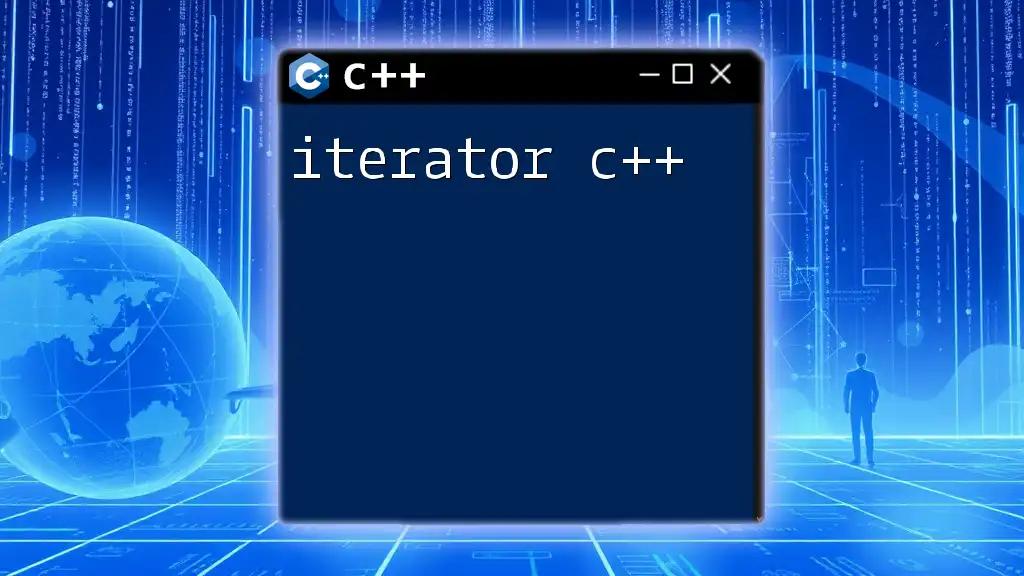
Characteristics of C++ Literals
Immutability of Literals
One crucial aspect of literals is their immutability; once defined, you cannot change them. Attempting to modify a literal will result in a compilation error, emphasizing the need for variables when mutable data is required.
Type Inference and Literals
C++ has a powerful type inference mechanism that automatically deduces the type of a literal during initialization. For example:
auto num = 42; // 'num' is inferred to be of type int
Comprehensive Rules for Literals
When working with literals, several rules apply:
- Integral types have a range based on the type specified (e.g., `int`, `long`).
- Suffixes are important for defining literal types explicitly.
- Floating-point behavior can differ significantly based on precision and type.

Best Practices When Using Literals
Clarity and Readability
Using literals correctly can enhance the clarity of your code. Making your literals clear and understandable helps others read and maintain your code with ease. For example, instead of directly using raw values, refer to well-named constants to give context.
Avoiding Magic Numbers
One best practice in programming is avoiding magic numbers, which are hard-coded values that appear without explanation. Instead, define constants for such values to improve readability and maintainability.
// Instead of using a hard-coded number:
int daysInYear = 365; // Magic number
// Use a constant:
const int DAYS_IN_YEAR = 365; // Clear and maintainable
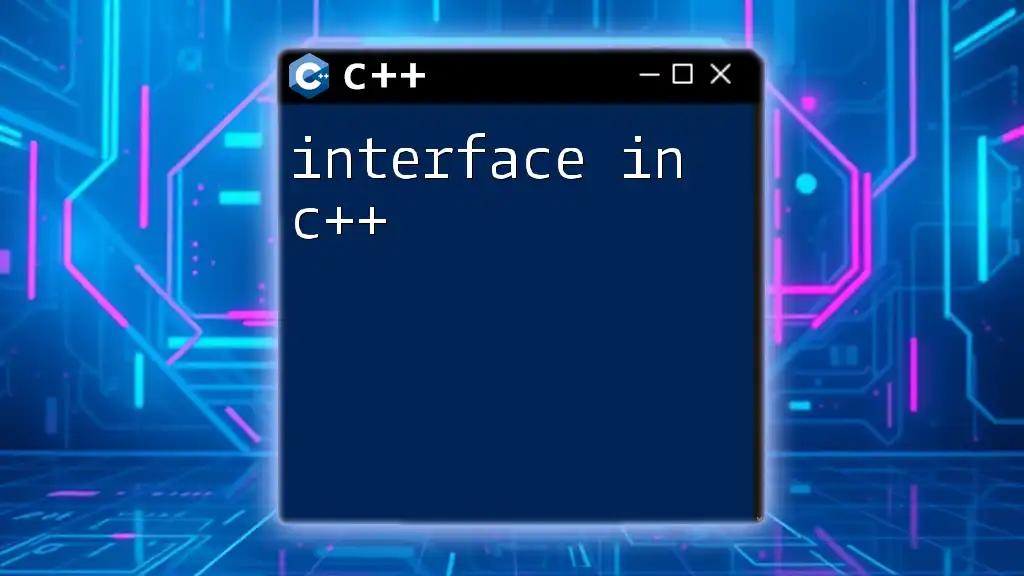
Conclusion
Understanding literals in C++ is fundamental for every programmer, as they represent the foundational fixed values used across programs. From integral and floating-point literals to user-defined literals, mastering how to work with these constant values will significantly impact the clarity, maintainability, and functionality of your code. By implementing best practices, you can ensure that your code remains clean, efficient, and easy to understand.