In C++, the asterisk (*) is used as both a dereference operator for pointers and to define pointers in variable declarations.
int main() {
int value = 10; // An integer variable
int *ptr = &value; // A pointer that holds the address of value
int dereferencedValue = *ptr; // Dereferencing ptr to access the value
return 0;
}
Understanding the Asterisk: Basic Functions
Definition of Asterisk in C++
The asterisk (`*`) is a powerful and versatile symbol in C++. It plays crucial roles in memory management, pointing to variables, and manipulating data structures. Understanding how and where to use the asterisk in C++ is fundamental for efficient programming.
Asterisk as a Pointer Operator
What are pointers? Pointers are variables that store memory addresses of other variables. They are essential for accessing and manipulating data in dynamic memory, as well as for passing variables to functions by reference.
Using the Asterisk to Define Pointers To declare a pointer in C++, you use the asterisk along with the data type. For example, if you want to create a pointer to an integer, the syntax is straightforward:
int* ptr; // Pointer to an integer
In this declaration, `ptr` is a pointer that can point to an integer variable. It does not hold an integer value itself but rather the address of an integer.
Dereferencing Pointers with Asterisk Dereferencing is the process of accessing the value at the address a pointer is pointing to. This is done using the asterisk operator. Here’s how you can dereference a pointer:
int value = 5;
int* ptr = &value; // ptr holds the address of value
std::cout << *ptr; // Outputs 5
In this example, `*ptr` fetches the value located at the memory address that `ptr` points to, which holds the integer value `5`. This illustrates how pointers can be used to reference and manipulate data stored in variables.
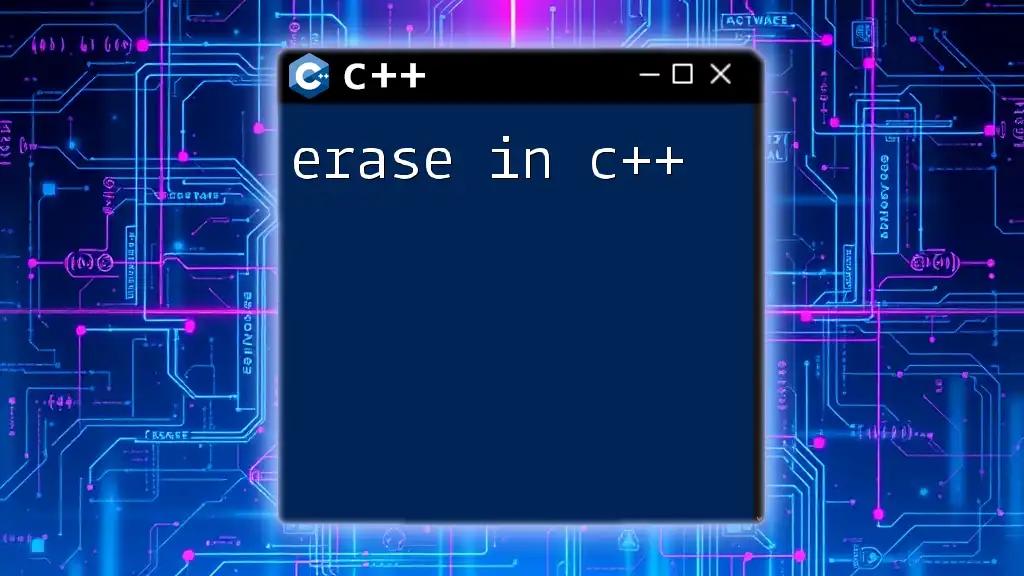
Asterisk in Function Parameters
Pass by Reference vs Pass by Value
In C++, when you pass parameters to a function, you can choose to pass by value or pass by reference. Passing by value creates a copy of the variable, whereas passing by reference allows the function to modify the original variable.
Using Asterisk to Pass Pointers to Functions
One common use of the asterisk is passing pointers to functions. This allows functions to alter the original values of variables without needing to return them.
Consider this example of a function that increments the value of an integer through a pointer:
void increment(int* ptr) {
(*ptr)++; // Incrementing the value at the pointer
}
When you call this function, the pointer `ptr` gives it access to the variable's memory address.
Here’s how you would use this function in your `main`:
int main() {
int value = 10;
increment(&value);
std::cout << value; // Outputs 11
}
Using `&value` as the argument in the `increment` function gives access to the actual memory address of `value`, enabling the function to modify its content directly.
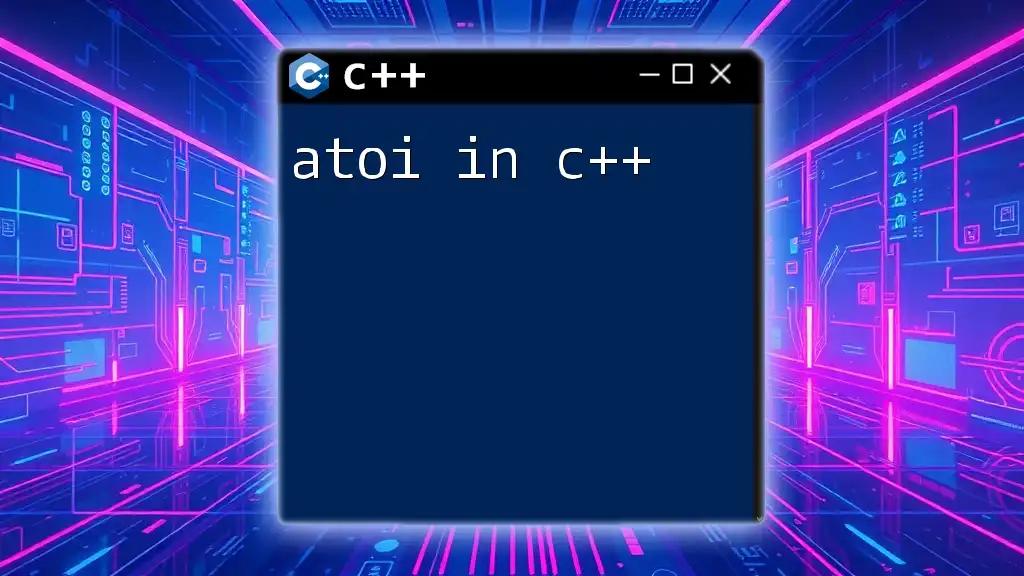
Asterisk in Dynamic Memory Allocation
Introduction to Dynamic Memory
Dynamic memory allocation allows you to allocate memory at runtime according to your needs. This is particularly useful in scenarios where the amount of data required is not known at compile time.
Using Asterisk with `new` Keyword
In C++, you can use the asterisk in conjunction with the `new` keyword to allocate memory dynamically.
To allocate a single integer:
int* ptr = new int; // Allocate memory for a single integer
In this statement, `ptr` is now pointing to a dynamically allocated integer in heap memory. You can store a value in that integer as follows:
*ptr = 42; // Assigning the value 42 to the allocated memory
To allocate an array of integers:
int* arr = new int[5]; // Allocate memory for an array of 5 integers
This line creates space in memory for five integers, and `arr` is a pointer to the first element of this array.
Remembering to Free Memory
It’s critical to free dynamically allocated memory to prevent memory leaks. This is done using the `delete` operator. Here’s how you can deallocate the memory:
delete ptr; // Delete single integer
delete[] arr; // Delete array of integers
In the above, `delete ptr` frees the memory allocated for a single integer, and `delete[] arr` does the same for the entire array.
![Understanding Literals in C++ [A Quick Guide]](/_next/image?url=%2Fimages%2Fposts%2Fl%2Fliterals-in-cpp.webp&w=1080&q=75)
Asterisk in Class and Object Manipulation
Pointers in Object-Oriented Programming
In object-oriented programming (OOP), pointers can be immensely useful for managing class instances. They allow for dynamic creation and manipulation of objects without being tied to stack memory.
Using Asterisk to Access Class Members
When working with class members, you can use pointers along with the asterisk operator to access and manipulate object attributes.
Here’s a brief example to illustrate this:
class Sample {
public:
int data;
};
Sample* obj = new Sample(); // Creating an object dynamically
obj->data = 20; // Using '->' with pointer to access data
std::cout << obj->data; // Outputs 20
In this example, `obj` points to a `Sample` object, created dynamically. The arrow operator `->` allows access to `data` efficiently.

Conclusion
Mastering the asterisk in C++ is essential for effective programming, particularly when dealing with pointers, dynamic memory, and object manipulation. Whether declaring pointers, manipulating variables via functions, or handling memory, understanding the various uses of the asterisk enriches your programming skills.
Engaging with practical examples and regularly practicing these concepts will solidify your fluency in using the asterisk in C++. As you move forward, experiment with more complex programs and scenarios to deepen your understanding of this fundamental aspect of C++.
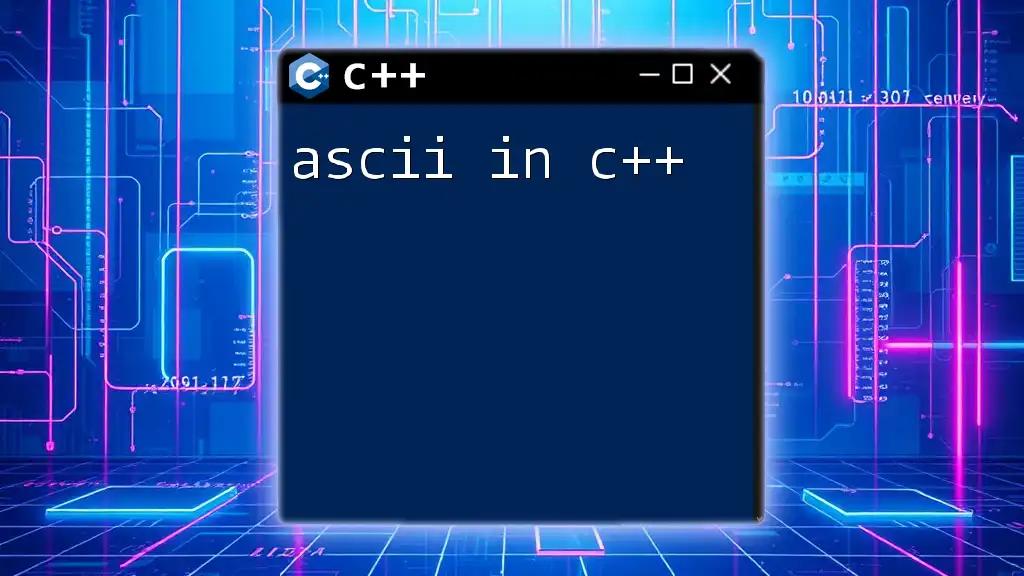
Additional Resources
For those looking to dive deeper into the world of C++, consider exploring official documentation and online coding platforms. Engaging with community forums can also provide practical insights and tips from experienced developers.