The `atoi` function in C++ converts a string to an integer, ignoring any leading whitespace and stopping at the first non-numeric character.
#include <iostream>
#include <cstdlib> // Include the header for atoi
int main() {
const char* str = "12345";
int num = atoi(str);
std::cout << "The integer value is: " << num << std::endl;
return 0;
}
Understanding the Purpose of `atoi`
`atoi` stands for "ASCII to integer." This function serves the primary purpose of converting a string representation of a number into its integer equivalent. It’s a straightforward mechanism widely used in C and C++ programming due to its simplicity.
When working with user inputs, data from files, or any textual representation of numeric values, you may find yourself needing to convert these strings into integers. That's where `atoi` becomes handy.

Syntax of `atoi`
The function is part of the C standard library, and its prototype is declared in the `<cstdlib>` header. The basic syntax is:
int atoi(const char *str);
- Parameters:
- `str`: This is the C-style string (character array) that is to be converted to an integer.
- Returns:
- An integer value converted from the string. If the string cannot be converted, it returns `0`.
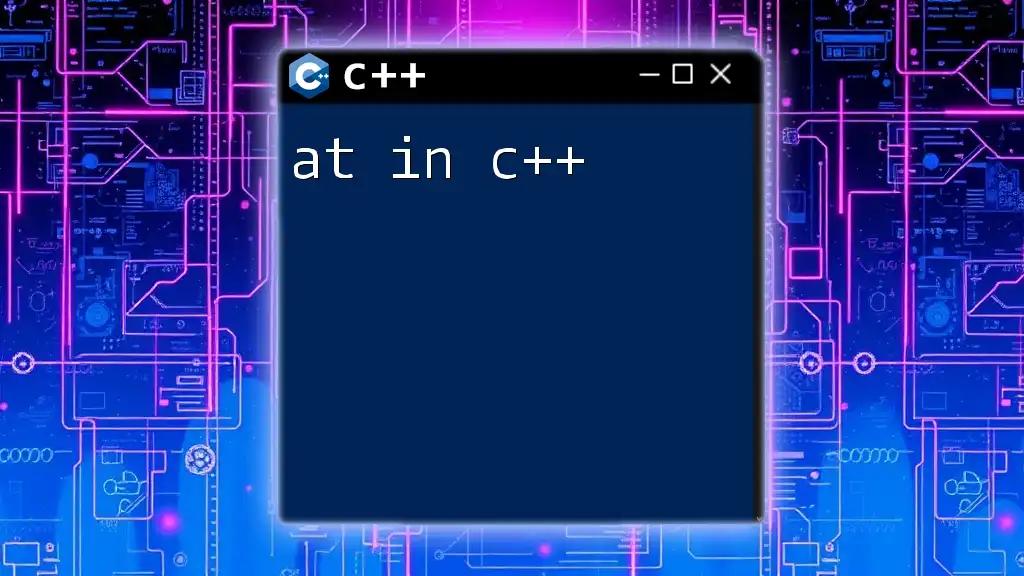
How to Use `atoi` in C++ Programs
Using `atoi` is quite straightforward. Below is a simple example demonstrating its application:
#include <iostream>
#include <cstdlib> // Required header for atoi
int main() {
const char* numberString = "1234";
int number = atoi(numberString);
std::cout << "Converted number: " << number << std::endl;
return 0;
}
In this example, the string `"1234"` is passed to `atoi`, which converts it to the integer `1234`. The result is printed to the console. This demonstrates the ease of converting strings to integers using `atoi`.

Handling Edge Cases with `atoi`
Non-Numeric Characters
One crucial feature of `atoi` is its behavior when the input string contains non-numeric characters. Let's look at how `atoi` handles such cases:
const char* invalidString = "123abc";
int invalidNumber = atoi(invalidString);
std::cout << "Converted invalid number: " << invalidNumber << std::endl; // Output: 123
In the above snippet, even though the string contains characters that follow the number, `atoi` reads until it encounters the first non-numeric character and stops. Thus, it successfully converts the valid part (`"123"`) into the integer `123`. This characteristic makes `atoi` somewhat lenient but can also lead to unexpected results if not handled correctly.
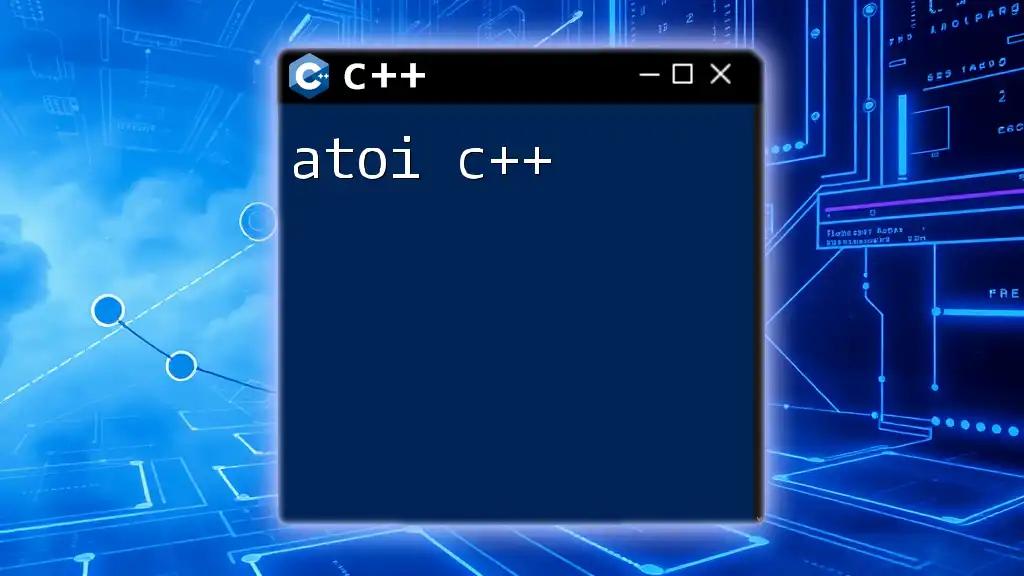
Common Pitfalls to Avoid
When using `atoi`, there are a few points developers should be cautious of:
-
Memory Management Issues: Since `atoi` takes a `const char*`, ensure that the string being passed is properly managed in memory and is null-terminated.
-
Undefined Behavior: The function does not handle cases of overflow or underflow. If the value represented by the string is outside the range of representable values for an integer, the behavior is undefined, which could cause unexpected runtime errors.
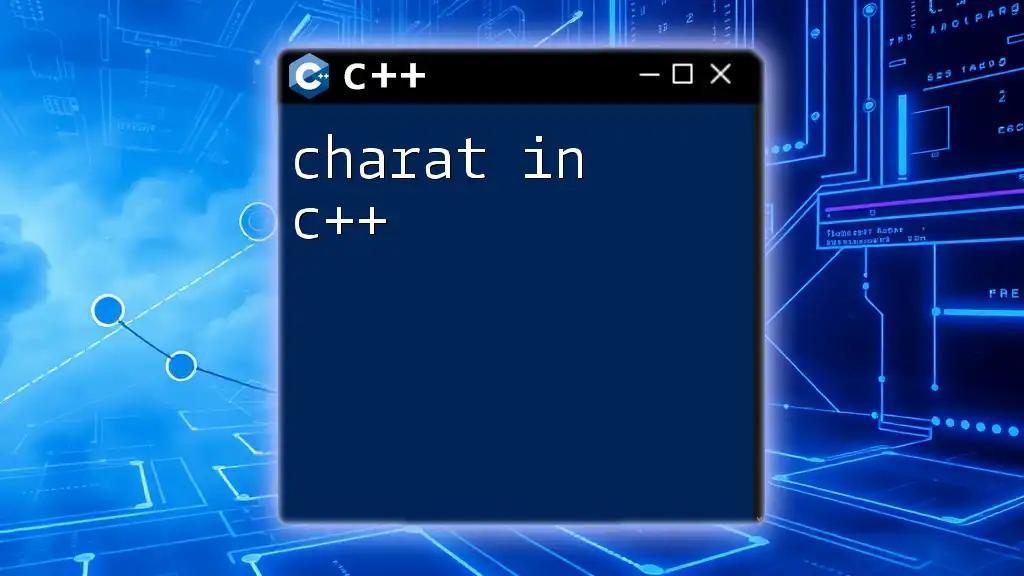
Alternatives to `atoi`
While `atoi` is convenient, it does have limitations. Here’s a comparison with `std::stoi`, which is part of the C++11 standard and uses a different approach for string conversion.
Using `std::stoi`
`std::stoi` offers several advantages over `atoi`:
-
Exception Handling: It throws exceptions when it encounters invalid input or when the number is out of range.
-
Versatility: It can handle various types of strings, such as `std::string`, which is native to C++.
Here's how to use `std::stoi` in C++:
#include <iostream>
#include <string>
int main() {
std::string numStr = "5678";
try {
int num = std::stoi(numStr);
std::cout << "Converted number using std::stoi: " << num << std::endl;
} catch (const std::invalid_argument& e) {
std::cerr << "Invalid input: " << e.what() << std::endl;
} catch (const std::out_of_range& e) {
std::cerr << "Number out of range: " << e.what() << std::endl;
}
return 0;
}
In this example, the conversion is wrapped in a `try-catch` block to handle potential exceptions, making your code more robust compared to using `atoi`.
When to Choose Which Function?
The choice between `atoi` and `std::stoi` depends on your specific use case. If you are dealing with simple, performance-critical scenarios, `atoi` may suffice. However, for complex applications requiring better error handling, `std::stoi` is the recommended approach.
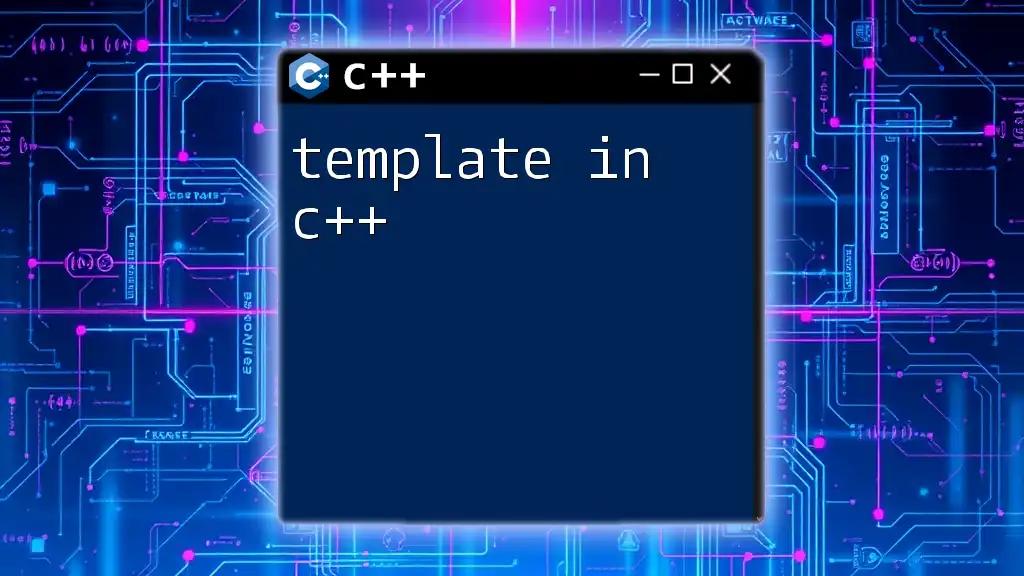
Conclusion
In summary, `atoi in C++` is a straightforward method for converting strings to integers. While it offers simplicity, it's essential to understand its limitations, especially regarding error handling and unexpected input. For better safety and versatility, consider exploring `std::stoi` as an alternative.
Eagerly experiment with both methods to see their impacts and choose the one that fits best with your coding needs.
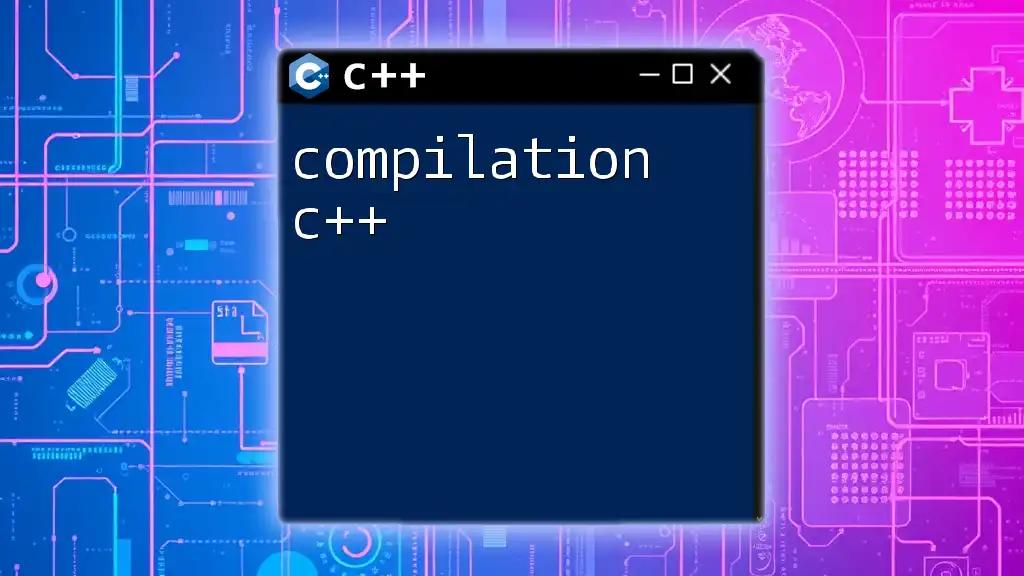
Additional Resources
For those looking to expand their understanding of string conversions in C++, various resources are available:
- C++ Standard Library documentation
- Books on C++ programming and data handling
- Online coding platforms offering courses on C++

FAQs about `atoi`
What happens if the input string is empty?
If an empty string is passed to `atoi`, the function returns `0` as there are no digits to convert.
Is `atoi` part of the C++ standard library?
Yes! `atoi` is part of the C standard library and is also available in C++ via the `<cstdlib>` header.
Can `atoi` handle negative numbers?
Yes, `atoi` can handle negative numbers correctly, provided the string starts with a minus sign. For example:
const char* negativeString = "-500";
int negativeNumber = atoi(negativeString);
std::cout << "Converted negative number: " << negativeNumber << std::endl; // Output: -500
Thus, understanding the nuances of `atoi` in C++ allows greater flexibility in handling string conversions. Embrace the intricacies, and happy coding!