In C++, the `charAt` equivalent can be achieved using the `operator[]` to access individual characters of a string, as shown in the following example:
#include <iostream>
#include <string>
int main() {
std::string text = "Hello";
char character = text[1]; // Accessing the second character
std::cout << character << std::endl; // Outputs: e
return 0;
}
What is `charAt`?
The term `charAt` typically refers to a function that retrieves a character from a given position in a string. While C++ does not have a built-in `charAt` method like languages such as Java or JavaScript, the concept can be easily implemented. Accessing individual characters in a string is essential for various tasks, from text manipulation to parsing and data analysis.
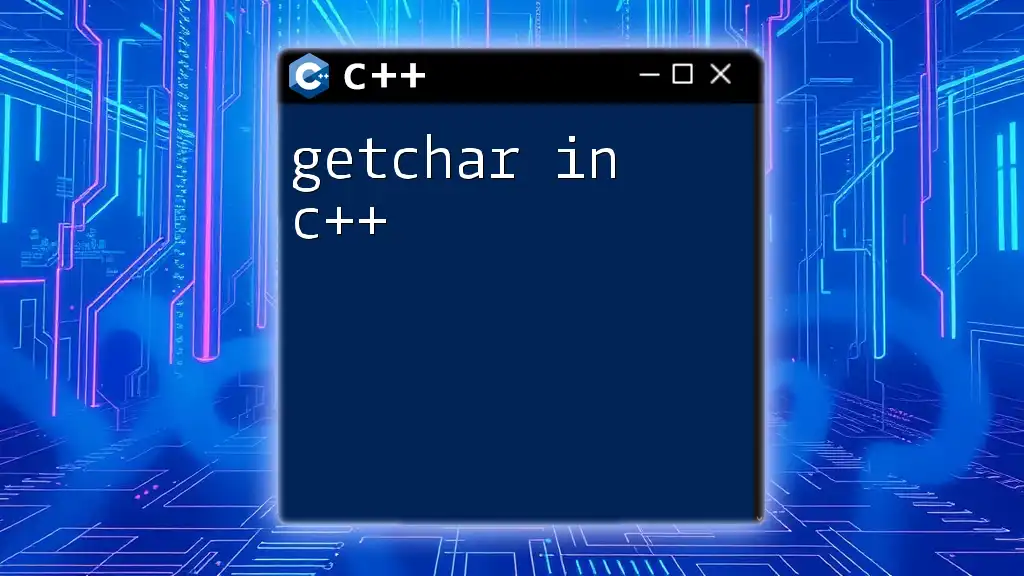
Understanding C++ Strings
Introduction to Strings in C++
In C++, strings can be represented using two primary data types: `std::string` and C-style strings (character arrays). The `std::string` class, part of the C++ Standard Library, provides many helpful functions and features, making it the preferred choice for handling textual data.
#include <string>
std::string greeting = "Hello, World!";
C-style strings are simpler but can lead to safety issues when manually managing memory. While using C-style strings is permissible, it's advisable to stick with `std::string` for modern C++ programming.
How Characters are Represented in C++
Characters in C++ are typically represented using the ASCII or Unicode encoding systems. ASCII can represent 128 characters, while Unicode can handle a vast range of characters from multiple languages. Understanding character encoding ensures you're adequately manipulating text to avoid misrepresentations.
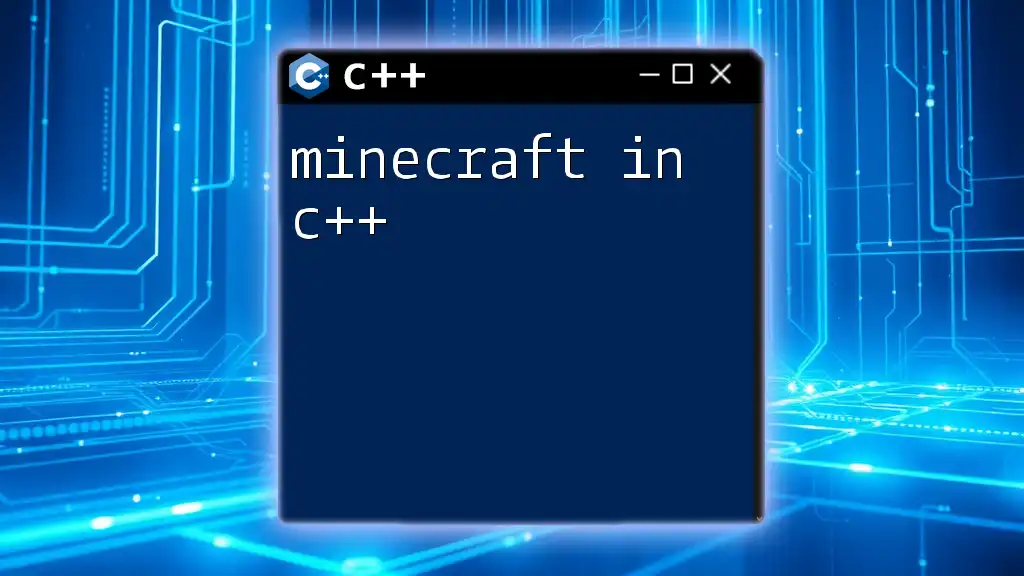
Accessing Characters in C++
Using the Index Operator with `std::string`
In C++, you can easily access individual characters of a string using the index operator (`[]`). This method is straightforward but can lead to undesirable behavior if you try to access an index outside the range of the string.
Example:
std::string text = "Hello";
char firstChar = text[0]; // 'H'
While this works most of the time, be aware that using an out-of-bounds index leads to undefined behavior.
Utilizing the `at()` Member Function
An alternative to using the index operator is the `at()` method, which provides a safer means of character access by performing bounds checking.
Example:
std::string text = "World";
char secondChar = text.at(1); // 'o'
If you attempt to access an index that is out of bounds using `at()`, it will throw an `std::out_of_range` exception, allowing you to handle errors gracefully.
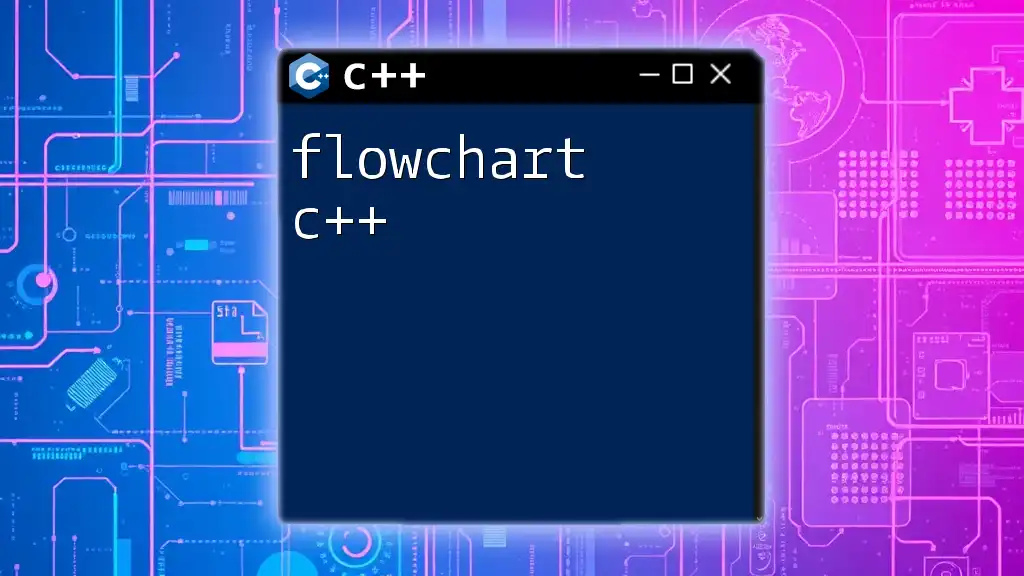
Using `charAt` Concept in C++
Creating a `charAt` Function in C++
Since C++ lacks a built-in `charAt` method, you can create one yourself. This can help reinforce understanding of string manipulations and improve code readability.
Example:
char charAt(const std::string& str, size_t index) {
if (index < str.size()) {
return str[index];
} else {
throw std::out_of_range("Index out of range");
}
}
This function checks whether the given index is valid and returns the respective character; otherwise, it raises an error, demonstrating good programming practice in handling exceptions.
Error Handling with `charAt`
Implementing error handling is crucial when creating your own `charAt` method. By using `try` and `catch`, you can manage exceptions effectively.
Example:
try {
char ch = charAt("Hello", 5); // This will throw an exception
} catch (const std::out_of_range& e) {
std::cerr << e.what() << std::endl; // Output: Index out of range
}
Using this method safeguards your code from potential runtime errors.
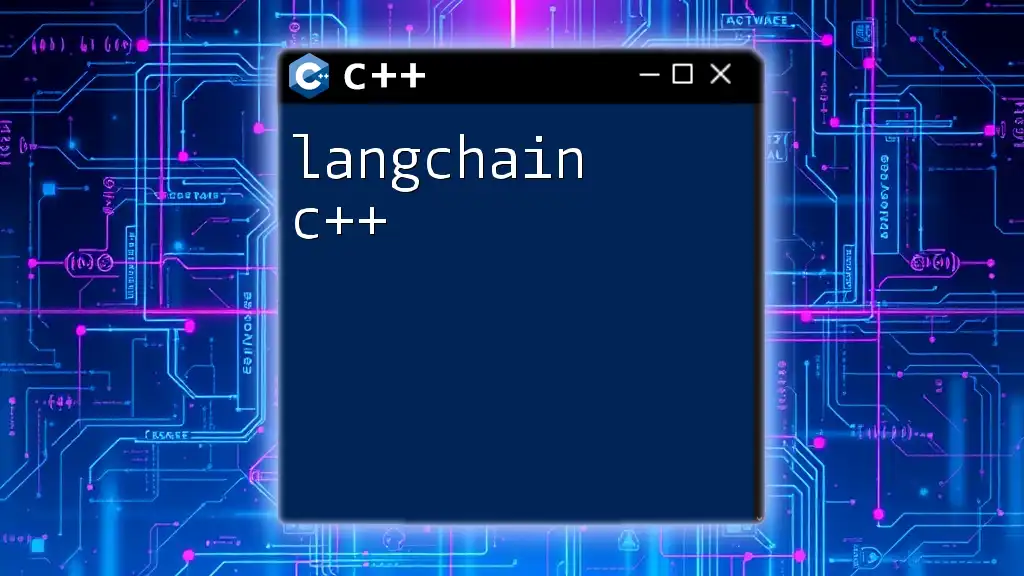
Practical Applications of `charAt` Concept
Iterating Over Strings
The custom `charAt` function can be particularly helpful when you need to iterate through each character of a string. This enables you to perform operations on each character seamlessly.
Example:
std::string name = "C++ Programming";
for (size_t i = 0; i < name.size(); i++) {
std::cout << charAt(name, i) << " ";
}
This code snippet displays each character in the string, demonstrating how easily you can access individual characters.
Modifying Characters in a String
Using the `charAt` concept, you can also create methods to modify characters at specific positions in a string. This enhances your ability to manipulate strings dynamically based on program needs.
Example:
void replaceChar(std::string& str, size_t index, char newChar) {
if (index < str.size()) {
str[index] = newChar; // Modifying the string
}
}
By replacing characters this way, you can create powerful text manipulation tools.
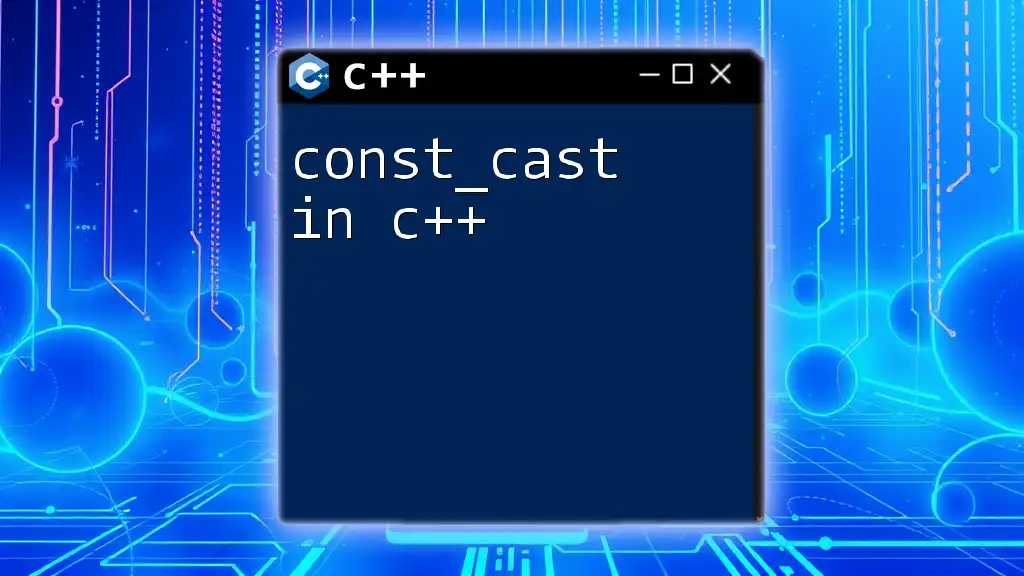
Best Practices
Choosing the Right Method for Character Access
When deciding how to access characters in a C++ string, consider the following:
- `[]` Operator: Faster and straightforward, but can lead to runtime issues if used improperly.
- `at()` Method: Safer alternative with built-in bounds checking, suited for error-prone situations.
Performance Considerations
Both methods perform in constant time (O(1)), but using `at()` may incur slight overhead due to bounds checking. Assess the context of your application to arrive at the best choice.
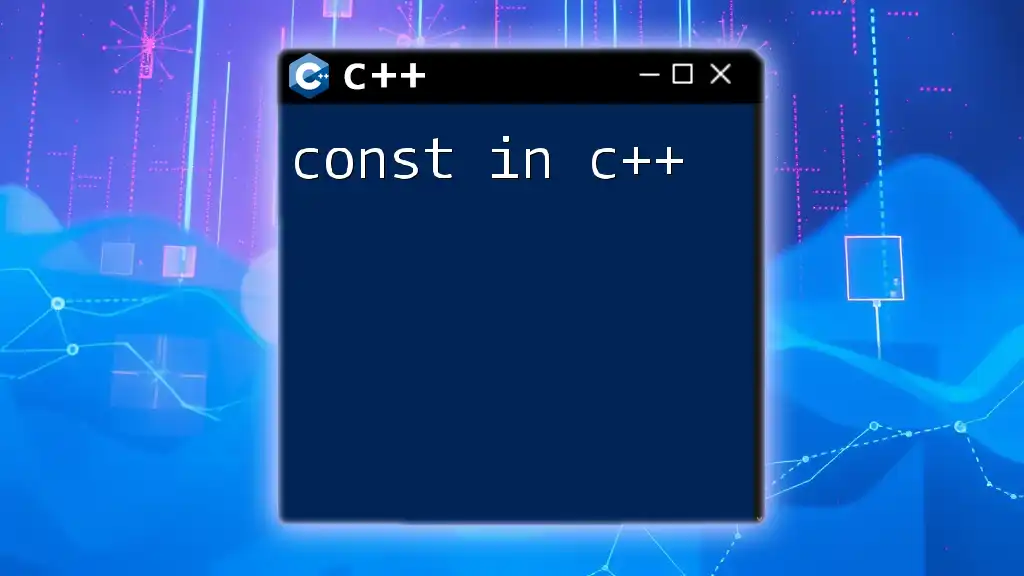
Conclusion
Understanding how to access and manipulate individual characters in a string is a fundamental skill in C++ programming. The concept of `charAt`, though not native to C++, can be efficiently implemented and applied for diverse use cases. By ensuring proper error handling and following best practices, you'll be well-equipped to handle string manipulation elegantly.
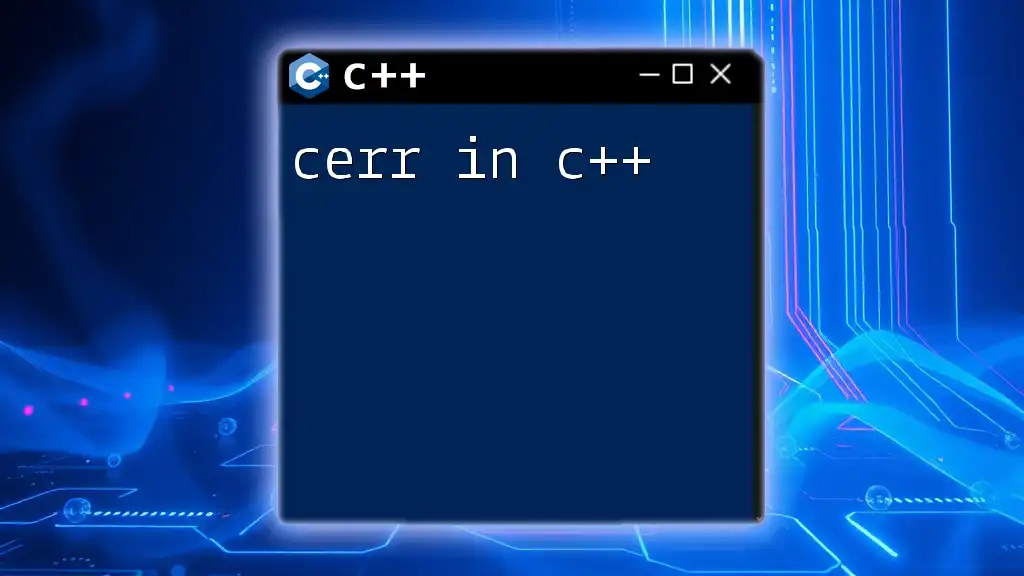
Further Resources
To expand your skills further, consider exploring additional readings on C++ strings, tutorials focused on exception handling, and community forums. These resources can provide deeper insights and collaborative support as you continue your journey in mastering C++.
Encourage experimentation with string manipulation, and you will discover the full power of text processing within the C++ programming language.