In this post, we explore how to create a simple Minecraft-like block placement system using C++ commands.
#include <iostream>
#include <vector>
class Block {
public:
std::string type;
Block(std::string t) : type(t) {}
};
class World {
private:
std::vector<Block> blocks;
public:
void placeBlock(const std::string& blockType) {
blocks.emplace_back(blockType);
std::cout << "Placed a " << blockType << " block." << std::endl;
}
};
int main() {
World myWorld;
myWorld.placeBlock("Dirt");
myWorld.placeBlock("Stone");
return 0;
}
Understanding the Basics of Minecraft
What is Minecraft?
Minecraft is a sandbox game developed by Mojang Studios that allows players to explore a blocky, procedurally generated 3D world, where they can mine resources, craft tools, and build structures. Central to its gameplay is the concept of blocks, each of which represents various materials from the environment. Players engage in activities such as mining, crafting, and exploring, making Minecraft a uniquely interactive experience.
Why Use C++ for Minecraft Modding and Development?
C++ is a powerful programming language that excels in performance and control over system resources. When it comes to gaming, particularly in resource-intensive environments like Minecraft, C++ is a preferred choice due to its capacity for high-speed execution and efficient memory management. C++ allows developers to create mods that can run smoothly, providing a richer experience without sacrificing performance.

Setting Up Your C++ Development Environment
Required Software and Tools
Before diving into coding, you need to establish a development environment. Popular Integrated Development Environments (IDEs) include Visual Studio and Code::Blocks. Both provide excellent support for C++ and come equipped with debugging and project management tools that streamline your coding process.
Along with an IDE, consider incorporating game development libraries such as SDL (Simple DirectMedia Layer) or SFML (Simple and Fast Multimedia Library). These libraries enable you to manage graphics, sounds, and input, essential components for game development.
Installing Minecraft C++ Libraries
To effectively develop mods for Minecraft, consider using libraries specific to Minecraft's architecture. For example, libminecraft is a library tailored for this purpose. Here's a basic example of initializing a project using this library:
#include <libminecraft.h>
int main() {
Minecraft::initialize();
// Additional Initialization Code
return 0;
}
This sets the stage for running Minecraft with C++ commands.
![Understanding Literals in C++ [A Quick Guide]](/_next/image?url=%2Fimages%2Fposts%2Fl%2Fliterals-in-cpp.webp&w=1080&q=75)
Exploring Minecraft’s Codebase
Overview of Minecraft's Source Code Structure
A solid understanding of Minecraft’s source code structure is vital for anyone wishing to modify or enhance the game. The codebase consists of numerous components, including rendering engines, input handlers, and game logic management. Knowing where to find features you're interested in modifying will save you time and effort during development.
Key Classes and Their Functions
Entity Class
The Entity class represents any object within the Minecraft world that can interact with the environment, including players and mobs. Creating an instance of this class is essential for developing new characters or objects. Here's a simplified version of how you might define an Entity class:
class Entity {
public:
int x, y, z; // Position coordinates
void move(int dx, int dy, int dz) {
x += dx;
y += dy;
z += dz;
}
};
In this code snippet, the `move()` function updates the entity’s position based on the provided delta values, allowing it to traverse the game world.
Block Class
The Block class serves as the foundation for all physical materials in Minecraft, such as dirt, stone, and wood. Understanding how to define new types of blocks can open up numerous possibilities. An example block declaration might look like this:
class Block {
public:
std::string type;
bool isSolid;
};
In this example, developers can specify block types and their properties (such as whether they are solid or not), which impacts player interaction.
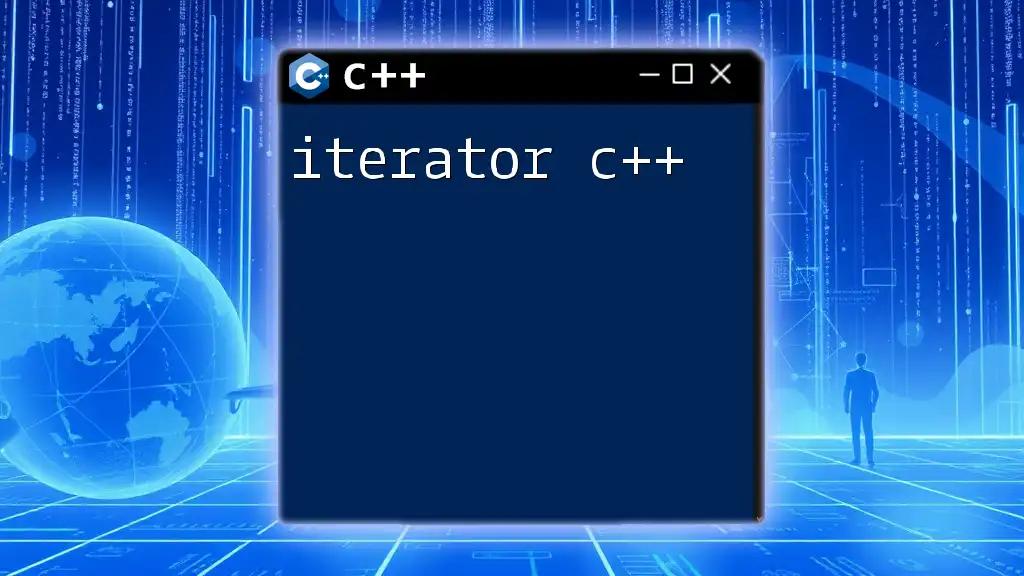
Creating Your First Minecraft Mod in C++
Setting Up Your Modding Environment
Creating mods for Minecraft requires specific tools tailored for development. Assess your project needs – such as the type of modifications you wish to implement – and ensure you have the necessary libraries and frameworks in place. There are several modding platforms available, each catering to different modding capabilities.
Code Snippet: Simple Block Addition
Once your development environment is prepared, adding your first block can be straightforward. Below is an illustrative code snippet for defining and registering a new block within your mod:
Block newBlock;
newBlock.type = "Mystic Stone"; // Custom block name
newBlock.isSolid = true; // Block solidity
This example demonstrates how to create a new block type called "Mystic Stone." The `isSolid` property indicates that players cannot walk through it, mimicking behaviors seen in existing Minecraft blocks.
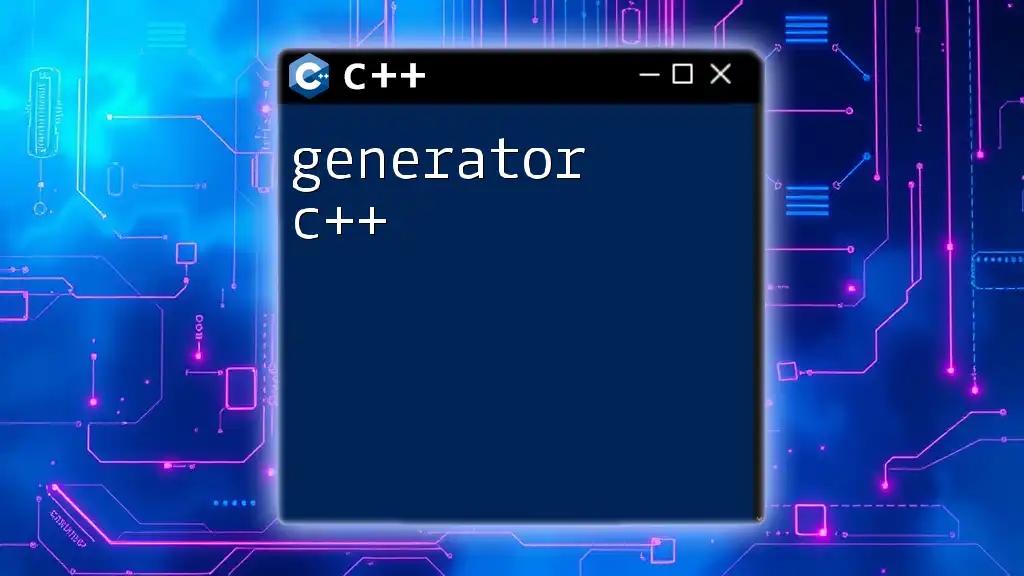
Enhancing Gameplay Elements
Adding New Game Mechanics
In addition to creating new blocks, developers can introduce new game mechanics that enhance gameplay. One popular modification is crafting new items. Here’s how to create a custom crafting recipe programmatically:
CraftingRecipe myRecipe;
myRecipe.addIngredient("Wood", 4);
myRecipe.addIngredient("Stone", 2);
This snippet showcases how to define a crafting recipe that requires four units of wood and two units of stone to create a new item. Understanding the crafting mechanics enhances the player's experience, allowing for deeper exploration and creativity.
Creating Unique Entities
To further personalize and enrich player interaction, you can create unique entities, such as mobs or characters with special abilities. Here’s a sample definition of a custom mob:
class CustomMob : public Entity {
public:
void attack() {
// Implement attack logic here
}
};
In this example, `CustomMob` inherits from `Entity`, adding specialized behavior (like an attack function) that signifies its distinct characteristics. This gives players a fresh experience with gameplay mechanics.
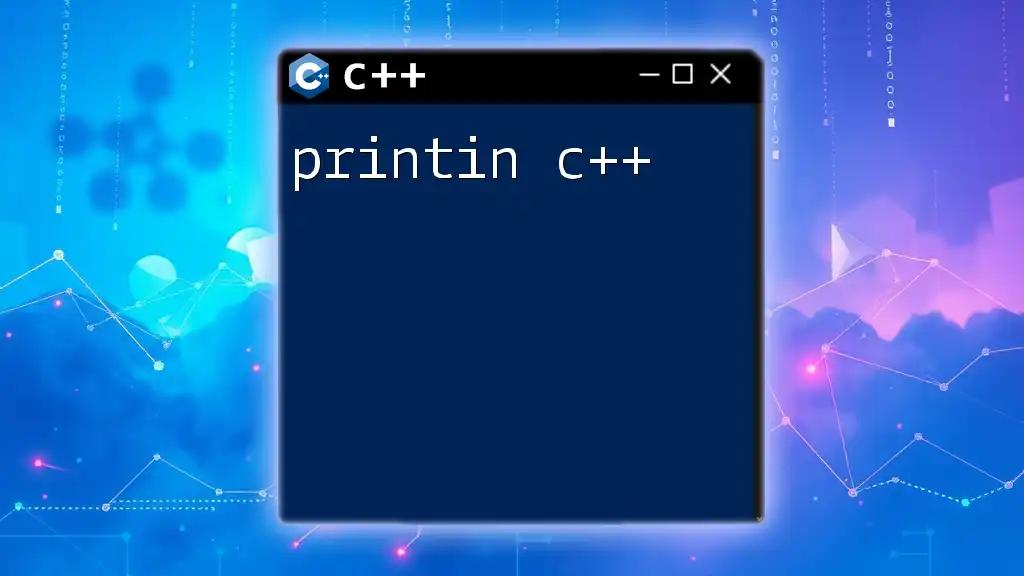
Testing and Debugging Your Minecraft Mods
Importance of Testing in Game Development
Testing your mods during development is critical; it ensures that the new features integrate seamlessly with existing game mechanics. Effective testing practices, such as unit testing and integration testing, help to identify and resolve issues early in the process.
Debugging Tips and Tools
Utilizing debugging tools specific to your IDE can significantly streamline troubleshooting. Pay attention to common bugs, such as logical errors or memory leaks. Keeping the code modular also aids in isolating issues, which makes the debugging process more manageable.
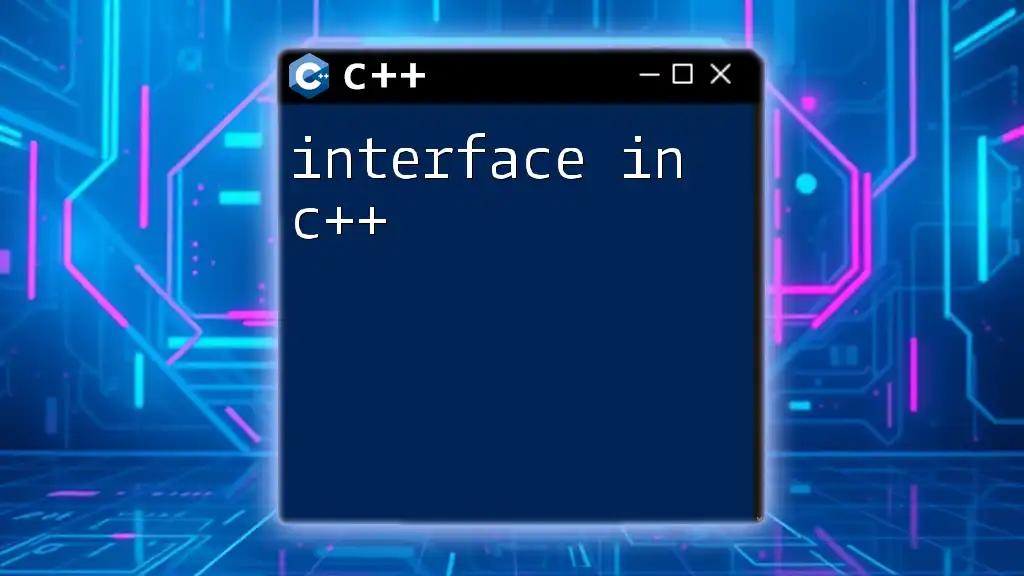
Community Resources and Further Learning
Online Forums and Communities
Engaging with the community is invaluable for any developer. Online forums, such as the Minecraft Forge Forum and GitHub repositories, provide a wealth of resources and support. Other developers can offer insights and solutions to common challenges.
Recommended Books and Tutorials
For those seeking a more structured learning approach, numerous books and online courses focus on C++ in game development. These resources can build foundational knowledge and introduce advanced concepts related to modding and game mechanics.
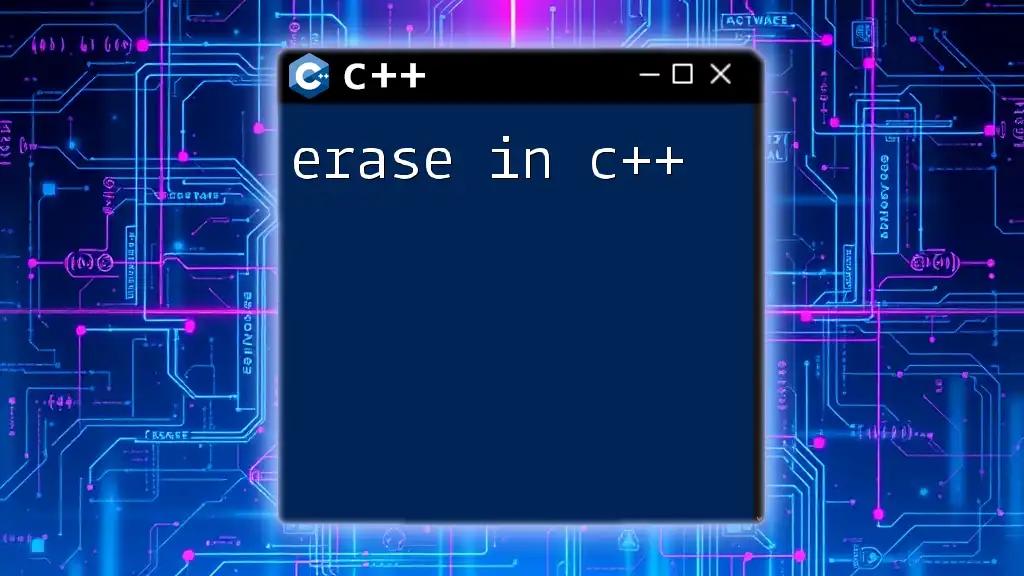
Conclusion
Through this guide, you have gained essential insights and skills in developing Minecraft mods using C++. You learned to navigate its source code, create unique gameplay elements, and set up a robust development environment. As you continue exploring the boundless possibilities that Minecraft in C++ offers, remember to connect with the community and share your creations. Happy modding!
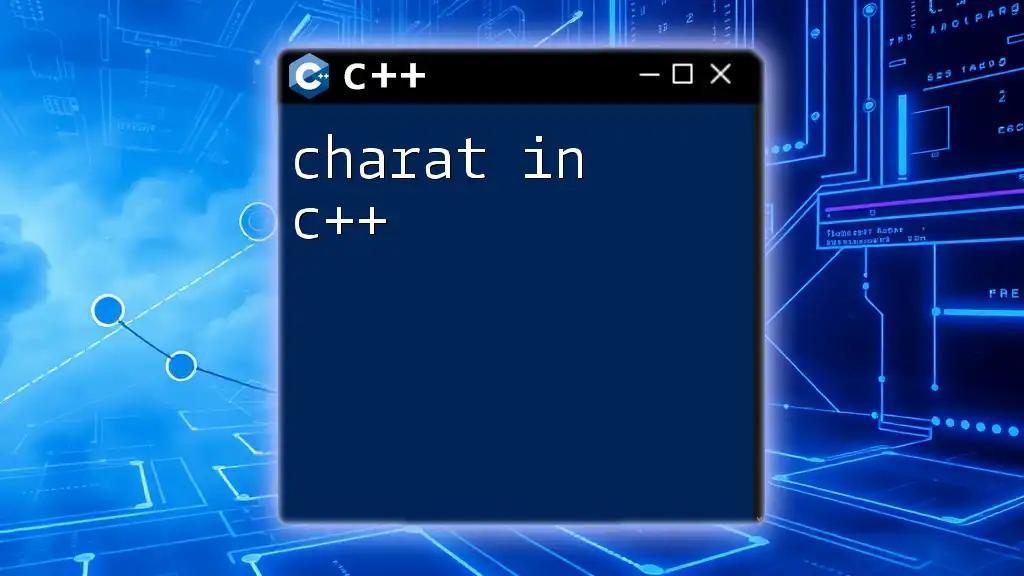
Additional Resources
Sample Code Repository
Explore the linked repositories for sample codes and project files to enhance your learning and experimentation.
FAQs About Minecraft in C++
Commonly asked questions will help clarify any uncertainties and encourage you to delve deeper into the world of Minecraft modding using C++.