In C++, `uint` is often used informally to refer to an unsigned integer type, which is represented as `unsigned int` and stores non-negative integer values.
unsigned int count = 10; // Initializes an unsigned integer with a value of 10
What is `uint`?
Definition of `uint`
In C++, `uint` is commonly used as an alias for `unsigned int`. It represents an integer data type that can hold only non-negative values. The absence of negative numbers allows for a broader range of positive integers compared to its signed counterpart.
Characteristics of `uint`
A `uint` typically has the same storage size as `int`, which is platform-dependent. On most platforms, `uint` occupies 4 bytes (32 bits). This translates to a range of values from 0 to 4,294,967,295. When compared to signed integers (`int`), which can represent values from -2,147,483,648 to 2,147,483,647, the unsigned variant effectively doubles the upper limit of positive values.
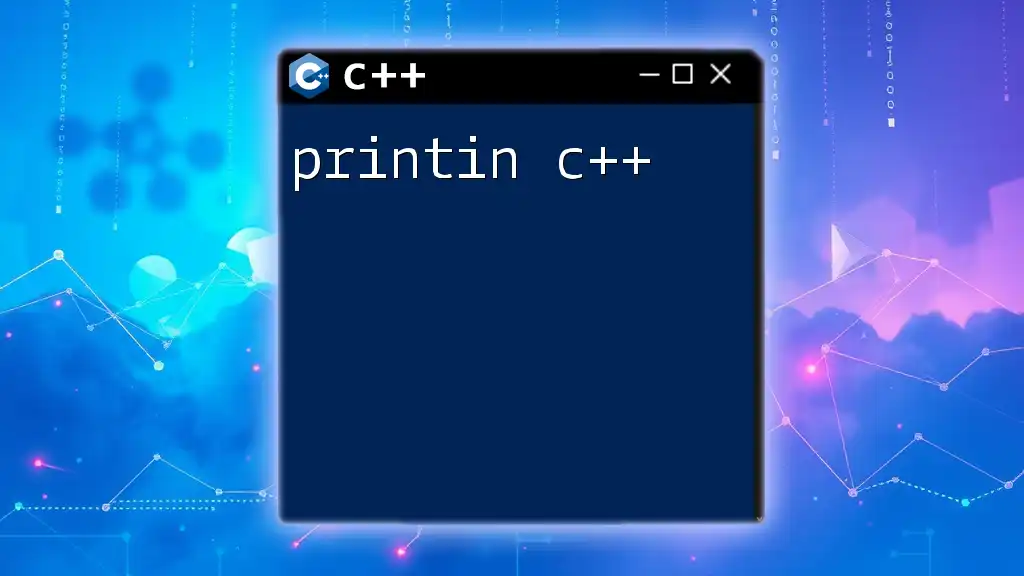
C++ Data Types
Basic Data Types
C++ offers a variety of built-in data types, including integers, floating-point numbers, characters, and more. Integer types are crucial for whole number representation, and the `uint` type falls under this category.
Unsigned Integers
Unsigned integers differ fundamentally from signed integers. While signed integers can hold both negative and positive values, unsigned integers like `uint` can only represent zero or positive values. This trait provides specific advantages, especially in contexts where negative values are not applicable.
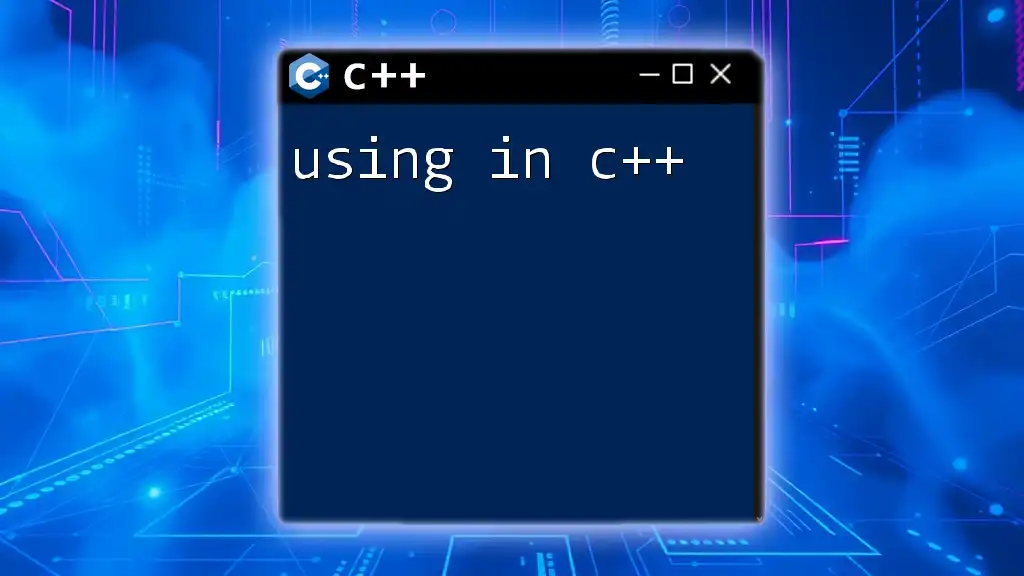
Declaring and Using `uint`
Syntax for Declaration
To declare a variable of type `uint`, the syntax is straightforward. This is particularly useful for coding clear, readable programs.
uint32_t myVariable; // Declare a 32-bit unsigned integer
Example Usage
Here’s a basic example of initializing and using a `uint` variable in a simple C++ program:
#include <iostream>
#include <cstdint>
int main() {
uint32_t myNumber = 10;
std::cout << "My unsigned number: " << myNumber << std::endl;
return 0;
}
In this snippet, we declare a `uint32_t` (a 32-bit unsigned integer), initialize it to 10, and print its value.
Operations on `uint`
You can perform various arithmetic operations on `uint`, just as you would with signed integers. Here’s an example showcasing addition, subtraction, multiplication, and division:
uint32_t a = 5;
uint32_t b = 10;
uint32_t sum = a + b; // sum = 15
This simple operation results in the sum of 5 and 10.
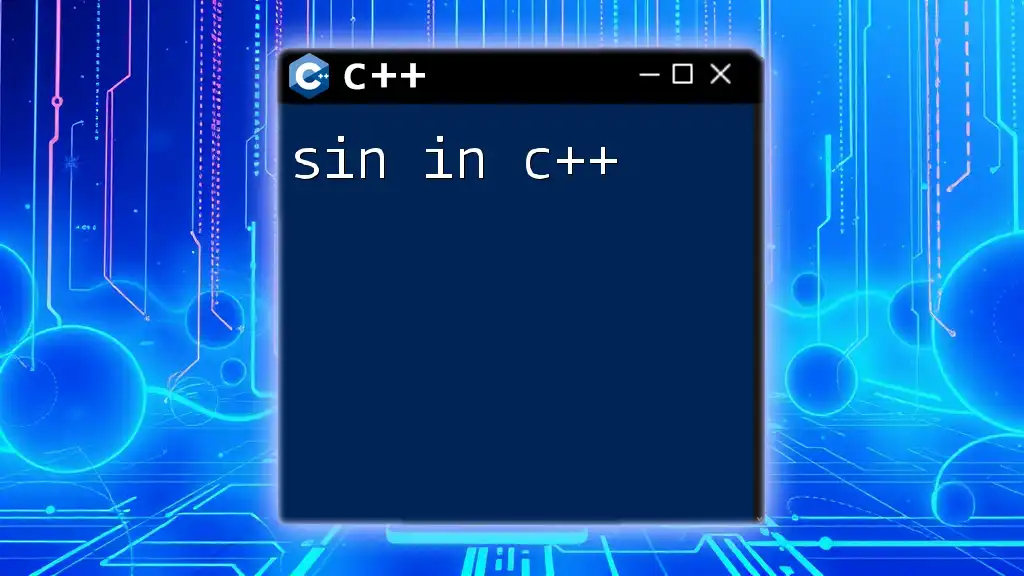
Best Practices for Using `uint`
When to Use `uint`
Using `uint` is particularly beneficial in scenarios that involve non-negative numbers. Consider using `uint` for:
- Counting items (e.g., the number of users).
- Addressing indices in data structures (e.g., arrays).
- Situations where negative values do not make sense, enhancing program clarity.
Common Pitfalls
While `uint` offers advantages, there are risks to watch out for. One common pitfall is related to overflow and underflow situations. Since `uint` cannot represent negative values, subtracting from zero leads to unintended behavior due to wrap-around:
uint32_t monsterHealth = 0;
monsterHealth -= 1; // Results in an unexpected value due to underflow
In this example, the operation yields a very large number (maximum value of `uint32_t`), which may lead to logical errors in your program.
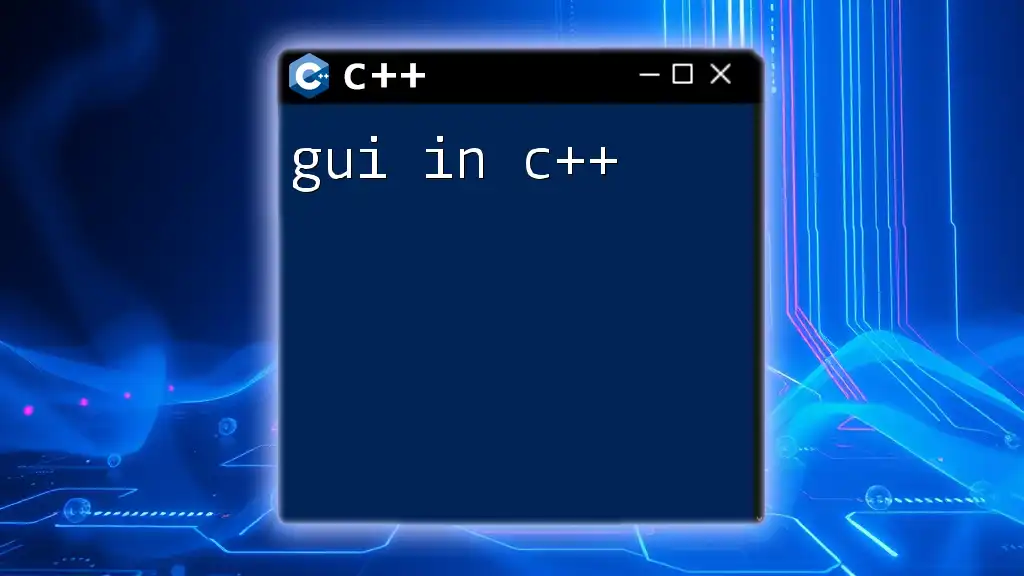
Comparison of `uint` with Other C++ Types
`uint` vs. `int`
When deciding between `uint` and `int`, consider the necessary range of values. If you need to represent negative values, opt for `int`. If your data is strictly non-negative and you wish to leverage the extended range, choose `uint`.
Other Unsigned Types
C++ offers various specific-width unsigned types, such as `uint8_t`, `uint16_t`, and `uint64_t`. Each of these types is engineered for particular use cases:
- `uint8_t`: Ideal for memory-constrained environments, as it uses only 1 byte.
- `uint16_t`: Often used for tasks requiring medium-range non-negative numbers.
- `uint64_t`: Suited for applications needing to store very large positive integers.
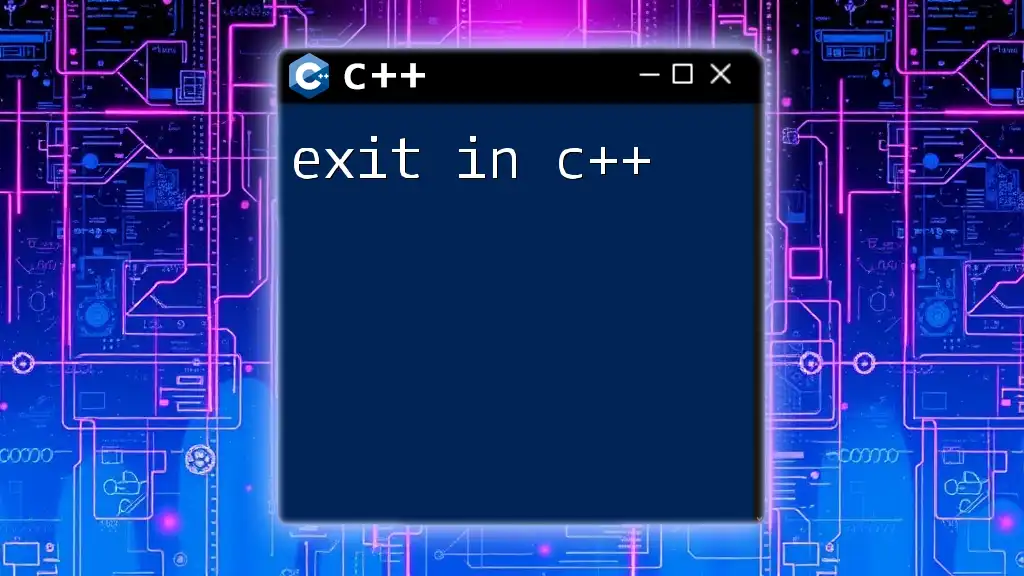
Working with `uint` in Functions
Passing `uint` as Function Arguments
Passing `uint` to functions is intuitive and aligns with standard practices in C++. Here’s an example:
void printUint(uint32_t number) {
std::cout << "The unsigned number is: " << number << std::endl;
}
This function receives a `uint` argument and prints its value.
Return Values of `uint`
Similarly, returning `uint` from functions follows the same principles. Here’s an example illustrating this:
uint32_t square(uint32_t x) {
return x * x;
}
This function takes a `uint` input and returns its square, ensuring that the result remains within the confines of the `uint` range.
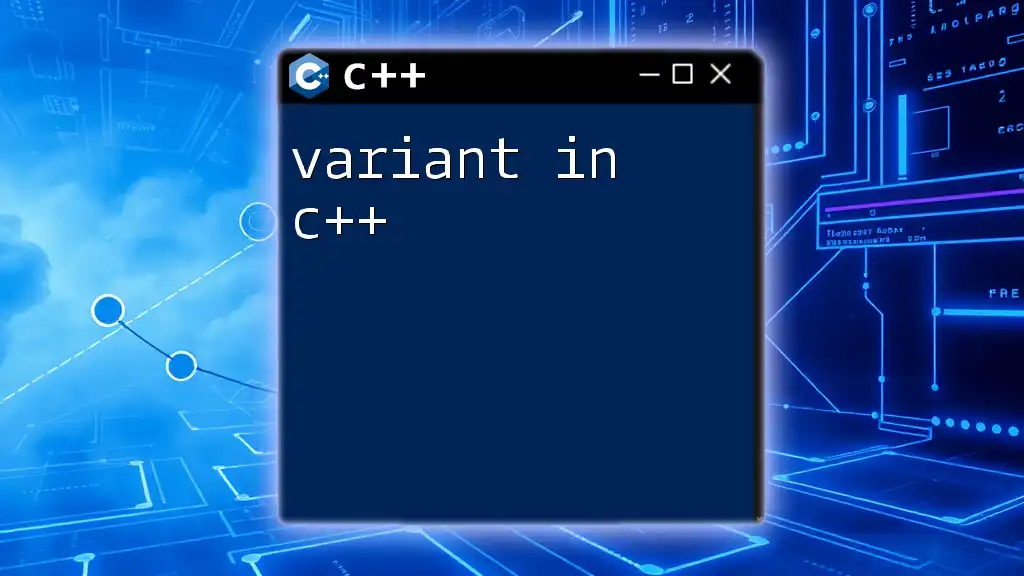
Conclusion
The use of `uint in C++` provides developers with a powerful tool to manage non-negative integers effectively. Understanding when and how to use `uint` can significantly enhance both the performance and clarity of your code. As you explore `uint`, consider its implications in your applications and embrace its capabilities!
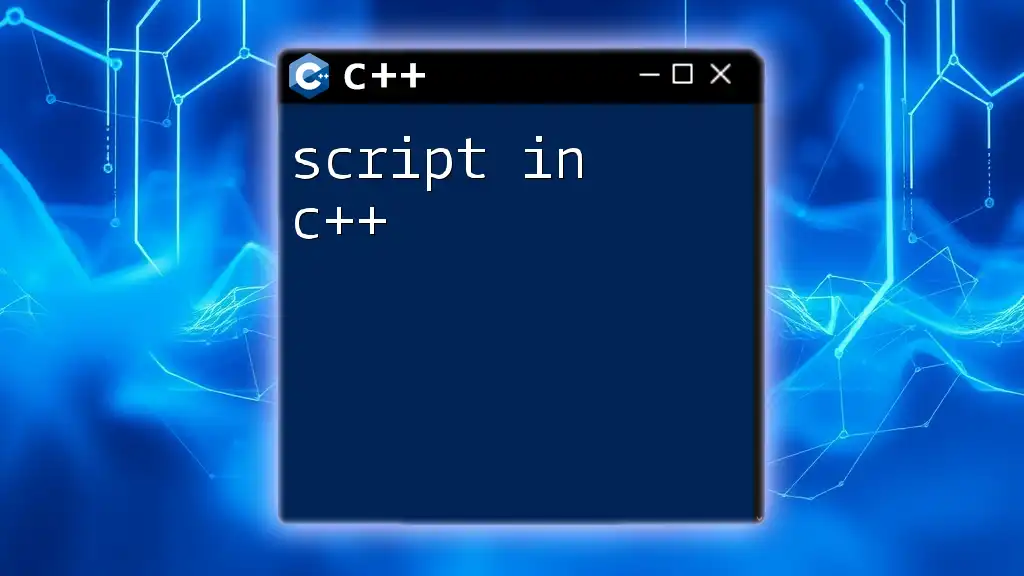
Additional Resources
For more in-depth exploration of C++ data types, consider consulting the official documentation, engaging with online forums, or diving into literature dedicated to C++ programming. These resources can bolster your understanding and facilitate an even smoother coding journey.