Swapping in C++ can be efficiently achieved using the `std::swap` function or by manually swapping two variables using a temporary variable.
Here's a code snippet illustrating both methods:
#include <iostream>
#include <utility> // for std::swap
int main() {
// Method 1: Using std::swap
int a = 5, b = 10;
std::swap(a, b);
std::cout << "After std::swap: a = " << a << ", b = " << b << std::endl;
// Method 2: Manual swapping
int x = 15, y = 20;
int temp = x;
x = y;
y = temp;
std::cout << "After manual swap: x = " << x << ", y = " << y << std::endl;
return 0;
}
What is Swapping?
Swapping is a fundamental concept in programming that involves exchanging the values of two variables. In the context of C++, swapping is especially crucial in various algorithms, including sorting techniques such as Bubble Sort and Selection Sort. Understanding how to swap values not only deepens your grasp of variable manipulation but also enhances your proficiency in creating efficient algorithms.
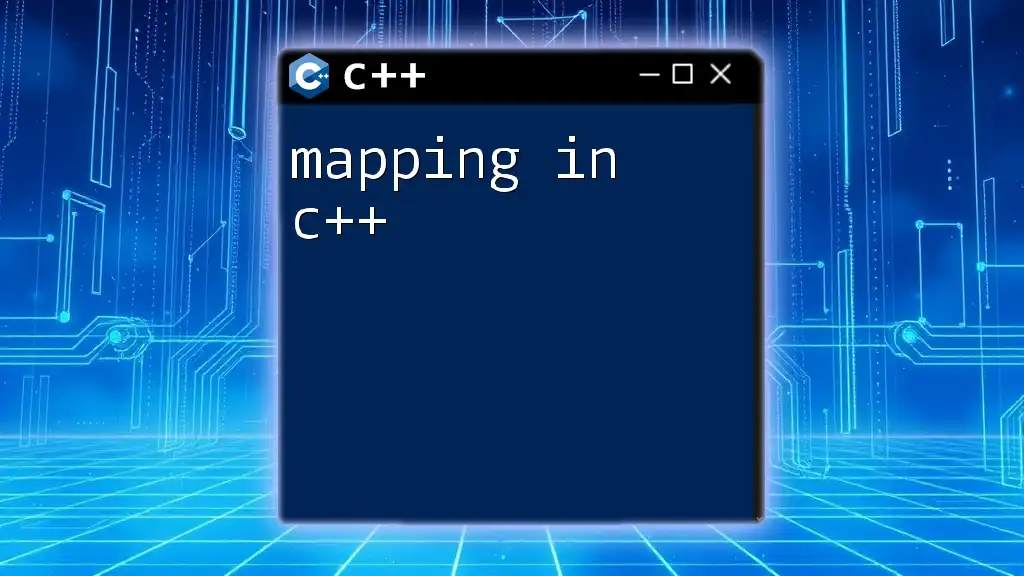
Basics of Swapping in C++
Fundamental Concepts
Before diving into swapping techniques, it's essential to understand two core concepts: variables and their values. A variable in C++ stores a value, which may be of types such as integers, floats, or user-defined classes. When you swap two variables, you temporarily need to hold one variable's value while assigning the other.
Additionally, in C++, there are two important ways to handle variables: references and pointers. A reference provides a way to directly access a variable without a copy, while a pointer holds the memory address of the variable. Swapping fundamentally relies on these concepts.
Simple Swapping with Temporary Variable
The most straightforward approach to swapping in C++ involves using a temporary variable. Here’s how it works:
#include <iostream>
void swap(int &a, int &b) {
int temp = a; // Hold the value of a
a = b; // Assign b to a
b = temp; // Assign the stored value to b
}
Explanation: In this method, we define a function `swap(int &a, int &b)` that accepts two reference parameters. The value of `a` is stored in a temporary variable `temp`. We then assign the value of `b` to `a`, followed by the value of `temp` to `b`. Using references ensures that the original variables are modified, and this technique is efficient for basic swaps.
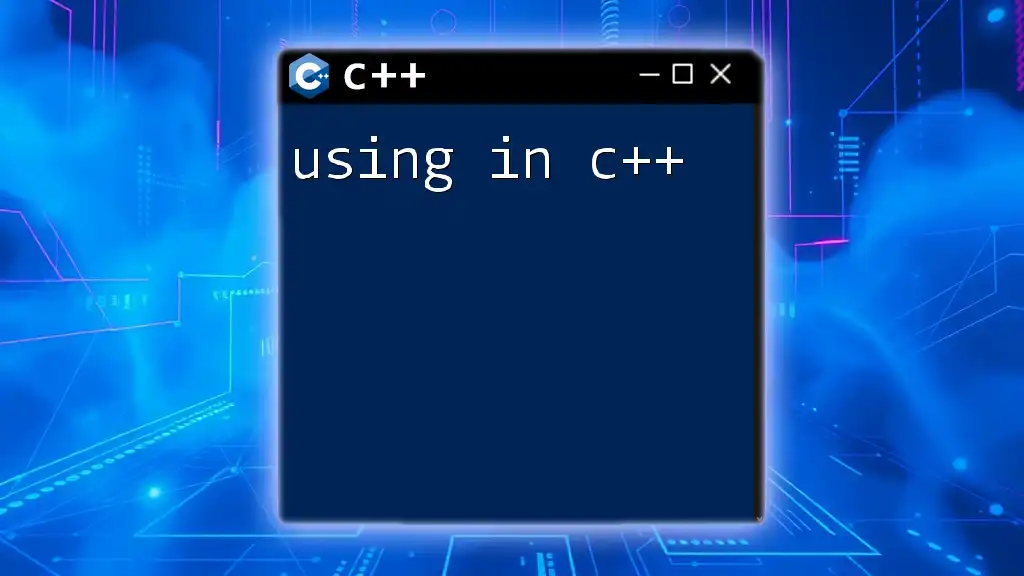
Swapping Using Built-in Functions
The `std::swap` Function
One of the most convenient ways to perform swapping in C++ is through the built-in `std::swap()` function. Available in the `<utility>` header, it streamlines the swap process and allows for improved readability and performance.
#include <iostream>
#include <utility> // Include for std::swap
int main() {
int x = 5, y = 10;
std::swap(x, y);
std::cout << "x: " << x << ", y: " << y << std::endl; // Output: x: 10, y: 5
}
Explanation: By including `<utility>`, you can invoke `std::swap(x, y)` directly. The beauty of this function lies in its ability to swap values efficiently while ensuring clarity in the code. It uses move semantics in modern C++ (C++11 and later versions) for optimal performance, especially with larger objects.
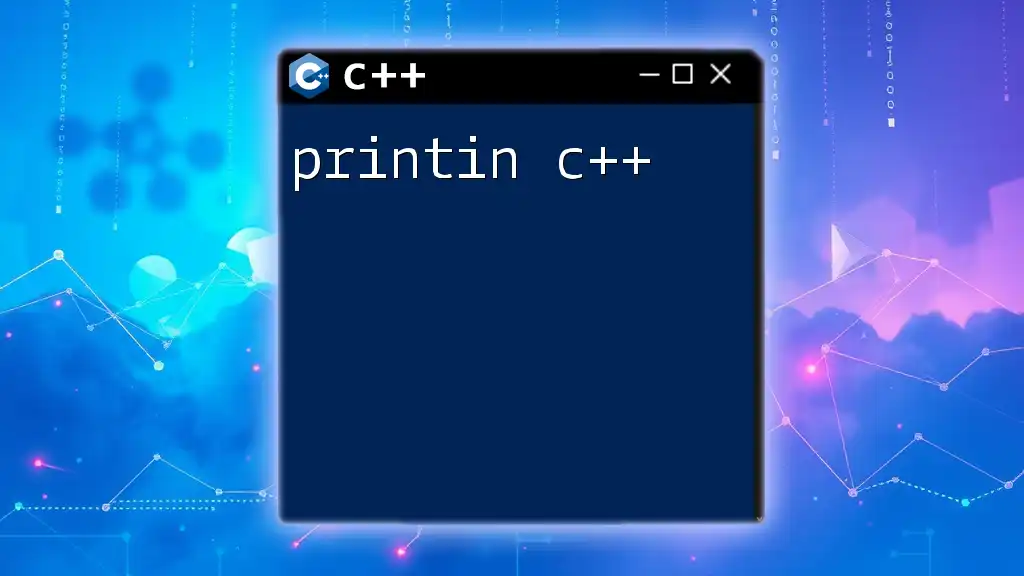
Advanced Swapping Techniques
Swapping Using XOR Operation
An intriguing method of swapping involves the XOR operation. This technique performs the swap without a temporary variable, which can be an educational exercise but is rarely used in practical scenarios due to readability concerns.
#include <iostream>
void swapXOR(int &a, int &b) {
if (a != b) { // Only swap if a and b are different
a = a ^ b; // Step 1
b = a ^ b; // Step 2
a = a ^ b; // Step 3
}
}
Explanation: Here, the XOR operator (`^`) is used to manipulate bits. The stepwise logic allows us to swap values without utilizing extra space. However, note that this method can lead to decreased code clarity and potential issues if the same variable is passed for both parameters.
Swapping in a Template Function
Templates in C++ allow for flexible functions that can work with any data type. Swapping using a template function makes the swapping mechanism adaptable to various data types.
#include <iostream>
template <typename T>
void swapGeneric(T &a, T &b) {
T temp = a;
a = b;
b = temp;
}
Explanation: The `swapGeneric()` function can accept any data type, making it versatile for use. Employing template functions is an effective way to write more generic and reusable code while ensuring type safety.
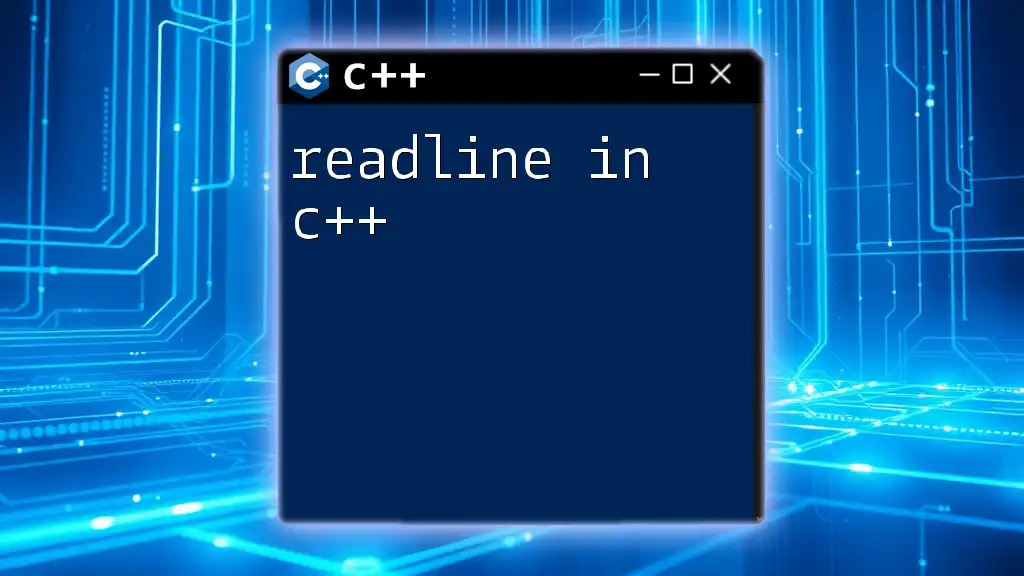
Common Mistakes When Swapping
When implementing swapping functions, there are common pitfalls to be aware of.
Issues with Passing by Value vs. Reference
Passing variables by value means that a copy is made, which is often not desirable in swapping situations. If you pass variables by value, the original variables remain unchanged after the swap operation. This confusion can lead to unexpected results, making it crucial to correctly use references.
Infinite Loops due to Incorrect Logic
Mistakes in the swapping logic may lead to infinite loops or corrupted data. Always ensure your swap function correctly handles scenarios where the same variable is passed for swapping.
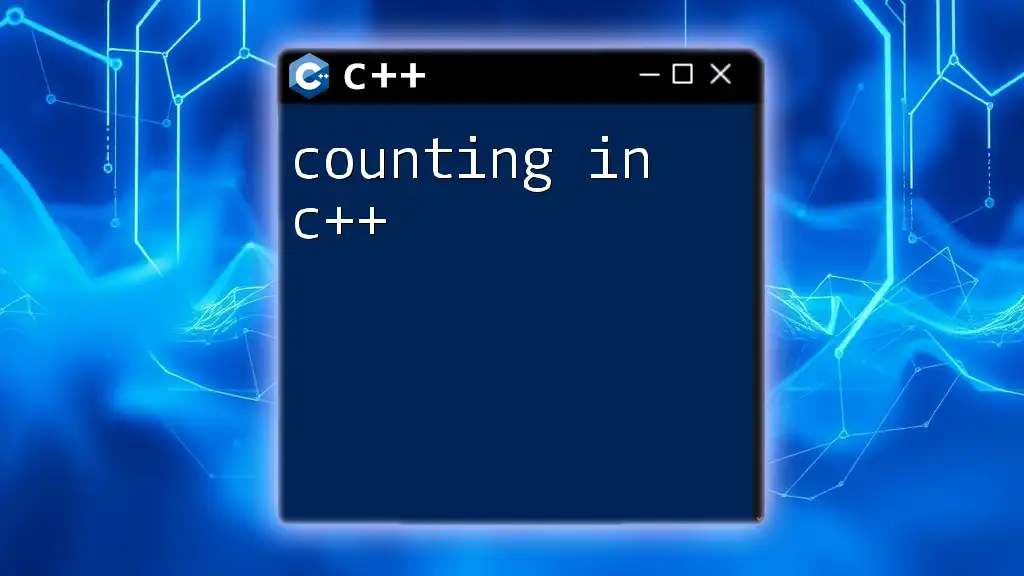
Performance Considerations
Time Complexity
Swapping operations typically have a time complexity of O(1), meaning it completes in constant time. However, the performance may vary when considering method implementations, especially with larger data structures.
Best Practices
Choosing the right method for swapping depends on the context. For basic cases, using a temporary variable or `std::swap()` works efficiently. For more advanced uses or template programming, swap functions can offer flexibility but may introduce complexity. Always consider readability and maintainability in your code.
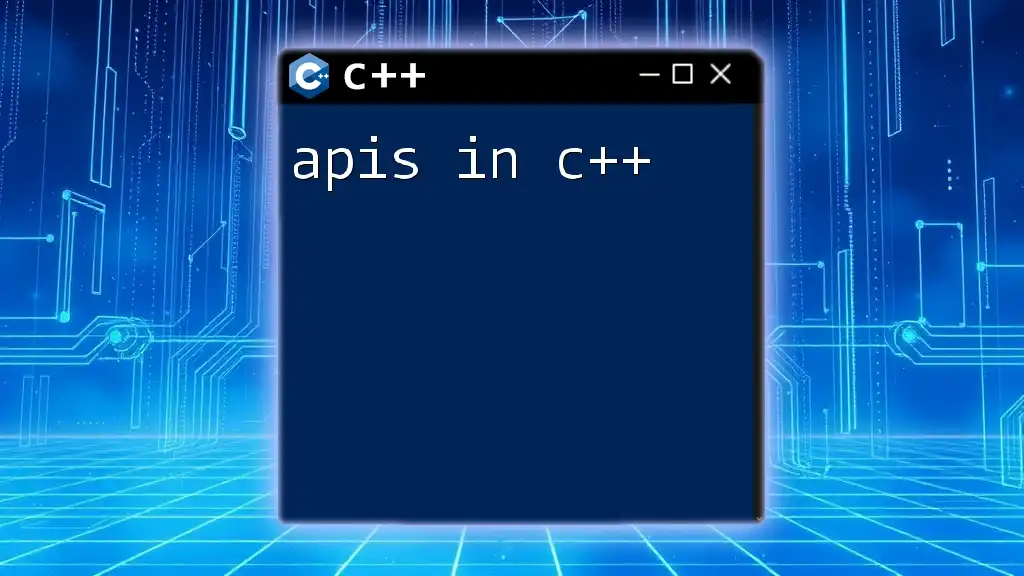
Conclusion
Mastering swapping in C++ is essential for effective programming. Whether employing simple methods or advanced techniques, understanding swapping empowers you to write more efficient algorithms and manage data operations skillfully. Practice implementing these methods in different scenarios to enhance your programming skills further.
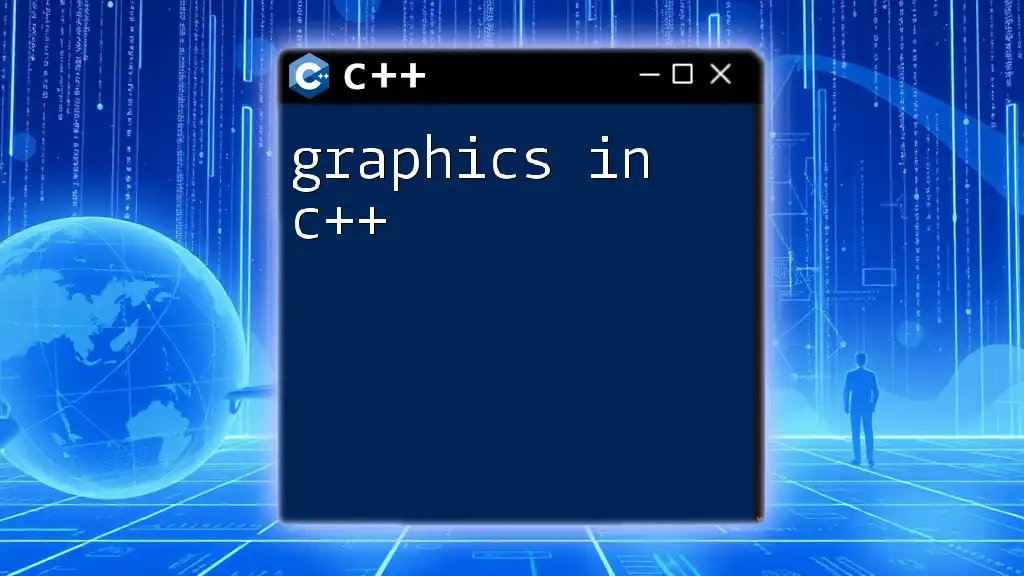
Additional Resources
Explore books, online courses, and community tutorials that delve into C++ and swapping techniques, offering opportunities for more in-depth learning and practical examples. Use platforms like GitHub for real-world code repositories that can further aid your understanding.