In C++, the "and" operator is a logical conjunction that returns true only if both operands are true, serving as an alternative to the traditional `&&` operator.
Here's an example of using the "and" operator in a simple conditional statement:
#include <iostream>
int main() {
bool a = true;
bool b = false;
if (a and b) {
std::cout << "Both are true" << std::endl;
} else {
std::cout << "At least one is false" << std::endl;
}
return 0;
}
The 'and' Operator
What is 'and' in C++?
The 'and' operator in C++ serves as an alternative representation of the logical AND operation. Logical operators are crucial for evaluating boolean expressions, which result in either `true` or `false`. In programming, these boolean evaluations play a vital role in decision-making processes, allowing developers to dictate the flow of execution based on specific conditions.
Syntax of 'and'
The syntax for using the 'and' operator is straightforward. It can be utilized in any expression where two or more conditions need to be evaluated together. Here's the basic structure:
bool result = condition1 and condition2;
In this line, `result` will be `true` only if both `condition1` and `condition2` are `true`. If either condition is `false`, then `result` becomes `false`.
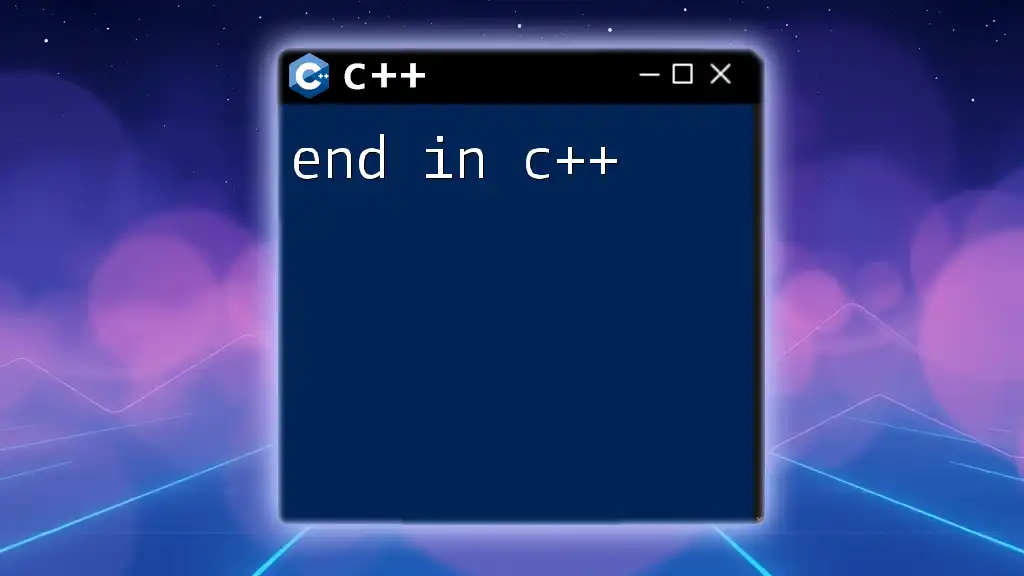
Logical Operations Explained
Importance of Logical Operators
Logical operators, including 'and', play a critical role in decision-making by combining conditions. In complex applications, where multiple conditions influence the control flow, the ability to express these conditions logically becomes essential. The 'and' operator allows developers to encapsulate two or more conditions seamlessly, improving readability while maintaining logic integrity.
Truth Tables for 'and'
To fully understand how the 'and' operator functions, it's helpful to consult a truth table. This table shows the results of the 'and' operation based on the truth values of its operands.
A | B | A and B |
---|---|---|
true | true | true |
true | false | false |
false | true | false |
false | false | false |
From this table, you can see that 'and' only returns true if both operands are true. This forms the basis of logical junctions in C++.
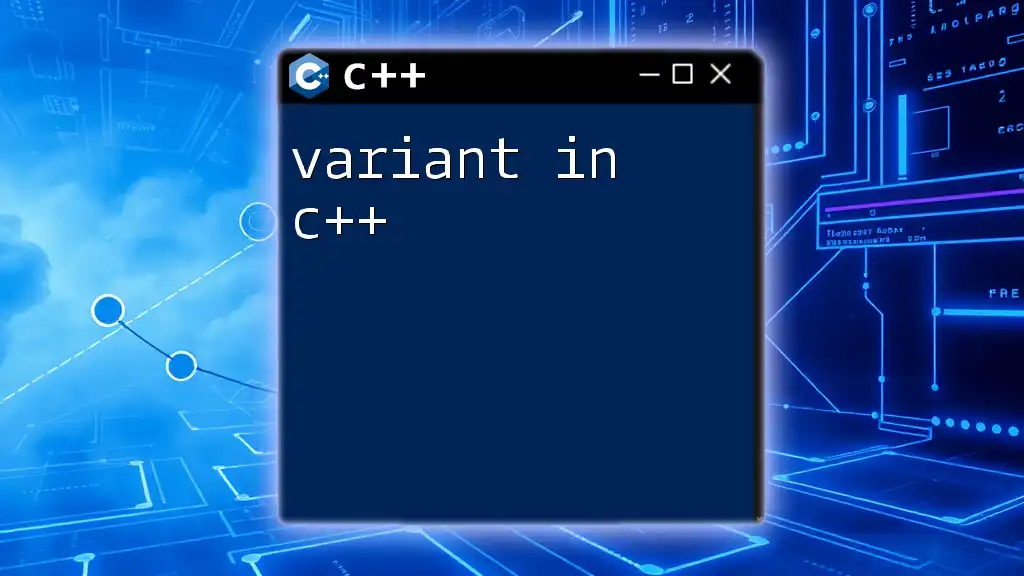
Comparing 'and' with Other Operators
'and' vs. '&&'
C++ also provides a symbolic representation of the logical AND operation using '&&'. While both 'and' and '&&' achieve the same result, they can differ in terms of readability depending on the preference of the programmer.
For instance, consider the following two conditional structures that are logically equivalent:
if (x > 0 and y > 0) {
// Do something
}
if (x > 0 && y > 0) {
// Do something
}
In this example, both pieces of code will execute the block if both `x` and `y` are greater than zero. Using 'and' can often enhance the readability for some developers, especially those who prefer more descriptive keywords over symbols.
When to Use 'and'
Choosing between the 'and' operator and the symbolic '&&' often boils down to coding style and the broader context of the code. For example, using 'and' can make the code appear more readable to someone unfamiliar with C++ symbols, making it a good choice in educational contexts or for beginners.
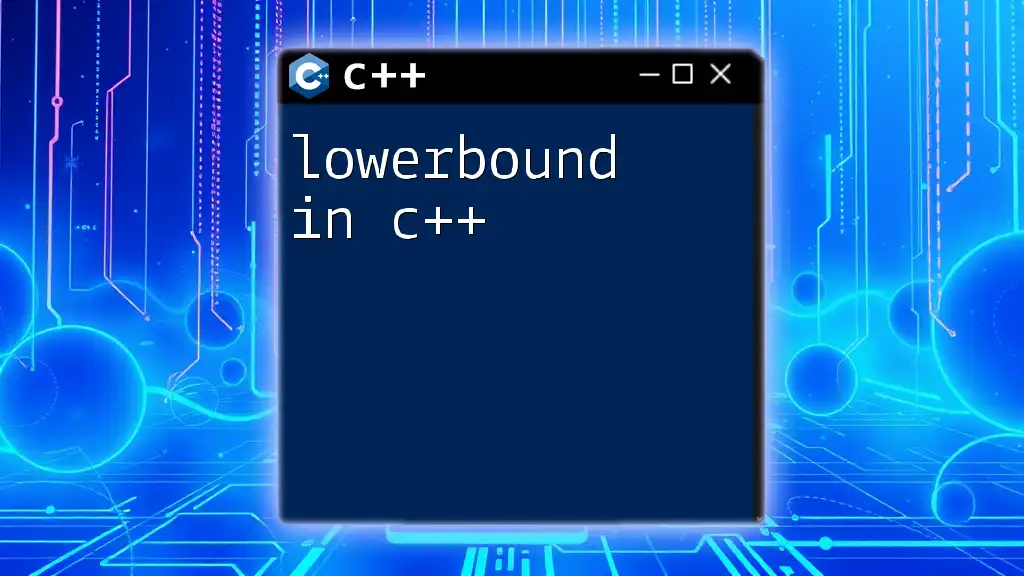
Practical Examples of 'and' in C++
Example 1: Basic Conditional Statement
Consider a simple example involving two boolean conditions:
#include <iostream>
using namespace std;
int main() {
bool condition1 = true;
bool condition2 = false;
if (condition1 and condition2) {
cout << "Both conditions are true." << endl;
} else {
cout << "One or both conditions are false." << endl;
}
return 0;
}
In this code, the message "One or both conditions are false." will be displayed since `condition2` is false. This example illustrates how the 'and' operator functions in a basic conditional evaluation.
Example 2: Loop Control
The 'and' operator can also be used effectively within loops. Here’s an example:
for (int i = 0; i < 10; i++) {
if (i > 2 and i < 8) {
cout << i << " is within range." << endl;
}
}
In this loop, only the values of `i` from 3 to 7 will trigger the message because they satisfy both conditions of being greater than 2 and less than 8.
Example 3: Complex Conditions
Using 'and' helps in defining complex conditions succinctly. For instance:
int age = 25;
bool hasLicense = true;
if (age >= 18 and hasLicense) {
cout << "You can drive!" << endl;
} else {
cout << "You cannot drive." << endl;
}
Here, the program checks if the person is at least 18 years old and possesses a driving license. If both conditions are satisfied, it allows driving; otherwise, it prevents it.
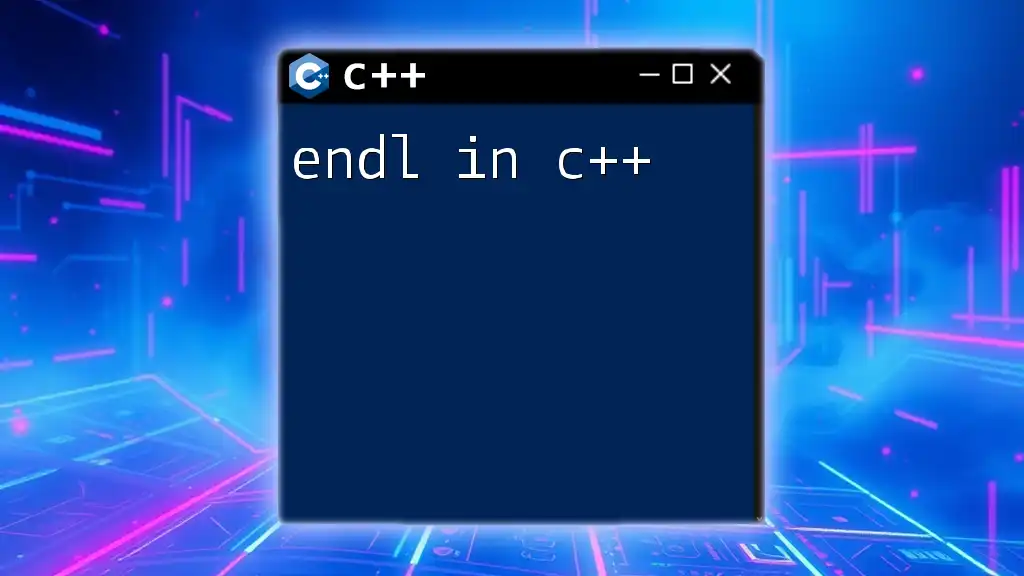
Best Practices with Logical Operators
Readable Code
When using the 'and' operator, focusing on readability is crucial, especially for complex expressions. Here are some tips for writing clear code:
- Use parentheses to clarify logical expressions. For instance, `(condition1 and condition2) or condition3` clearly outlines the intended order of evaluation.
- Write descriptive comments to explain the logic, especially if the conditions are complex, ensuring that someone reading the code can understand its purpose quickly.
Performance Considerations
It's important to note that logical operators in C++ exhibit short-circuiting behavior. For example, in the expression `condition1 and condition2`, if `condition1` is false, `condition2` is never evaluated because the entire expression will evaluate to false regardless. This can lead to performance optimizations, though additional care should be taken when side effects are associated with these conditions.
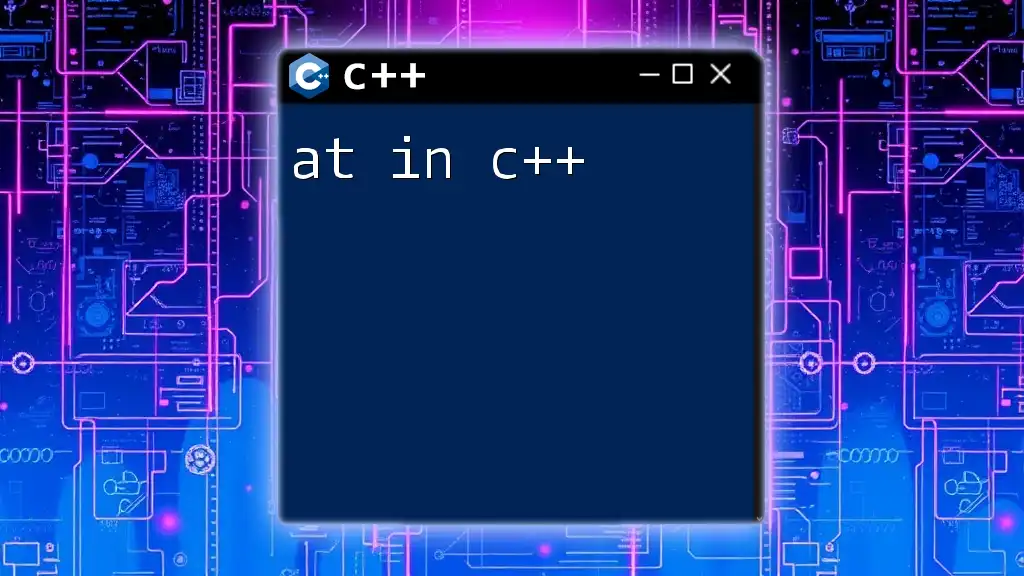
Conclusion
The 'and' operator in C++ is an indispensable tool in logical evaluations, allowing developers to assess multiple conditions efficiently. Understanding its application and how it compares to other operators enriches your programming toolkit. Practice using 'and' and explore its various contexts to enhance your C++ skills further!
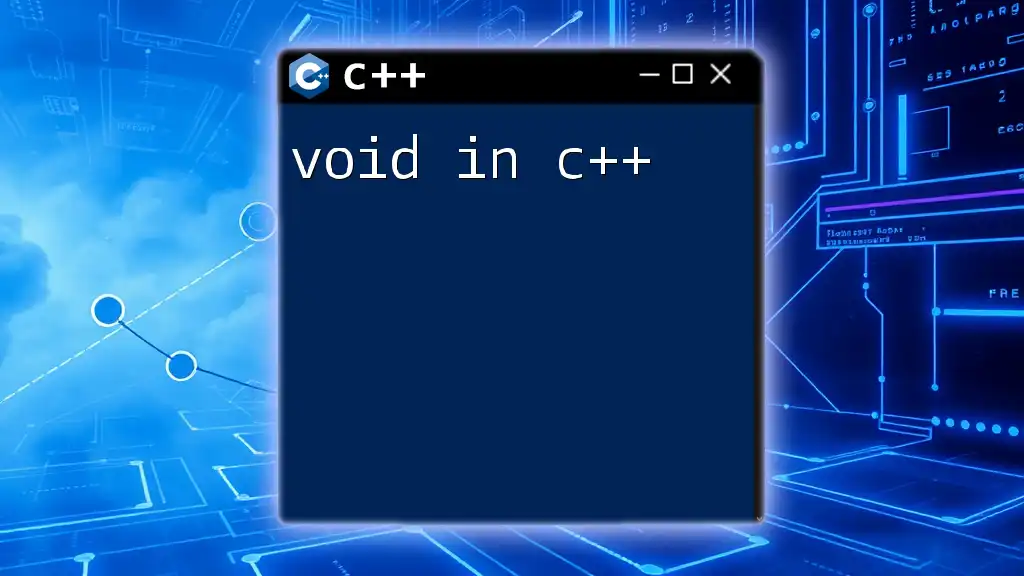
Additional Resources
For those looking to deepen their understanding of logical operators in C++, consider exploring comprehensive C++ resources, documentation, or other programming tutorials that focus on control flow and boolean logic.