The `erase` function in C++ is used to remove elements from a container, such as a vector or a list, by specifying the position or range of elements to be deleted.
Here's a code snippet illustrating how to use the `erase` function with a `std::vector`:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.erase(vec.begin() + 2); // Removes the element at index 2 (the value 3)
for (int num : vec) {
std::cout << num << " "; // Outputs: 1 2 4 5
}
return 0;
}
The Basics of the `erase` Function
What is `erase`?
The `erase` function is a powerful utility in C++ that allows developers to remove elements from containers. Its primary purpose is to provide an efficient means of manipulating data structure contents without the need for manual shifting or reallocation.
Where is `erase` Used?
The `erase` function can be found in various C++ Standard Library containers such as:
- std::vector
- std::list
- std::map
- std::set
- std::string
Understanding how to effectively use the `erase` function within these containers is essential for any C++ programmer.

Using `erase` in Different C++ Containers
Erasing Elements from Vectors
Syntax for Vector Erase
To erase an element from a vector, the syntax generally follows this pattern:
vector_name.erase(iterator);
Here, the `iterator` represents the position of the element you want to remove.
Code Example: Erasing an Element at a Specified Position
Consider the following example, where we remove the element at index 2 from a vector:
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec = {10, 20, 30, 40, 50};
vec.erase(vec.begin() + 2); // Erases the element 30
for (int val : vec) {
std::cout << val << " ";
}
return 0;
}
In this example, the output will be `10 20 40 50`, demonstrating how the element `30` was successfully removed.
Erasing a Range of Elements
To erase a range of elements from a vector, you can use:
vector_name.erase(start_iterator, end_iterator);
Here’s an example illustrating this:
vec.erase(vec.begin(), vec.begin() + 2); // Erases 10 and 20
After executing this code, the vector now contains `30 40 50`.
Erasing Elements from Strings
Syntax for String Erase
When working with strings, the syntax is slightly different:
string_name.erase(start_position, length);
This specifies the starting index and how many characters to erase.
Code Example: Erasing a Substring from a String
Here is how you can erase a substring:
#include <string>
#include <iostream>
int main() {
std::string str = "Hello, World!";
str.erase(7, 5); // Erases 'World'
std::cout << str; // Outputs: Hello, !
return 0;
}
In this example, the output will be `Hello, !`, showing the removal of the substring `World`.
Erasing Elements from Lists
Syntax for List Erase
In lists, you can also erase elements efficiently. The syntax mirrors that of vectors:
list_name.erase(iterator);
Code Example: Removing an Element by Value
For a practical example, consider the following:
#include <list>
#include <iostream>
int main() {
std::list<int> myList = {1, 2, 3, 2, 4};
myList.remove(2); // Removes all occurrences of 2
for (int val : myList) {
std::cout << val << " ";
}
return 0;
}
This results in the output `1 3 4`, effectively removing all instances of the value `2`.
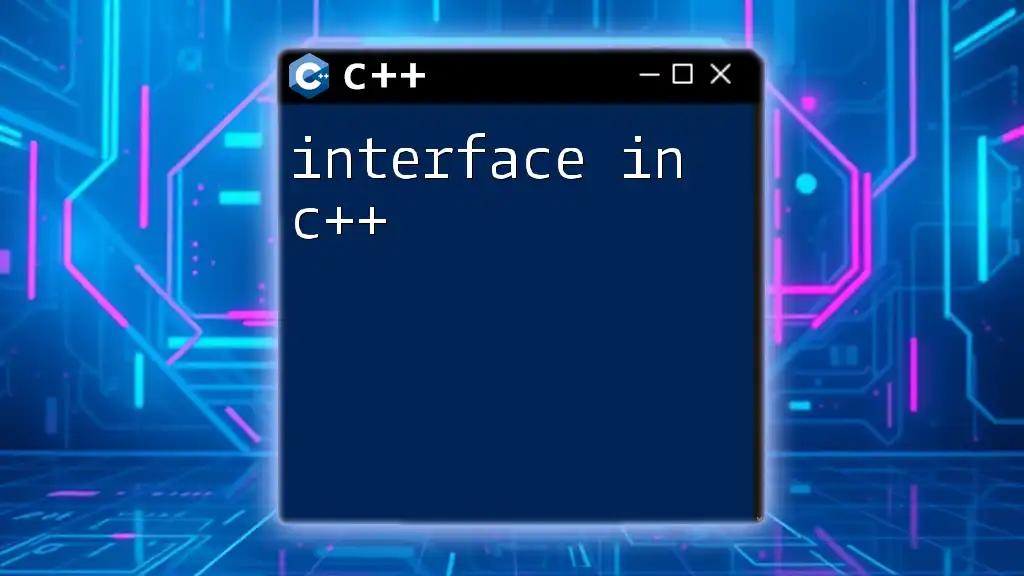
The `erase` Function with Maps and Sets
Erasing Elements from Maps
Syntax for Map Erase
Erasing elements from maps uses the key to remove a specific key-value pair:
map_name.erase(key);
Code Example: Erasing an Element by Key
Consider this example using a map:
#include <map>
#include <iostream>
int main() {
std::map<int, std::string> myMap = {{1, "one"}, {2, "two"}, {3, "three"}};
myMap.erase(2); // Erases the pair with key 2
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << "\n";
}
return 0;
}
The output will show `1: one` and `3: three`, confirming the removal of the pair with the key `2`.
Erasing Elements from Sets
Syntax for Set Erase
Sets also include a simple `erase` function:
set_name.erase(value);
Code Example: Removing an Element from a Set
Here’s an example demonstrating how to erase an element from a set:
#include <set>
#include <iostream>
int main() {
std::set<int> mySet = {1, 2, 3, 4};
mySet.erase(2); // Removes 2 from the set
for (int val : mySet) {
std::cout << val << " ";
}
return 0;
}
This will output `1 3 4`, successfully removing the number `2` from the set.
![Understanding Literals in C++ [A Quick Guide]](/_next/image?url=%2Fimages%2Fposts%2Fl%2Fliterals-in-cpp.webp&w=1080&q=75)
Performance Considerations
Time Complexity of `erase`
The performance of the `erase` function can vary significantly depending on the container type:
- For `std::vector`, the time complexity is O(n) in the worst case, as elements may need to be shifted.
- For `std::list`, the complexity is O(1) after finding the element, since lists maintain pointers to their nodes.
- For `std::map` and `std::set`, the complexity is O(log n) because of the underlying balanced tree structure.
Best Practices for Using `erase`
It's crucial to be mindful when using `erase`, especially in loops. Here are a few best practices:
- Avoid calling `erase` within loops that iterate through your container, which can lead to stale iterators.
- Consider collecting elements to be removed and erasing them in a second pass.
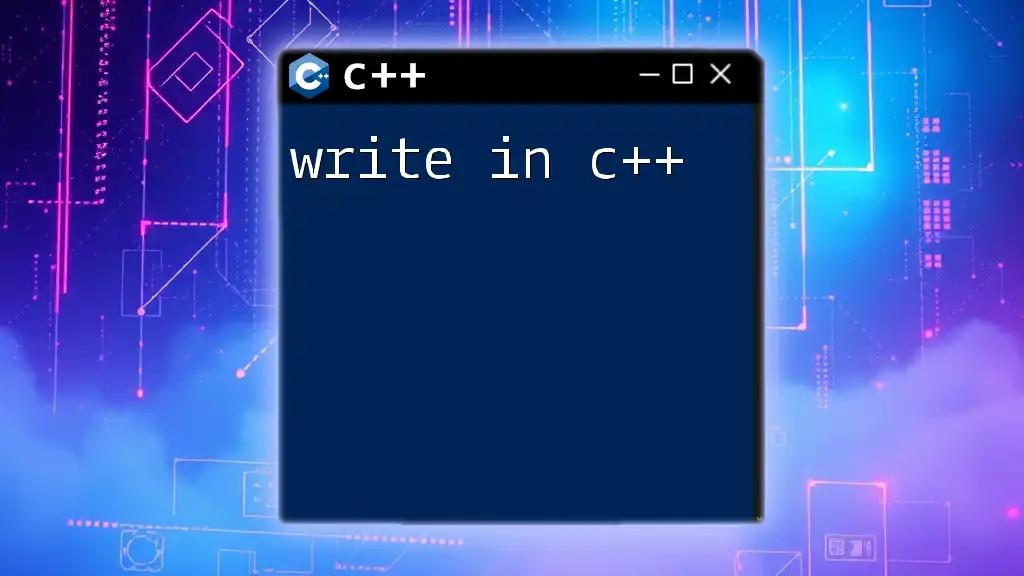
Common Pitfalls
Forgetting to Update Iterators
One common mistake is assuming that iterators remain valid after an `erase`. For example:
auto it = vec.begin();
vec.erase(it); // Iterator is invalidated after this
Attempting to use `it` after this line will cause undefined behavior.
Modifying Containers While Iterating
Altering a container while iterating through it can result in unexpected behavior or crashes. Instead, consider using algorithms like `std::remove_if` combined with `erase`. This allows for safe modifications without directly affecting the iterator being used. Here’s a basic example:
vec.erase(std::remove(vec.begin(), vec.end(), value), vec.end());
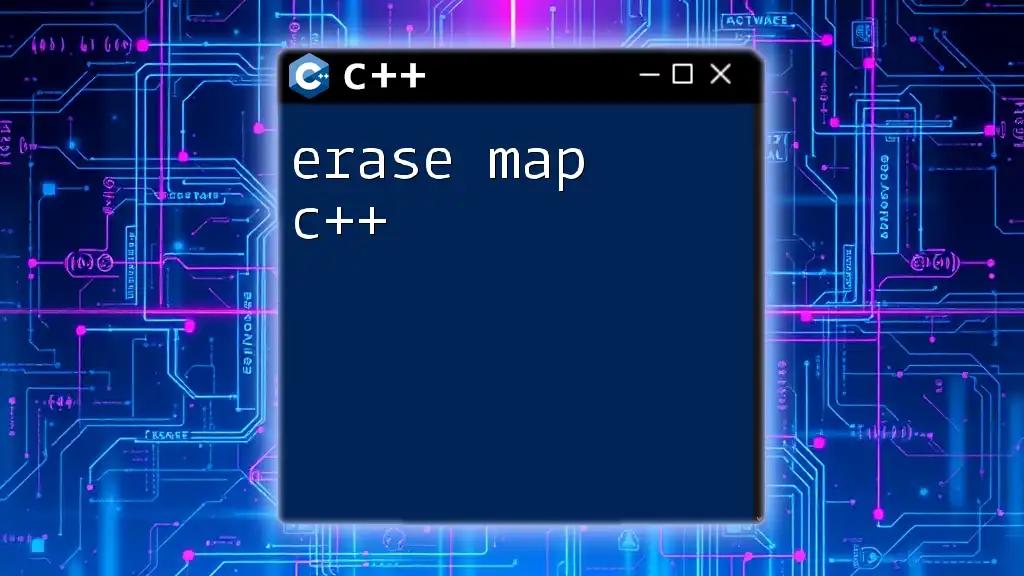
Conclusion
The `erase` function is a versatile tool in C++ that allows for effective manipulation of elements within various data structures. Understanding how to utilize it efficiently across different containers will enhance your programming capabilities. Practice using `erase` in your coding endeavors, and you'll find it to be an invaluable asset in your C++ toolkit.
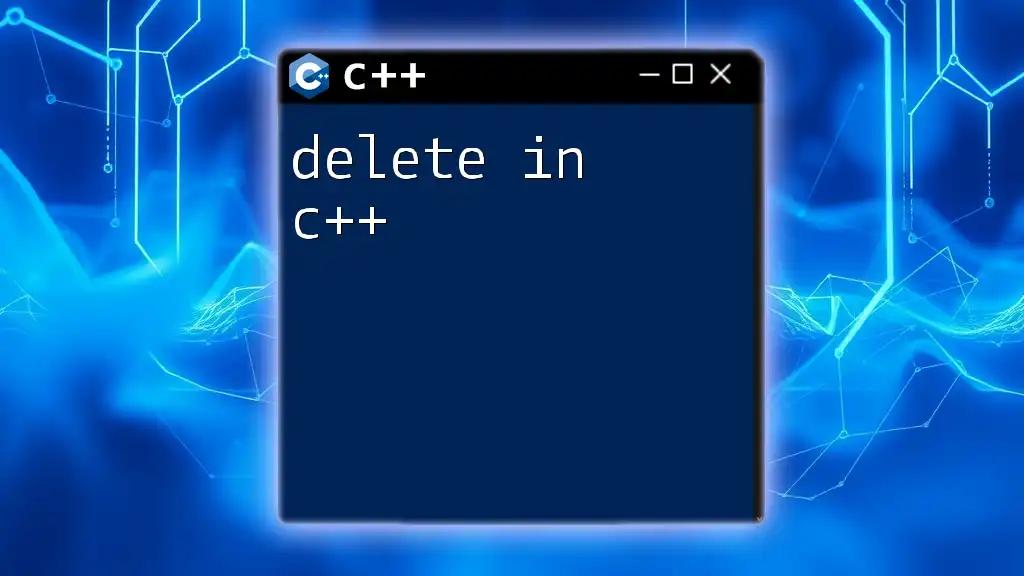
Additional Resources
For further exploration, consider diving into the following resources:
- C++ Standard Library documentation
- Online coding platforms for hands-on experimentation
- C++ programming blogs and tutorials focusing on STL containers and algorithms