In C++, the `erase` method is used to remove elements from a map, either by specifying a key or by providing an iterator to the element.
Here’s a code snippet demonstrating how to erase an element from a map using a key:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
myMap[1] = "One";
myMap[2] = "Two";
myMap[3] = "Three";
myMap.erase(2); // Erase element with key 2
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
What is a Map in C++?
A map in C++ is a versatile associative container that stores elements as key-value pairs. It effectively associates unique keys with corresponding values, ensuring efficient retrieval, insertion, and deletion of elements. The flexibility and speed of maps make them ideal for various programming tasks, such as creating lookup tables, managing relationships between data, and implementing algorithms that require sorted data.
Why Use Maps?
Maps provide several advantages over other data structures like arrays or lists:
- Fast retrieval: Maps utilize a balanced tree structure, allowing for logarithmic time complexity, O(log n), for most operations, ensuring quick access to data.
- Associativity: Since maps store data as key-value pairs, data retrieval is straightforward, making maps intuitive and easy to use.
- Sorting: Maps are sorted based on their keys, which can simplify various data management tasks.
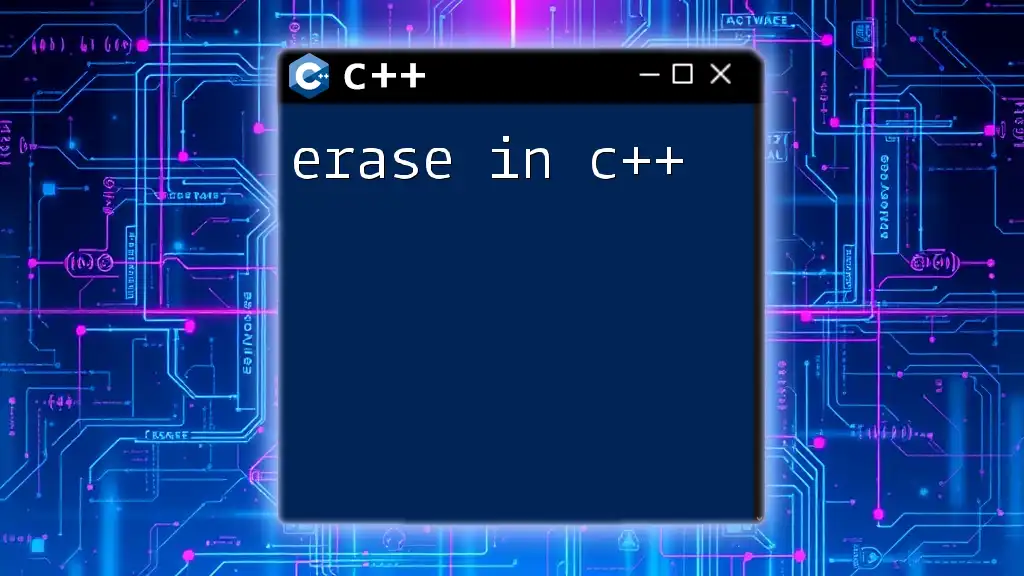
Understanding Map Operations
To effectively manage the contents of a map, understanding its various operations is crucial. Common operations include:
- Insert: Adding new key-value pairs to the map.
- Access: Retrieving the value associated with a specific key.
- Delete and Erase: Removing elements from the map, which we will focus on in this article.
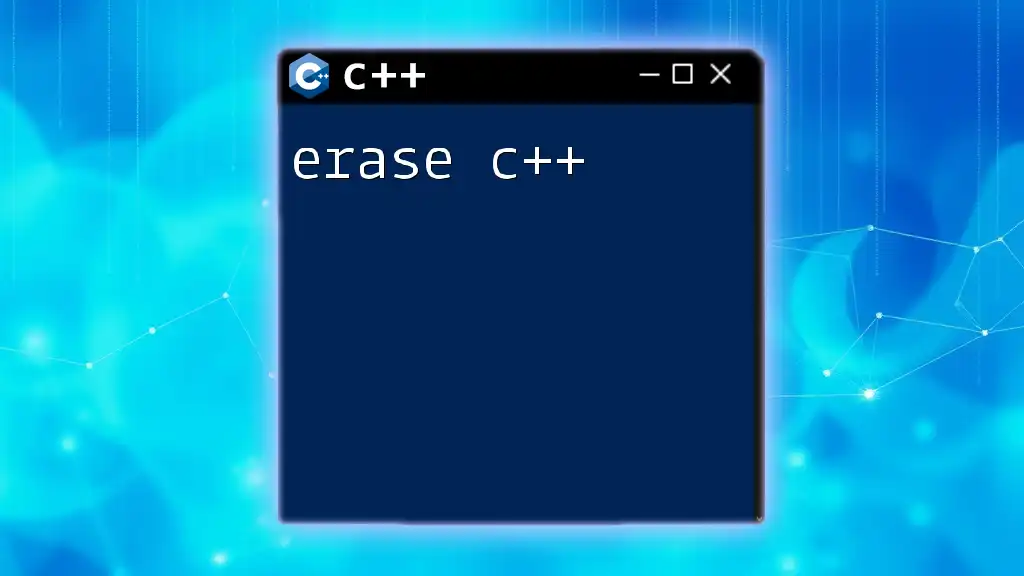
The `erase` Function in C++
Introduction to the `erase` Method
The `erase` function is a powerful tool in C++ for removing elements from a map. The functionality provided by this method ensures that you can efficiently eliminate unnecessary data, maintaining an optimal map structure.
Syntax of `map::erase`
The syntax of the `erase` function varies depending on how you wish to identify the elements to be deleted:
- To erase a single element by key: `myMap.erase(key)`
- To erase an element using an iterator: `myMap.erase(iterator)`
- To erase a range of elements: `myMap.erase(startiterator, enditerator)`
Each of these approaches has its own utility depending on the context.
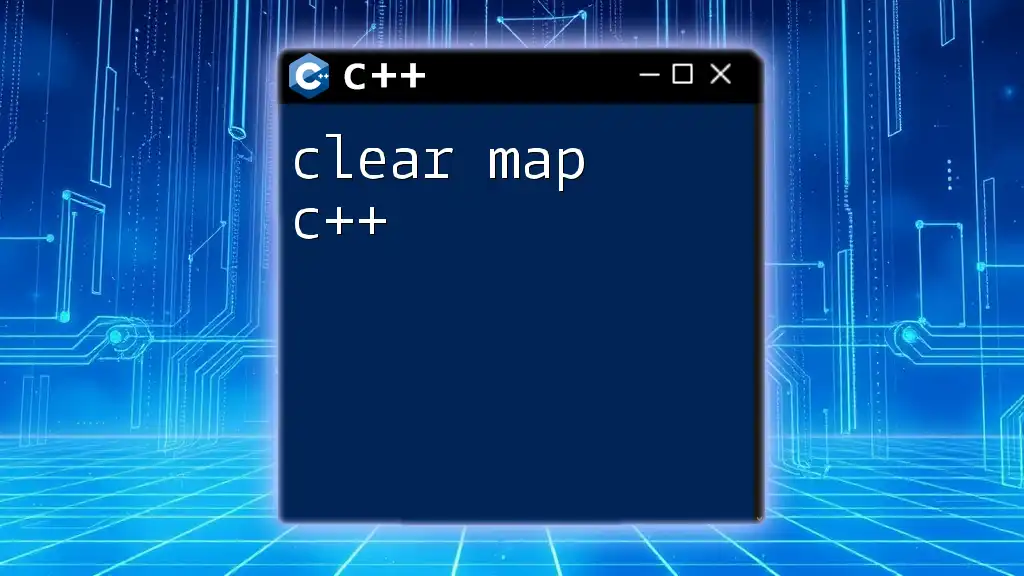
Erasing Elements from a C++ Map
Erasing by Key
You can erase an element from a map by specifying its key directly. This method is straightforward and commonly used.
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
myMap[1] = "One";
myMap[2] = "Two";
myMap.erase(1); // Erases the element with key 1
// Now myMap contains only {2: "Two"}
return 0;
}
In this example, we first add key-value pairs to `myMap`, then remove the element with the key `1`. After executing this code, only the entry `{2: "Two"}` remains.
Erasing by Iterator
Another approach is to use iterators for more complex manipulations, especially when you're not sure of the keys you want to erase.
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap{{1, "One"}, {2, "Two"}, {3, "Three"}};
auto it = myMap.find(2); // Find the iterator for key 2
if (it != myMap.end()) {
myMap.erase(it); // Erases the element pointed by the iterator
}
// Now myMap contains {1: "One", 3: "Three"}
return 0;
}
In this code snippet, we search for the key `2` using `find`, which returns an iterator pointing to the desired element. If the iterator is valid (not at the end of the map), we proceed to erase the element.
Erasing a Range of Elements
C++ allows you to remove multiple consecutive elements by specifying a range of iterators. This can be particularly useful when you want to delete a subset of elements.
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap{{1, "One"}, {2, "Two"}, {3, "Three"}};
myMap.erase(myMap.find(1), myMap.find(3)); // Erases elements with keys 1 and 2
// Now myMap contains only {3: "Three"}
return 0;
}
In this example, we use `find` to locate two elements, erasing all elements in the specified range. As a result, only the entry `{3: "Three"}` remains in the map.
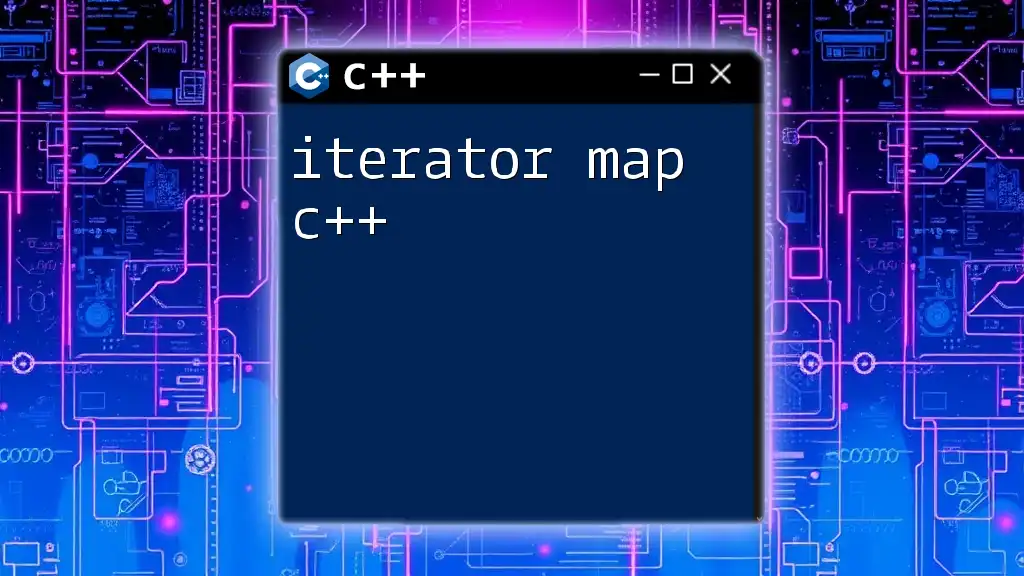
Special Cases and Considerations
What Happens When Key is Not Found?
An important point to note when using `erase` is that if the specified key does not exist in the map, calling `erase` will have no effect, and the map remains unchanged. This can simplify error handling since there are no exceptions thrown.
Performance Considerations
The performance of the `erase` operation varies depending on how the map is implemented. In most standard implementations, the time complexity of `erase` for a key or iterator is O(log n). However, erasing a range of elements may have a more significant impact on performance, especially if it involves many deletions.
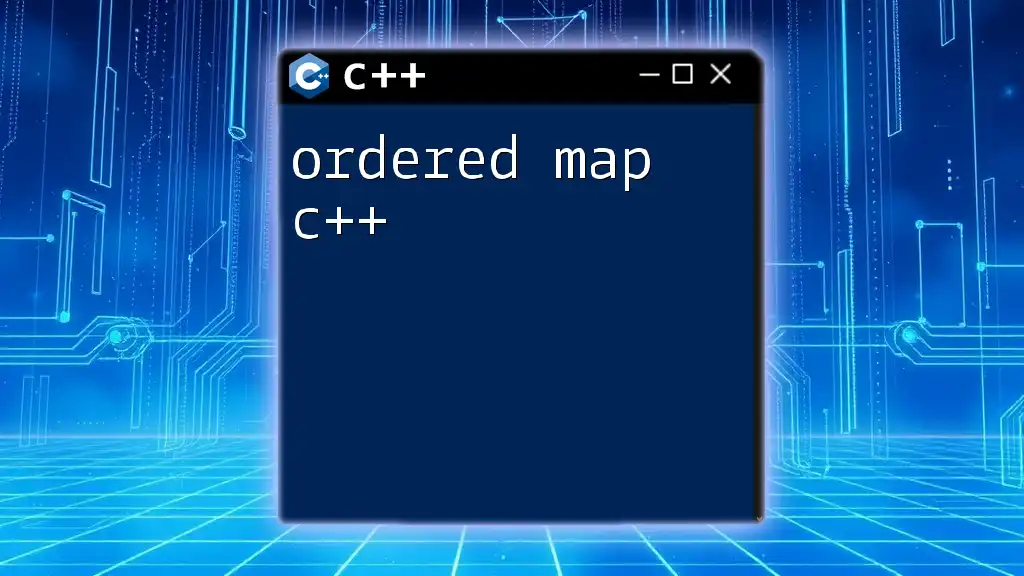
Real-world Applications of Map Erase
Use Cases for Map Erase
The ability to remove elements from a map is essential in various software development scenarios. For instance, you might need to clear outdated data in a caching system, remove elements based on specific business logic, or manage user sessions in web applications.
Best Practices for Using `map::erase`
When working with `map::erase`, consider the following best practices:
- Iterate carefully: When erasing elements while iterating, it's crucial to use iterators to avoid invalidating the loop.
- Check existence: While passing a key to `erase`, it doesn't hurt to check if the key exists beforehand, especially when logic relies on key presence.
- Optimize for bulk operations: If you need to remove many elements, consider optimizing your approach to enhance performance, such as batching deletions.
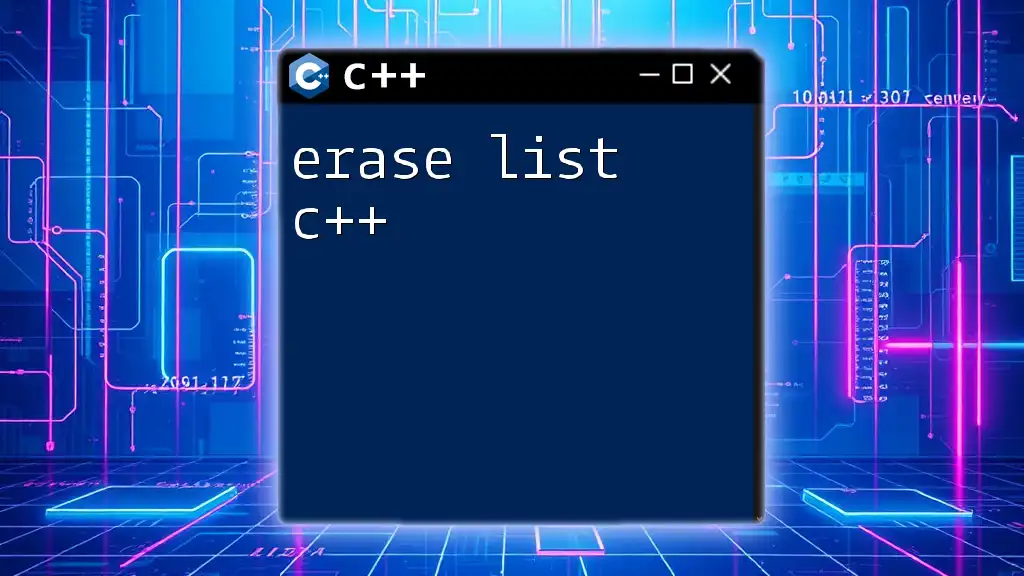
Conclusion
Using the `erase` function in C++ maps is an essential skill for developers looking to manage data effectively within their applications. This article provided a comprehensive overview of how to erase map elements, supported by practical code examples to enhance understanding. Mastering these operations can significantly improve data integrity and the overall performance of your C++ applications.
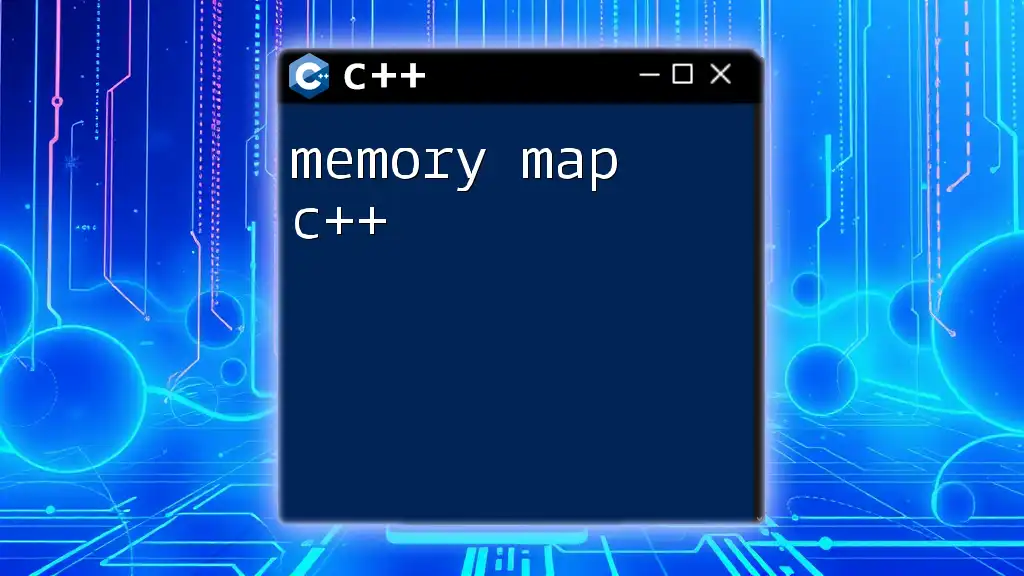
Additional Resources
For those eager to dive deeper, consider accessing C++ documentation or exploring related articles about map operations. Practical exercises focused on real-world applications will further reinforce your understanding of map functionalities and enable you to leverage these skills in your programming projects.