Creating a simple C++ game can be as straightforward as implementing a text-based adventure where users navigate through choices using conditional statements. Here’s a basic example:
#include <iostream>
using namespace std;
int main() {
int choice;
cout << "Welcome to the Adventure Game!" << endl;
cout << "1. Go to the forest" << endl;
cout << "2. Go to the cave" << endl;
cout << "3. Go home" << endl;
cout << "Choose an option (1-3): ";
cin >> choice;
switch (choice) {
case 1:
cout << "You encounter a wild animal!" << endl;
break;
case 2:
cout << "You find a treasure chest!" << endl;
break;
case 3:
cout << "You are safe at home." << endl;
break;
default:
cout << "Invalid choice!" << endl;
}
return 0;
}
Setting Up Your Development Environment
Before you dive into the exciting journey of game development, it’s essential to set up your development environment properly. This ensures you have all the tools required to efficiently create a C++ game.
Choosing a C++ IDE
Selecting a compatible Integrated Development Environment (IDE) is vital. Popular choices include:
- Visual Studio: Widely used for C++ development; it supports powerful debugging and has a rich feature set.
- Code::Blocks: An open-source IDE that's lightweight and easy to use.
Once you've chosen your IDE, follow the installation instructions provided on their official websites to get started. Make sure to configure your IDE to target the correct framework for game development.
Installing Necessary Libraries and Tools
C++ relies heavily on libraries for graphics rendering and sound management. Two widely used libraries are:
- SFML (Simple and Fast Multimedia Library) for handling graphics, sounds, and window management.
- SDL (Simple DirectMedia Layer), which is more extensive and often used in larger projects.
To install these libraries, refer to their documentation for detailed steps on configuration specific to your operating system and chosen IDE.
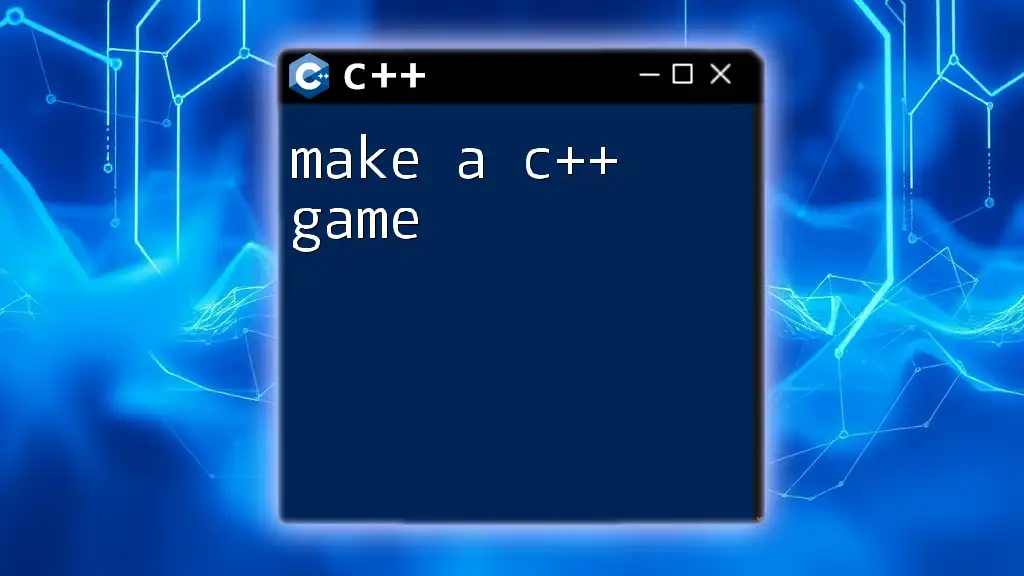
Understanding the Basics of Game Programming
Before venturing into coding, it's crucial to grasp some core concepts fundamental to game development:
Core Concepts of Game Development
One of the central components of any game is the game loop. This continuous loop processes user input, updates game state, and renders graphics.
Another important aspect is event handling, which deals with player interactions, such as keyboard or mouse inputs. You’ll need to develop an efficient way to capture and respond to these events, ensuring a responsive gameplay experience.
Game states are also key; they represent different phases of your game, such as loading, in-game, or game-over. By managing these states effectively, you create a smooth transition between different parts of your game.
Essential C++ Concepts for Game Development
Familiarizing yourself with Object-Oriented Programming (OOP) is crucial, as it allows you to create reusable code and design complex game mechanics with classes and objects.
Data structures and algorithms are also important to understand, as they optimize how you manage game data, like player stats, inventory systems, or enemy behaviors.
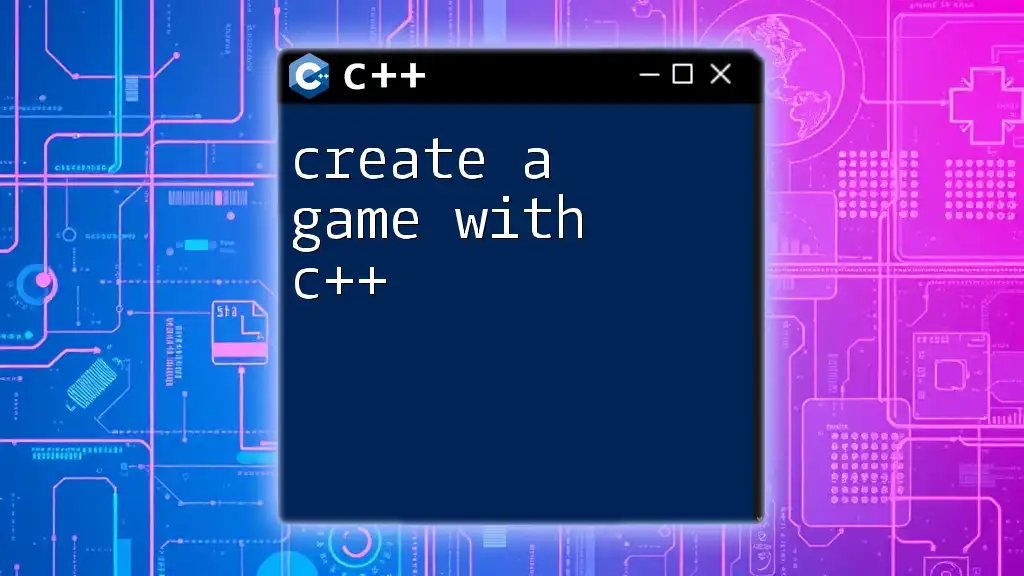
Creating Your First Simple C++ Game
Game Idea and Conceptualization
Before you write any code, it’s beneficial to brainstorm your game idea. Think about creating a simple 2D game like a platformer or an arcade shooter. Define the game genre and its mechanics to give your project direction.
Setting Up Your Project Structure
Once your concept is settled, it’s time to organize your project. A clear file structure ensures that you can easily navigate your project as it grows. Common directories include:
- src/: For source code
- assets/: For images and sounds
- include/: For headers
Consistent naming conventions help maintain clarity and organization across your project.
Implementing the Game Logic
The heart of your game lies in its logic. Begin implementing the game loop. Here’s a basic structure:
while (gameRunning) {
handleInput();
updateGameLogic();
renderGraphics();
}
In this snippet, `handleInput`, `updateGameLogic`, and `renderGraphics` are functions you will define, each serving a different role in the game loop.
Handling User Input
Creating responsive controls is vital. For example, to capture player input, you can use the following code snippet:
if (isKeyPressed(KEY_ESCAPE)) {
gameRunning = false; // Example of handling quit input
}
This simple input handling showcases how to listen for a specific key press, indicating that it's time to exit the game.
Building Game Assets
Creating visual and audio assets is an integral part of game development. You can use software like GIMP for 2D graphics or Blender for 3D models. Ensure your assets fit the style and theme of your game to enhance the immersive experience.
To add sound effects or music, use audio libraries such as FMOD or OpenAL. Here's how you could implement a simple audio function in your game:
playSound("jump.wav"); // Play the jump sound
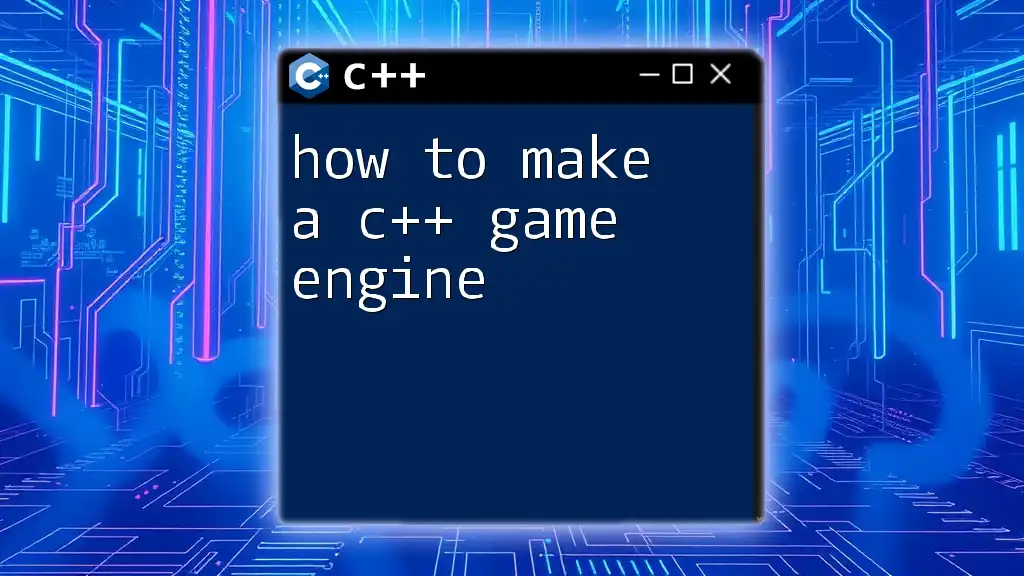
Developing Game Features
As you gain more confidence, you can start developing essential game features.
Creating a Character or Player Class
You’ll likely have multiple entities in your game, such as players and enemies. A class can encapsulate the functionality of your player. For example:
class Player {
public:
void move(int x, int y);
void jump();
};
This `Player` class outlines basic functionality you would implement further, such as player movement or jumping mechanics.
Implementing Game Mechanics
Incorporating collision detection is a key feature of any interactive game. Start with simple bounding box collisions, and as you become more comfortable, explore more advanced techniques. Additionally, consider implementing a scoring system that keeps track of points earned throughout the game, enhancing player engagement.
Adding User Interface Elements
A User Interface (UI) enhances the gaming experience by providing important information to players. You can create menus, health bars, and score displays using UI libraries. Here’s an example of how to display a score on the screen:
drawText("Score: " + std::to_string(score), x, y);
This code snippet dynamically shows the player's current score, keeping them engaged in the gameplay.
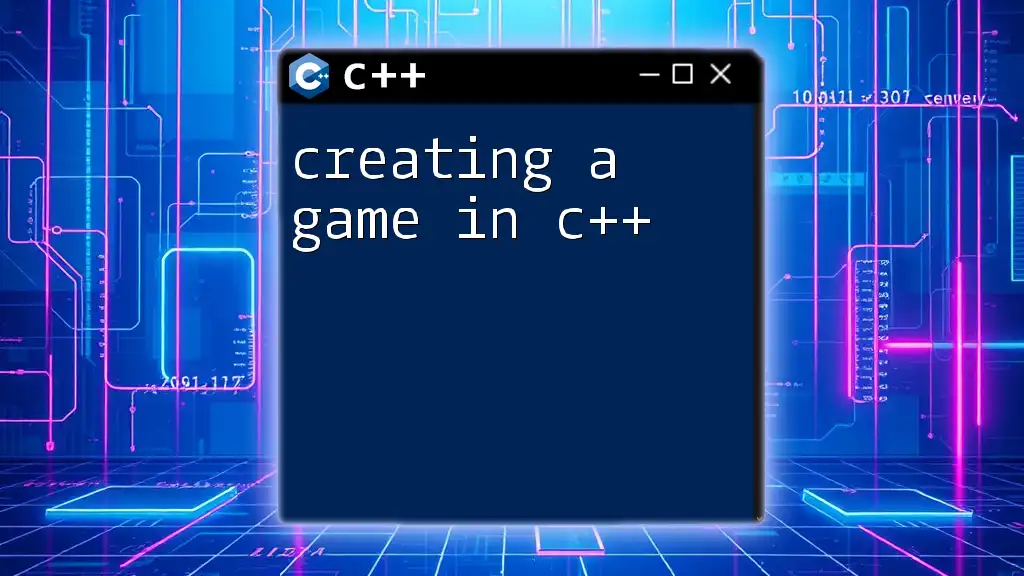
Testing and Debugging Your Game
Testing is crucial to ensure a smooth gaming experience. Identify bugs and inconsistencies that may disrupt gameplay and refine the code accordingly. Utilizing debugging tools specific to your IDE will significantly assist you in finding and fixing issues.
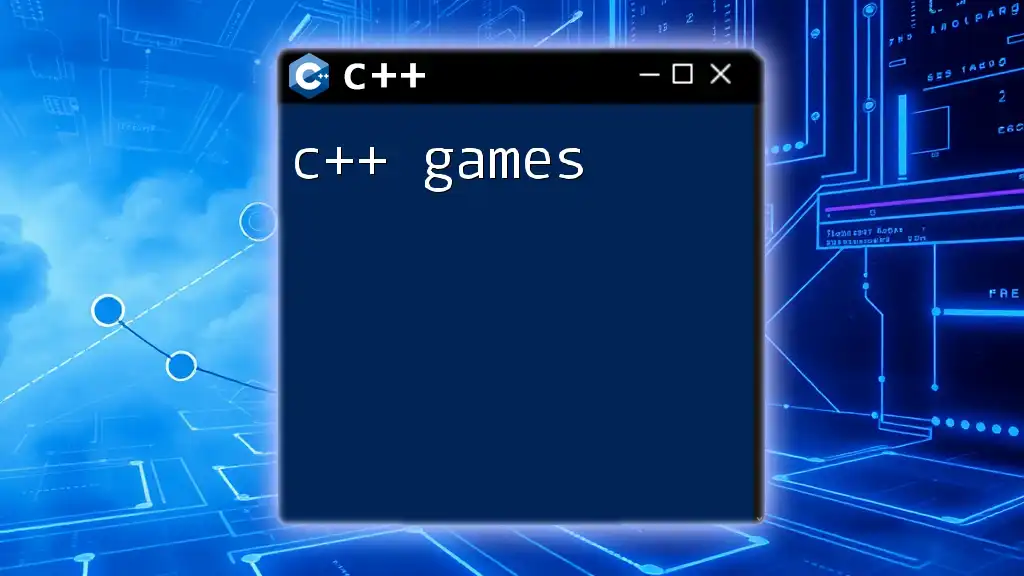
Finalizing Your Game for Release
As your game approaches completion, prepare it for public release. Decide on platforms, such as Windows or Linux, and build your game to create an executable.
Lastly, focus on polishing graphics and sound. A polished game attracts more players and can substantially improve the overall experience.
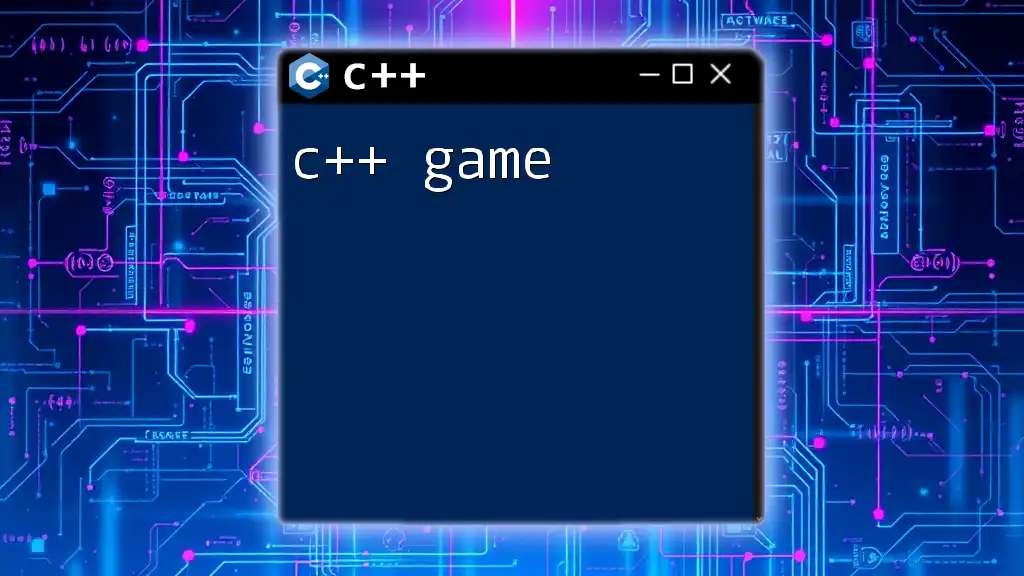
Conclusion
Creating a C++ game is a rewarding endeavor, combining creativity and technical skill. By following structured steps, from setting up your environment to finalizing your product, you can successfully bring your game idea to life.
Continue pushing your limits and exploring new technologies in game development. The world of C++ is vast, and there’s always more to learn and experiment with.
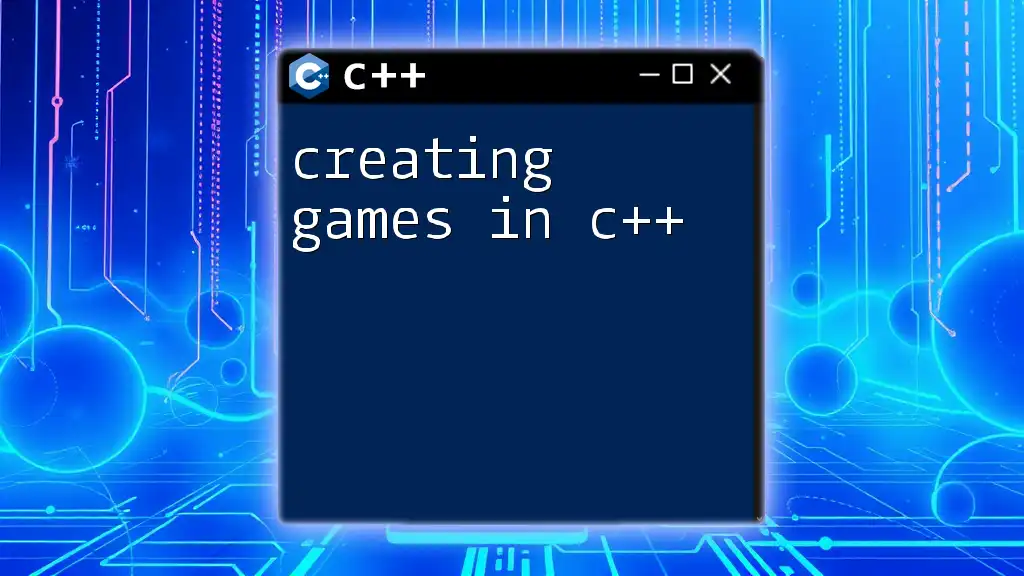
Call to Action
Join our community for more insights into C++ game development and keep expanding your skills. Check out our courses for in-depth learning and expert guidance as you embark on your game development journey!