C++ game development allows programmers to create interactive experiences by utilizing various libraries and techniques, making it easy to implement graphics, sound, and gameplay logic.
Here's a simple example of a C++ program that initializes a window for a game using the SFML library:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "C++ Game");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.display();
}
return 0;
}
Getting Started with C++
Setting Up the Development Environment
To dive into C++ game development, you first need to set up your development environment. This involves choosing a suitable Integrated Development Environment (IDE) and installing the necessary compilers.
Popular IDEs include Visual Studio, which provides robust debugging features, Code::Blocks, known for its simplicity, and CLion, offering powerful code assistance and integration. When it comes to compilers, the GCC (GNU Compiler Collection) is often recommended due to its cross-platform support, while MSVC (Microsoft Visual C++) is widely used for Windows applications.
Understanding Basic Syntax and Structure
Start your journey by writing your first C++ program. This foundational step helps you grasp essential syntax and structure.
#include <iostream>
int main() {
std::cout << "Hello, Game Development!" << std::endl;
return 0;
}
In this snippet, `#include <iostream>` allows for input-output operations, and the `main` function serves as the entry point of the program. This demonstrates important concepts, including functions, variables, and data types.
Basic control structures like if statements, switch cases, and loops form the backbone of your game logic, allowing for decision-making and repetitive tasks essential in game mechanics.
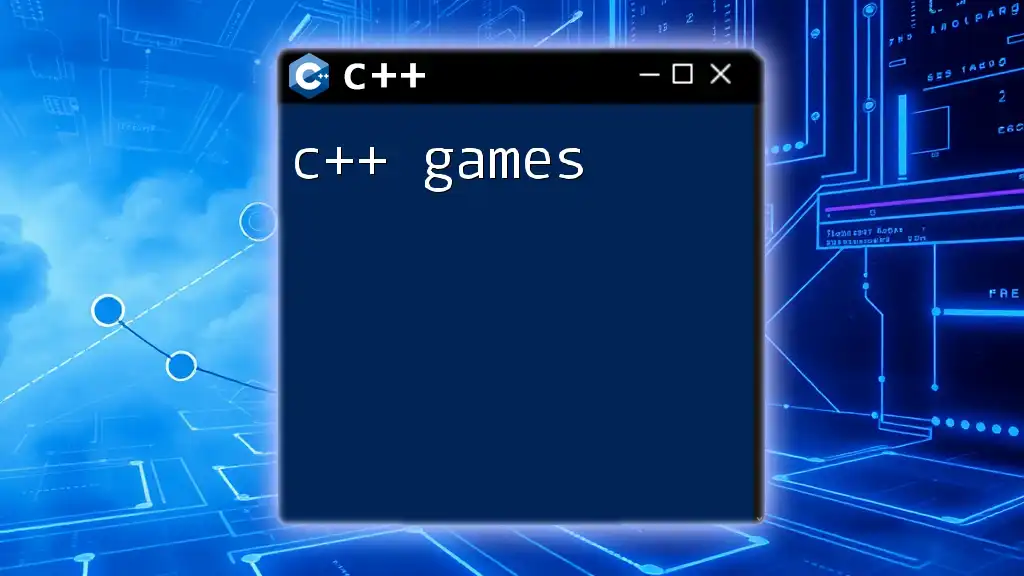
Fundamentals of Game Development
Key Concepts in Game Development
Understanding the Game Loop is vital to C++ game development—it’s the core structure that enables your game to run continuously. A typical game loop resembles the following:
while (gameIsRunning) {
processInput();
updateGameLogic();
renderFrame();
}
This structure allows for real-time gameplay, processing inputs, updating the game's logic, and rendering frames to the screen in a seamless manner.
Game State Management is another critical aspect. This involves creating distinct game states like menu, playing, or paused and effectively transitioning between them. Proper management of game states enhances user experience and flow within the game.
Graphics and Game Engines
Choosing the right Game Engine is integral to your development process. C++ is commonly used within powerful engines such as Unreal Engine, known for its stunning graphics and robust tools.
If you’re starting with graphics programming in C++, consider integrating a library like SFML (Simple and Fast Multimedia Library) or SDL (Simple DirectMedia Layer). Here’s a brief example using SFML to create a window:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "Basic Window");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.display();
}
return 0;
}
In this snippet, we create a simple window and set up an event loop that allows the window to respond to user interactions, demonstrating the fundamental operations for any C++ game.
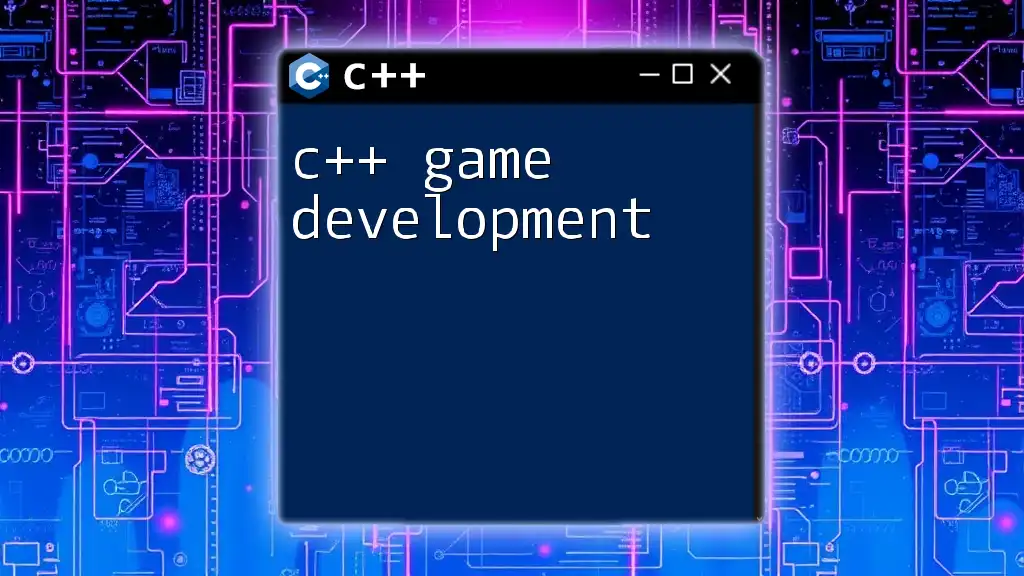
Game Development Concepts in C++
Object-Oriented Programming (OOP) in Game Development
C++'s Object-Oriented Programming (OOP) capabilities allow you to design games more structurally. By defining Classes and Objects, you can model real-world entities and behaviors efficiently. OOP promotes code reuse and scalability.
For instance, consider a simple `Player` class:
class Player {
public:
int health;
void move(int x, int y);
};
This design encapsulates player attributes and behaviors, allowing easy extension and modification.
Inheritance and Polymorphism are OOP principles that can be exploited for game development to create diverse character types with shared traits.
Memory Management
In C++, understanding Dynamic Memory Allocation is crucial. Using pointers facilitates the creation and management of objects at runtime. Here’s an example of how to allocate and delete memory:
Player* player1 = new Player();
delete player1;
This snippet illustrates creating a new player object and ensuring memory is deallocated, which is essential to prevent memory leaks.
Smart Pointers—like `std::unique_ptr` and `std::shared_ptr`—help manage memory automatically. They reduce common issues associated with manual memory management, making your C++ game more robust.
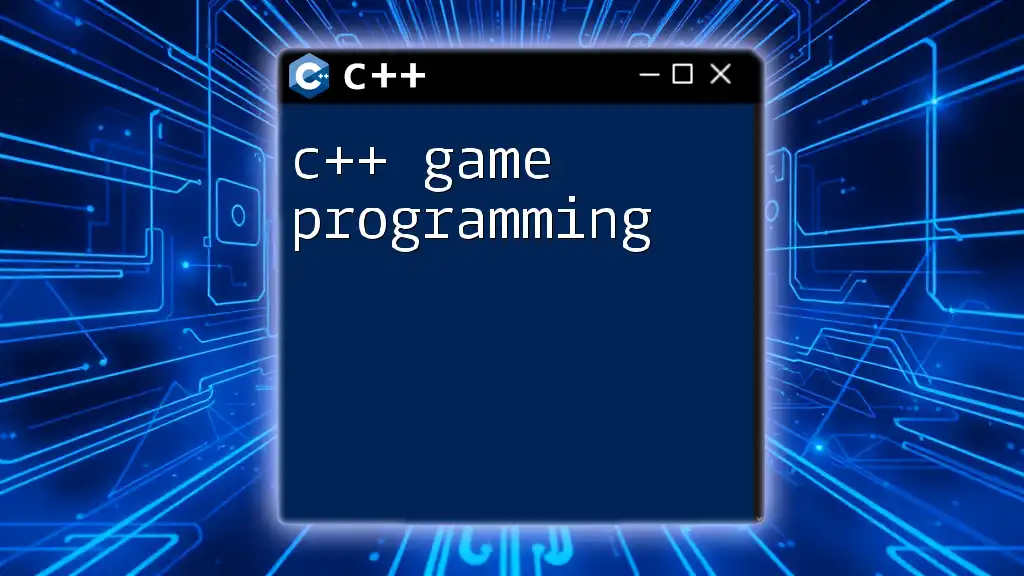
Game Features and Mechanics
Implementing Player Controls
Effective user interactions are paramount in any C++ game. You will need to handle Keyboard and Mouse Input efficiently. Libraries like SFML make this straightforward, allowing you to capture player actions seamlessly.
Game Physics Basics
Integrating a Physics Engine is often necessary for realistic movements and interactions within your game. Popular engines like Box2D or Bullet Physics provide the tools needed to handle physics without having to code from scratch.
Building User Interfaces
Creating intuitive menus and in-game Heads-Up Displays (HUDs) adds significant value to player experience. Libraries like ImGui allow for easy customization and rapid development of user interfaces.
ImGui::Begin("Menu");
ImGui::Text("Play");
ImGui::End();
This snippet demonstrates how to create a simple menu using ImGui, highlighting the flexibility and power of this library for developing interactive UIs.
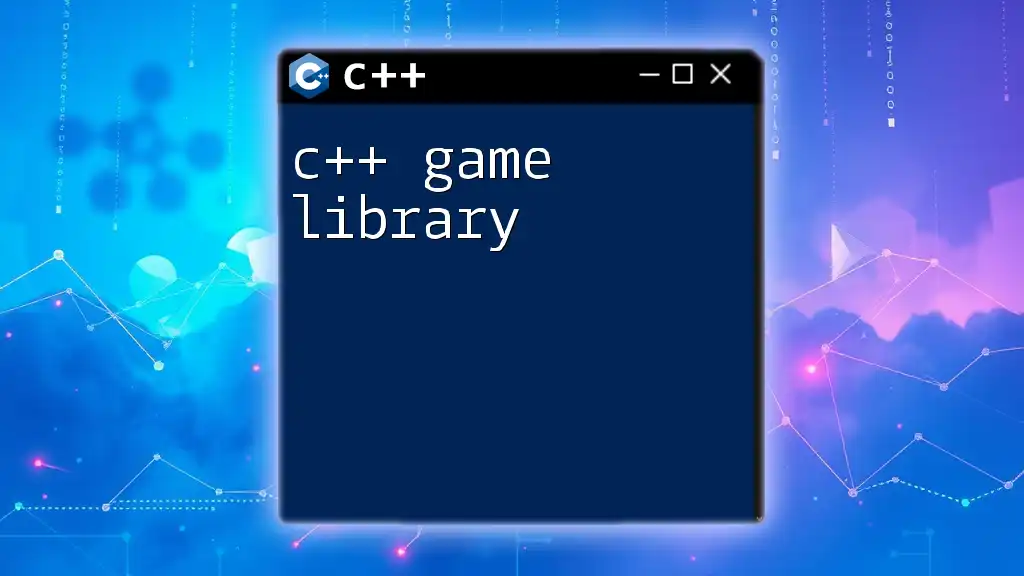
Testing and Debugging
Common Errors in C++
As you develop your C++ game, it’s essential to understand the difference between Compilation Errors and Runtime Errors. Compilation errors occur when the code violates language rules, while runtime errors occur during the execution of a program.
Debugging Techniques
Utilize Debugging Tools such as gdb or the Visual Studio Debugger to pinpoint issues. These tools help you inspect variables, control flow, and overall game performance, providing insights to refine your code.
Playtesting and Feedback Loop
Engaging in playtesting is vital. Gathering user feedback can help identify gameplay issues and improvement areas. Iterative development strategies allow you to refine your C++ game based on real player experiences, ultimately enhancing quality.
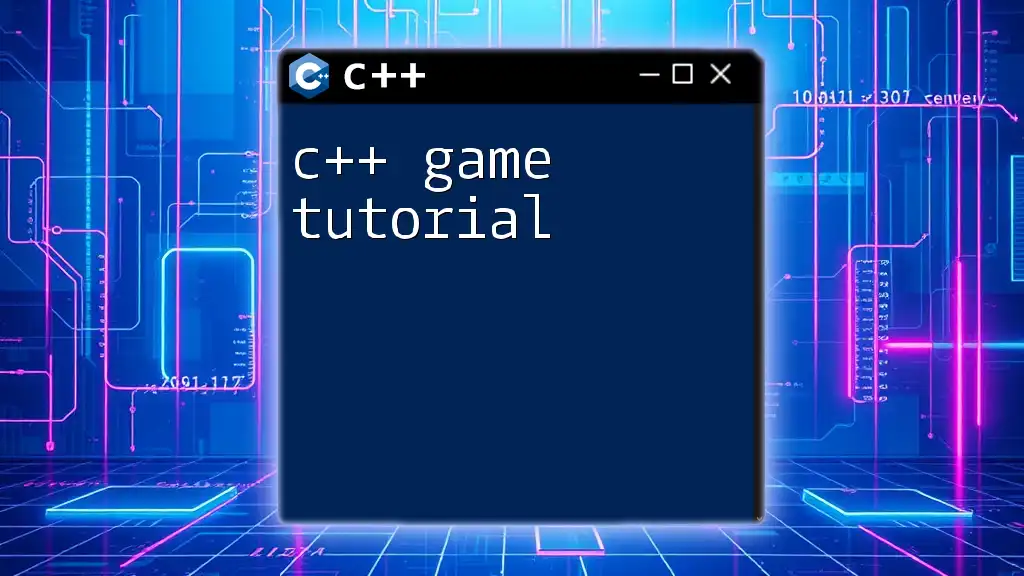
Conclusion
Recapping the essentials covered in this guide, we have explored several aspects crucial to C++ game development. From setting up your development environment to integrating libraries, managing game states, and debugging, understanding these elements will lay a strong foundation for your journey.
Call to Action
If you're looking to deepen your skills or need assistance, we offer tutorials and courses focused on C++ games tailored to different skill levels. Connect with us and elevate your game development skills to the next level!
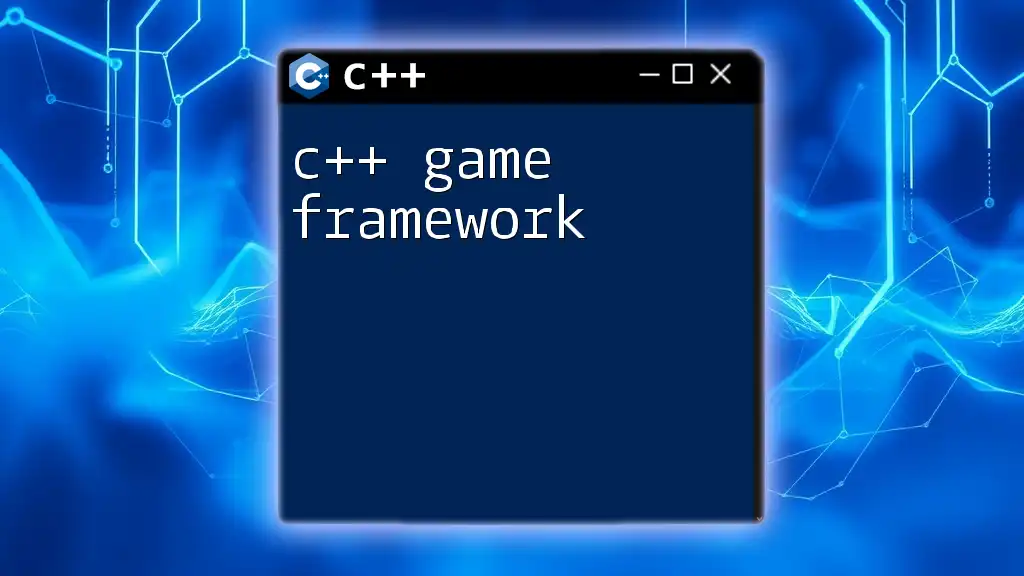
Additional Resources
For further learning, consider exploring books, tutorials, and online platforms that specialize in C++ game development. Engage with the community through forums like Stack Overflow and GameDev.net to share ideas and gain insights.
Embarking on a C++ game development journey can be challenging yet rewarding. With patience and persistence, you can create captivating experiences for players to enjoy!