A C++ game library is a collection of pre-written code that simplifies the game development process by providing tools and functions for graphics, sound, and input handling.
Here’s a simple example of using the SFML library to create a window:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "My Game");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.display();
}
return 0;
}
What are C++ Game Libraries?
C++ game libraries are collections of pre-written code that provide developers with tools and functionalities for game development. These libraries streamline the development process by offering features such as graphics rendering, sound, input management, and physics simulations. Using a C++ game library can significantly reduce the time it takes to develop a game and allows developers to focus more on creating unique gameplay experiences.
Why Choose C++ for Game Development?
C++ remains one of the top choices for game development due to several compelling reasons:
- Performance and Efficiency: C++ allows developers to write high-performance applications with fine-grained control over system resources.
- Object-Oriented Programming Advantages: C++ supports object-oriented programming, which promotes cleaner code organization and reusability. This is beneficial in large-scale game projects where maintaining code is critical.
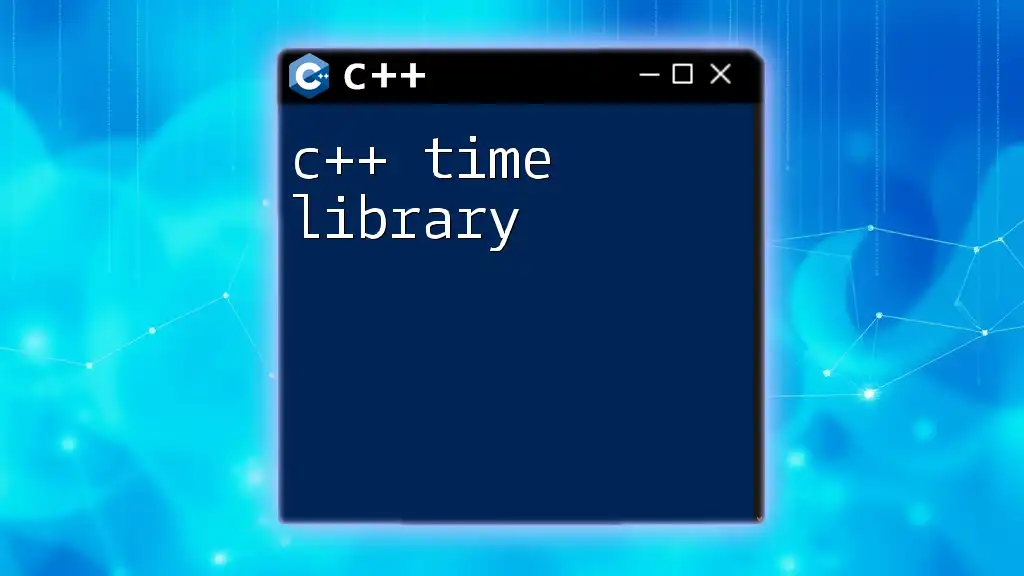
Top C++ Game Libraries
A variety of C++ game libraries cater to different needs and preferences. Here we take a closer look at some of the most popular options.
SDL (Simple DirectMedia Layer)
Introduction to SDL
SDL is a cross-platform library that provides low-level access to audio, keyboard, mouse, joystick, and graphics hardware via OpenGL and Direct3D. It is lightweight and suitable for 2D games.
How to Set Up SDL in Your Project
To start using SDL, you need to download the library from the official SDL website and follow the installation guide for your operating system. Doing so typically involves linking the SDL libraries in your project configuration.
Basic SDL Example
Here’s a simple code snippet to create a basic window with SDL:
#include <SDL.h>
int main(int argc, char* argv[]) {
SDL_Init(SDL_INIT_VIDEO); // Initialize SDL
SDL_Window* window = SDL_CreateWindow("Hello SDL", SDL_WINDOWPOS_CENTERED, SDL_WINDOWPOS_CENTERED, 640, 480, 0);
SDL_Delay(3000); // Keep window open for 3 seconds
SDL_DestroyWindow(window);
SDL_Quit();
return 0;
}
In this example, we initialize SDL, create a window, and keep it open for three seconds before closing it. Key functions like `SDL_Init`, `SDL_CreateWindow`, and `SDL_Quit` are vital for managing the lifecycle of your application.
SFML (Simple and Fast Multimedia Library)
What is SFML?
SFML is designed for ease of use in multimedia programming. It provides a simple interface to handle graphics, audio, input, and networking.
Getting Started with SFML
To use SFML, install it via a package manager or manually download it from the SFML website. Make sure to link the appropriate libraries in your project.
SFML Resource Management
Here's a basic SFML code snippet that creates a game window:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "Hello SFML");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.display();
}
return 0;
}
In this code, we create a rendering window and handle its events, showcasing how easily SFML allows for looped rendering and user interaction.
Allegro
Overview of Allegro
Allegro is a cross-platform library specifically focused on game development. It provides functionalities for graphics, sound, input, and much more.
Setting Up Allegro for Development
Download Allegro from the official site, follow the installation instructions based on your operating system, and configure it according to your project settings.
Easy Game Implementation with Allegro
Here’s how you might set up a simple game loop with Allegro:
#include <allegro.h>
int main() {
allegro_init(); // Initialize Allegro
install_keyboard(); // Start the keyboard handling
set_color_depth(32);
set_gfx_mode(GFX_AUTODETECT_WINDOWED, 800, 600, 0, 0);
while (!key[KEY_ESC]) {
clear_to_color(screen, makecol(0, 0, 0));
textout(screen, font, "Hello Allegro!", 350, 290, makecol(255, 255, 255));
rest(100);
}
return 0;
}
END_OF_MAIN();
This snippet demonstrates how to create a window and display a message, while waiting for the Escape key to close the program.
Cocos2d-x
Introduction to Cocos2d-x
Cocos2d-x is an open-source game development framework that accelerates the development of 2D games. It provides rich features like animations, scenes, and interactions.
Setting Up Cocos2d-x
Download Cocos2d-x from its official GitHub repository and follow the installation guide to set up your development environment.
Creating Your First Cocos2d-x Game
A simple Cocos2d-x example to create a basic scene might look as follows:
#include "cocos2d.h"
USING_NS_CC;
int main(int argc, char** argv) {
// Create the application instance
auto app = new AppDelegate();
return Application::getInstance()->run();
}
This code establishes the foundation for a Cocos2d-x project, demonstrating how to initiate the application.
Unreal Engine (UE4)
Overview of Unreal Engine and C++ Integration
Unreal Engine is a highly versatile game engine that supports C++ for high-performance game programming. C++ can be used for all aspects of game development, providing deep control over engine features.
Getting Started with Unreal Engine
Unreal Engine can be downloaded from the Epic Games Launcher. After setting it up, you can create a new project that enables C++.
Building a Simple Game in Unreal Engine
Here’s a basic example of creating a simple actor:
#include "GameFramework/Actor.h"
#include "MyActor.generated.h"
UCLASS()
class MYPROJECT_API AMyActor : public AActor {
GENERATED_BODY()
public:
AMyActor() {
PrimaryActorTick.bCanEverTick = true;
}
virtual void Tick(float DeltaTime) override {
Super::Tick(DeltaTime);
// Perform actions here
}
};
This example introduces a custom actor in Unreal Engine, demonstrating how to create a class derived from AActor and override its functionality.
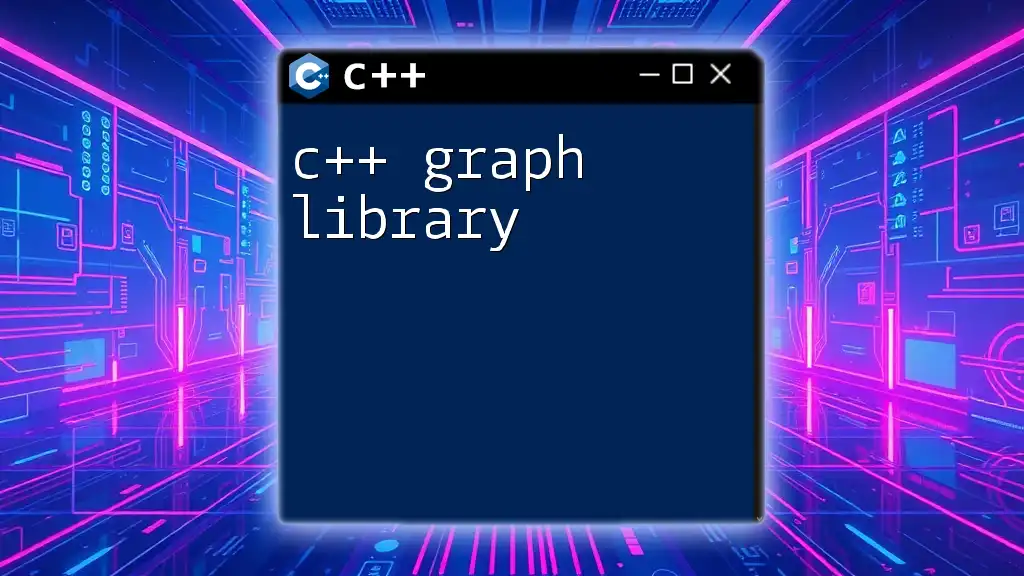
Choosing the Right Library
When selecting a C++ game library, consider factors such as:
- Types of Games: Are you developing a 2D or 3D game? Some libraries excel in specific areas.
- Performance Requirements: Analyze resource constraints and performance needs of your game.
- Community Support: A strong community around a library can be incredibly beneficial for troubleshooting and support.
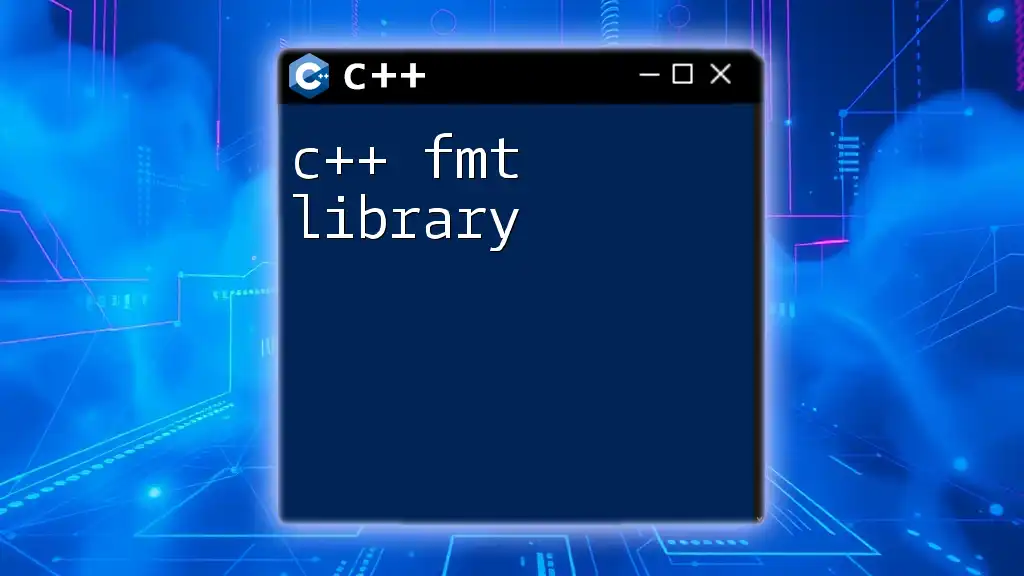
Advanced Topics in C++ Game Libraries
Integrating Graphics and Audio
Combining multiple libraries may enhance game features, such as using OpenGL or Direct3D for rendering visuals alongside dedicated audio libraries for sound management. C++ provides flexibility for such integrations.
Networking and Multiplayer Capabilities
If your game requires multiplayer functionality, C++ libraries such as Boost.Asio can be paired with game libraries for networking. This allows you to handle real-time player interactions effectively.
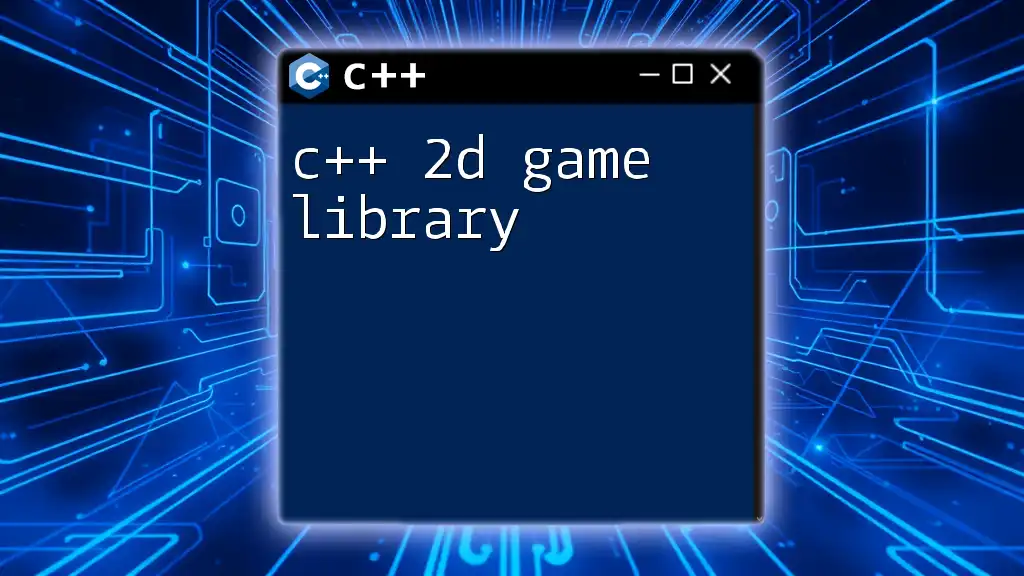
Conclusion
C++ game libraries are powerful tools that greatly enhance the game development process. They provide vital functionalities that allow developers to streamline creation and focus on developing engaging gameplay. As C++ continues to evolve, staying informed about emerging libraries and trends in game development will keep you at the forefront of the industry.
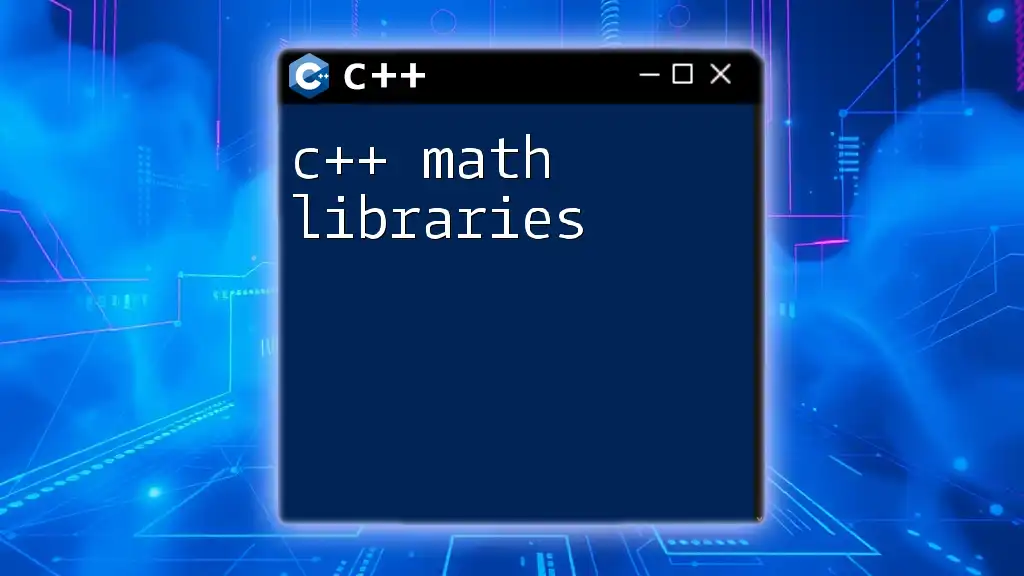
Additional Resources
To deepen your understanding of C++ game libraries, consider exploring:
- Books and Tutorials: Find materials that cover specific libraries in detail.
- Online Communities and Forums: Engage with peers on platforms like Stack Overflow or Reddit.
- Contribution Opportunities: Get involved with open-source projects to gain practical experience.