The C++ `cout` library, part of the standard I/O stream library, is used to output data to the standard console in a clean and efficient manner. Here’s a simple example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is the c++ cout Library?
The `cout` library in C++ is a part of the standard input-output library, specifically provided by `iostream`. It allows for output of data to the standard output, typically the console. The `cout` part stands for "character output," making it a fundamental tool for displaying information within a C++ program.
Why Use the c++ cout Library?
Using the `cout` library comes with several advantages:
- Simplicity: It offers an easy syntax for printing messages and variable contents to the console.
- Standardized Usage: Being part of the standard library, it adheres to C++ standards, ensuring compatibility across various platforms.
- Chaining: You can easily chain multiple output statements together, enhancing code readability.
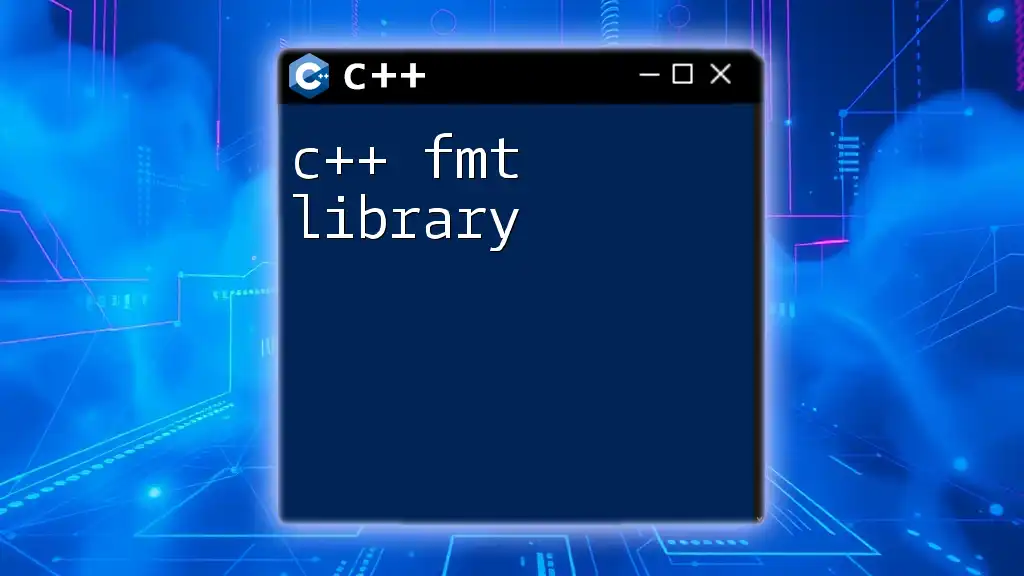
How to Include the c++ cout Library
Basic Syntax for Including cout in Your Program
To use `cout`, you need to include the header file `iostream` at the top of your program:
#include <iostream>
This inclusion allows you to leverage functionalities associated with input-output operations, particularly `cout`.
Standard Namespace
To prevent typing `std::` before every `cout` statement, you can use the `using` directive. However, it's essential to understand both methods:
std::cout << "Hello, World!" << std::endl;
versus
using namespace std;
cout << "Hello, World!" << endl;
While using the whole namespace simplifies coding, it might introduce name clashes in larger programs.
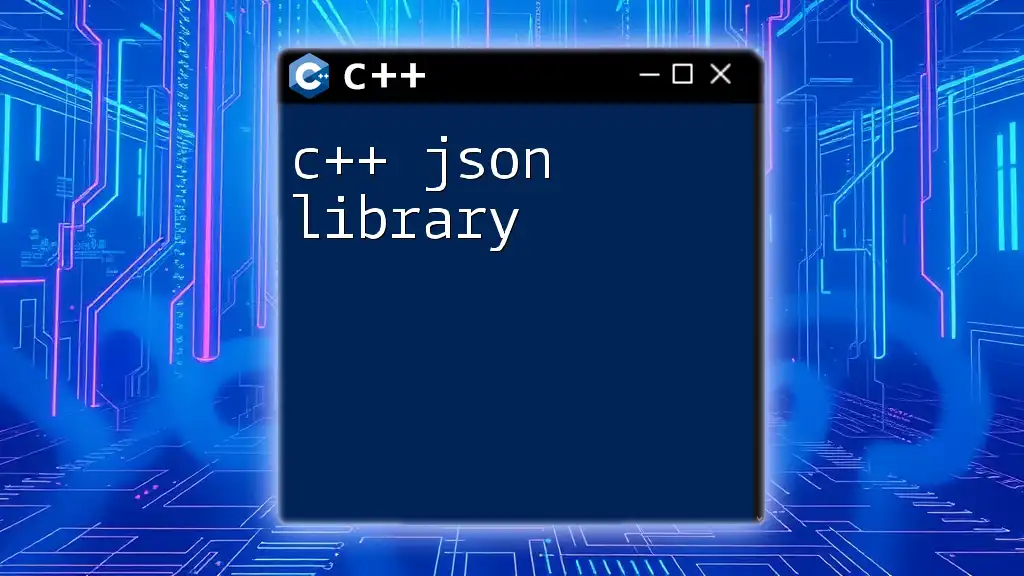
Basic Usage of the c++ cout Library
Outputting Text
The fundamental operation of `cout` is outputting string literals. The syntax is straightforward, and every output should ideally end with `std::endl`, which not only adds a new line but also flushes the output buffer:
std::cout << "This is a simple output." << std::endl;
Outputting Variables
The `cout` library allows you to output various variable types effectively. For instance:
- Integers:
int age = 25;
std::cout << "Age: " << age << std::endl;
- Floating Points:
float height = 5.9;
std::cout << "Height: " << height << " feet" << std::endl;
- Strings:
std::string name = "Alice";
std::cout << "Name: " << name << std::endl;
Using Multiple Outputs
Another powerful aspect of `cout` is its ability to chain multiple outputs. This feature allows you to format strings and variables in a single line seamlessly.
std::cout << "Name: " << name << ", Age: " << age << std::endl;
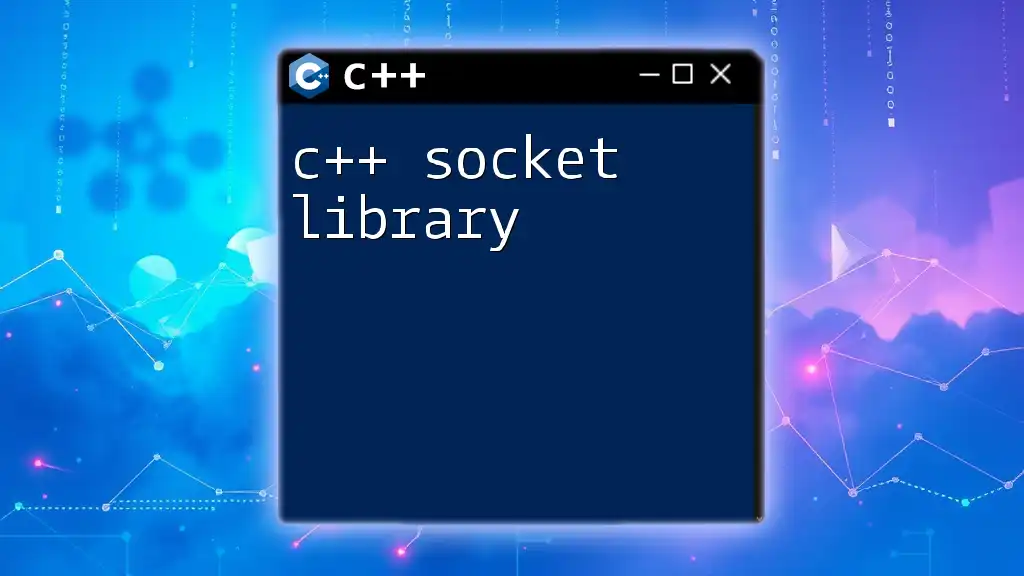
Formatting Output with cout
Manipulating Output with Manipulators
Manipulators provide control over the output format in the `cout` library. Here are some common ones:
-
`std::endl`: Ends the current line and flushes the output buffer.
-
`std::fixed` and `std::setprecision()`: Useful for formatting floating-point numbers to a specific number of decimal places.
Here's an example demonstrating the use of `std::setprecision()`:
#include <iomanip> // Required for std::setprecision
std::cout << std::fixed << std::setprecision(2);
std::cout << "Value: " << 3.14159 << std::endl;
Controlling Width and Alignment
When you want more control over how the output appears, you can use `std::setw()` (set width). This is extremely useful for aligning output in tabular form.
#include <iomanip> // Required for std::setw
std::cout << std::setw(10) << std::left << "Left" << std::endl;
std::cout << std::setw(10) << std::right << "Right" << std::endl;
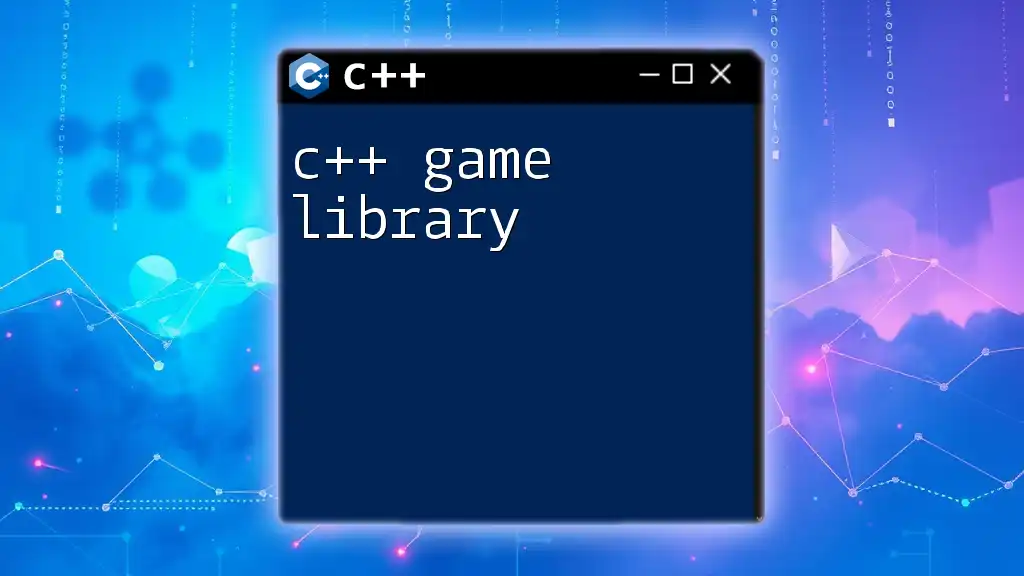
Error Handling in Output Operations
Understanding Stream States
Understanding the state of your output stream is crucial for reliable error handling. C++ offers various stream state flags, such as `good`, `bad`, `eof`, and `fail`. Here’s how you can check the state of `cout`:
if (std::cout.good()) {
std::cout << "Stream is good!" << std::endl;
}
Handling Errors Graciously
Sometimes, even the simplest output can fail. This is where error handling becomes important. The `std::cerr` stream is often used for outputting error messages. If an error occurs with `cout`, you can check the status and act upon it:
if (!std::cout) {
std::cerr << "Output error occurred!" << std::endl;
}
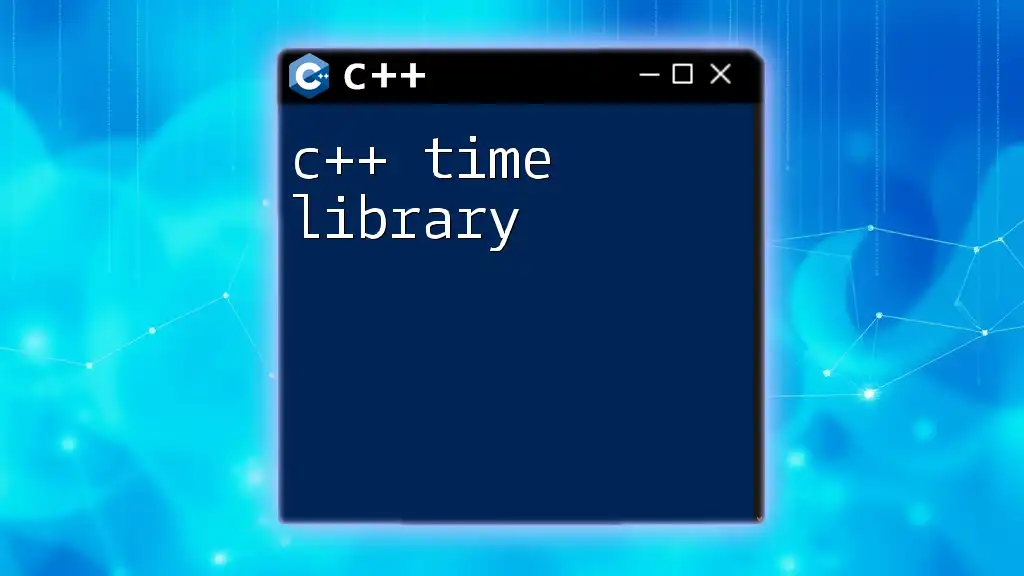
Advanced Features of the c++ cout Library
Using cout with Custom Types
One of the advanced functionalities of the `cout` library is its ability to work with user-defined types. This requires you to overload the `<<` operator, allowing `cout` to understand how to output your custom types. Here’s a simple illustration:
class Person {
public:
std::string name;
int age;
};
std::ostream& operator<<(std::ostream& os, const Person& p) {
return os << "Name: " << p.name << ", Age: " << p.age;
}
// Usage
Person john{"John", 30};
std::cout << john << std::endl;
This overload enables seamless integration of custom classes with `cout`.
Streams and Buffering
Understanding how data is buffered within output streams is essential for effective programming. Buffers temporarily hold data before it's actually sent to the output device. You can force the flush operation to ensure the data is output immediately:
std::cout << "Buffering demonstration" << std::flush; // Flushes buffer immediately
Using flush judiciously can enhance performance in real-time output scenarios.
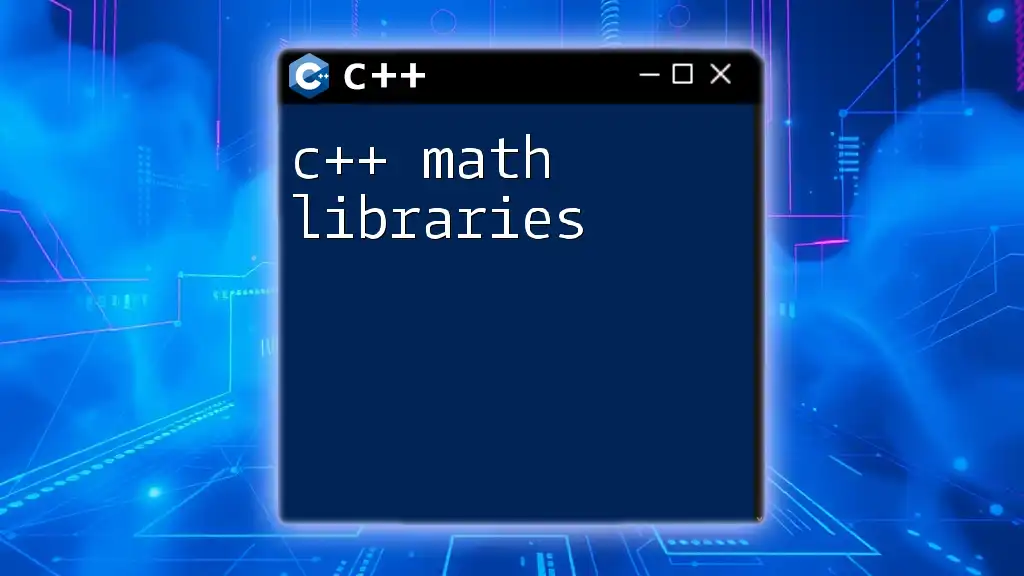
Conclusion
The c++ cout library is an invaluable asset in C++ programming, providing a simple yet powerful framework for outputting information to the console. From basic text and variable output to advanced formatting and custom types, `cout` equips developers with versatile tools essential for effective programming. Take time to experiment and explore the plethora of capabilities that the `cout` library offers; your understanding will significantly improve as you engage with practical exercises and real-world applications.