The C++ graph library provides data structures and algorithms for representing and manipulating graphs, enabling efficient implementation of graph-related tasks such as traversal and pathfinding.
Here’s a simple example using the Boost Graph Library to create a directed graph:
#include <iostream>
#include <boost/graph/adjacency_list.hpp>
int main() {
using namespace boost;
typedef adjacency_list<vecS, vecS, directedS> Graph;
Graph g;
add_edge(0, 1, g);
add_edge(1, 2, g);
add_edge(2, 0, g);
std::cout << "Edges in the graph:\n";
for (auto edge : boost::make_iterator_range(edges(g))) {
std::cout << source(edge, g) << " -> " << target(edge, g) << "\n";
}
return 0;
}
What is a Graph?
A graph is a fundamental data structure in computer science, composed of a set of vertices (or nodes) and a set of edges (or links) that connect pairs of vertices. Graphs can be classified into several types:
- Directed vs. Undirected: In a directed graph, edges have a direction, indicating a one-way relationship, while in an undirected graph, the relationship is bidirectional.
- Weighted vs. Unweighted: Weighted graphs assign a numerical value (weight) to each edge, useful for representing costs or distances, whereas unweighted graphs treat edges equally with no associated value.
Understanding the structure of graphs is essential because they are widely used to model relationships in various domains including social networks, transportation systems, and more.
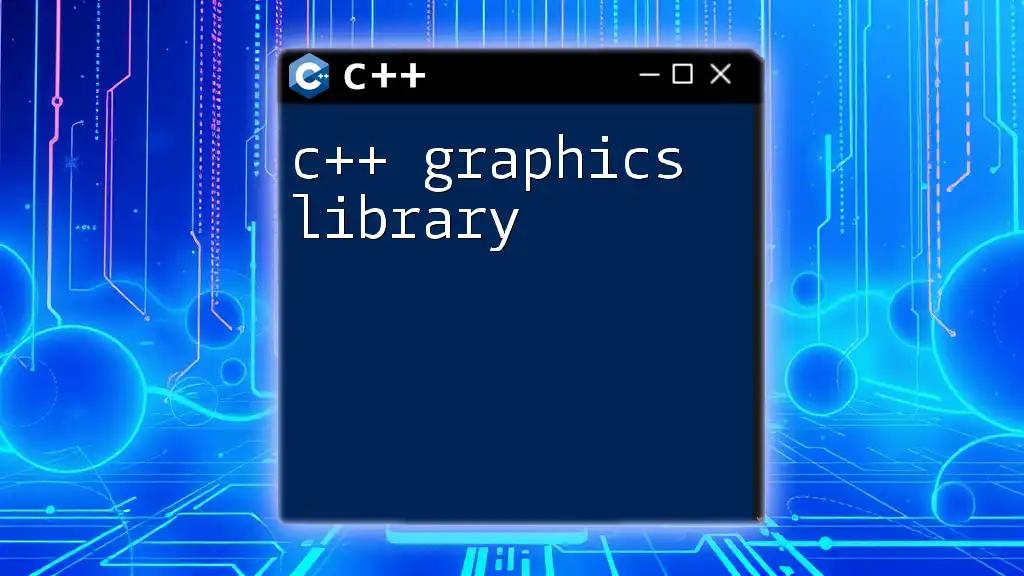
What is a Graph Library?
A graph library is a collection of pre-written code that provides various functionalities to create, manipulate, and operate on graphs. By leveraging a graph library, developers can save time and resources, as they do not need to implement common graph algorithms from scratch.
Importantly, graph libraries in C++ come with optimized implementations, allowing for efficient performance which is critical when dealing with large graphs.
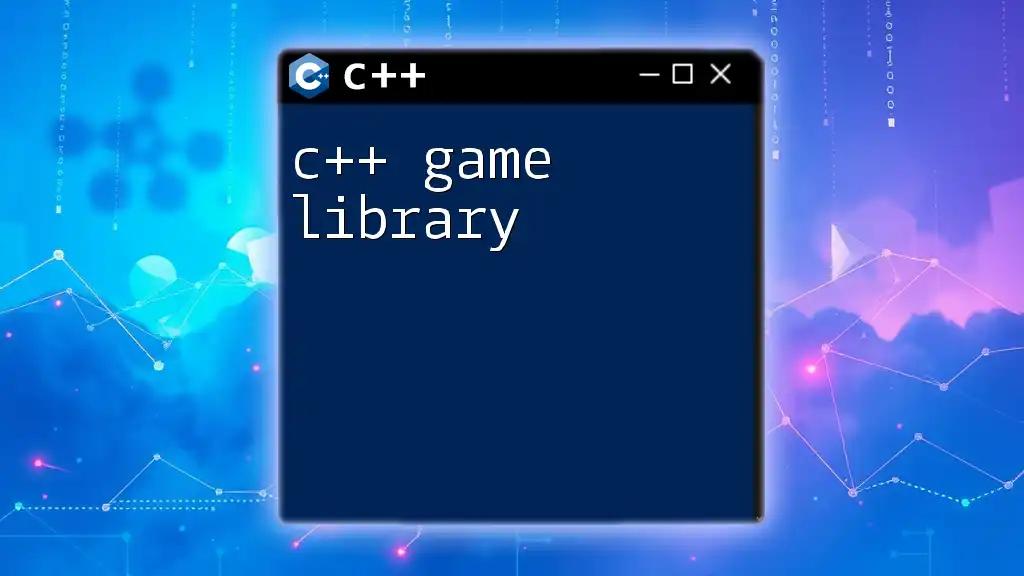
Why Use a C++ Graph Library?
Benefits of C++ for Graph Algorithms
C++ is a powerful programming language known for its high performance and ability to handle complex data structures effectively. Its object-oriented features support data abstraction, making it easier to model graph concepts.
Common Use Cases for Graph Libraries
Graph libraries can be employed in numerous applications, including:
- Social Networks: Modeling user connections and interactions.
- Pathfinding Algorithms: Optimizing routes in mapping and navigation systems.
- Network Flow Problems: Managing and optimizing resource flow in networks.
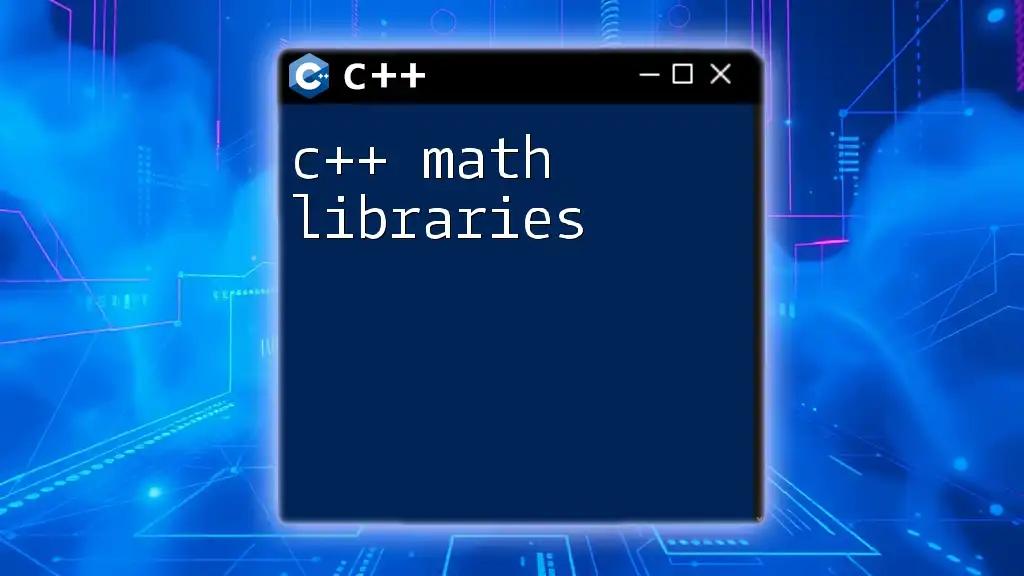
Popular C++ Graph Libraries
Boost Graph Library (BGL)
The Boost Graph Library is one of the most widely used C++ graph libraries, known for its flexibility and robustness. BGL supports various graph representations and a plethora of algorithms.
To get started, first install the Boost library and include the necessary headers. Here’s a simple example of creating a graph:
#include <boost/graph/adjacency_list.hpp>
using namespace boost;
int main() {
// Define a graph type
typedef adjacency_list<> Graph;
// Create a graph object
Graph g;
// Add an edge between nodes 0 and 1
add_edge(0, 1, g);
return 0;
}
This snippet demonstrates the basic construction of a graph using an adjacency list representation.
Graph-tool
Graph-tool is another powerful library that is notable for its performance in analyzing large graphs. Although it requires Python bindings, its efficient C++ core offers substantial speed advantages for computation-heavy tasks.
LEDA
LEDA (Library of Efficient Data types and Algorithms) combines a collection of efficient algorithms with data structures for graph-related applications. It is especially beneficial for academic endeavors concerning graph theory and complex algorithms. Installation procedures vary based on the licensing model, and users are encouraged to explore its vast feature set.
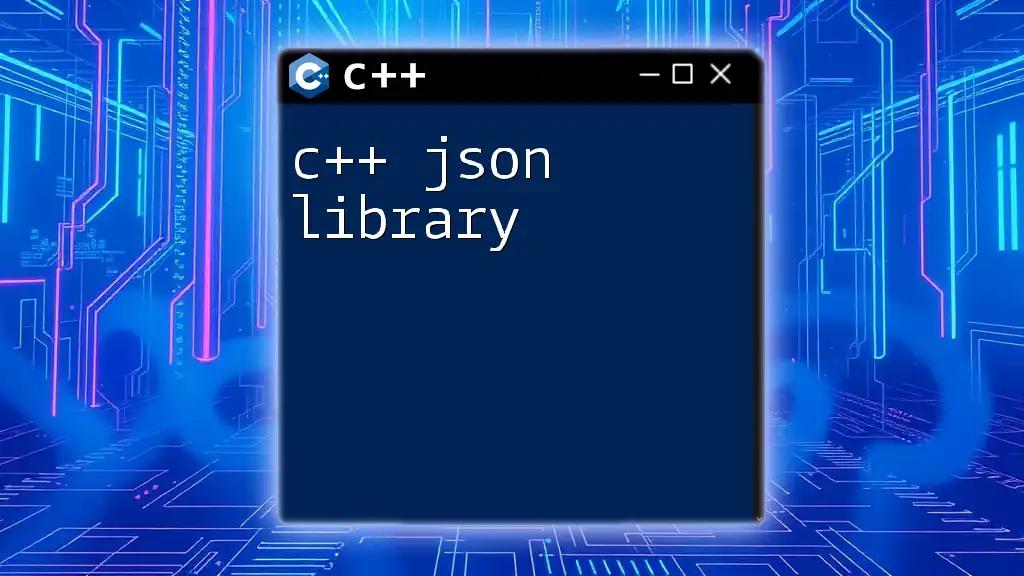
Creating and Managing Graphs in C++
Data Structures for Graph Representation
Adjacency Matrix
An adjacency matrix is a 2D array used to represent a graph, where each cell at position (i, j) indicates whether there is an edge between vertex i and vertex j.
Pros: Works well for dense graphs (lots of edges).
Cons: Memory-intensive, especially for large graphs.
Here’s a simple illustration of using an adjacency matrix:
class Graph {
private:
vector<vector<int>> adjMatrix;
public:
Graph(int vertices) {
adjMatrix.resize(vertices, vector<int>(vertices, 0));
}
void addEdge(int start, int end) {
adjMatrix[start][end] = 1; // Assume simple unweighted graph
}
};
Adjacency List
An adjacency list is a more space-efficient way to represent a graph, particularly beneficial for sparse graphs. Each vertex maintains a list of its adjacent vertices.
Pros: Uses less memory in sparse graphs.
Cons: Slight overhead when checking for the existence of edges.
Example of an adjacency list representation:
class Graph {
private:
list<int>* adjList;
int vertices;
public:
Graph(int v) {
vertices = v;
adjList = new list<int>[v];
}
void addEdge(int start, int end) {
adjList[start].push_back(end); // Add edge to the list
}
};
Adding and Removing Nodes/Edges
To effectively manage a graph, you need functions to add and remove nodes and edges. In both adjacency matrices and lists, the respective methods can be defined.
For instance, an addEdge method might look like:
void addEdge(int start, int end) {
// Implementation specific to the structure used (matrix or list)
}
Similarly, a removeEdge method will involve checking the structure and removing the connection as necessary.
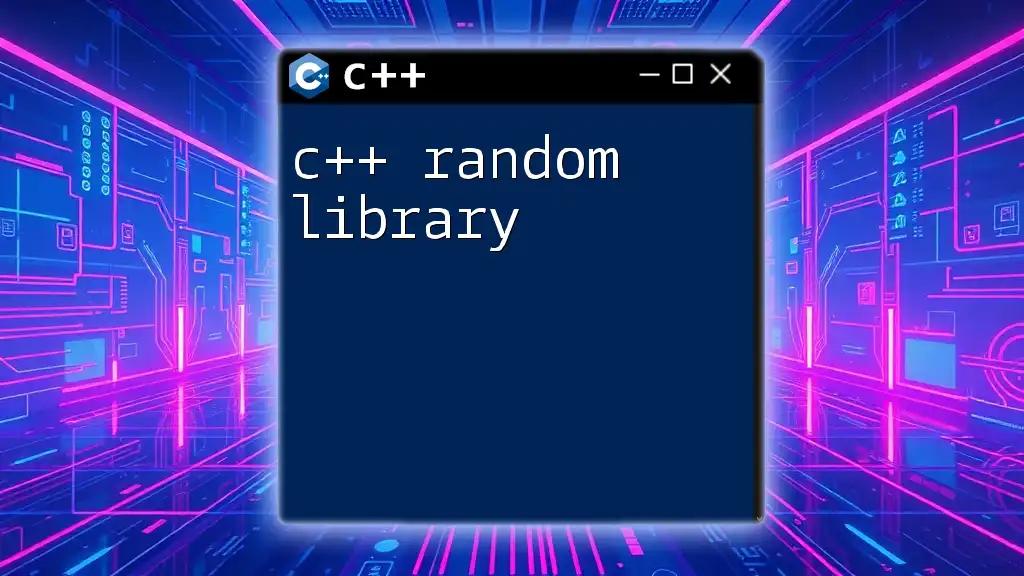
Graph Algorithms in C++
Traversal Algorithms
Depth-First Search (DFS)
DFS is a traversal algorithm that explores as far as possible along a branch before backtracking. It's valuable for pathfinding and connected component identification.
Here's a simple implementation:
void DFS(int vertex, vector<bool>& visited) {
visited[vertex] = true;
// Explore neighbors...
for (int neighbor : adjList[vertex]) {
if (!visited[neighbor]) {
DFS(neighbor, visited);
}
}
}
Breadth-First Search (BFS)
BFS, on the other hand, explores all neighbors at the present depth prior to moving on to nodes at the next depth level. It is often used for finding the shortest path in unweighted graphs.
Example of a BFS implementation:
void BFS(int start) {
queue<int> q;
vector<bool> visited(vertices, false);
q.push(start);
visited[start] = true;
while (!q.empty()) {
int current = q.front();
q.pop();
// Process the current node...
for (int neighbor : adjList[current]) {
if (!visited[neighbor]) {
visited[neighbor] = true;
q.push(neighbor);
}
}
}
}
Shortest Path Algorithms
Dijkstra’s Algorithm
Dijkstra’s algorithm finds the shortest path from a source vertex to all other vertices in a weighted graph. It works by iterating over vertices, updating their distance as shorter paths are found.
Bellman-Ford Algorithm
This algorithm can handle graphs with negative weight edges. Bellman-Ford uses relaxation techniques on all edges in the graph repeatedly to find the shortest paths.
Sample implementation of Dijkstra’s might look like:
void dijkstra(int source) {
vector<int> dist(vertices, INT_MAX);
dist[source] = 0;
// Implement priority queue and loop through nodes...
}
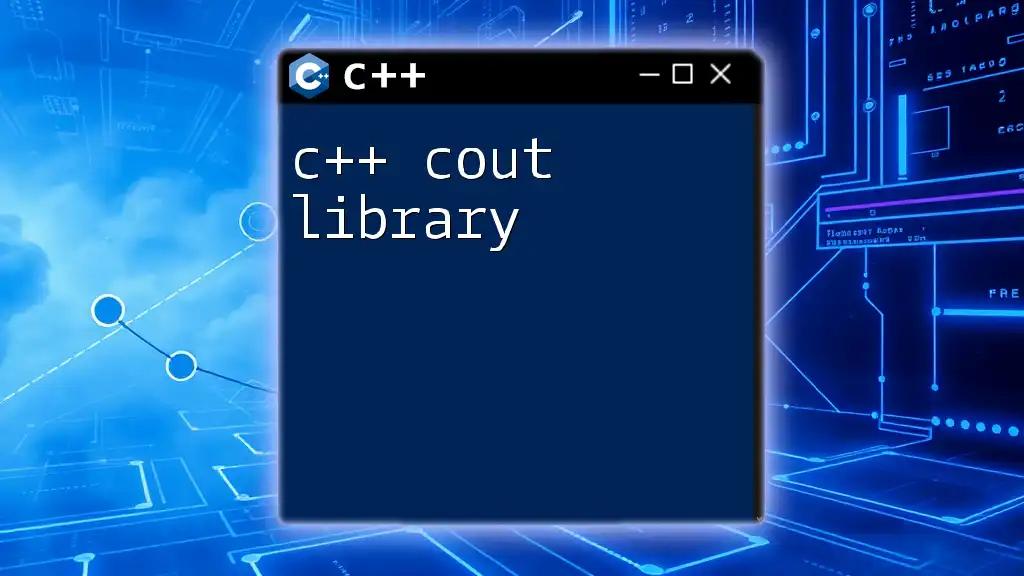
Visualizing Graphs in C++
Importance of Visualization
Visualizing graphs is crucial as it helps in understanding complex relationships and structures more intuitively. Graphs can exhibit intricate patterns that are often easier to comprehend through visual means.
Integrating Visualization Libraries
C++ provides several libraries for visualizing graphs, one of which is Graphviz. The integration facilitates the generation of visual illustrations based on graph descriptions, enhancing the ability to convey complex information simply.
An example of code to generate a graphical representation would look like this:
#include <graphviz/gvc.h>
// Typical code to create and render graph using Graphviz...
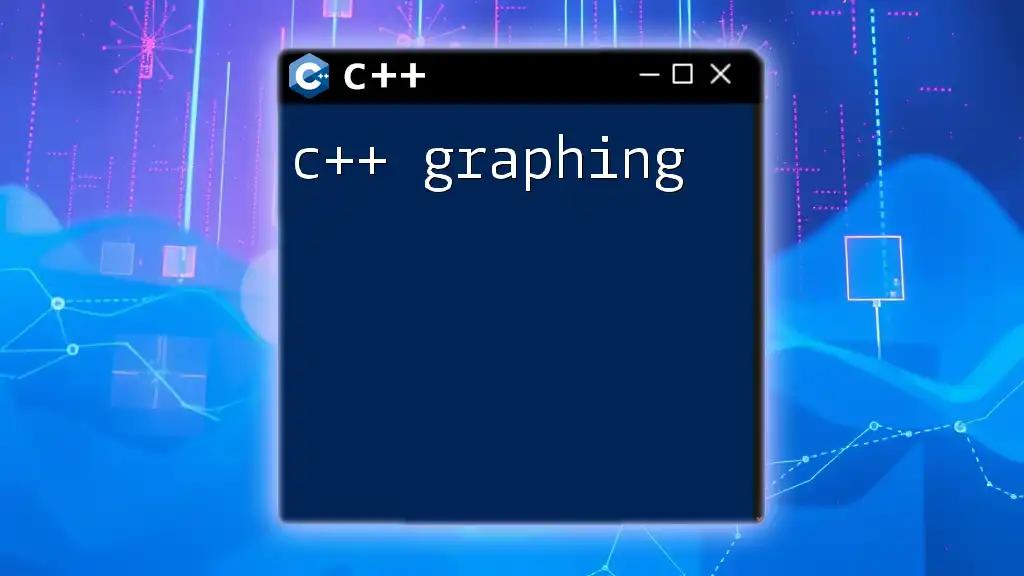
Conclusion
Using a C++ graph library empowers developers to engage with graph theory effectively. Leveraging existing libraries like Boost, Graph-tool, and LEDA, one can handle complex graph structures and algorithms efficiently. As graphs play an essential role in diverse real-world applications, investing time in understanding these libraries is invaluable for programmers. Whether you're handling network designs, optimizing data flows, or exploring social interactions, mastering graph libraries can unlock new possibilities in your C++ programming journey.
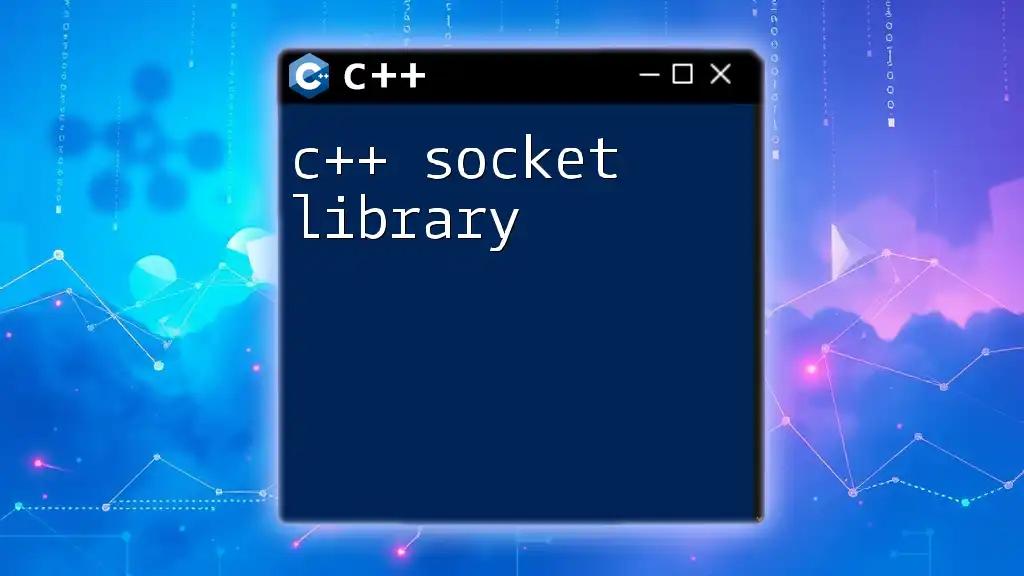
Additional Resources
To expand your knowledge further, consider exploring documentation, tutorials specific to each library, and recommended books focused on graph theory and C++ programming. Online courses and workshops can also provide structured learning opportunities to deepen your understanding and practical skills in utilizing C++ graph libraries.